- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Writing Async Tests in Flutter Using Mockito
Master mocking techniques for API calls, streams, and error handling with practical examples.
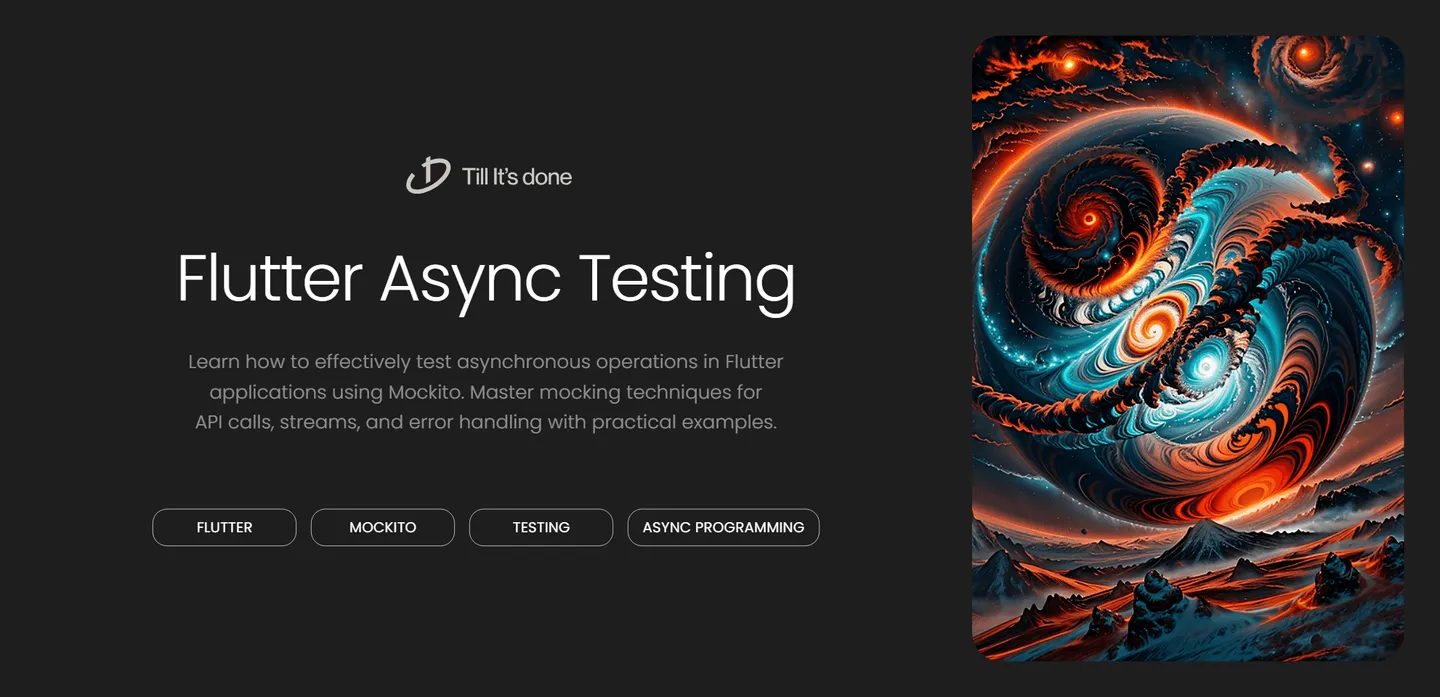
Writing Async Tests in Flutter Using Mockito
Testing asynchronous operations in Flutter can be tricky, but with Mockito, we can make our lives much easier. Today, let’s dive into how to write effective async tests that will help keep your Flutter applications robust and reliable.
Why Mock Async Operations?
When testing our apps, we often deal with operations that depend on external services - API calls, database operations, or file system interactions. These operations are inherently unpredictable and can make our tests flaky. This is where Mockito comes to the rescue.
Setting Up Your Testing Environment
First things first, let’s add the necessary dependencies to your pubspec.yaml
:
dev_dependencies: mockito: ^5.4.0 build_runner: ^2.4.0
Creating Your First Async Mock
Let’s say we have a simple API service that fetches user data. Here’s how we can mock it:
@GenerateMocks([ApiService])void main() { late MockApiService mockApiService; late UserBloc userBloc;
setUp(() { mockApiService = MockApiService(); userBloc = UserBloc(mockApiService); });
test('fetches user data successfully', () async { when(mockApiService.fetchUserData()) .thenAnswer((_) async => UserModel(id: '1', name: 'John'));
await userBloc.fetchUser(); verify(mockApiService.fetchUserData()).called(1); });}
Advanced Mocking Techniques
Sometimes we need to mock more complex scenarios, like network failures or timeout situations:
test('handles network error gracefully', () async { when(mockApiService.fetchUserData()) .thenThrow(NetworkException('No internet connection'));
expect(() => userBloc.fetchUser(), throwsA(isA<NetworkException>()));});
Best Practices for Async Testing
- Always use
async/await
in your test cases - Don’t forget to handle timeouts
- Mock all external dependencies
- Verify important interactions
- Test both success and failure scenarios
Testing Stream Operations
Flutter loves streams, and Mockito handles them beautifully:
test('emits correct user states', () async { when(mockApiService.userStream()) .thenAnswer((_) => Stream.fromIterable([user1, user2, user3]));
expect( userBloc.userStream, emitsInOrder([user1, user2, user3]), );});
Conclusion
Testing async operations doesn’t have to be intimidating. With Mockito, we can create predictable, reliable tests that give us confidence in our code’s behavior under various conditions.
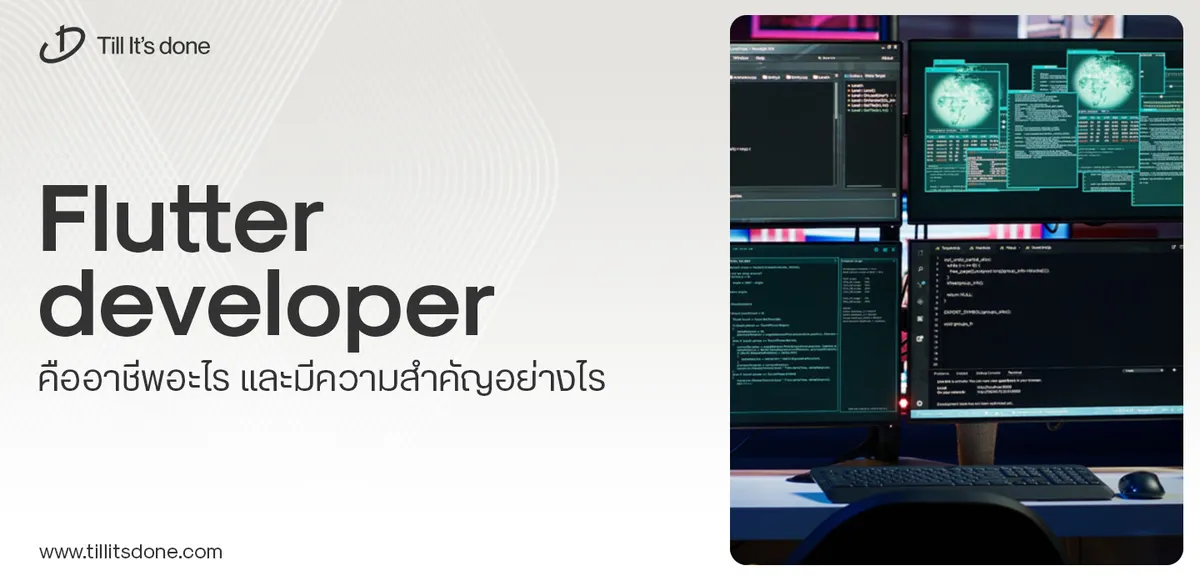
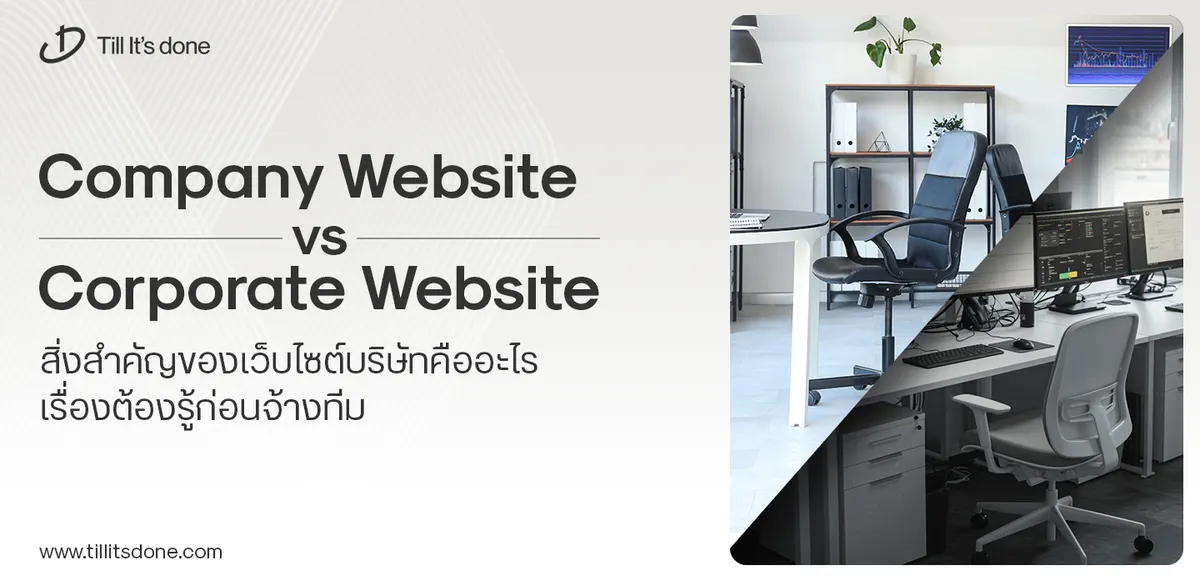
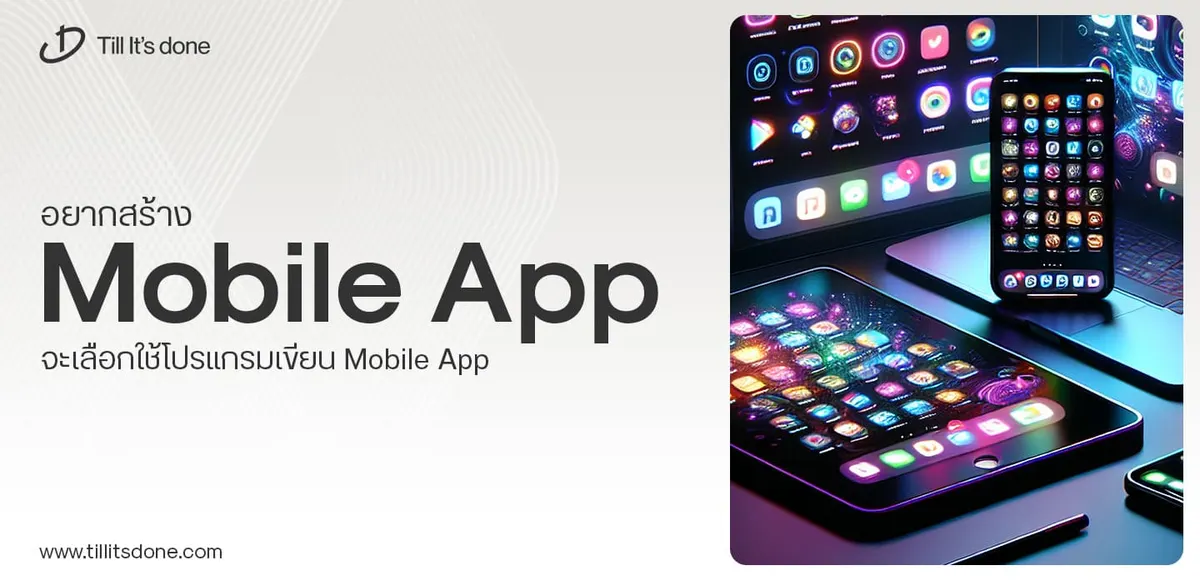
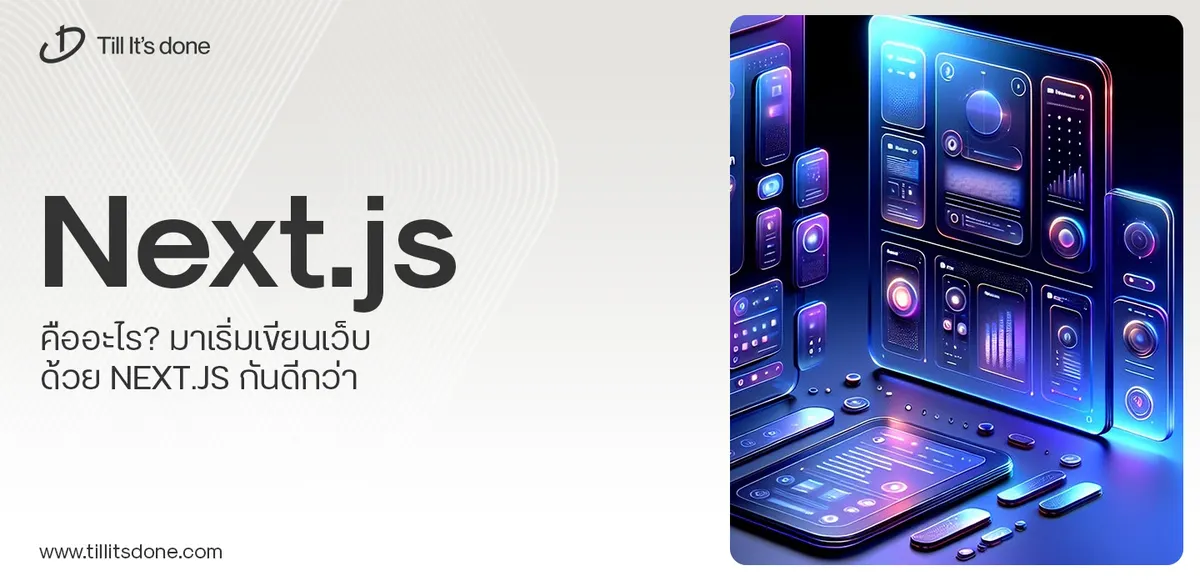
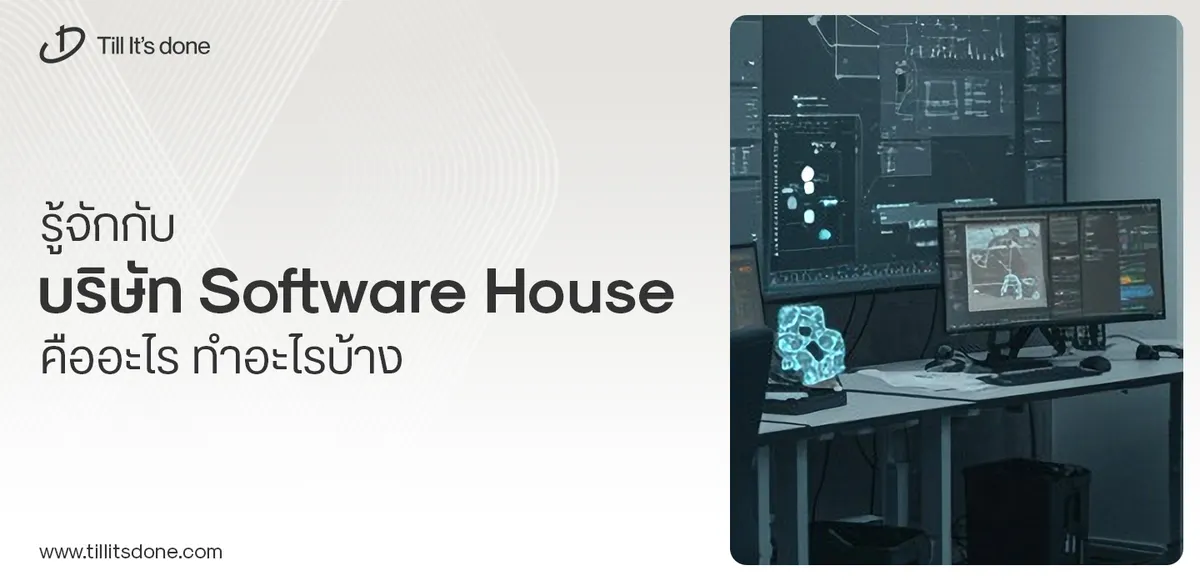
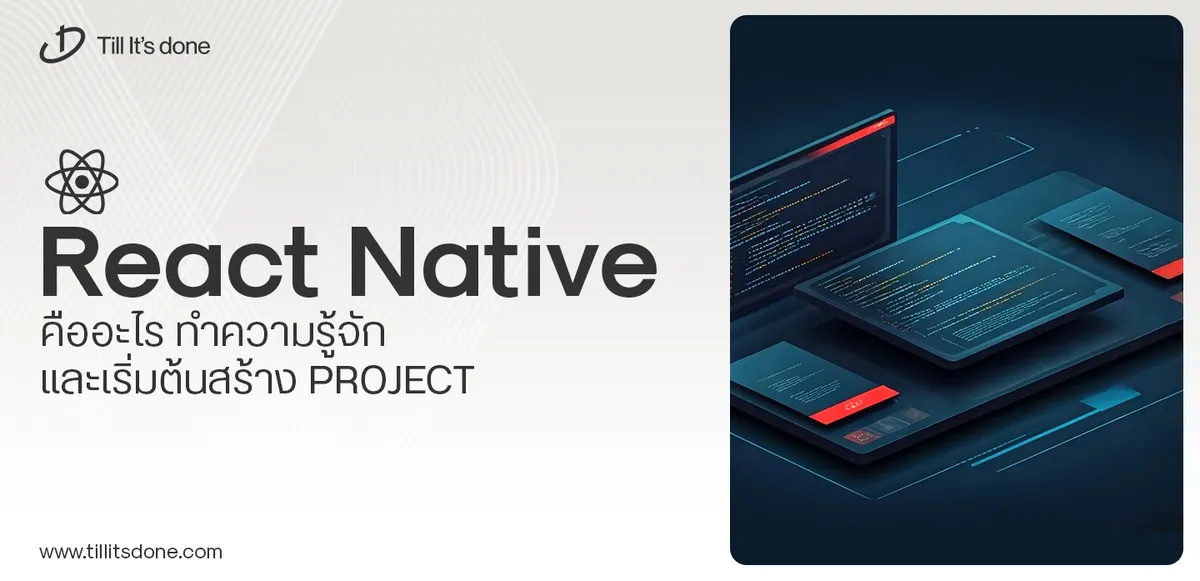
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.