- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Effective Error Handling in Go: Best Practices
Discover how to implement robust error handling patterns, custom error types, and production-ready error management strategies.

Effective Error Handling in Go: Best Practices for Robust Applications
Error handling is a crucial aspect of writing reliable and maintainable Go applications. Unlike many other programming languages that use exceptions, Go takes a different approach by making errors first-class citizens. Let’s dive into some best practices that will help you handle errors effectively in your Go applications.
Understanding Go’s Error Philosophy
Go’s error handling philosophy is straightforward: errors are values. This simple yet powerful concept means that errors can be handled just like any other data type. The language encourages explicit error checking, making code more predictable and easier to debug.
Key Best Practices
1. Always Check Errors
One of the fundamental rules in Go is to never ignore errors. While it might be tempting to bypass error checking during rapid development, this practice can lead to subtle bugs that are difficult to track down later.
file, err := os.Open("config.json")if err != nil { return fmt.Errorf("failed to open config: %w", err)}defer file.Close()
2. Use Custom Error Types
When building larger applications, custom error types can provide more context and make error handling more specific:
type ValidationError struct { Field string Message string}
func (v *ValidationError) Error() string { return fmt.Sprintf("%s: %s", v.Field, v.Message)}
3. Wrapping Errors with Context
Go 1.13 introduced error wrapping, which allows you to add context to errors while preserving the original error:
if err != nil { return fmt.Errorf("processing payment failed: %w", err)}
4. Error Handling Patterns
The Sentinel Error Pattern
For specific error conditions that clients might want to check for, use predefined error values:
var ( ErrNotFound = errors.New("resource not found") ErrInvalidInput = errors.New("invalid input"))
The Error Type Pattern
When you need to provide additional information about an error:
type RequestError struct { StatusCode int Err error}
func (r *RequestError) Error() string { return fmt.Sprintf("status %d: %v", r.StatusCode, r.Err)}
Best Practices for Production Applications
- Log errors appropriately with context
- Don’t expose sensitive information in error messages
- Use structured error handling for better debugging
- Implement proper error recovery mechanisms
- Consider using error aggregation for batch operations
Conclusion
Effective error handling is not just about catching and returning errors—it’s about building robust applications that can gracefully handle unexpected situations. By following these best practices, you can create more reliable and maintainable Go applications that are easier to debug and support in production environments.
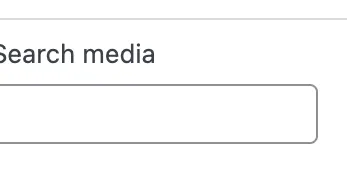





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.