- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Zod to Validate API Responses in React
Discover best practices for schema definition, error handling, and type safety in your React projects.
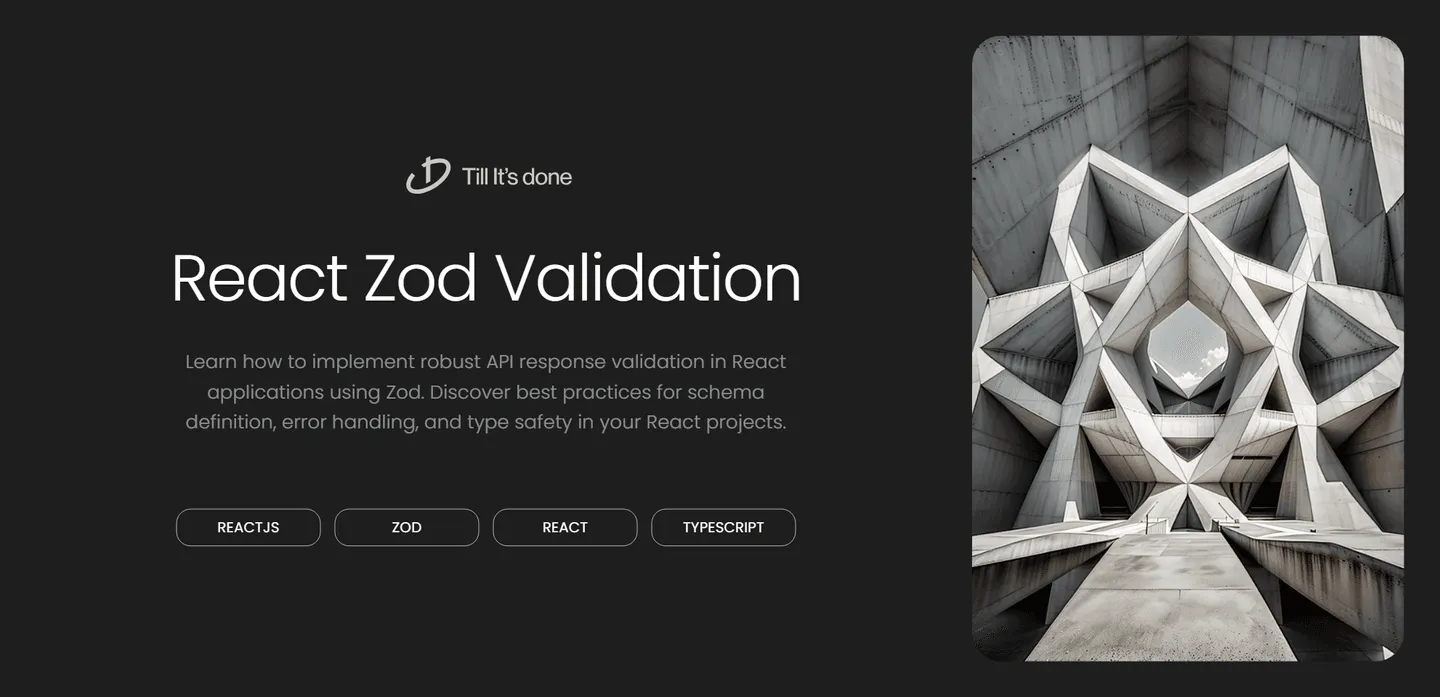
Using Zod to Define and Validate API Response Schemas in React
In the world of modern web development, type safety and runtime validation have become crucial aspects of building robust applications. While TypeScript provides excellent static type checking, we often need runtime validation for API responses. This is where Zod comes into play – a powerful schema declaration and validation library that works beautifully with React applications.
Why Zod?
Think of Zod as your data’s bouncer – it checks every piece of information that enters your application, ensuring it matches exactly what you expect. This becomes particularly valuable when dealing with external APIs where the response structure might not always be what we anticipate.
Setting Up Zod in Your React Project
First, let’s get our project set up with Zod. If you’re using npm, it’s as simple as:
npm install zod
Defining Your First Schema
Let’s say we’re building a weather application that fetches data from an API. Here’s how we might define our response schema:
import { z } from 'zod';
const WeatherDataSchema = z.object({ temperature: z.number(), conditions: z.string(), windSpeed: z.number(), humidity: z.number().min(0).max(100), forecast: z.array(z.object({ day: z.string(), high: z.number(), low: z.number(), }))});
type WeatherData = z.infer<typeof WeatherDataSchema>;
Implementing Validation in Your React Components
Here’s a practical example of how to use this schema in a React component:
const WeatherDisplay: React.FC = () => { const [weatherData, setWeatherData] = useState<WeatherData | null>(null); const [error, setError] = useState<string | null>(null);
useEffect(() => { const fetchWeather = async () => { try { const response = await fetch('api/weather'); const data = await response.json();
// This is where the magic happens const validatedData = WeatherDataSchema.parse(data); setWeatherData(validatedData); setError(null); } catch (err) { if (err instanceof z.ZodError) { setError('Invalid data format received from API'); console.error('Validation error:', err.errors); } else { setError('Failed to fetch weather data'); } } };
fetchWeather(); }, []);
// Rest of your component code};
Advanced Validation Techniques
Zod really shines when dealing with complex data structures. Let’s look at some more advanced features:
const UserSchema = z.object({ id: z.string().uuid(), email: z.string().email(), age: z.number().min(13).optional(), preferences: z.object({ theme: z.enum(['light', 'dark']), notifications: z.boolean(), }).nullable(), lastLogin: z.string().datetime(),});
Best Practices
- Define Schemas Once: Create a separate file for your schemas and reuse them across components.
- Use Type Inference: Leverage Zod’s type inference to maintain DRY code.
- Error Handling: Always implement proper error handling for validation failures.
- Composition: Break down complex schemas into smaller, reusable parts.
Performance Considerations
While Zod validation is generally fast, it’s still runtime validation. For optimal performance:
- Validate data as close to the API boundary as possible
- Cache validated results when appropriate
- Consider using partial validation for large datasets
Conclusion
By incorporating Zod into your React applications, you’re adding a powerful layer of runtime validation that complements TypeScript’s static typing. This combination helps catch potential issues early, making your applications more robust and maintainable.
Remember, the goal isn’t just to validate data – it’s to build confidence in your application’s data handling capabilities. With Zod, you’re taking a significant step toward that goal.
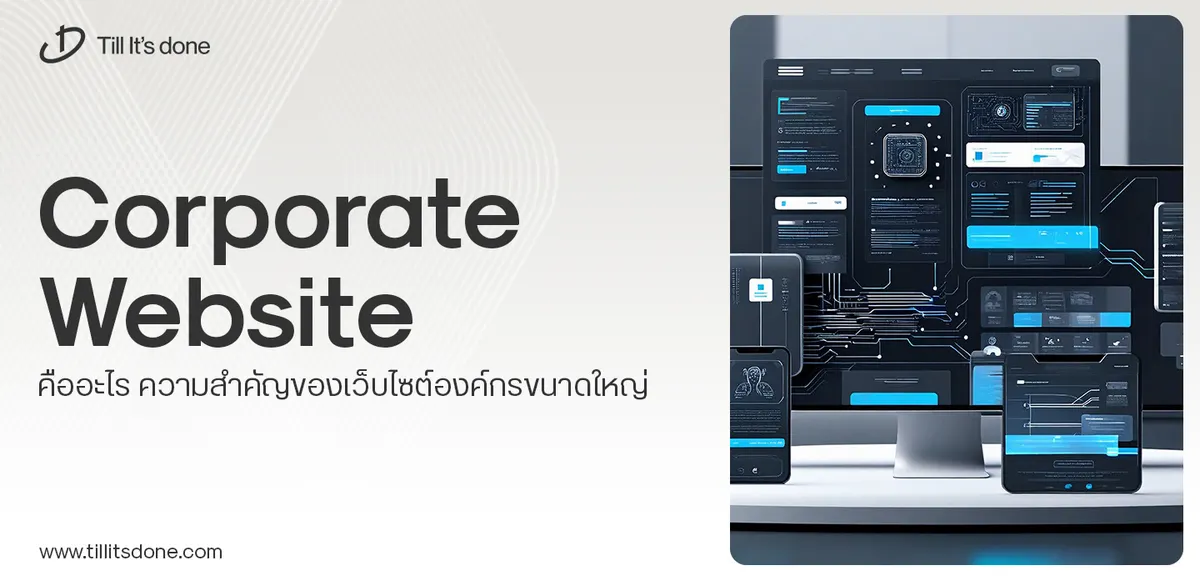
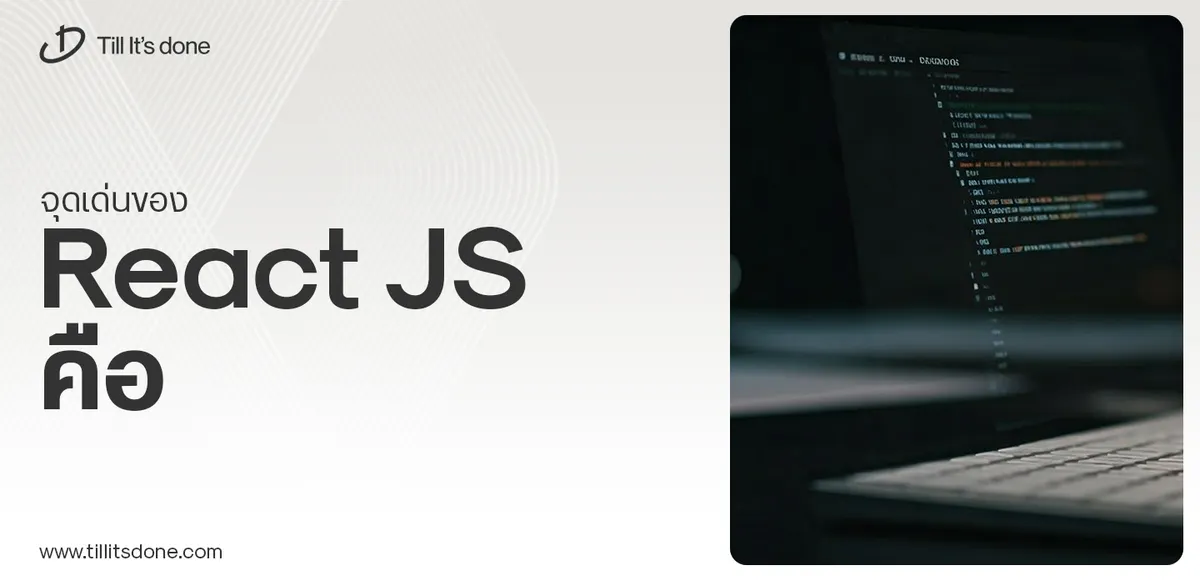
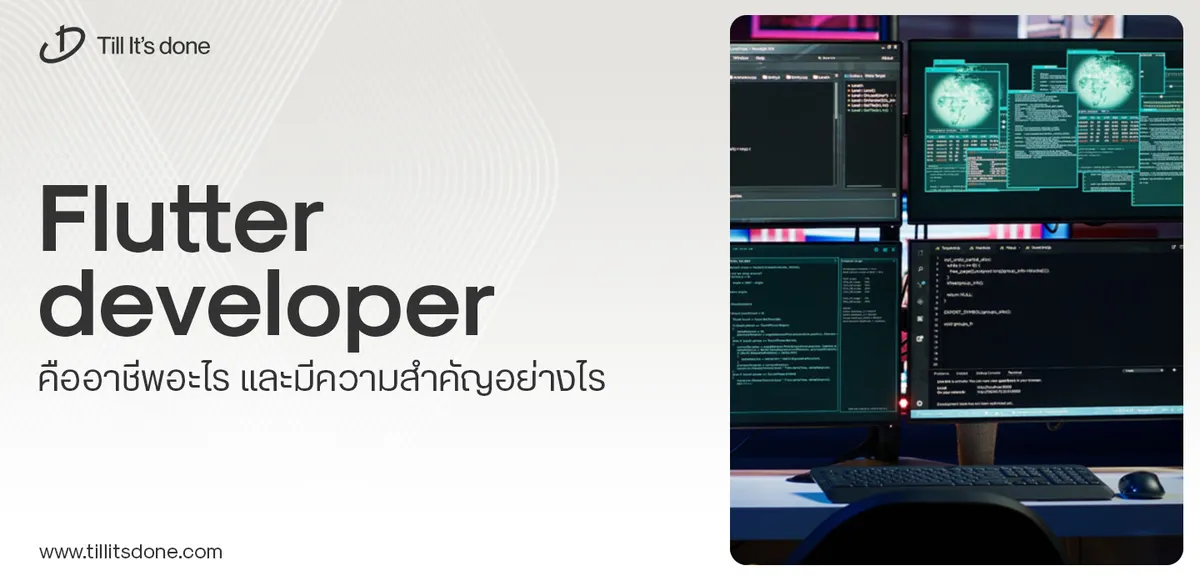
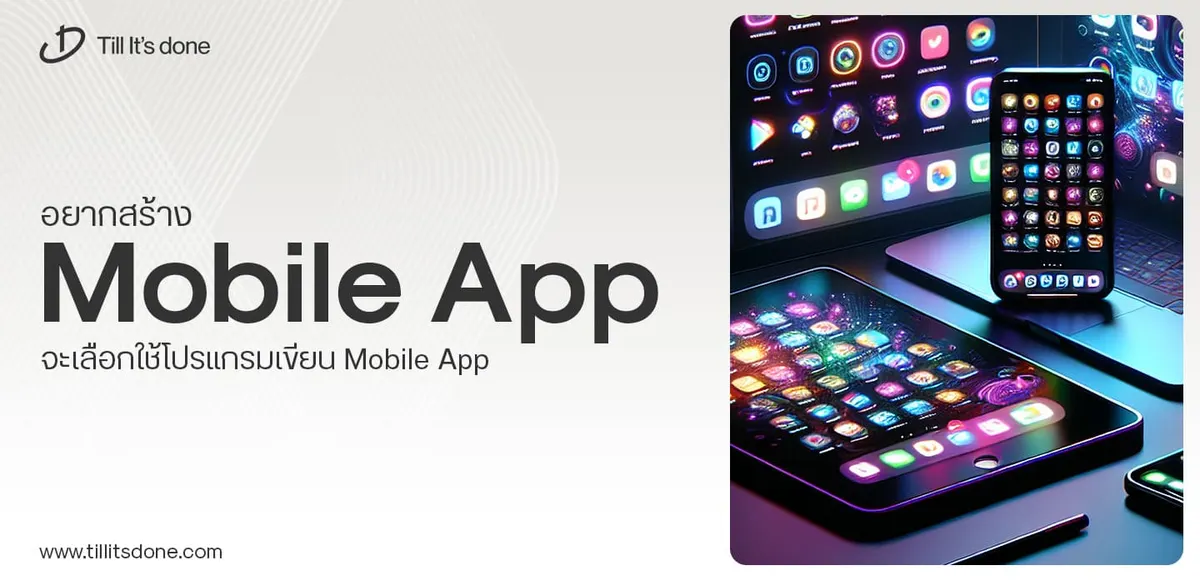
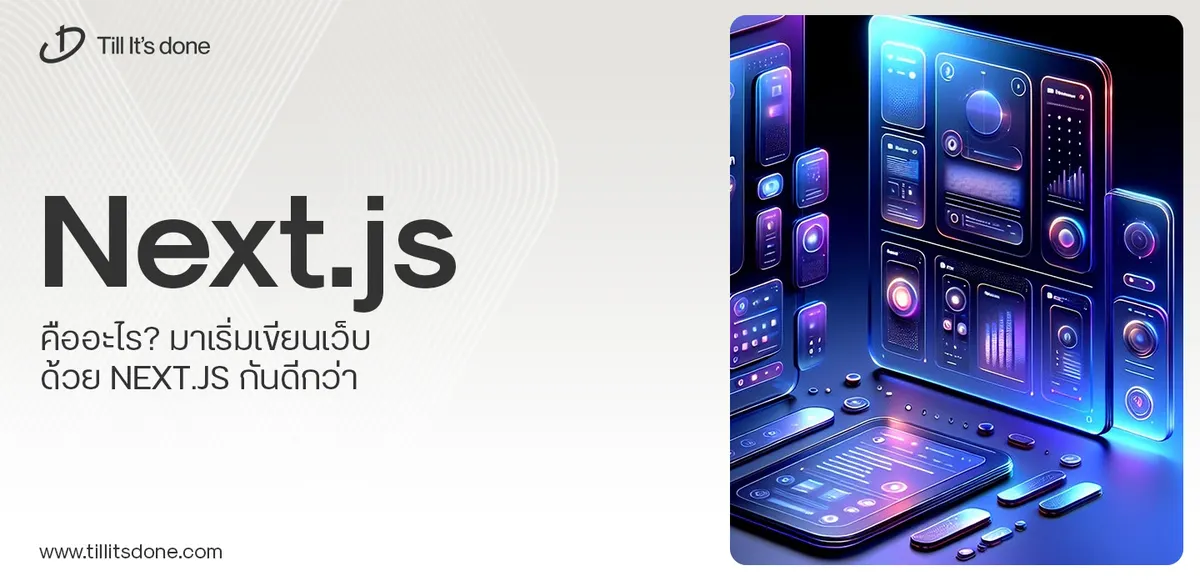
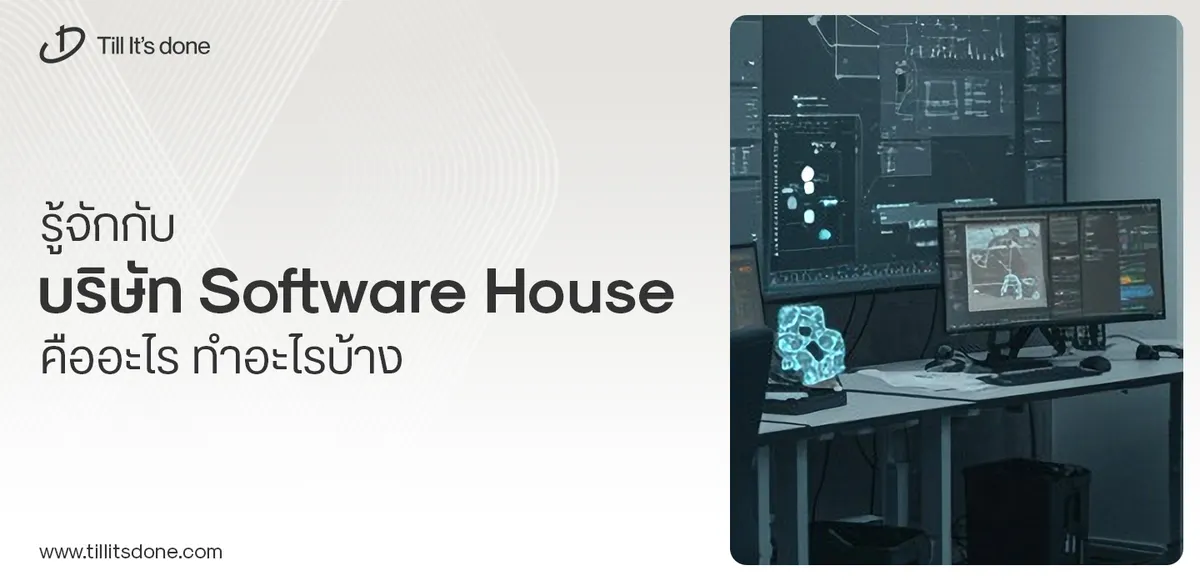
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.