- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Code Splitting in React for Performance
Discover best practices, implementation strategies, and performance monitoring tips.

Code Splitting in React for Scalable Applications
As React applications grow larger and more complex, optimizing performance becomes crucial for maintaining a smooth user experience. One powerful technique that can significantly improve your app’s loading time is code splitting. Let’s dive deep into this essential React optimization strategy.
Understanding Code Splitting
When you build a React application without code splitting, all your code gets bundled into a single file. While this might work fine for smaller applications, it can lead to unnecessarily long loading times for larger ones. Users end up downloading code they might never need, just to access the initial page.
Think of it like ordering your entire wardrobe when you only need a single outfit – it’s inefficient and wastes resources.
Implementing Code Splitting
1. Route-Based Splitting
The most common approach is splitting code based on routes. Instead of loading everything at once, load components only when the user navigates to specific routes:
import { lazy, Suspense } from 'react';
const Dashboard = lazy(() => import('./pages/Dashboard'));const Profile = lazy(() => import('./pages/Profile'));
function App() { return ( <Suspense fallback={<LoadingSpinner />}> <Routes> <Route path="/dashboard" element={<Dashboard />} /> <Route path="/profile" element={<Profile />} /> </Routes> </Suspense> );}
2. Component-Based Splitting
For complex components that aren’t immediately visible (like modals or tabs), consider splitting at the component level:
const HeavyChart = lazy(() => import('./components/HeavyChart'));
function Dashboard() { return ( <div> <Suspense fallback={<ChartPlaceholder />}> {showChart && <HeavyChart data={chartData} />} </Suspense> </div> );}
Best Practices
-
Strategic Splitting: Don’t split everything. Focus on larger components and routes that aren’t needed immediately.
-
Preloading: Consider preloading important routes when the user hovers over a link:
const ProductPage = lazy(() => import('./pages/Product'));
function handleMouseEnter() { const component = import('./pages/Product');}
return <button onMouseEnter={handleMouseEnter}>View Product</button>;
-
Error Boundaries: Always implement error boundaries around split components to handle loading failures gracefully.
-
Loading States: Design meaningful loading states that maintain your app’s visual consistency.
Measuring Impact
Monitor your application’s performance before and after implementing code splitting. Key metrics to track include:
- Initial bundle size
- Time to Interactive (TTI)
- First Contentful Paint (FCP)
- Route transition times
Remember, code splitting is not a silver bullet. It’s most effective when combined with other optimization techniques like proper caching, image optimization, and efficient state management.
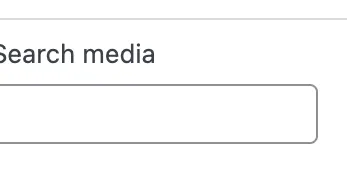





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.