- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Test Custom Hooks with React Testing Library
Discover best practices, common patterns, and avoid pitfalls when writing tests for your custom hooks.
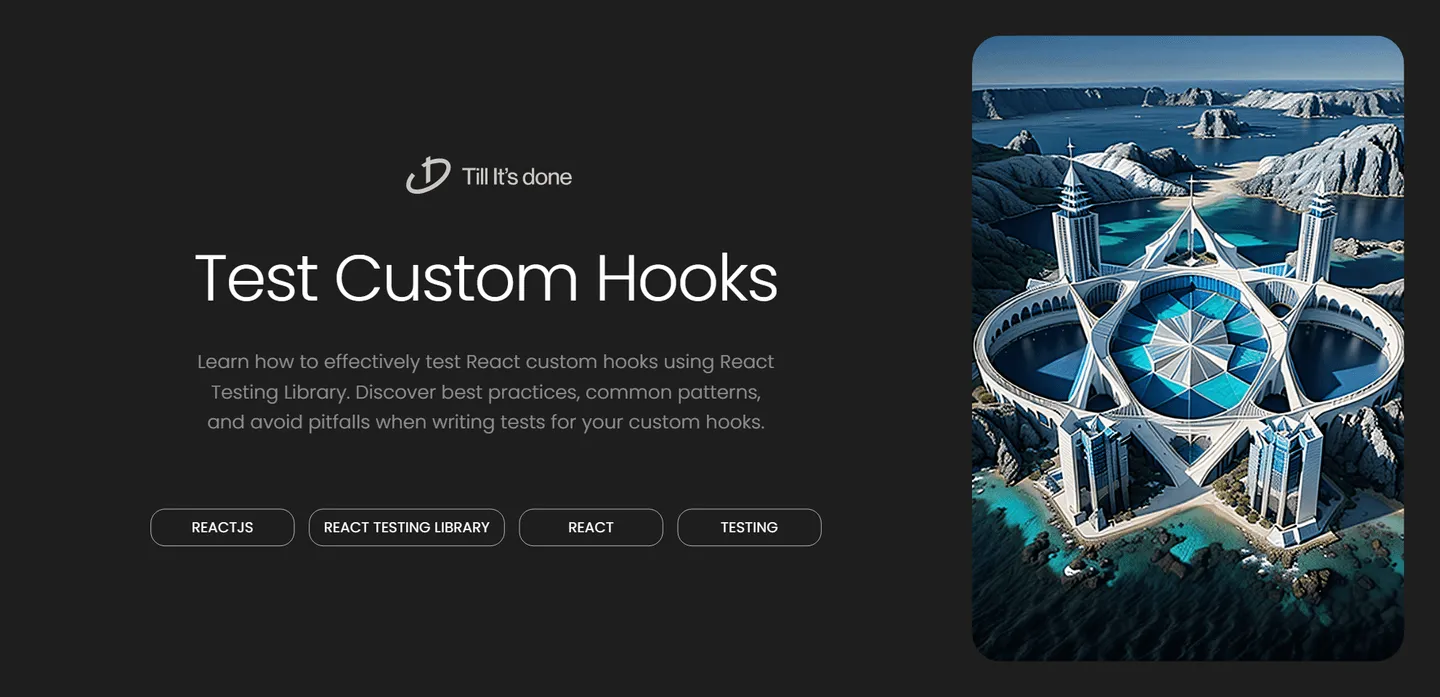
How to Test Custom Hooks with React Testing Library
Custom hooks are a powerful feature in React that allows us to extract component logic into reusable functions. But how do we ensure they work correctly? Let’s dive into testing custom hooks using React Testing Library.
Understanding the Basics
When testing custom hooks, we can’t test them directly since hooks can only be called inside React components. Instead, we need to create a test component that uses our hook. React Testing Library provides a special utility called renderHook
that makes this process straightforward.
Let’s start with a simple example. Imagine we have a custom hook that manages a counter:
import { useState } from 'react';
function useCounter(initialValue = 0) { const [count, setCount] = useState(initialValue);
const increment = () => setCount(prev => prev + 1); const decrement = () => setCount(prev => prev - 1);
return { count, increment, decrement };}
Writing Your First Hook Test
Here’s how we can test our useCounter
hook:
import { renderHook, act } from '@testing-library/react';import { useCounter } from './useCounter';
describe('useCounter', () => { test('should initialize with default value', () => { const { result } = renderHook(() => useCounter()); expect(result.current.count).toBe(0); });
test('should increment counter', () => { const { result } = renderHook(() => useCounter());
act(() => { result.current.increment(); });
expect(result.current.count).toBe(1); });});
Best Practices and Common Patterns
-
Always wrap state updates in
act()
Theact
utility ensures that all state updates are processed and applied before making assertions. -
Test initial states and props Make sure your hook initializes correctly with different props and default values.
-
Test error cases Don’t forget to test how your hook handles errors and edge cases.
Testing Complex Scenarios
Sometimes hooks interact with APIs or have complex state management. Here’s how to handle those cases:
test('should handle async operations', async () => { const { result } = renderHook(() => useDataFetching());
await act(async () => { await result.current.fetchData(); });
expect(result.current.data).toBeDefined();});
Common Pitfalls to Avoid
- Not cleaning up after tests
- Forgetting to wrap state updates in
act()
- Testing implementation details instead of behavior
- Not testing error states
Remember, the goal is to test the behavior of your hook from the perspective of the components that will use it, not its internal implementation.
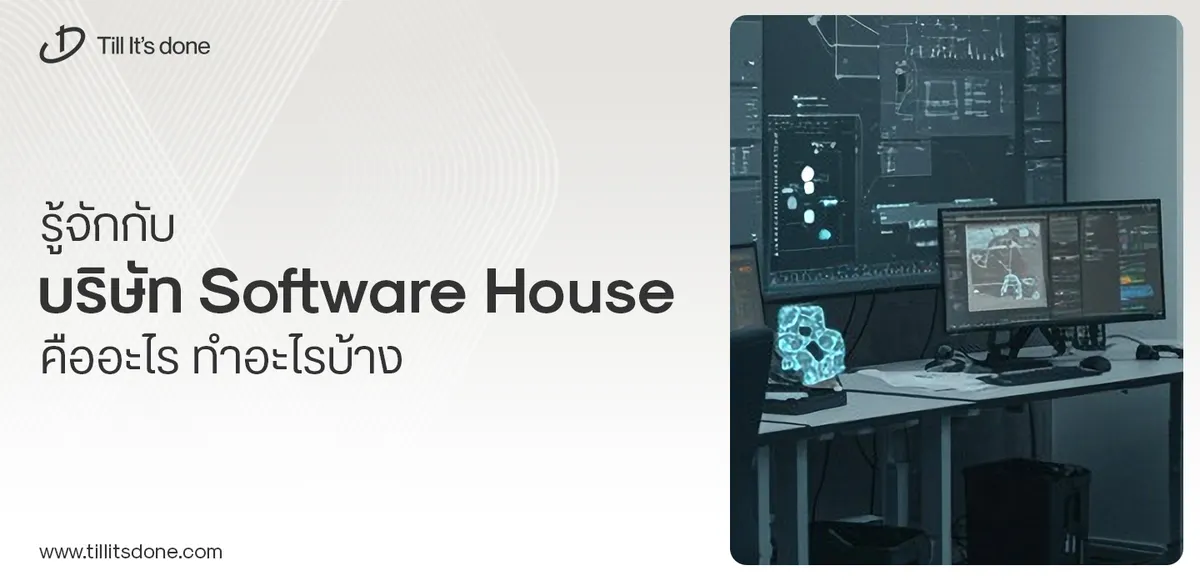
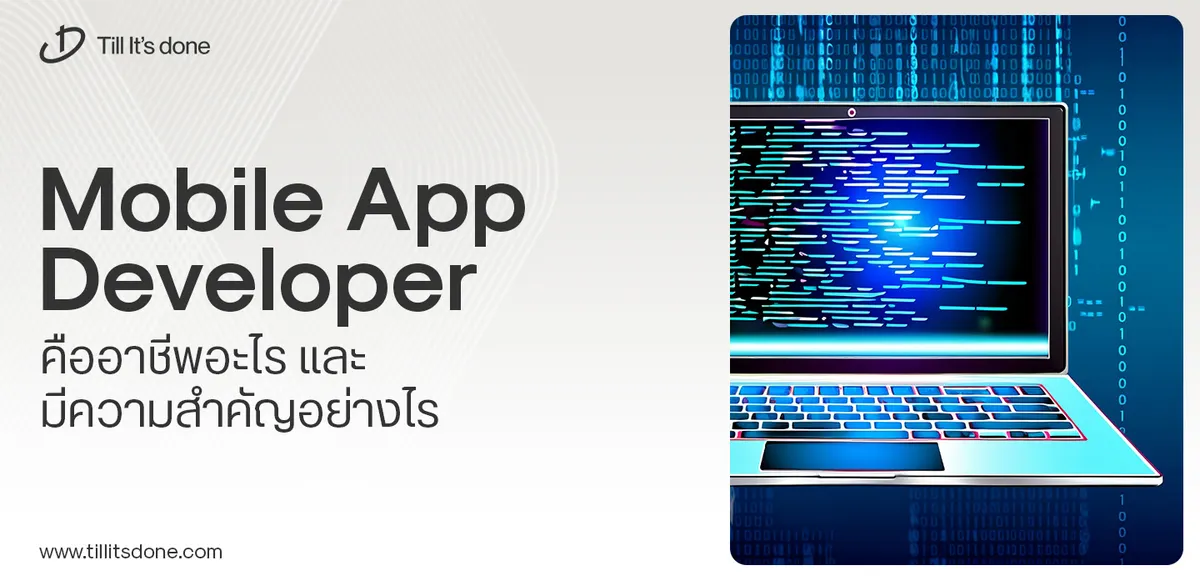
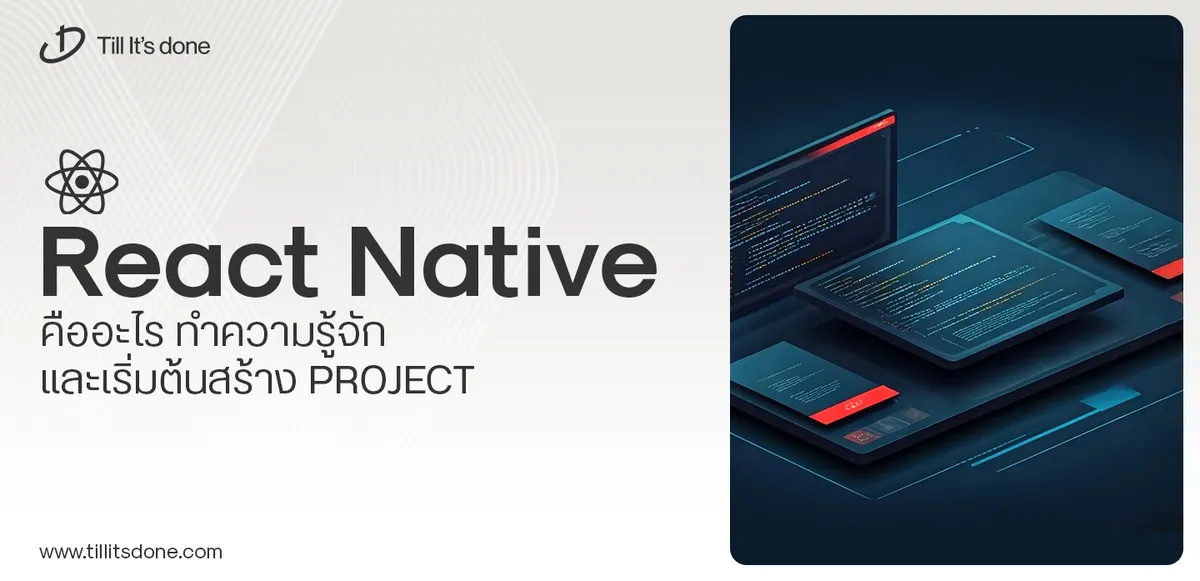
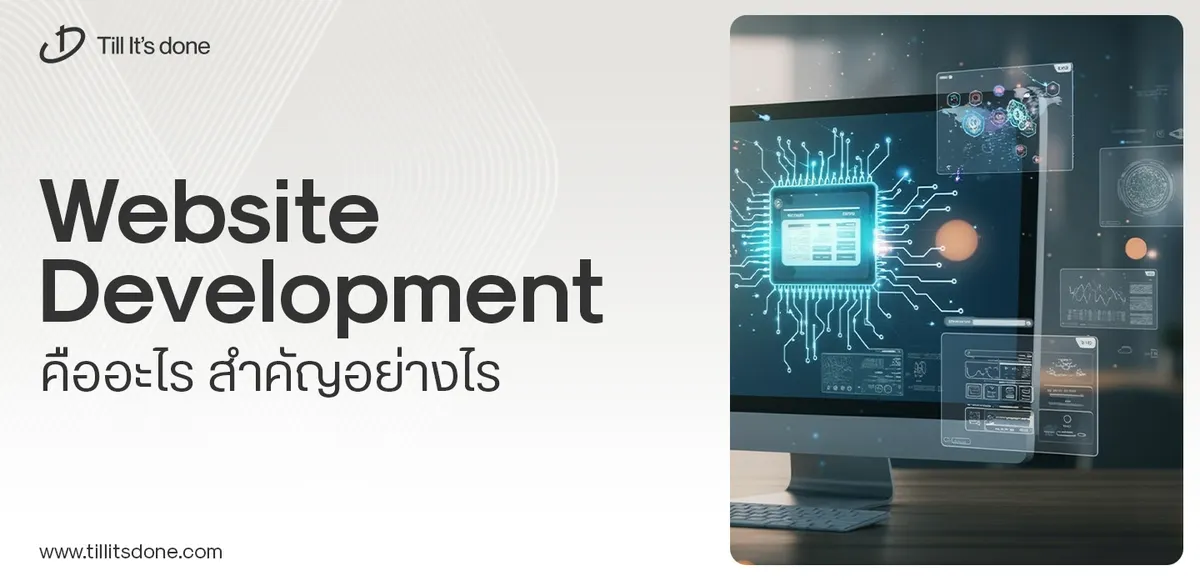
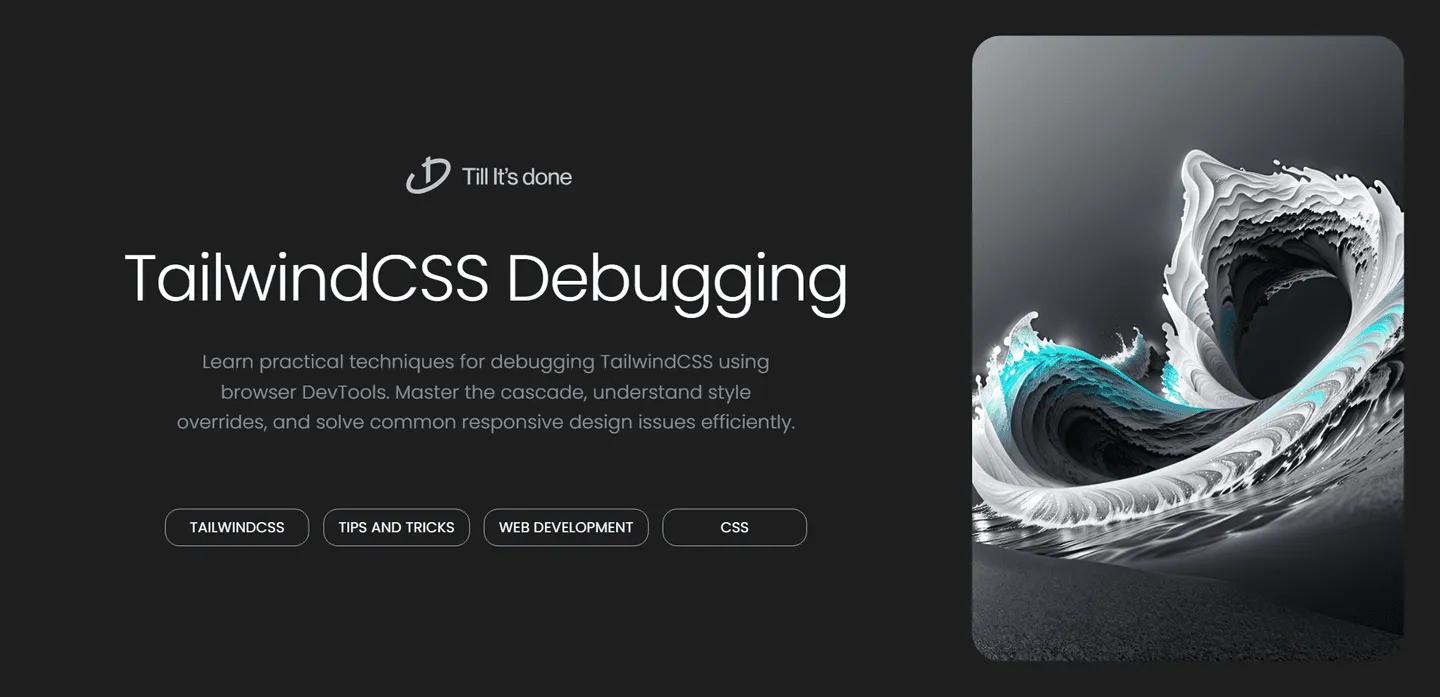
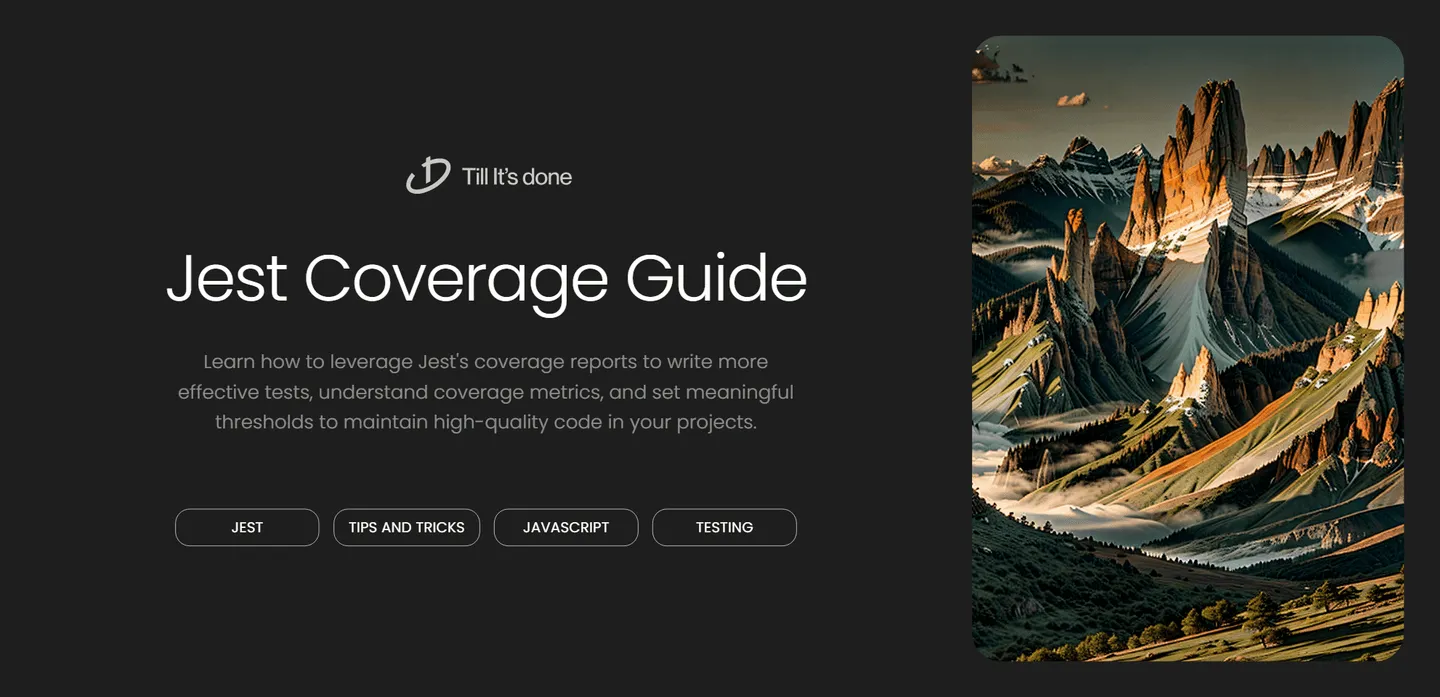
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.