- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing Async Code with React Testing Library
Master findBy queries, waitFor utility, and best practices for reliable testing.

How to Test Asynchronous Code with React Testing Library
Testing asynchronous operations in React applications can be tricky, but React Testing Library provides powerful tools to make this process straightforward and reliable. Let’s dive into how we can effectively test async operations while maintaining clean and maintainable test code.
Understanding Asynchronous Operations
In modern web applications, async operations are everywhere - from API calls to data fetching, user interactions that trigger animations, and more. When testing these operations, we need to ensure our tests wait for the right moments and assert the expected outcomes correctly.
Essential Async Testing Tools
React Testing Library provides several utilities for handling async operations:
findBy Queries
The findBy
family of queries are your first line of defense when testing async behavior. Unlike getBy
, these queries return a Promise and will keep trying to find the element for a few seconds before timing out.
test('should load user data', async () => { render(<UserProfile />);
// This will wait up to the default timeout const userName = await screen.findByText('John Doe'); expect(userName).toBeInTheDocument();});
waitFor
When you need more flexibility, waitFor
is your friend. It allows you to wait for any assertion or condition to be met:
test('should update counter after delay', async () => { render(<DelayedCounter />);
await waitFor(() => { expect(screen.getByText('Count: 5')).toBeInTheDocument(); });});
Best Practices for Async Testing
-
Avoid Arbitrary Timeouts Instead of using
setTimeout
in tests, preferwaitFor
orfindBy
queries. They’re more reliable and make your tests less flaky. -
Mock Time When Necessary For operations that involve specific timing, use Jest’s timer mocks:
test('should show notification after delay', async () => { jest.useFakeTimers(); render(<NotificationComponent />);
jest.advanceTimersByTime(2000);
await screen.findByText('Notification Message');
jest.useRealTimers();});
- Handle API Calls Properly When testing components that make API calls, mock the responses and ensure proper error handling:
test('should handle API error gracefully', async () => { server.use( rest.get('/api/user', (req, res, ctx) => { return res(ctx.status(500)); }) );
render(<UserProfile />);
const errorMessage = await screen.findByText('Failed to load user data'); expect(errorMessage).toBeInTheDocument();});
Common Pitfalls to Avoid
- Don’t mix async and sync queries unnecessarily
- Always clean up after your tests
- Be careful with race conditions in tests
- Avoid testing implementation details
Remember, the goal is to test your components the way users would interact with them. Focus on behavior, not implementation.
By following these patterns and best practices, you’ll be able to write reliable tests for even the most complex async operations in your React applications. Keep your tests focused on user behavior, use the right tools for the job, and remember that good tests should give you confidence in your code without being a burden to maintain.





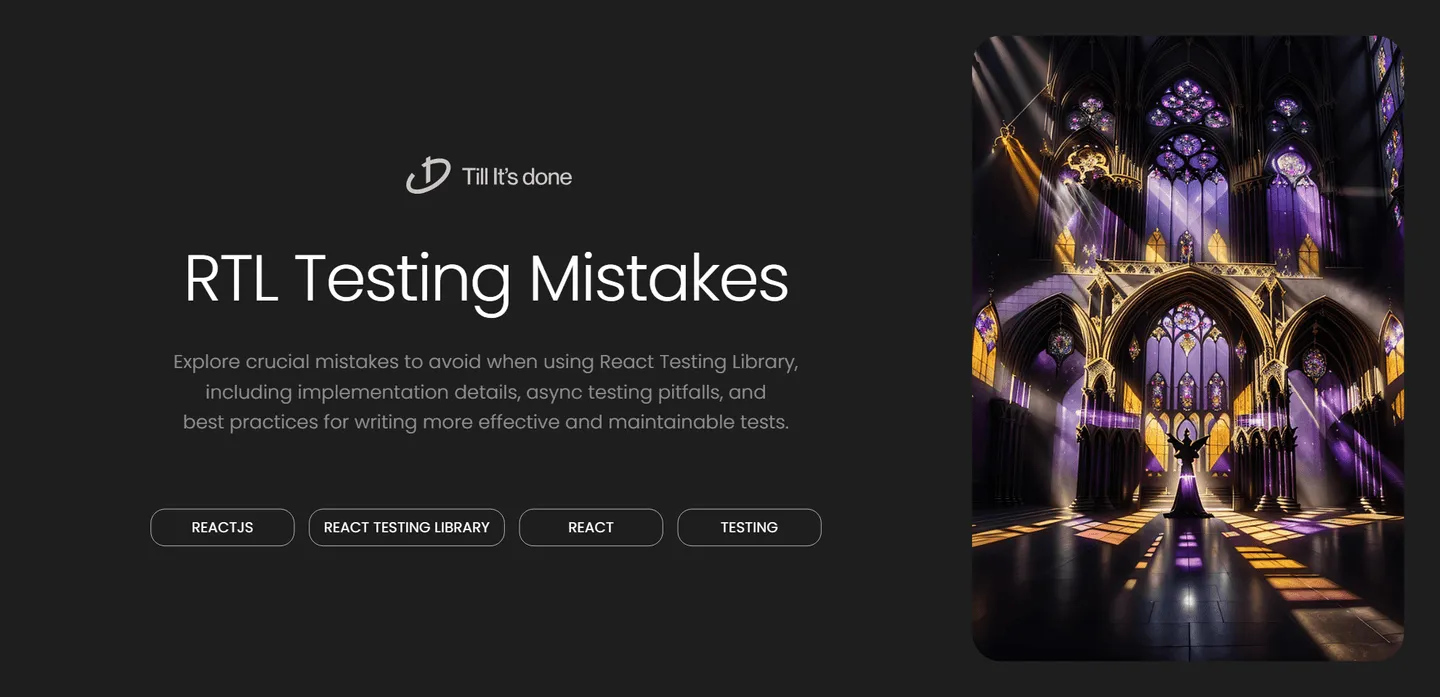
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.