- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
State Management Simplified: Flutter Tips
Learn best practices, avoid common pitfalls, and build more efficient Flutter applications.
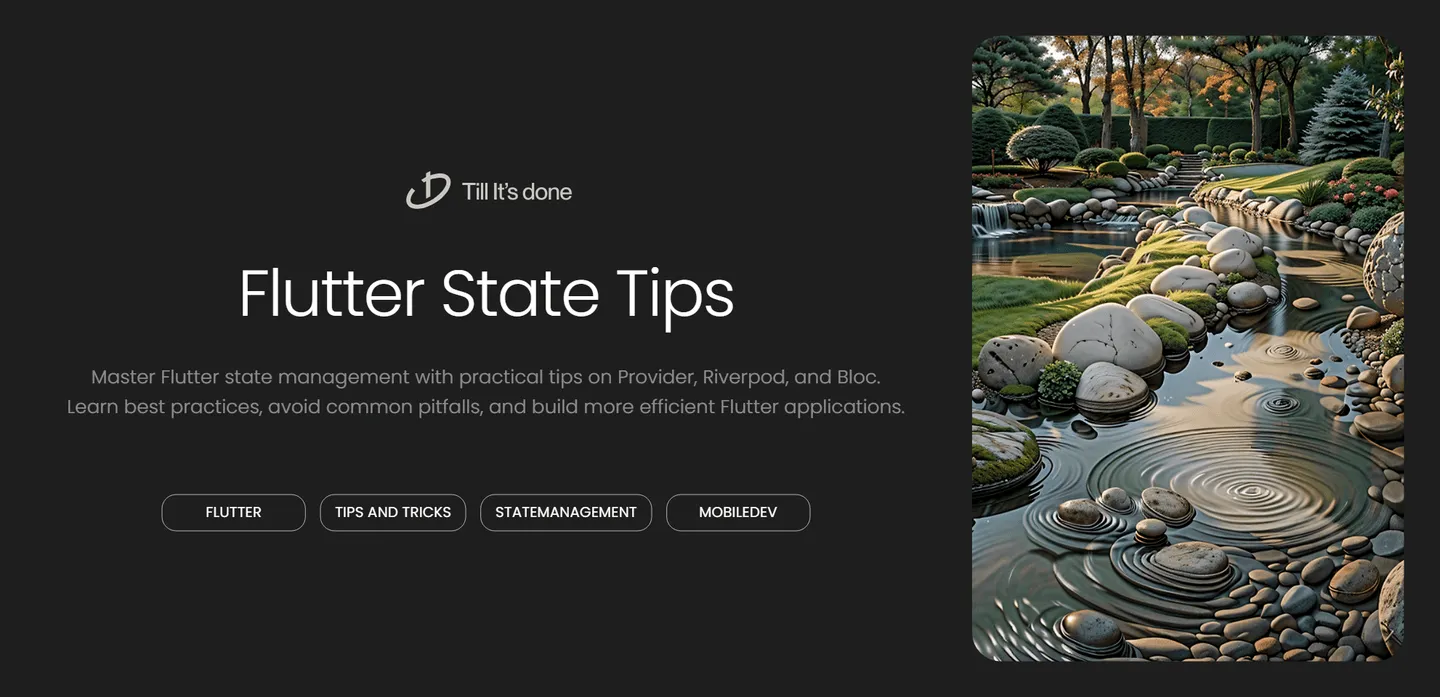
State Management Simplified: Tips for Efficient Flutter Apps
Managing state effectively in Flutter applications can be the difference between a smooth, performant app and a sluggish, bug-prone one. Let’s dive into some practical tips and strategies that will help you master state management in your Flutter projects.
Understanding State Management Basics
Think of state management like organizing your workspace. Just as you wouldn’t want tools scattered everywhere when working on a project, you don’t want your app’s data scattered across different widgets. Good state management keeps your data organized and accessible exactly where it needs to be.
Choosing the Right Solution
There’s no one-size-fits-all approach to state management in Flutter. Here are some popular solutions and when to use them:
Provider: Your First Step
Provider is like training wheels for state management. It’s simple enough for beginners but powerful enough for real applications. Use Provider when:
- You’re building a small to medium-sized app
- You want a gentle learning curve
- You need a solution that’s officially recommended by the Flutter team
Riverpod: The Evolution
Riverpod takes everything good about Provider and makes it better. Consider Riverpod when:
- You want compile-time safety
- You need better dependency management
- You’re building a larger application that requires scalability
Bloc: For Complex Applications
Think of Bloc as the power tool of state management. It’s perfect when:
- Your app has complex business logic
- You need a clear separation of concerns
- You’re working with a larger team
Best Practices for State Management
-
Keep it Simple Start with the simplest solution that meets your needs. You can always refactor later as your app grows.
-
Single Source of Truth Maintain one source of truth for your data. This prevents inconsistencies and makes debugging easier.
-
Separate Business Logic Keep your business logic separate from your UI code. This makes your code more maintainable and testable.
-
Smart State Updates Only update state when necessary. Unnecessary rebuilds can impact performance.
Common Pitfalls to Avoid
- Don’t mix different state management solutions without good reason
- Avoid storing duplicate state in different places
- Don’t put all your state in one giant provider/bloc
- Remember to dispose of controllers and streams properly
Testing Your State Management
Writing tests for your state management logic is crucial. Focus on:
- Unit tests for your business logic
- Widget tests for state-dependent UI
- Integration tests for complex state interactions
Remember, the goal of state management isn’t to use the most sophisticated solution, but to make your app maintainable and performant.
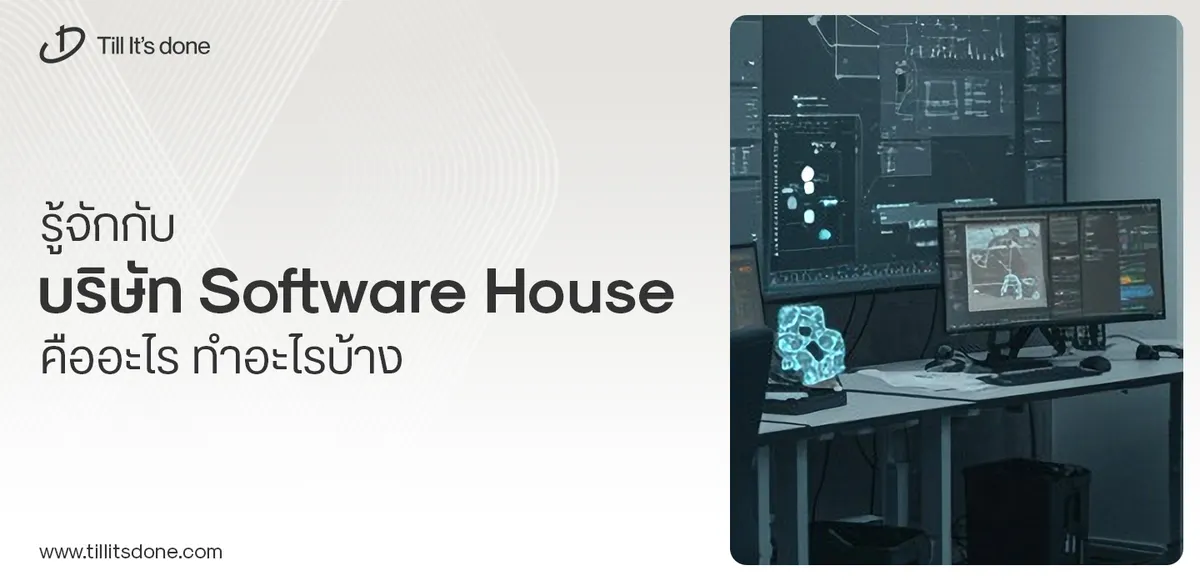
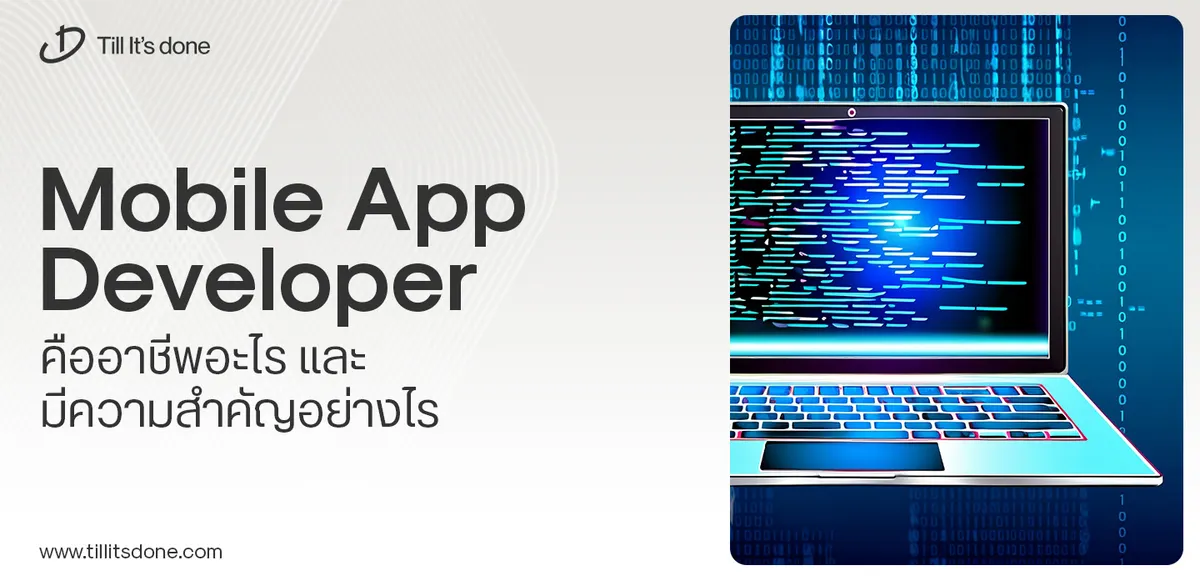
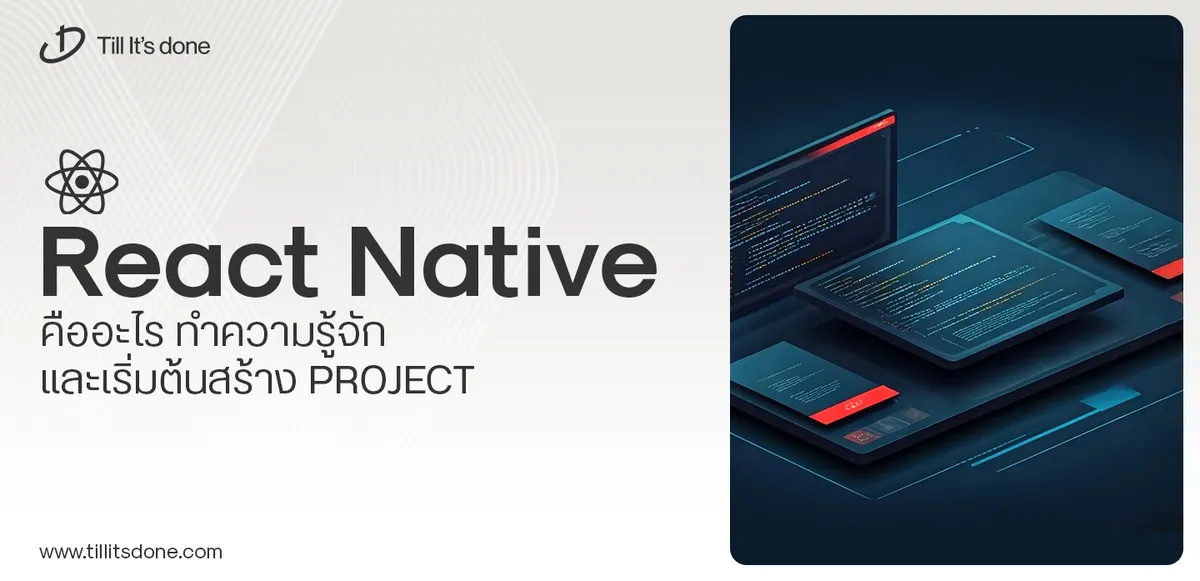
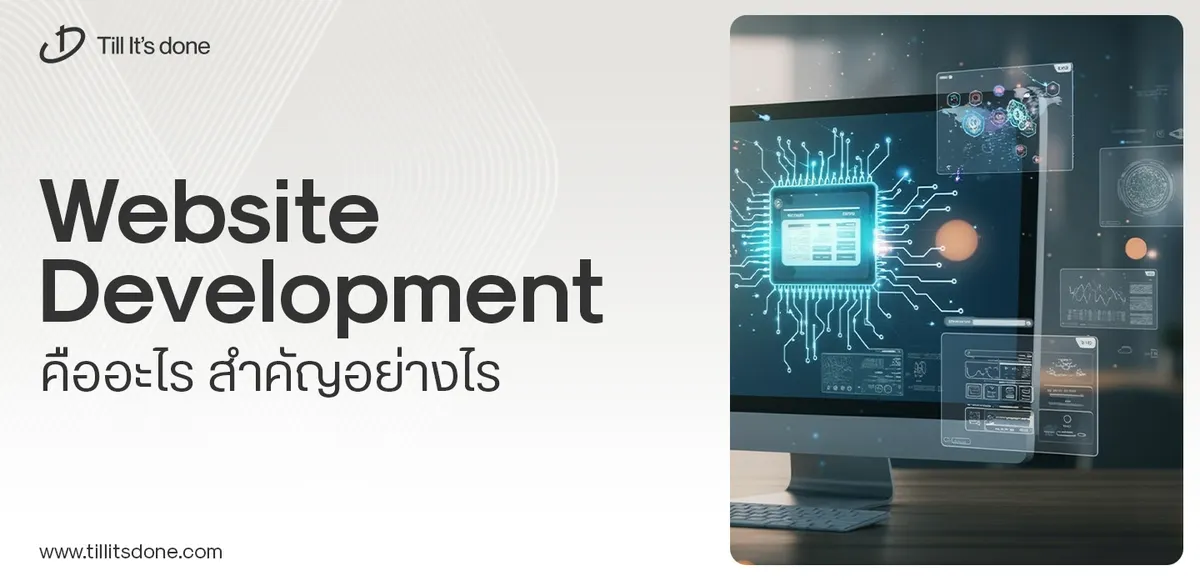
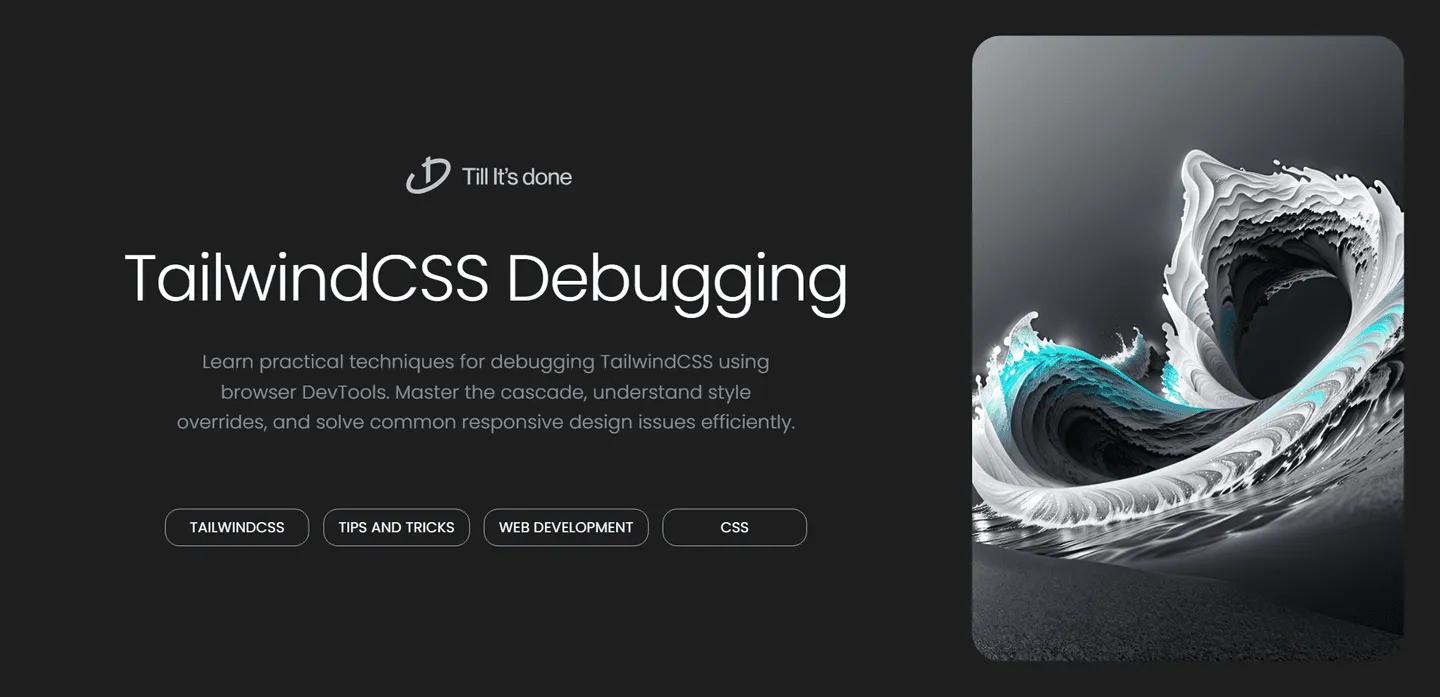
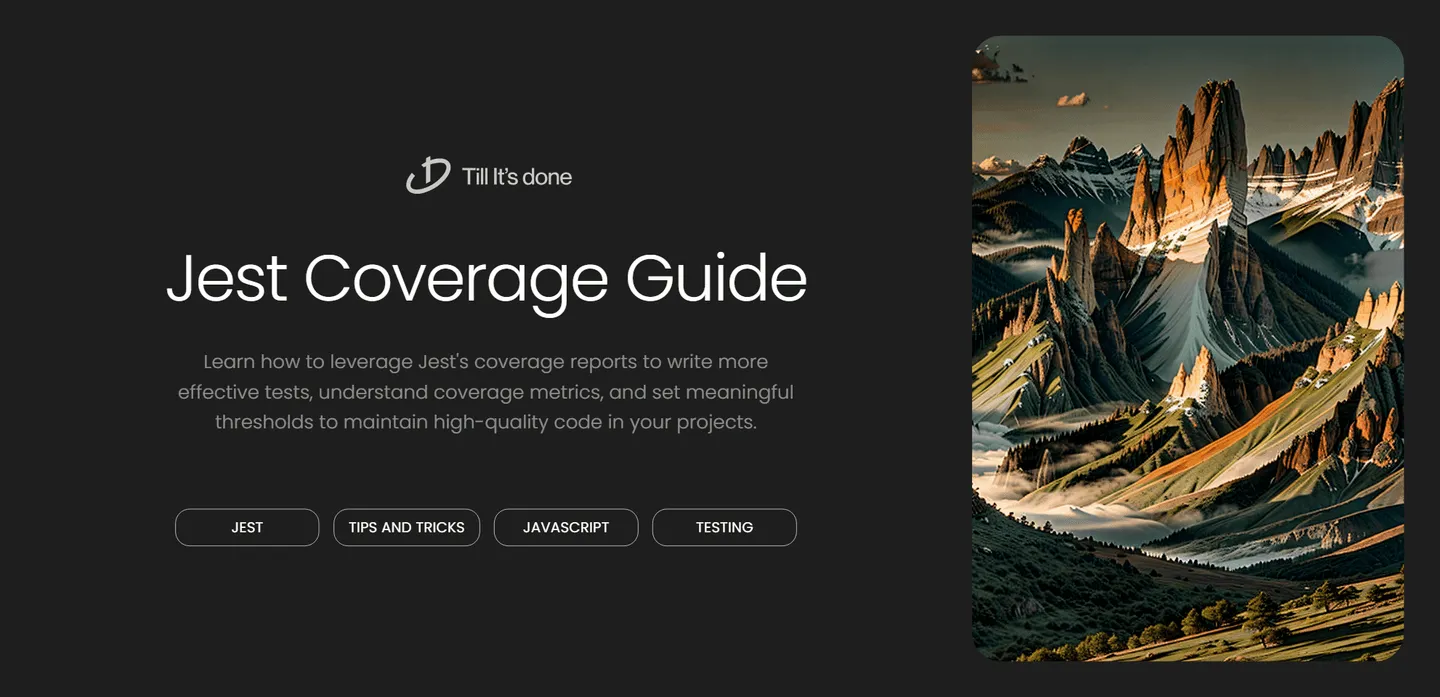
พูดคุยกับซีอีโอ
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.