- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for Mocking APIs with Mockito
Discover how to write maintainable tests, handle errors, and avoid common pitfalls in your Flutter applications.

Best Practices for Mocking APIs with Mockito in Flutter
Testing is a crucial part of building robust Flutter applications, and when it comes to testing API interactions, Mockito is your best friend. Let’s dive into some best practices that will help you write better, more maintainable tests using Mockito in Flutter.
Understanding the Basics
Before we jump into advanced techniques, let’s understand why we need mocking in the first place. When testing our apps, we don’t want to make actual API calls – they’re slow, unreliable, and can cost money. Instead, we want to simulate these interactions in a controlled environment. This is where Mockito shines.
Setting Up Your Testing Environment
First things first, you’ll need to add the right dependencies to your pubspec.yaml
:
dev_dependencies: mockito: ^5.4.0 build_runner: ^2.4.0
Best Practices for API Mocking
1. Create Interface for Your API Services
Always start by defining clear interfaces for your API services. This makes your code more testable and maintainable:
abstract class ApiService { Future<User> getUser(String id); Future<List<Post>> getPosts();}
2. Use @GenerateMocks Annotation
Let Mockito generate the mock classes for you:
@GenerateMocks([ApiService])void main() { // Your tests here}
3. Implement Proper Error Handling
Don’t just test the happy path. Make sure to test error scenarios too:
test('should handle API error gracefully', () async { when(mockApiService.getUser('123')) .thenThrow(Exception('Network error'));
expect(() => userBloc.fetchUser('123'), throwsException);});
4. Verify API Calls
Always verify that your mock methods are called as expected:
verify(mockApiService.getUser('123')).called(1);
5. Reset Mocks Between Tests
Keep your tests isolated by resetting mocks:
setUp(() { mockApiService = MockApiService();});
Advanced Techniques
Stubbing Sequential Responses
Sometimes you need your mock to return different values on successive calls:
when(mockApiService.getStatus()) .thenAnswer((_) => Future.value('pending')) .thenAnswer((_) => Future.value('completed'));
Argument Matchers
Use argument matchers for more flexible testing:
when(mockApiService.getUser(any)) .thenAnswer((_) => Future.value(User()));
Common Pitfalls to Avoid
- Don’t overuse mocking – mock only what’s necessary
- Avoid complex mock setups that are hard to maintain
- Don’t forget to test error scenarios
- Keep your test cases focused and simple
By following these best practices, you’ll create more reliable and maintainable tests for your Flutter applications. Remember, good tests are an investment in your application’s future.





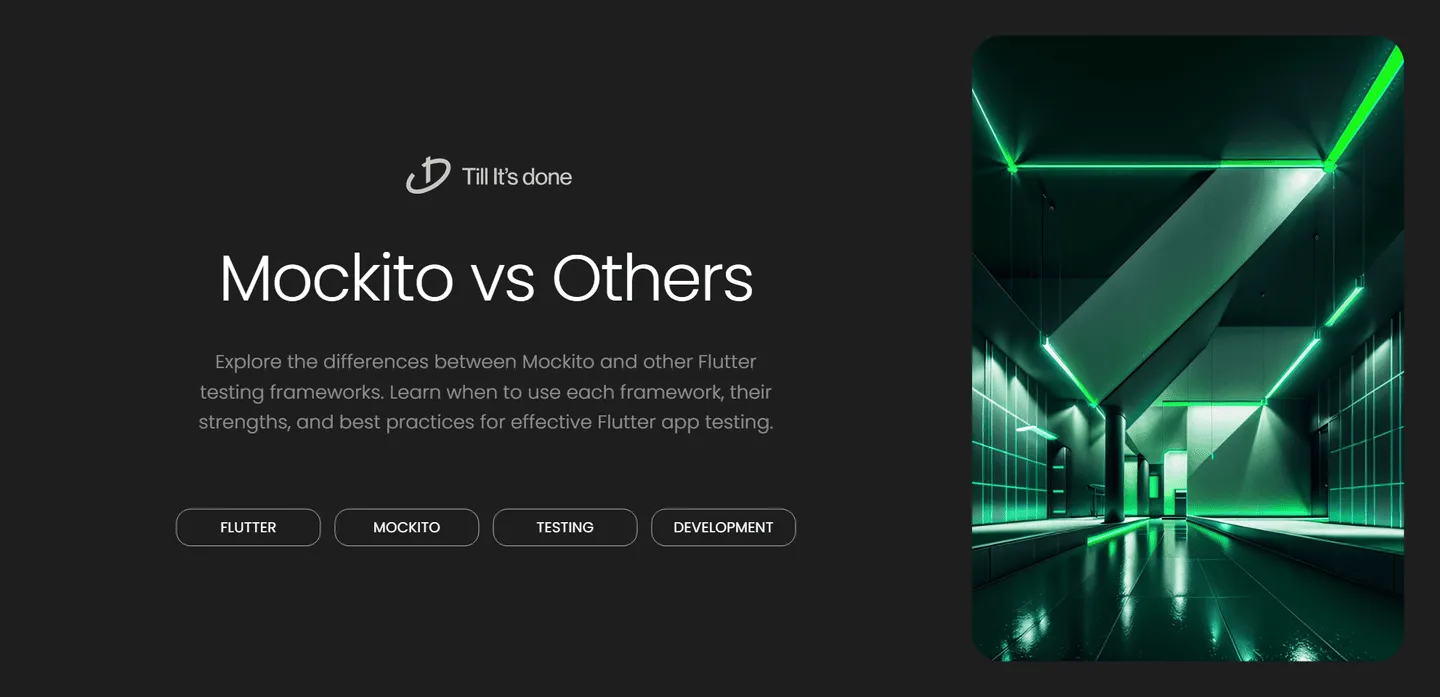
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.