- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Optimizing React App Performance with Memoization
Discover practical examples of React.memo, useMemo, and useCallback with best practices and implementation tips.
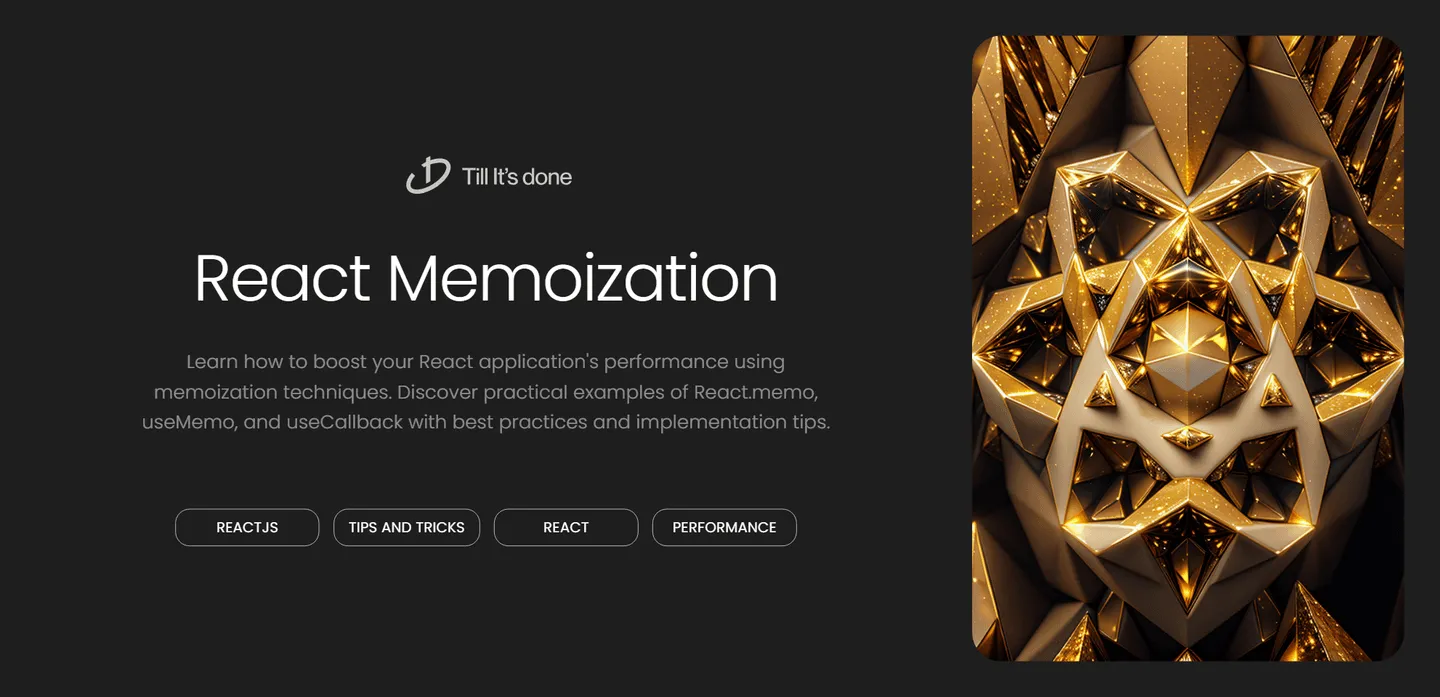
Optimizing React App Performance with Memoization
Performance optimization is crucial for creating smooth, responsive React applications. Let’s dive into how memoization can help us achieve this, with practical examples and best practices.
Understanding Memoization in React
Think of memoization as your app’s memory notebook. Instead of recalculating the same things repeatedly, it remembers the results from previous calculations. This can significantly boost your app’s performance, especially when dealing with complex computations.
React.memo: Your First Line of Defense
const ExpensiveComponent = React.memo(({ data }) => { // Component logic here return <div>{/* Rendered content */}</div>;});
React.memo is like a smart security guard for your components. It prevents unnecessary re-renders by comparing the old and new props. If nothing’s changed, why rebuild the whole component?
useMemo: For Complex Calculations
const memoizedValue = useMemo(() => { return expensiveOperation(dependencies);}, [dependencies]);
When you have heavy calculations in your component, useMemo is your best friend. It’s like having a calculator that remembers its previous answers - why solve the same math problem twice?
useCallback: Function Memoization
const memoizedCallback = useCallback(() => { doSomething(dependencies);}, [dependencies]);
Function memoization is particularly useful when passing callbacks to optimized child components. Think of it as writing a note once and making photocopies instead of writing it again and again.
Best Practices and Common Pitfalls
- Don’t overuse memoization - it comes with its own overhead
- Always measure performance before and after optimization
- Watch out for dependency arrays - missing dependencies can cause bugs
- Consider using the React DevTools Profiler to identify unnecessary re-renders
When to Use Each Type of Memoization
- Use React.memo for preventing re-renders of expensive components
- Use useMemo for expensive calculations
- Use useCallback when passing callbacks to optimized child components
Remember, premature optimization is the root of all evil. Always profile first, then optimize where needed.
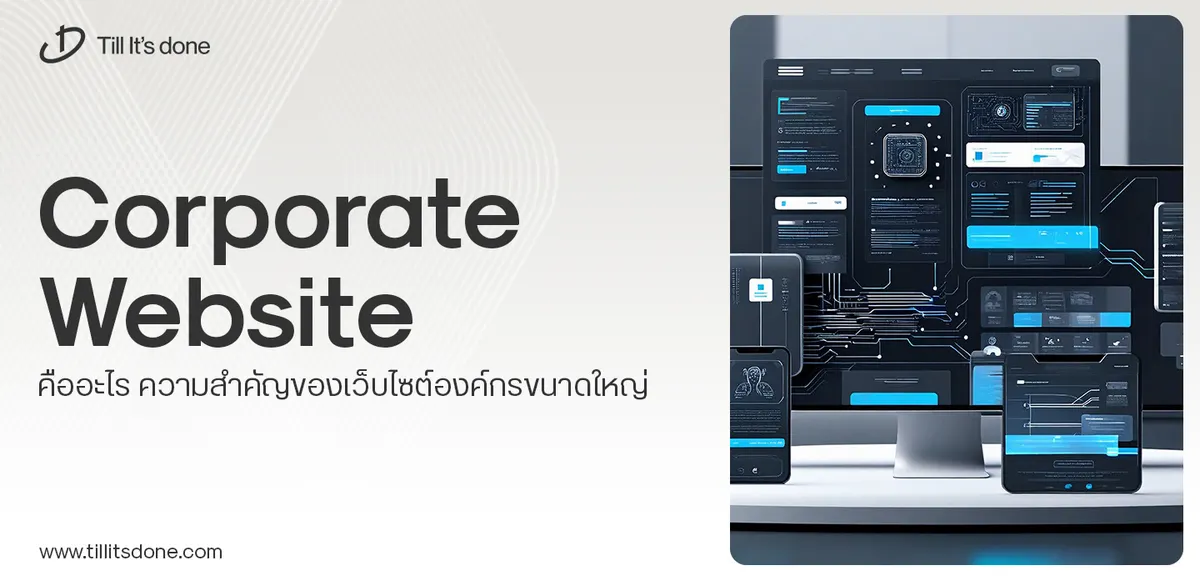
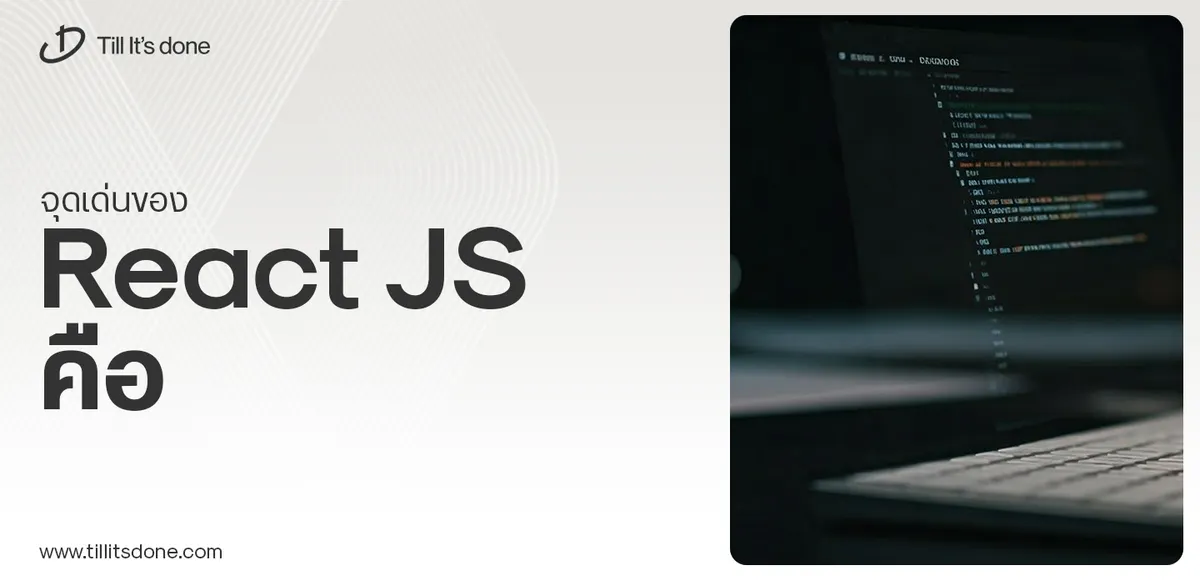
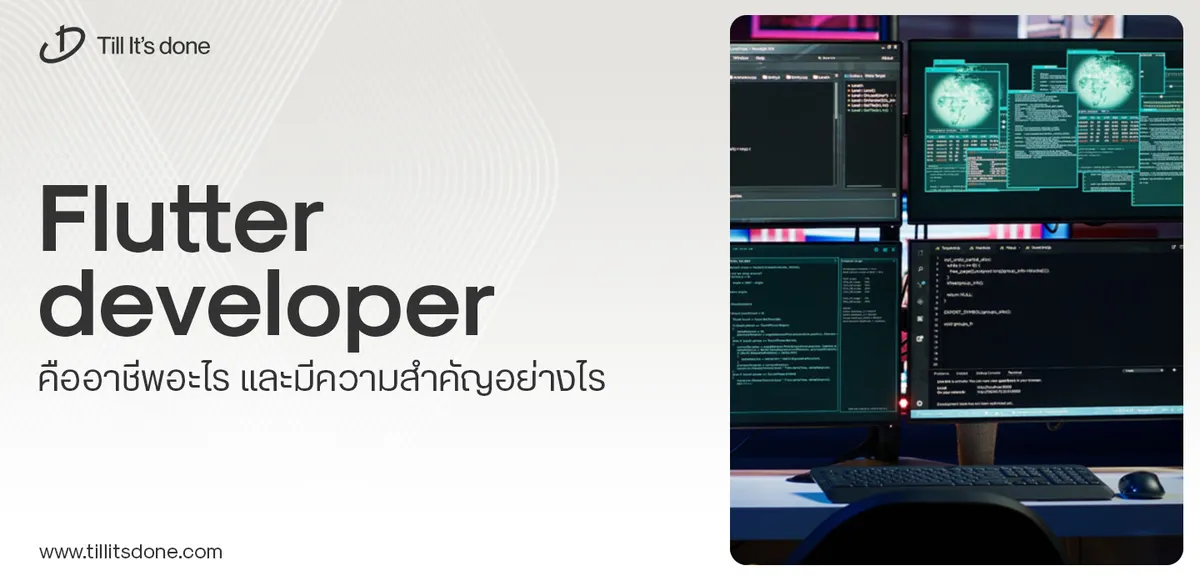
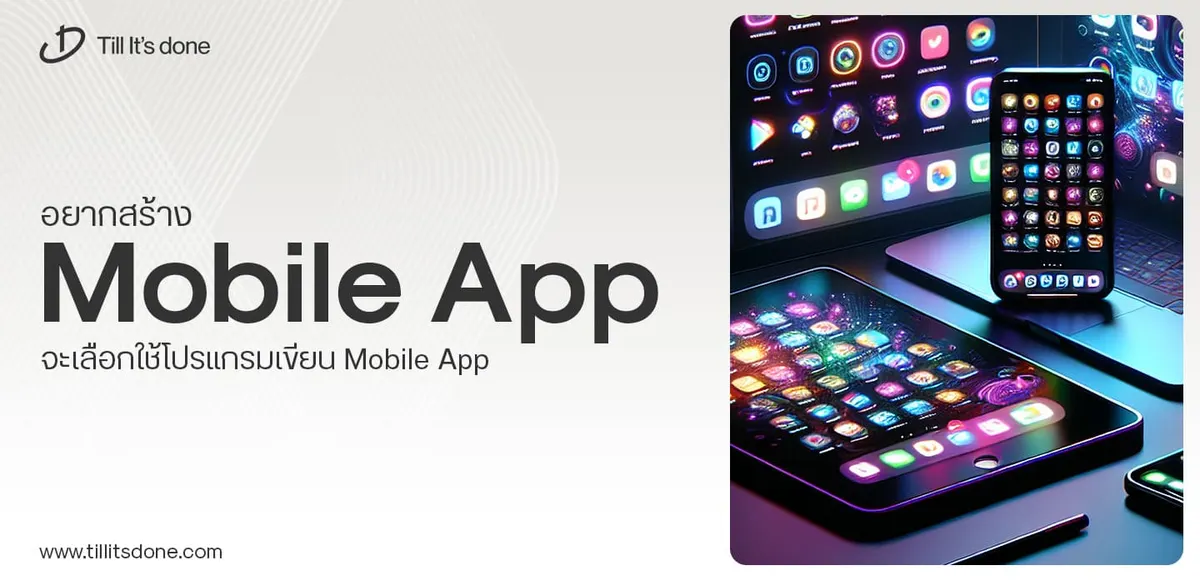
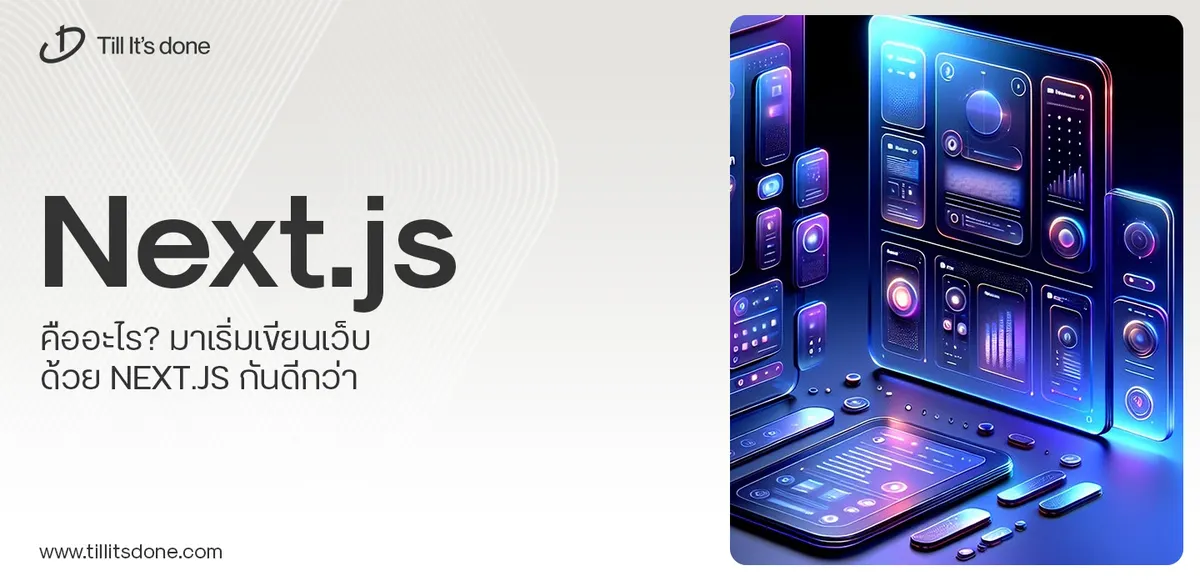
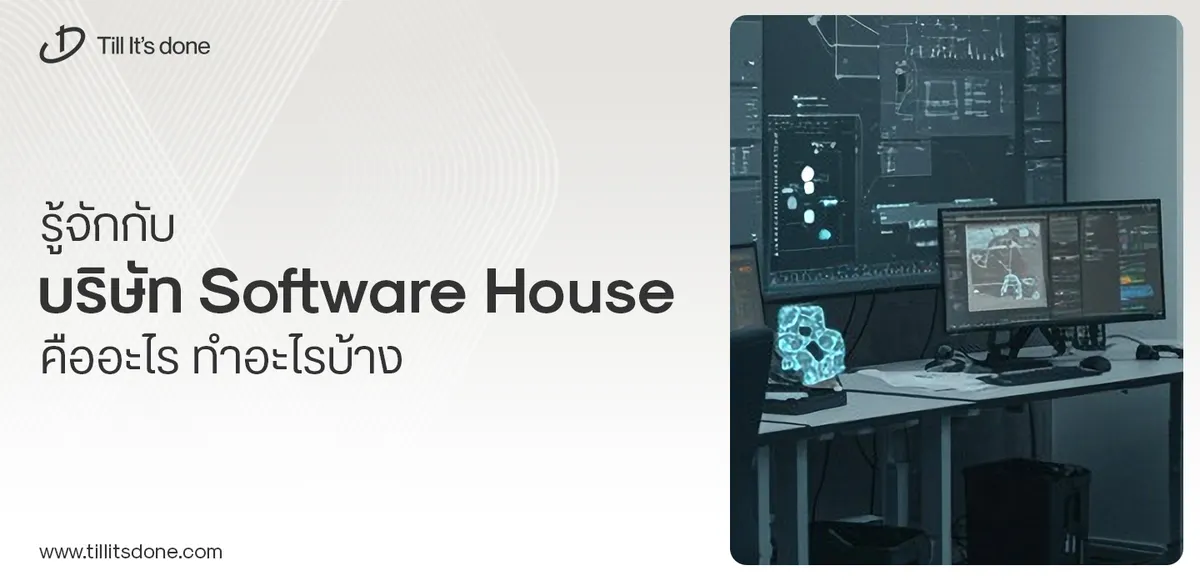
พูดคุยกับซีอีโอ
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.