- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for React Testing Library Tests

Best Practices for Writing Tests with React Testing Library
Testing is a crucial part of developing robust React applications. React Testing Library has emerged as the go-to solution for testing React components, emphasizing testing behavior over implementation. Let’s dive into the best practices that will help you write more effective and maintainable tests.
Query Priority: Following the Right Order
One of the fundamental principles of React Testing Library is querying elements in a way that reflects how users interact with your application. Here’s the recommended order of queries:
- getByRole
- getByLabelText
- getByPlaceholderText
- getByText
- getByDisplayValue
This order ensures your tests are more resilient to changes and better reflect user behavior. For example:
// ❌ Don'tconst submitButton = screen.getByTestId('submit-button')
// ✅ Doconst submitButton = screen.getByRole('button', { name: /submit/i })
Avoiding Implementation Details
The key philosophy behind React Testing Library is testing behavior rather than implementation. Your tests should focus on what the user sees and does, not on the internal workings of components.
// ❌ Don'texpect(component.state.isLoading).toBe(true)
// ✅ Doexpect(screen.getByText(/loading/i)).toBeInTheDocument()
Writing Clean and Maintainable Tests
Arrange-Act-Assert Pattern
Structure your tests using the Arrange-Act-Assert pattern to make them more readable and maintainable:
test('submitting the form displays success message', async () => { // Arrange render(<RegistrationForm />)
// Act await userEvent.type(screen.getByLabelText(/username/i), 'testuser') await userEvent.type(screen.getByLabelText(/password/i), 'password123') await userEvent.click(screen.getByRole('button', { name: /submit/i }))
// Assert expect(screen.getByText(/registration successful/i)).toBeInTheDocument()})
Use Custom Render Functions
For components that require specific context or setup, create custom render functions:
const customRender = (ui, { providerProps, ...renderOptions}) => { return render( <ThemeProvider {...providerProps}>{ui}</ThemeProvider>, renderOptions )}
Async Testing Best Practices
When testing asynchronous operations, use findBy
queries and waitFor
appropriately:
test('loads and displays user data', async () => { render(<UserProfile />)
expect(screen.getByText(/loading/i)).toBeInTheDocument()
const userData = await screen.findByText(/john doe/i) expect(userData).toBeInTheDocument()})
Common Pitfalls to Avoid
- Don’t use
container.querySelector
unless absolutely necessary - Avoid testing implementation details like state or props
- Don’t use
cleanup
manually (it’s automatic) - Don’t overuse
data-testid
attributes
Remember, the goal is to write tests that give you confidence in your application’s behavior while being maintainable and resistant to refactoring.





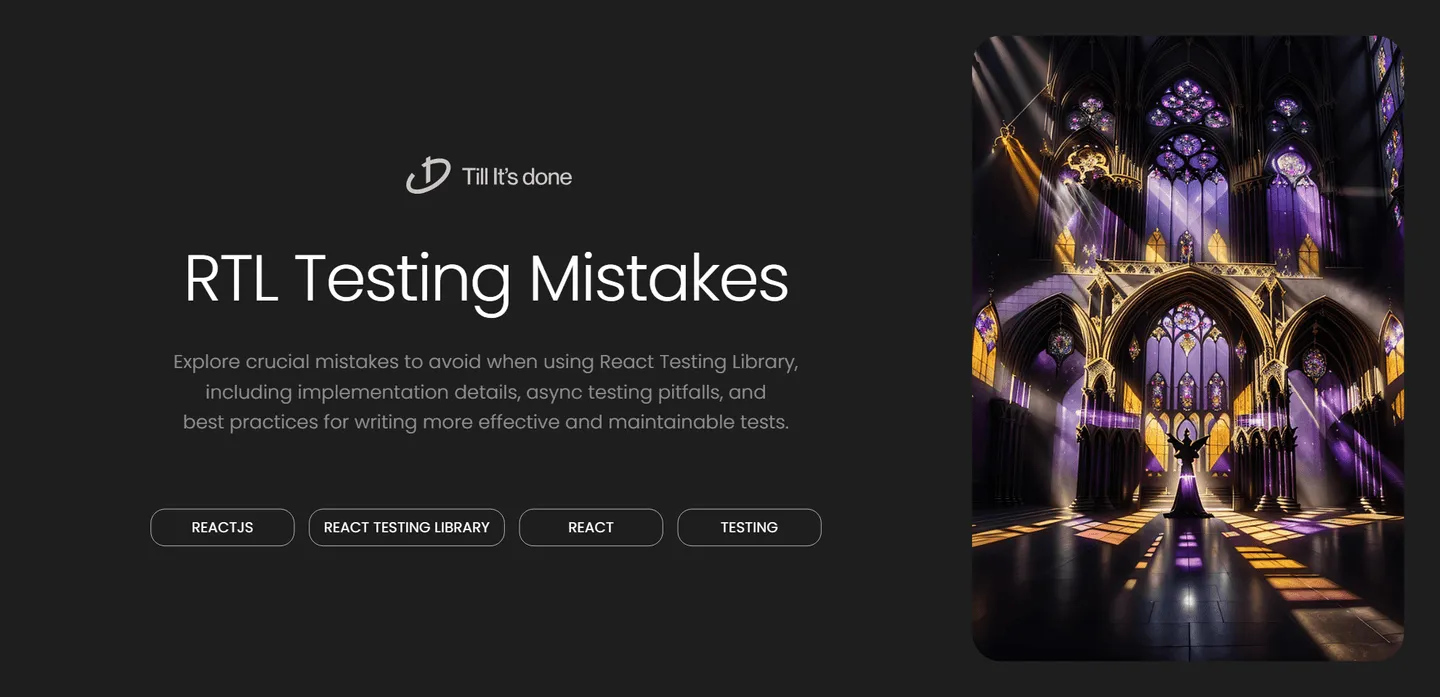
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.