- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Write Unit Tests with GoMock for Coverage
Master mocking techniques, improve code coverage, and create more reliable test suites for your Go applications.

How to Write Unit Tests with GoMock for Better Code Coverage
Testing is a crucial aspect of software development that helps ensure our code works as intended. When it comes to Go programming, GoMock is a powerful mocking framework that enables us to write comprehensive unit tests. In this guide, we’ll explore how to leverage GoMock to improve your code coverage and create more reliable tests.
Understanding GoMock
GoMock is a mocking framework that lets you replace real implementations with mock objects during testing. This is particularly useful when testing code that depends on external services, databases, or complex interfaces. By using mocks, we can isolate the code we’re testing and ensure our tests are fast, reliable, and deterministic.
Getting Started with GoMock
First, let’s set up GoMock in your project:
go install github.com/golang/mock/mockgen@latest
Creating Your First Mock
Let’s say we have a simple interface for a user service:
type UserService interface { GetUser(id string) (*User, error) CreateUser(user *User) error}
To generate a mock for this interface, use mockgen:
mockgen -source=user_service.go -destination=mocks/mock_user_service.go
Writing Tests with GoMock
Here’s a practical example of how to use GoMock in your tests:
func TestUserHandler_GetUser(t *testing.T) { ctrl := gomock.NewController(t) defer ctrl.Finish()
mockService := mocks.NewMockUserService(ctrl) handler := NewUserHandler(mockService)
// Set up expectations mockService.EXPECT(). GetUser("123"). Return(&User{ID: "123", Name: "John"}, nil)
user, err := handler.GetUser("123") assert.NoError(t, err) assert.Equal(t, "John", user.Name)}
Best Practices for Using GoMock
-
Mock at Interface Boundaries: Create interfaces at your application’s boundaries and mock those instead of concrete implementations.
-
Use Strict Mocking: Enable strict mocking to catch unexpected calls early:
ctrl := gomock.NewController(t)mockService := NewMockService(ctrl)
- Test Edge Cases: Use GoMock to simulate error conditions and edge cases:
mockService.EXPECT(). GetUser(gomock.Any()). Return(nil, errors.New("database error"))
- Match Arguments Flexibly: Use GoMock matchers for more flexible argument matching:
mockService.EXPECT(). CreateUser(gomock.Any()). DoAndReturn(func(user *User) error { // Custom validation logic return nil })
Improving Code Coverage
To maximize your test coverage with GoMock:
- Test both success and failure paths
- Verify mock expectations
- Use gomock.InOrder when sequence matters
- Test timeout scenarios using gomock.After
Remember that high code coverage doesn’t automatically mean good tests. Focus on testing business-critical paths and edge cases that could cause issues in production.





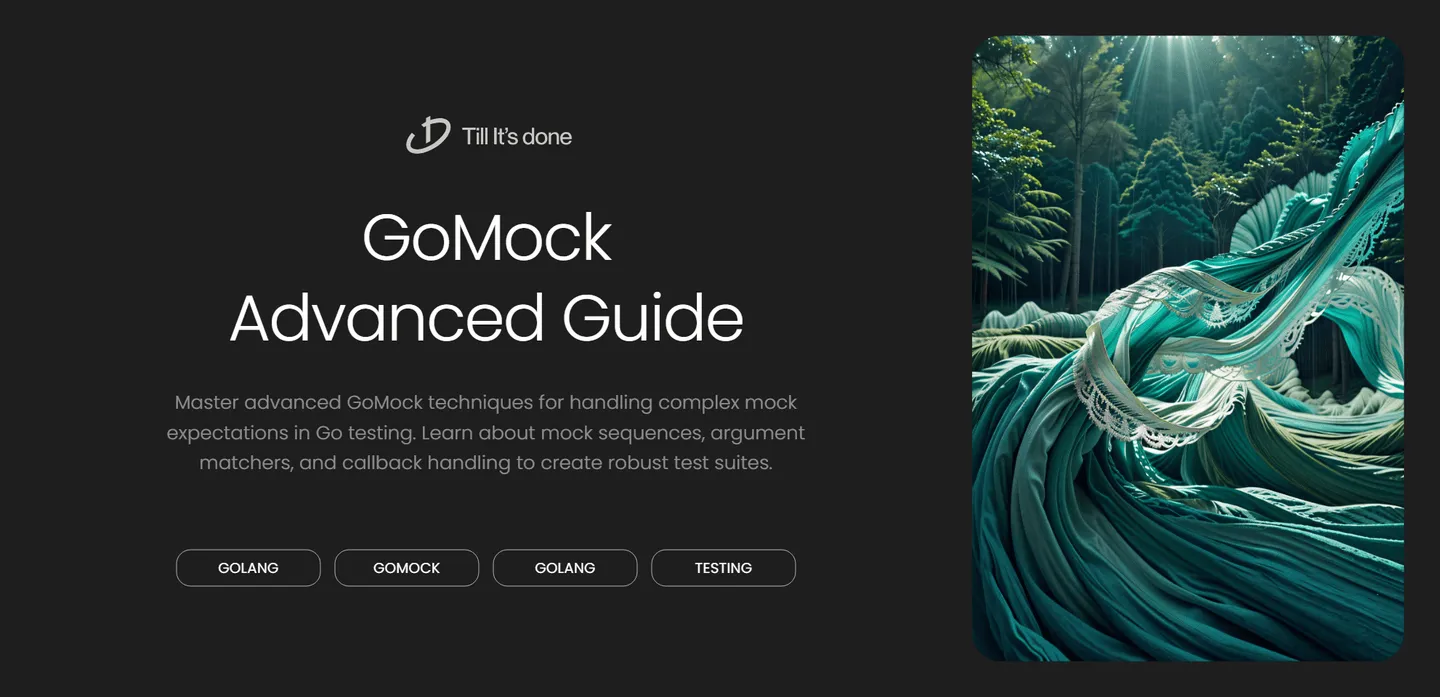
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.