- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Migrating PropTypes to Zod in React Apps
Discover the benefits and best practices for seamless migration.
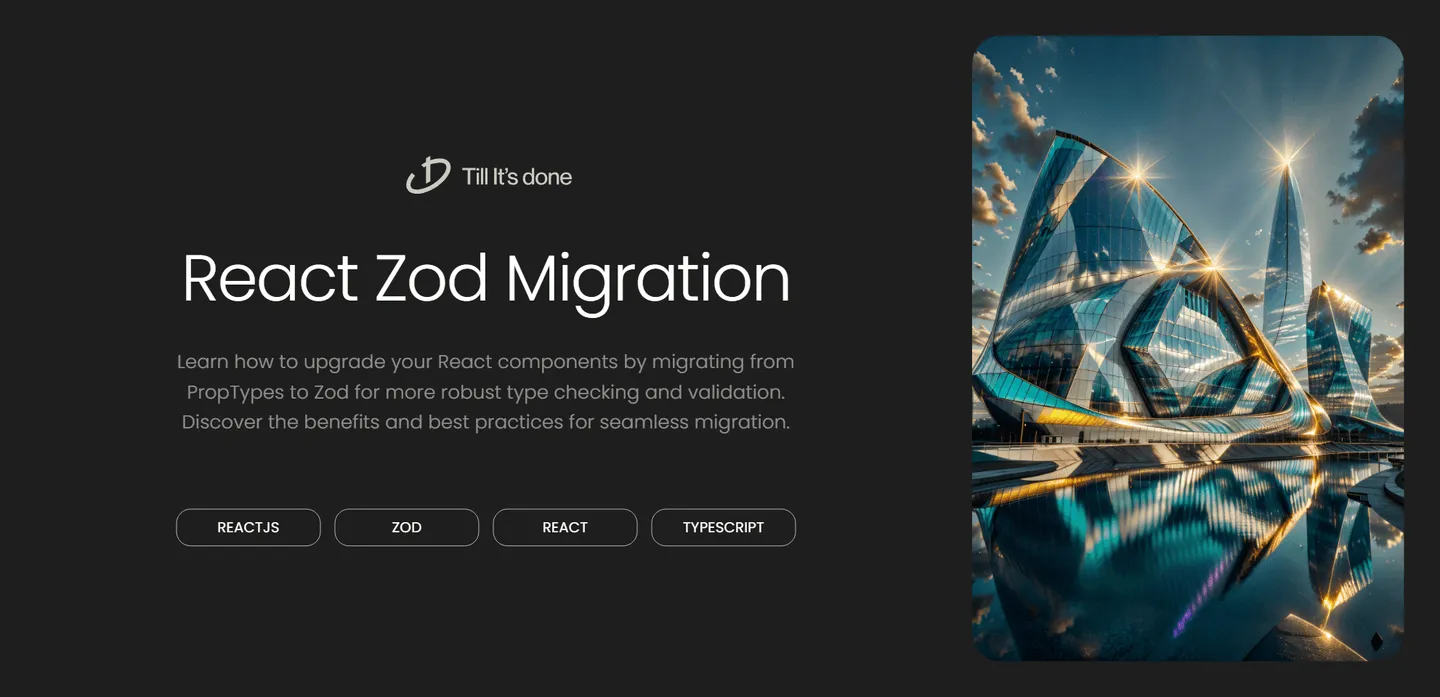
Migrating from PropTypes to Zod for Prop Validation in React
As React applications grow in complexity, robust type checking becomes increasingly crucial. While PropTypes has served us well, modern React development demands more powerful validation tools. Enter Zod – a TypeScript-first schema validation library that’s changing how we handle prop validation in React applications.
Why Make the Switch?
PropTypes has been a faithful companion for React developers, but it has limitations. It only performs runtime checks during development and offers basic type validation. Zod, on the other hand, brings a whole new level of type safety and validation capabilities to your React components.
The Migration Process
Let’s walk through a practical example of migrating from PropTypes to Zod. Here’s how we can transform a typical React component:
// Before - Using PropTypesimport PropTypes from 'prop-types';
const UserProfile = ({ name, age, email }) => { return ( <div> <h2>{name}</h2> <p>Age: {age}</p> <p>Email: {email}</p> </div> );};
UserProfile.propTypes = { name: PropTypes.string.isRequired, age: PropTypes.number.isRequired, email: PropTypes.string.isRequired,};
Now, let’s see how we can enhance this using Zod:
// After - Using Zodimport { z } from 'zod';
const userSchema = z.object({ name: z.string().min(1, 'Name is required'), age: z.number().min(0).max(120), email: z.string().email('Invalid email format')});
type UserProfileProps = z.infer<typeof userSchema>;
const UserProfile = ({ name, age, email }: UserProfileProps) => { return ( <div> <h2>{name}</h2> <p>Age: {age}</p> <p>Email: {email}</p> </div> );};
Benefits of Using Zod
-
Type Inference: Zod automatically generates TypeScript types from your schemas, eliminating the need to maintain separate type definitions.
-
Rich Validation Rules: Beyond basic type checking, Zod allows you to define complex validation rules, including custom error messages and transformations.
-
Runtime Safety: While TypeScript provides compile-time type checking, Zod ensures your data is valid at runtime too.
-
Composable Schemas: Zod makes it easy to compose and reuse validation schemas across your application.
Best Practices for Migration
- Start with smaller, less complex components
- Migrate one component at a time
- Use Zod’s parsing capabilities to validate data at API boundaries
- Keep validation logic centralized in schema files
- Leverage Zod’s error handling for better user feedback
Remember, migration doesn’t have to happen all at once. You can gradually transition your codebase, starting with the most critical components or newly developed features.






Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.