- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Zod for Runtime Type Validation in React
Discover practical examples for form validation, API response handling, and best practices for type safety.

Using Zod for Runtime Type Validation in React
Have you ever found yourself debugging React components because of unexpected data shapes? Or spent hours adding type checks to ensure your forms handle user input correctly? If so, you’re not alone. While TypeScript provides excellent compile-time type checking, we often need runtime validation to ensure our applications handle data safely and reliably. This is where Zod comes in - a powerful validation library that makes runtime type checking in React applications a breeze.
Why Zod?
Before diving deep, let’s understand why Zod has become a go-to solution for React developers. Unlike traditional validation libraries, Zod provides a type-safe, schema-based approach that feels natural in the React ecosystem. It’s like having a security guard that not only checks IDs at compile-time but also verifies them when your application is running.
Getting Started with Zod
Setting up Zod in your React project is straightforward. First, install the package:
npm install zod# oryarn add zod
Let’s start with a simple example - validating a user registration form:
import { z } from 'zod';
const userSchema = z.object({ username: z.string().min(3).max(20), email: z.string().email(), age: z.number().min(18), password: z.string().min(8).regex(/[A-Z]/, 'Must contain at least one capital letter')});
type User = z.infer<typeof userSchema>;
Practical Applications
Form Validation
One of the most common use cases for Zod is form validation. Here’s how you can integrate it with a login form:
const LoginForm = () => { const [errors, setErrors] = useState<string[]>([]);
const handleSubmit = (formData: FormData) => { try { const validated = loginSchema.parse({ email: formData.get('email'), password: formData.get('password') }); // Proceed with login } catch (error) { if (error instanceof z.ZodError) { setErrors(error.errors.map(e => e.message)); } } }; // ... rest of the component};
API Response Validation
Another powerful use case is validating API responses. This ensures your application handles backend data safely:
const apiResponseSchema = z.object({ data: z.array(z.object({ id: z.number(), title: z.string(), completed: z.boolean() })), meta: z.object({ total: z.number(), page: z.number() })});
const fetchTodos = async () => { const response = await fetch('/api/todos'); const json = await response.json();
try { const validated = apiResponseSchema.parse(json); return validated; } catch (error) { console.error('Invalid API response:', error); throw new Error('Invalid API response'); }};
Best Practices and Tips
- Define Schemas Once: Create a central location for your schemas and reuse them across components.
- Compose Schemas: Take advantage of Zod’s composition features to build complex schemas from simpler ones.
- Custom Error Messages: Customize error messages to provide better user feedback.
- Type Inference: Use
z.infer<typeof schema>
to generate TypeScript types from your schemas.
Advanced Features
Zod offers several advanced features that can make your validation logic more powerful:
// Union typesconst statusSchema = z.union([ z.literal('pending'), z.literal('active'), z.literal('suspended')]);
// Optional fields with defaultsconst configSchema = z.object({ theme: z.string().default('light'), notifications: z.boolean().default(true)});
// Array validationconst todoListSchema = z.array(z.object({ title: z.string(), priority: z.number().min(1).max(5)})).min(1, 'List cannot be empty');
Conclusion
Zod brings peace of mind to React development by providing robust runtime type validation. Its TypeScript integration, intuitive API, and powerful features make it an excellent choice for ensuring type safety in your applications. Start small, perhaps with form validation, and gradually expand your usage as you become more comfortable with the library.
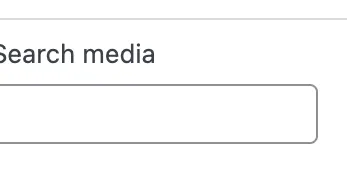





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.