- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Generics in TypeScript: Best Practices
Master type-safe programming with reusable code patterns and real-world applications.
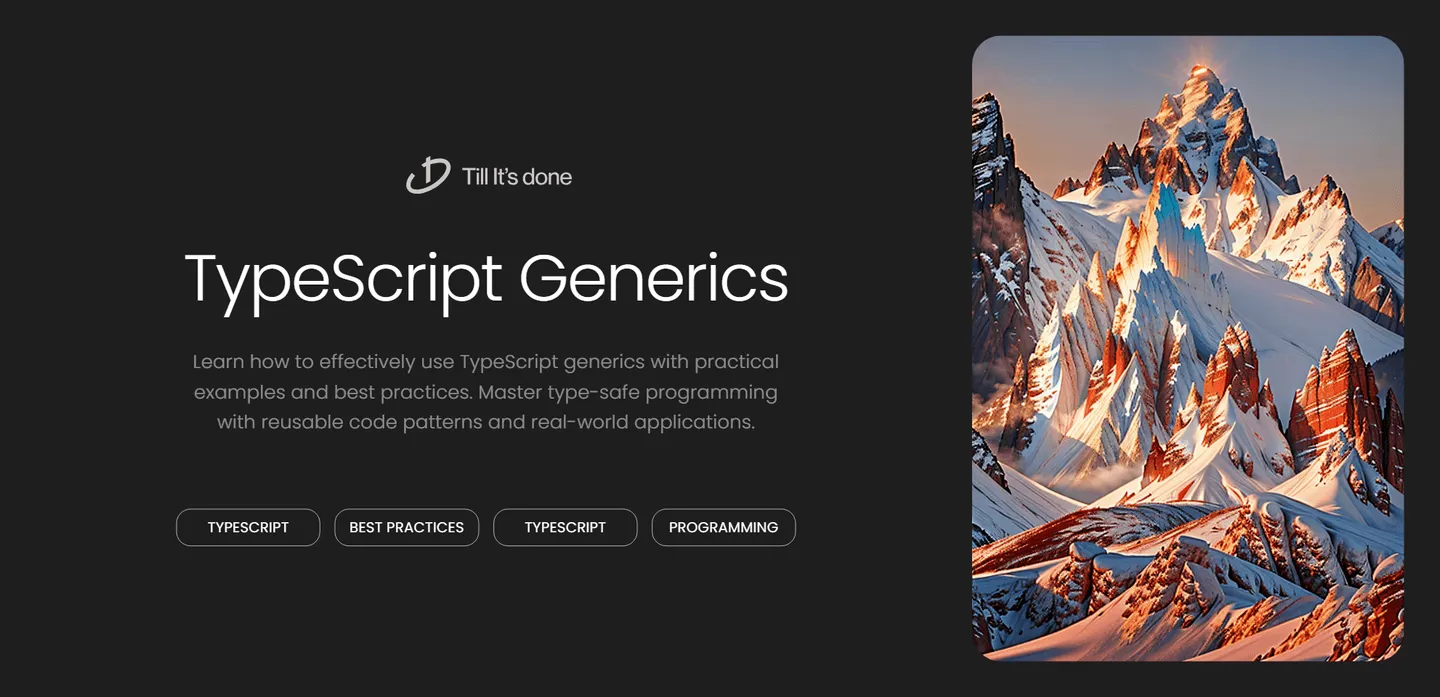
Using Generics in TypeScript: Best Practices and Examples
Diving into TypeScript’s generics might seem daunting at first, but they’re like having a Swiss Army knife in your coding toolkit. Let’s break down this powerful feature and explore how to use it effectively in your projects.
Understanding the Basics
Think of generics as placeholders for types that you’ll specify later. It’s like telling TypeScript, “Hey, I’ll let you know what type I want to use when I actually use this code.” This flexibility makes your code more reusable and type-safe.
function identity\<T\>(arg: T): T { return arg;}
// Using itconst numberResult = identity(42); // Type is numberconst stringResult = identity("Hello"); // Type is string
Real-World Use Cases
Creating Type-Safe Collections
One of the most common uses for generics is building type-safe collections. Here’s how you might create a simple queue:
class Queue\<T\> { private data: T[] = [];
push(item: T) { this.data.push(item); }
pop(): T | undefined { return this.data.shift(); }}
const numberQueue = new Queue<number>();numberQueue.push(123); // ✅ WorksnumberQueue.push("123"); // ❌ Type error
Generic Constraints
Sometimes you need to restrict what types can be used with your generic code. That’s where constraints come in:
interface HasLength { length: number;}
function logLength<T extends HasLength>(arg: T): void { console.log(arg.length);}
logLength("Hello"); // ✅ WorkslogLength([1, 2, 3]); // ✅ WorkslogLength(123); // ❌ Error: number doesn't have length
Best Practices
1. Use Descriptive Type Parameters
Instead of single letters, use descriptive names when it makes the code clearer:
// Less clearfunction process<T, U>(input: T, options: U): T { // ...}
// More clearfunction process<InputType, OptionsType>(input: InputType, options: OptionsType): InputType { // ...}
2. Avoid Over-Genericizing
Don’t make everything generic just because you can. Use generics only when you need to preserve type information or create truly reusable components.
3. Combine with Union Types
Generics work great with union types for more flexible, yet type-safe code:
function firstElement\<T\>(arr: T[]): T | undefined { return arr[0];}
const numbers = firstElement([1, 2, 3]); // Type: numberconst strings = firstElement(["a", "b", "c"]); // Type: stringconst empty = firstElement([]); // Type: undefined
Conclusion
Generics in TypeScript are a powerful tool that can make your code more flexible and maintainable. By following these best practices and understanding the core concepts, you’ll be well-equipped to write better, type-safe TypeScript code.
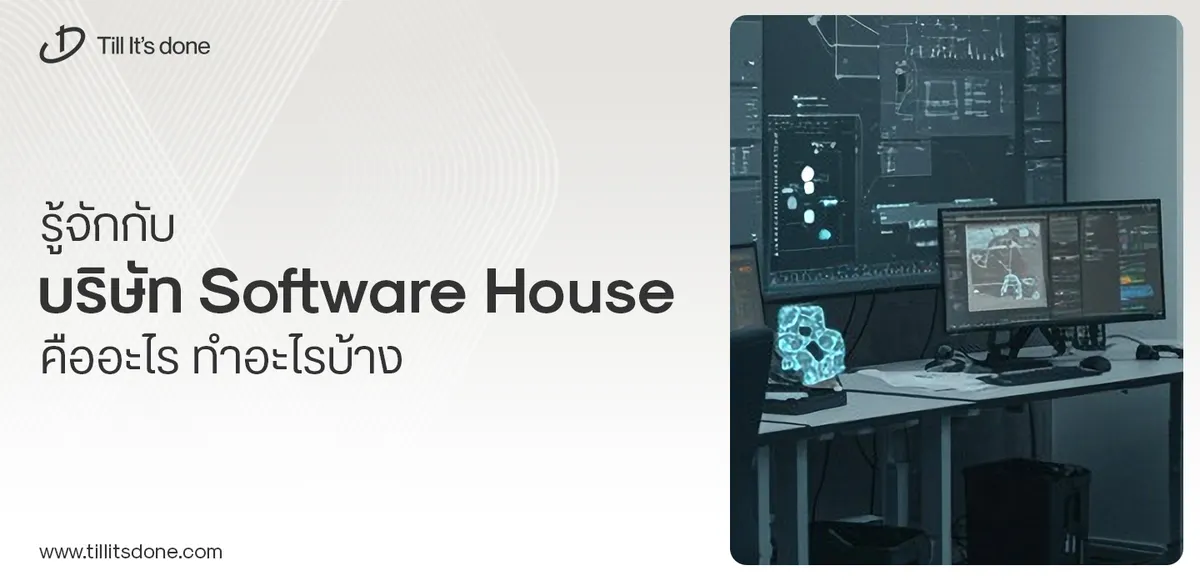
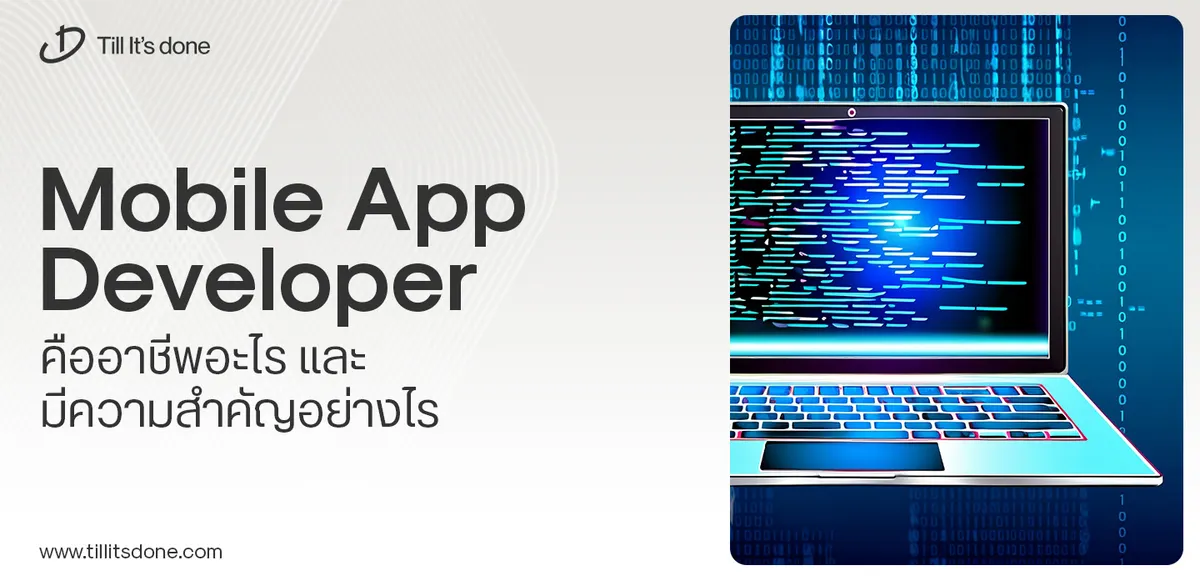
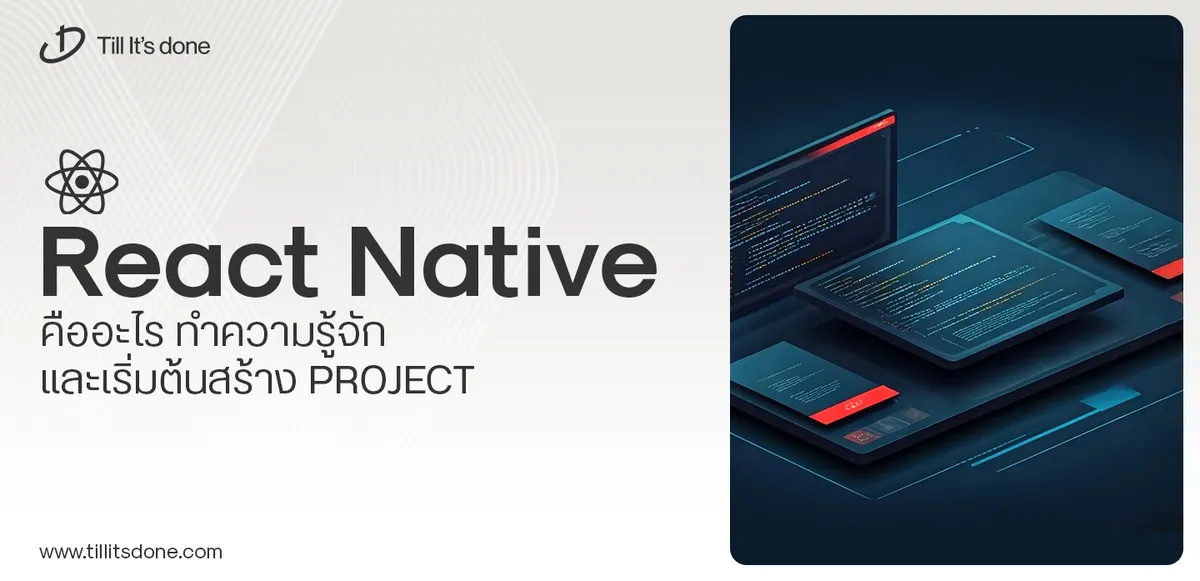
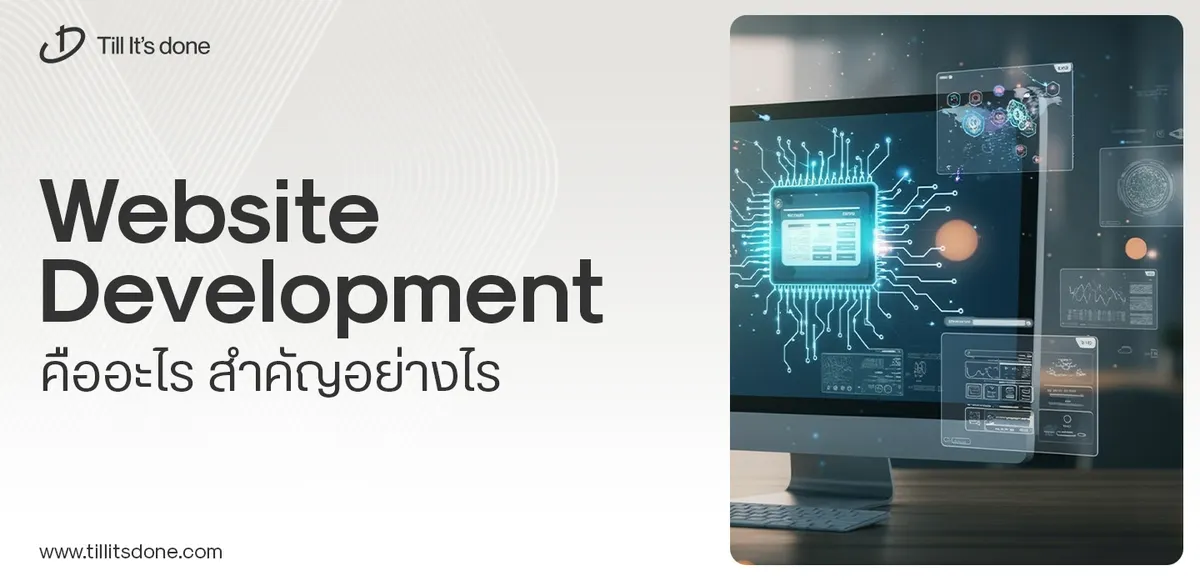
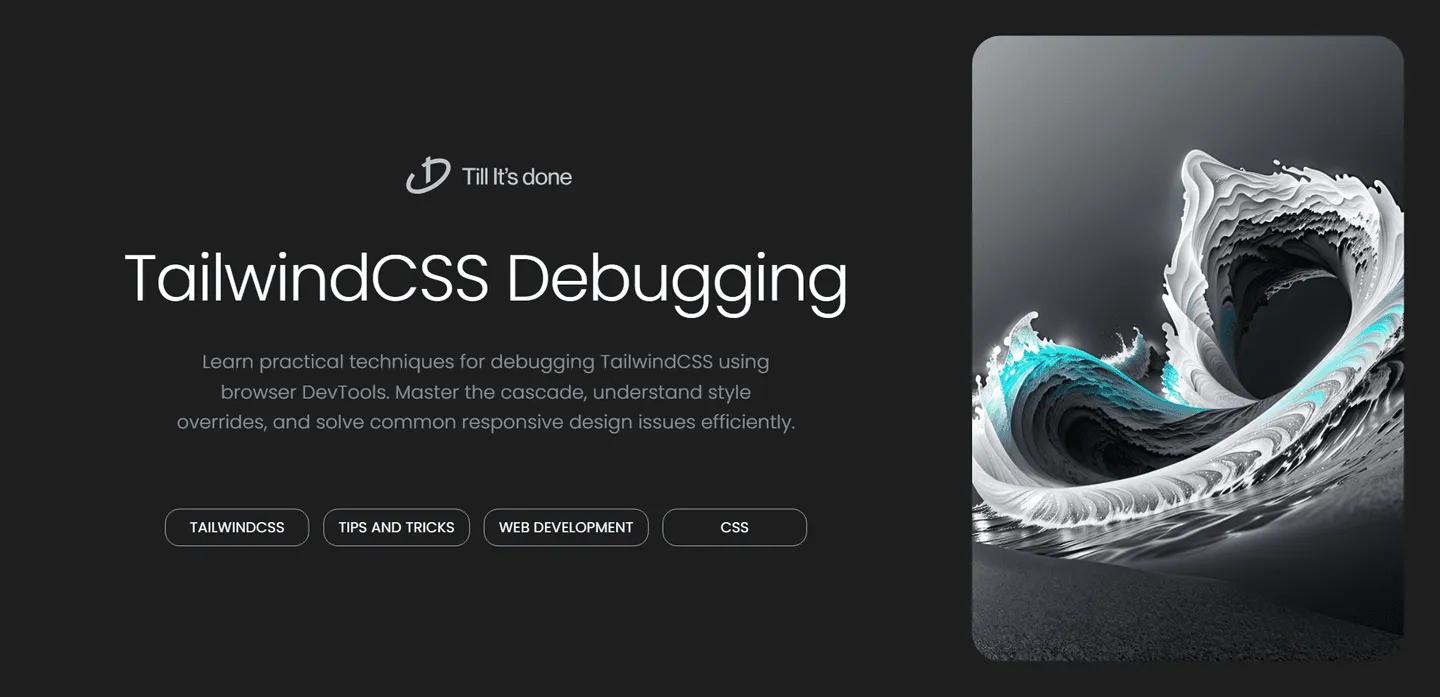
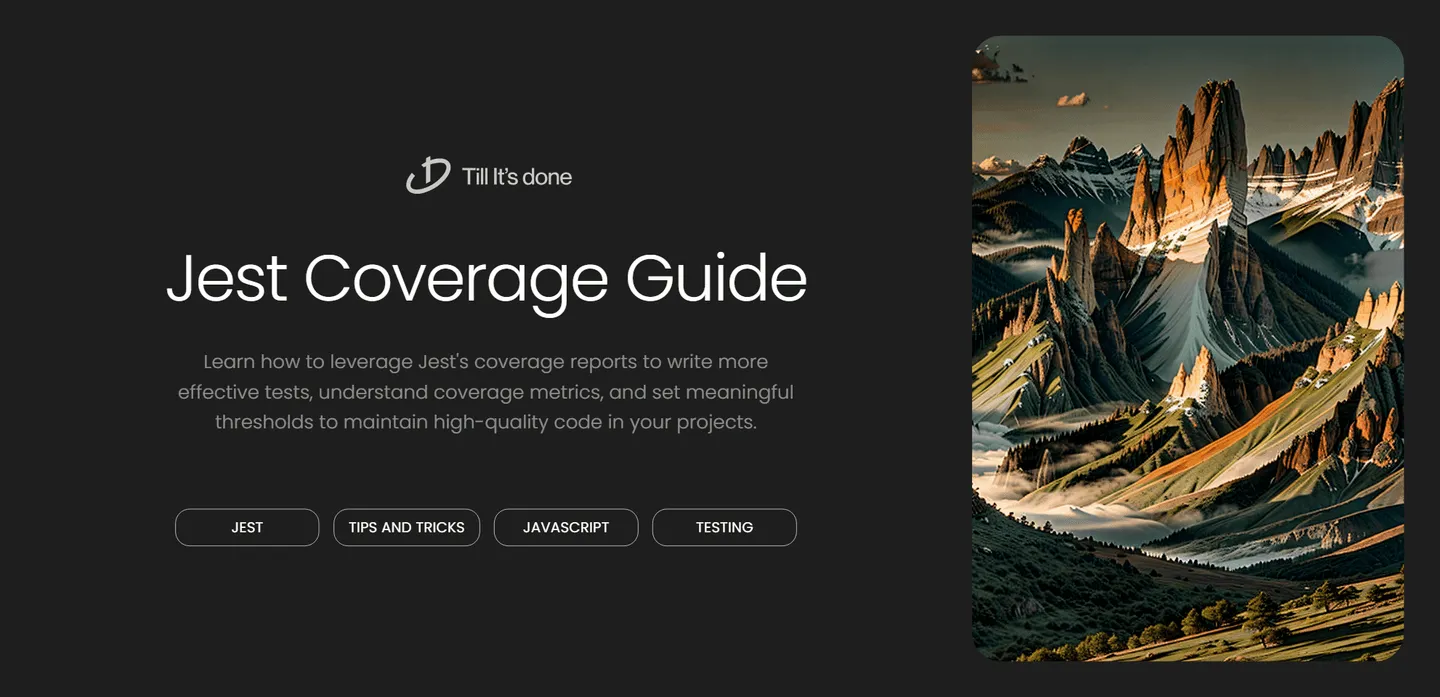
พูดคุยกับซีอีโอ
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.