- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing BLoC in Flutter: A Step-by-Step Guide
Covers testing fundamentals, environment setup, writing tests, and best practices for robust Flutter applications.
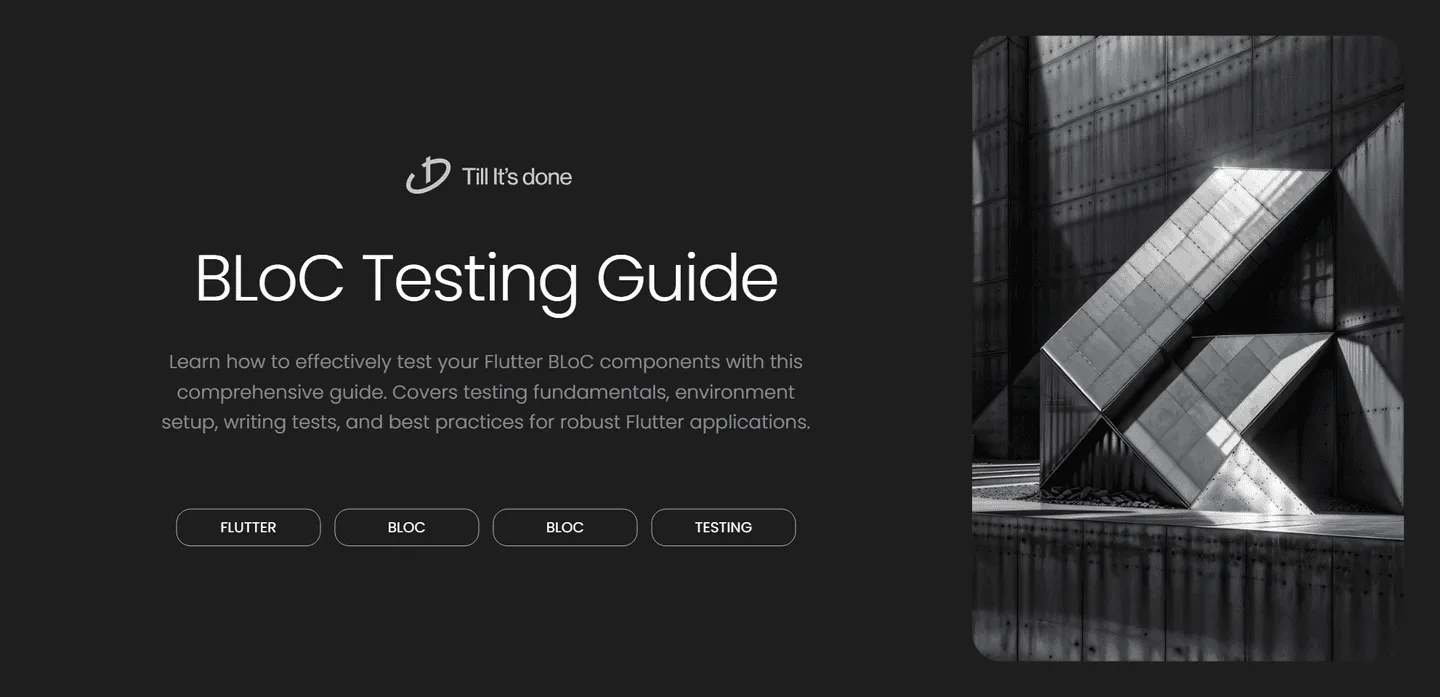
Testing BLoC in Flutter: A Step-by-Step Guide
Testing is a crucial aspect of developing robust Flutter applications, especially when working with state management solutions like BLoC (Business Logic Component). In this comprehensive guide, we’ll explore how to effectively test your BLoC components to ensure your app’s reliability and maintainability.
Understanding BLoC Testing Fundamentals
Before diving into the technical details, it’s essential to understand what we’re actually testing in a BLoC. A BLoC component typically consists of three main elements: events, states, and the business logic that transforms events into states.
Setting Up Your Testing Environment
First, let’s set up our testing environment by adding the necessary dependencies to our pubspec.yaml
file:
dev_dependencies: bloc_test: ^9.1.0 test: ^1.24.0 mockito: ^5.4.0
Writing Your First BLoC Test
Let’s start with a simple counter BLoC test. We’ll test a basic increment functionality:
void main() { group('CounterBloc', () { late CounterBloc counterBloc;
setUp(() { counterBloc = CounterBloc(); });
tearDown(() { counterBloc.close(); });
test('initial state should be 0', () { expect(counterBloc.state, equals(0)); });
blocTest<CounterBloc, int>( 'emits [1] when Increment is added', build: () => CounterBloc(), act: (bloc) => bloc.add(Increment()), expect: () => [1], ); });}
Testing Complex BLoC Scenarios
When testing more complex scenarios, we need to consider:
- Multiple state changes
- Error handling
- Dependencies and external services
- Asynchronous operations
Here’s an example testing a BLoC with asynchronous behavior:
blocTest<UserBloc, UserState>( 'emits [loading, success] when fetching user data succeeds', build: () { when(mockUserRepository.getUser()) .thenAnswer((_) async => User('John Doe')); return UserBloc(userRepository: mockUserRepository); }, act: (bloc) => bloc.add(FetchUser()), expect: () => [ UserLoading(), UserSuccess(User('John Doe')), ],);
Best Practices for BLoC Testing
- Test one behavior at a time
- Use meaningful test descriptions
- Mock external dependencies
- Test error scenarios
- Verify state transitions
- Keep tests maintainable
Common Pitfalls to Avoid
- Don’t test implementation details
- Avoid testing trivial code
- Don’t forget to test error cases
- Don’t skip testing edge cases
Conclusion
Testing your BLoC components is crucial for maintaining a reliable Flutter application. By following these guidelines and best practices, you can create a robust test suite that helps catch bugs early and makes your codebase more maintainable.
Remember to run your tests frequently and integrate them into your CI/CD pipeline. Happy testing!
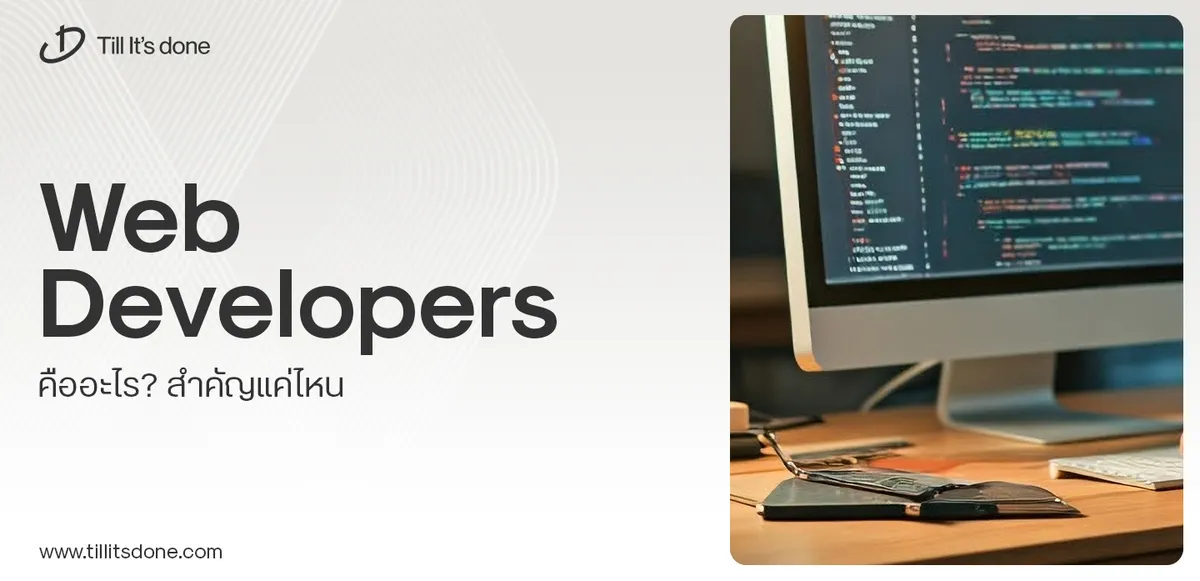
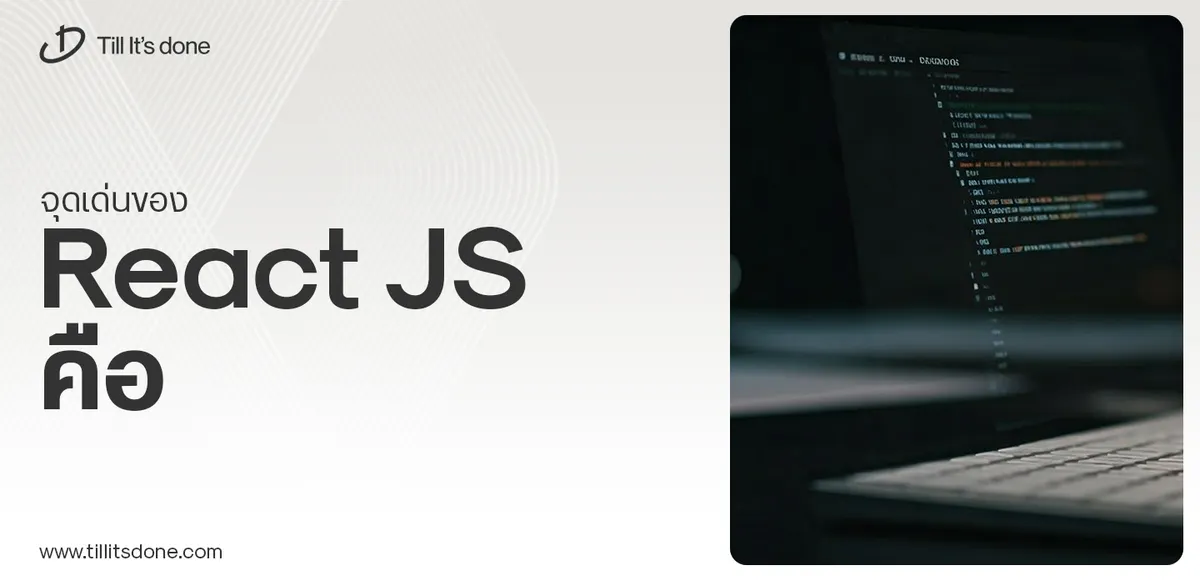
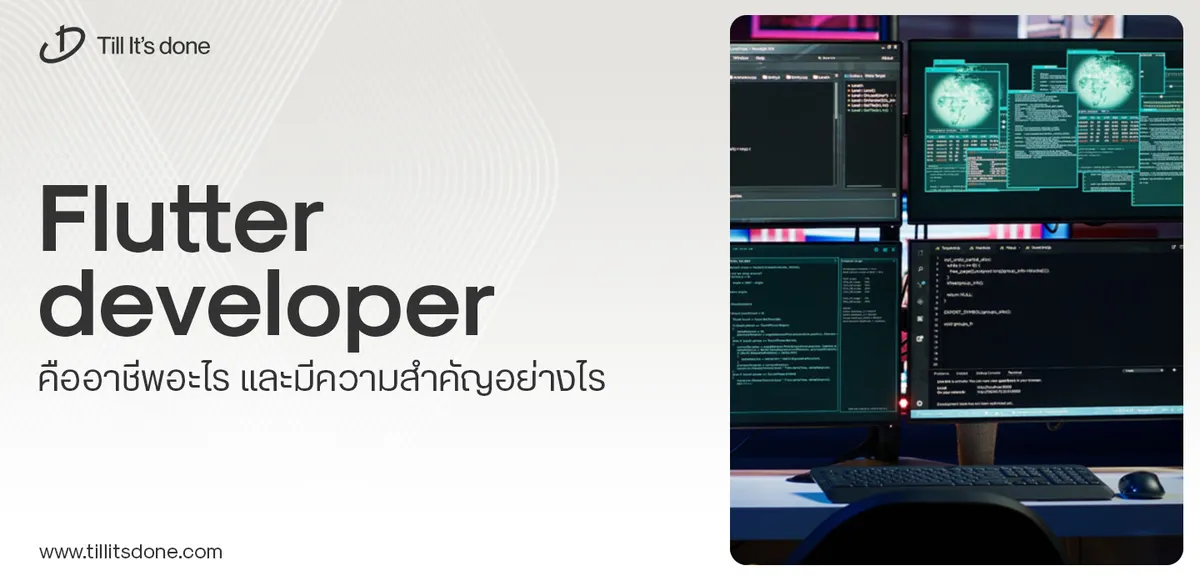
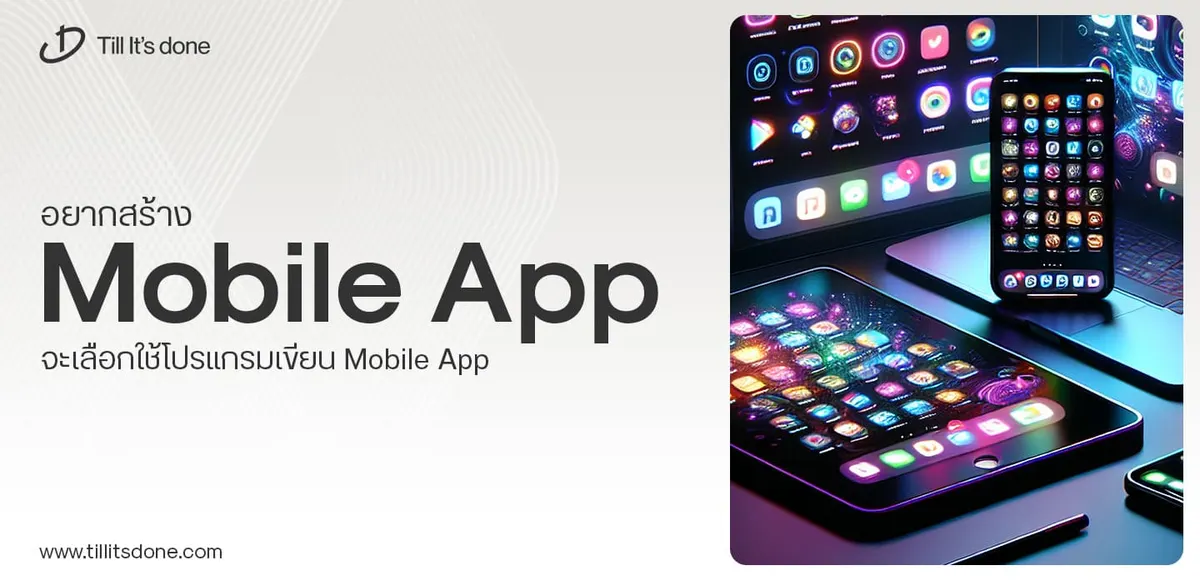
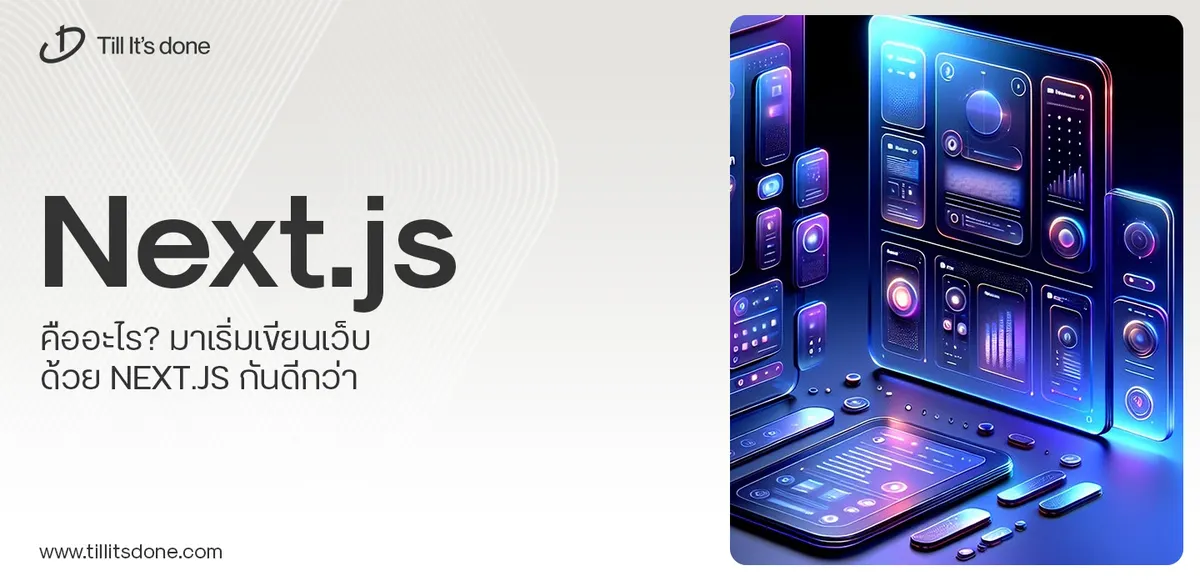
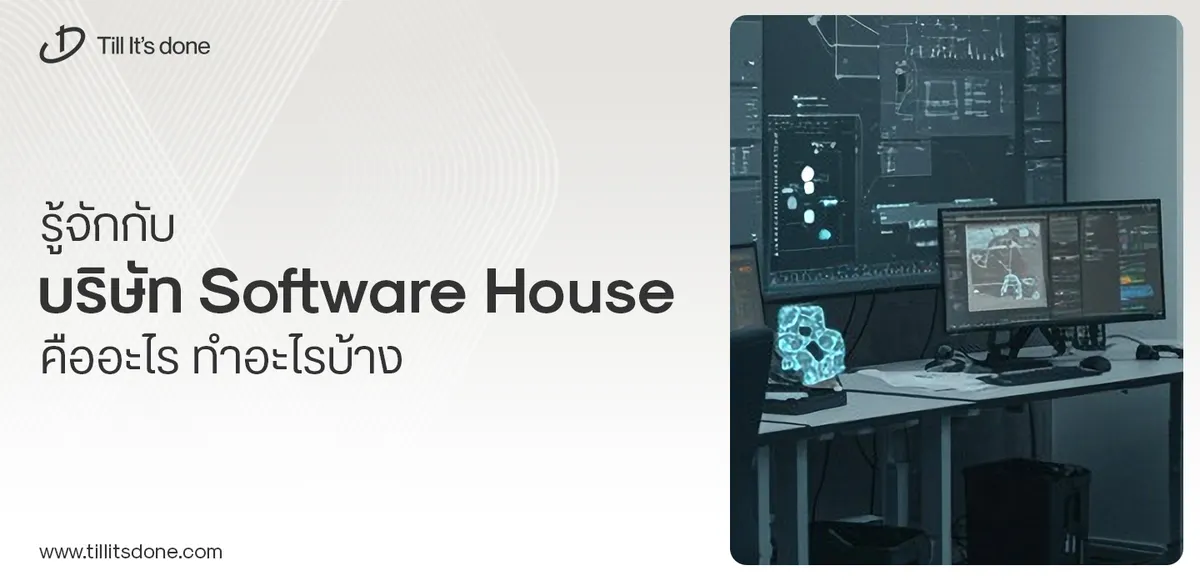
พูดคุยกับซีอีโอ
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.