- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Writing Clean and Maintainable Go Code Guide
Discover tips on package organization, interface design, error handling, and testing to create more efficient and sustainable Go applications.
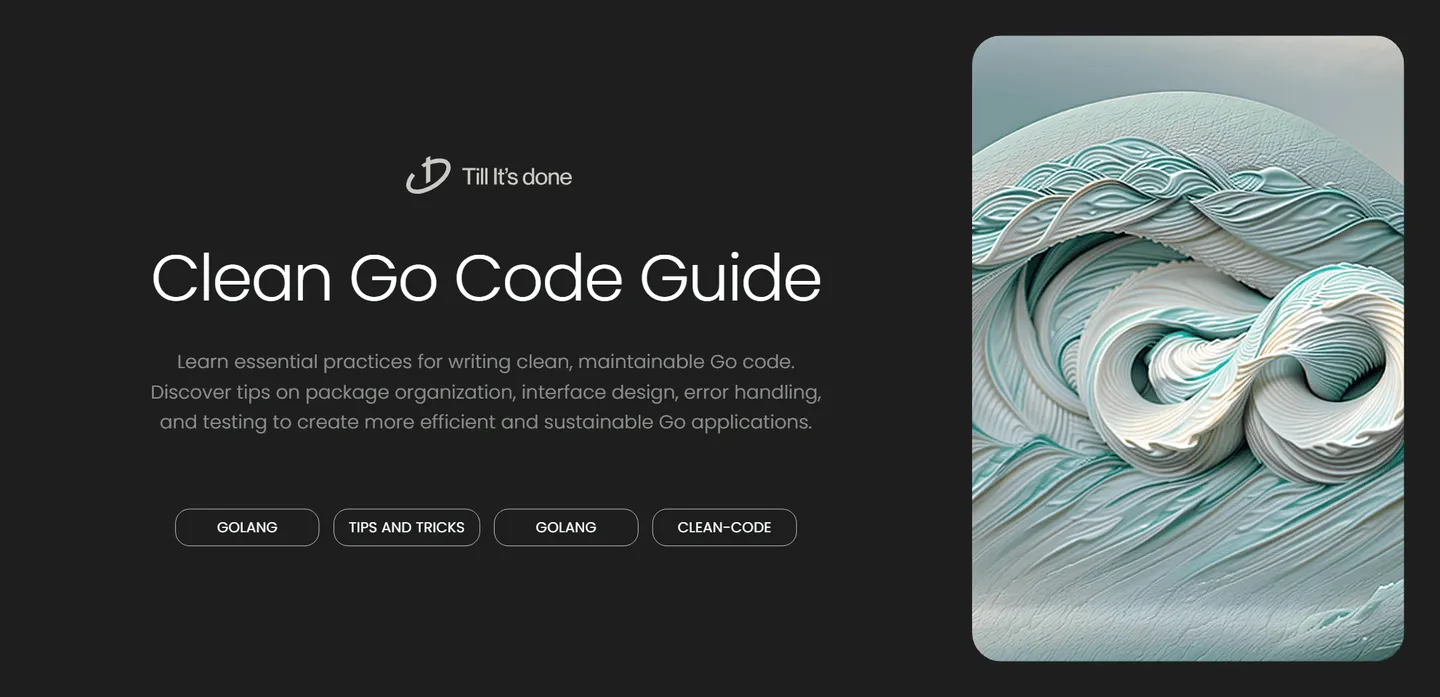
Writing Clean and Maintainable Go Code: A Practical Guide
As Go continues to gain popularity in the software development world, writing clean and maintainable code has become more crucial than ever. Let’s explore some battle-tested practices that will help you write better Go code and make your codebase a joy to work with.
Embrace Go’s Simplicity
Go was designed with simplicity in mind, and this philosophy should reflect in your code. Instead of creating complex abstractions, focus on writing straightforward, readable code that future maintainers (including yourself) will thank you for.
Package Organization
One of the most important aspects of maintainable Go code is proper package organization. Follow these principles:
Package Naming
- Use short, concise package names that describe their purpose
- Avoid generic names like “util” or “common”
- Use lowercase names without underscores
// Goodpackage userauth
// Not so goodpackage user_authentication_utils
Package Structure
Keep your packages focused and cohesive. Each package should have a single, well-defined purpose. A good rule of thumb is that if you can’t describe the package’s purpose in one sentence, it might be doing too much.
Interface Design
Go’s interfaces are one of its most powerful features when used correctly. Keep them small and focused:
// Goodtype Reader interface { Read(p []byte) (n int, err error)}
// Not so goodtype DoEverything interface { Read(p []byte) (n int, err error) Write(p []byte) (n int, err error) Close() error Flush() error // ... many more methods}
Error Handling
Proper error handling is crucial for maintaining reliable Go applications:
- Always check errors
- Create custom error types when needed
- Use meaningful error messages
- Wrap errors with context using
fmt.Errorf
and%w
if err != nil { return fmt.Errorf("failed to process user data: %w", err)}
Code Organization
Function Design
- Keep functions focused and small
- Use meaningful variable names
- Return early for error conditions
- Limit the number of parameters
// Goodfunc processUser(user User) error { if err := validateUser(user); err != nil { return fmt.Errorf("invalid user: %w", err) }
return saveUser(user)}
// Not so goodfunc process(u User, d Database, c Cache, l Logger, m Metrics) (User, error) { // ... many lines of code}
Testing
Write tests that are:
- Clear and concise
- Focused on one thing
- Easy to understand what’s being tested
- Using table-driven tests when appropriate
func TestValidateUser(t *testing.T) { tests := []struct { name string user User wantErr bool }{ { name: "valid user", user: User{Name: "John", Age: 25}, wantErr: false, }, { name: "invalid age", user: User{Name: "John", Age: -1}, wantErr: true, }, }
for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { err := validateUser(tt.user) if (err != nil) != tt.wantErr { t.Errorf("validateUser() error = %v, wantErr %v", err, tt.wantErr) } }) }}
Documentation
Good documentation is crucial for maintainable code:
- Write clear package documentation
- Document exported functions and types
- Include examples in documentation
- Use meaningful comments that explain “why” rather than “what”
Conclusion
Writing clean and maintainable Go code is more about discipline and consistency than clever tricks. Follow these practices, and your codebase will be easier to maintain, debug, and extend. Remember, the best code is often the simplest code that gets the job done effectively.
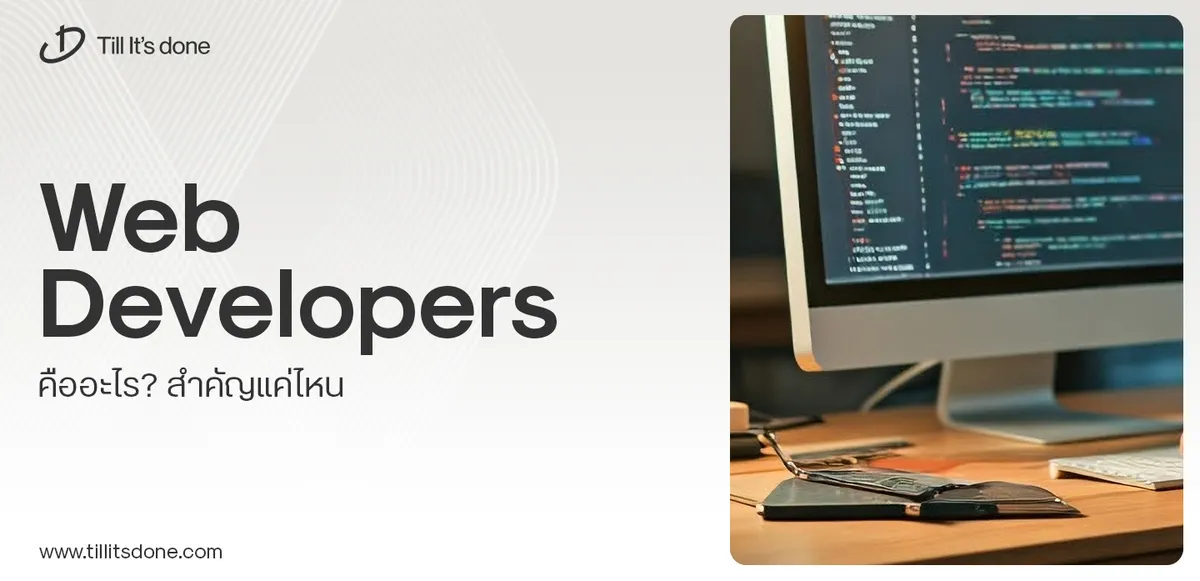
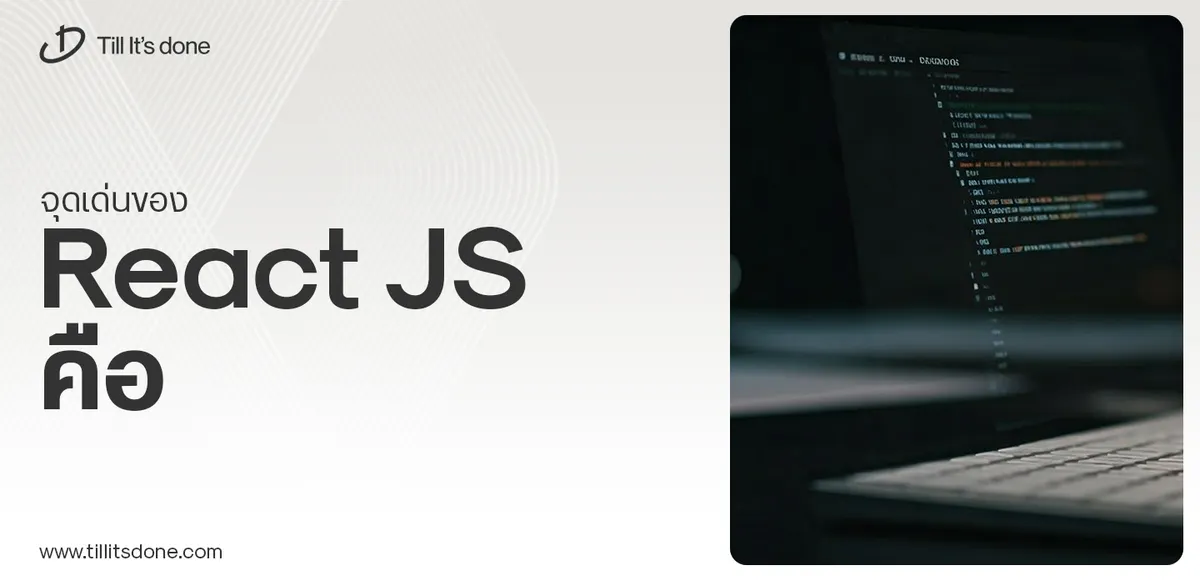
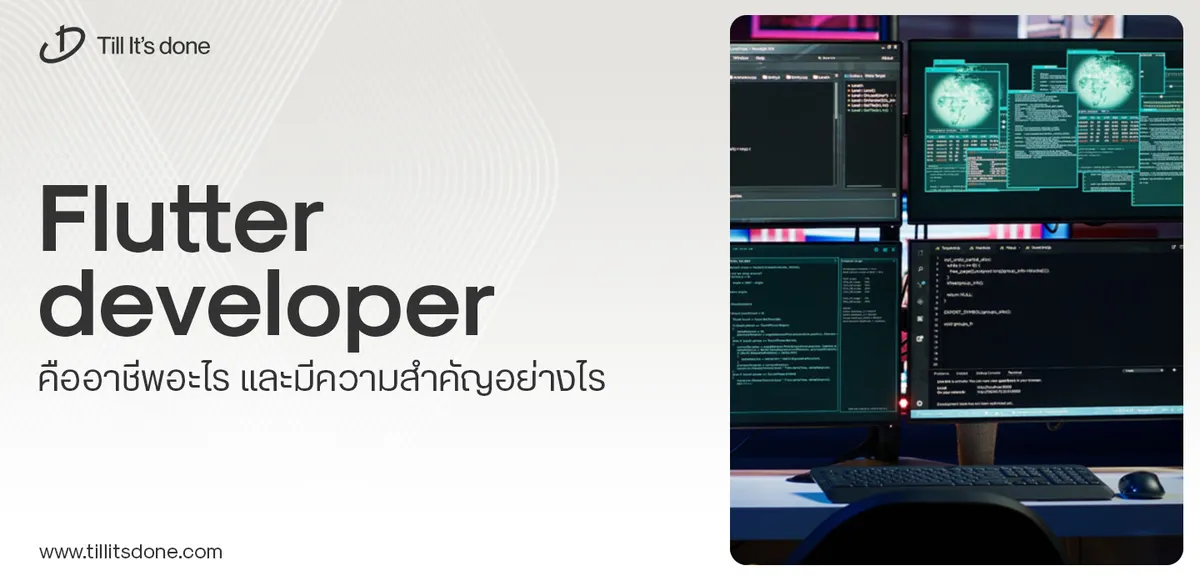
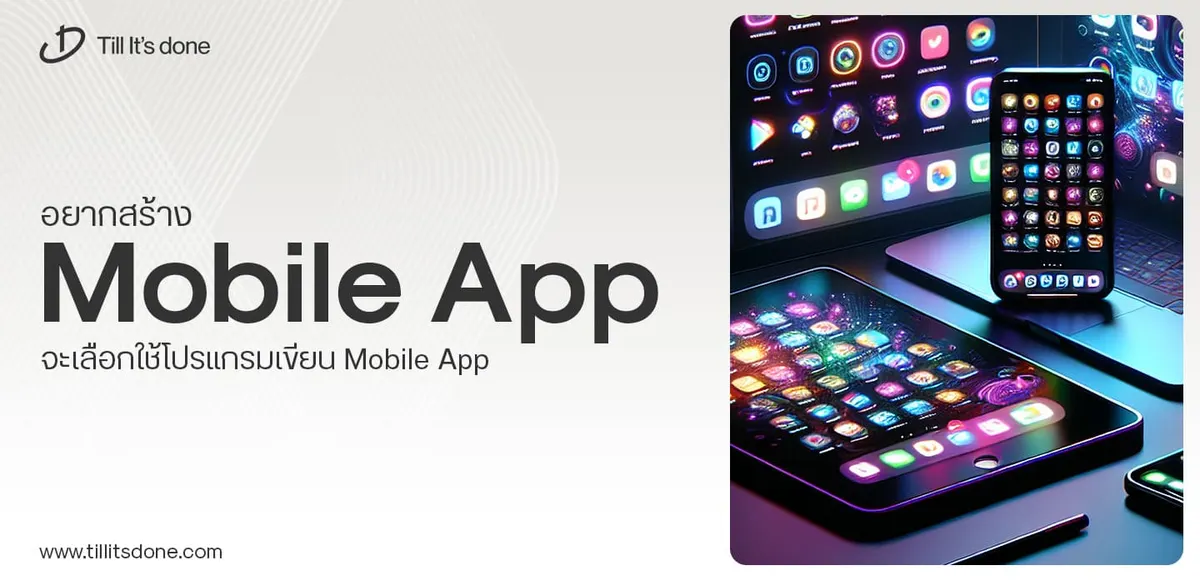
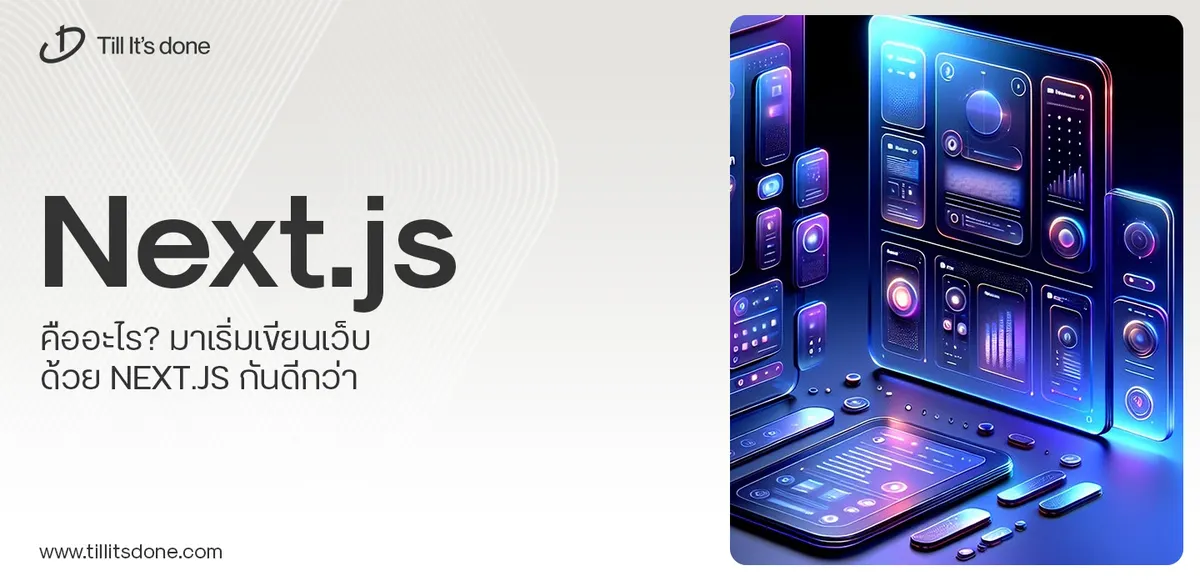
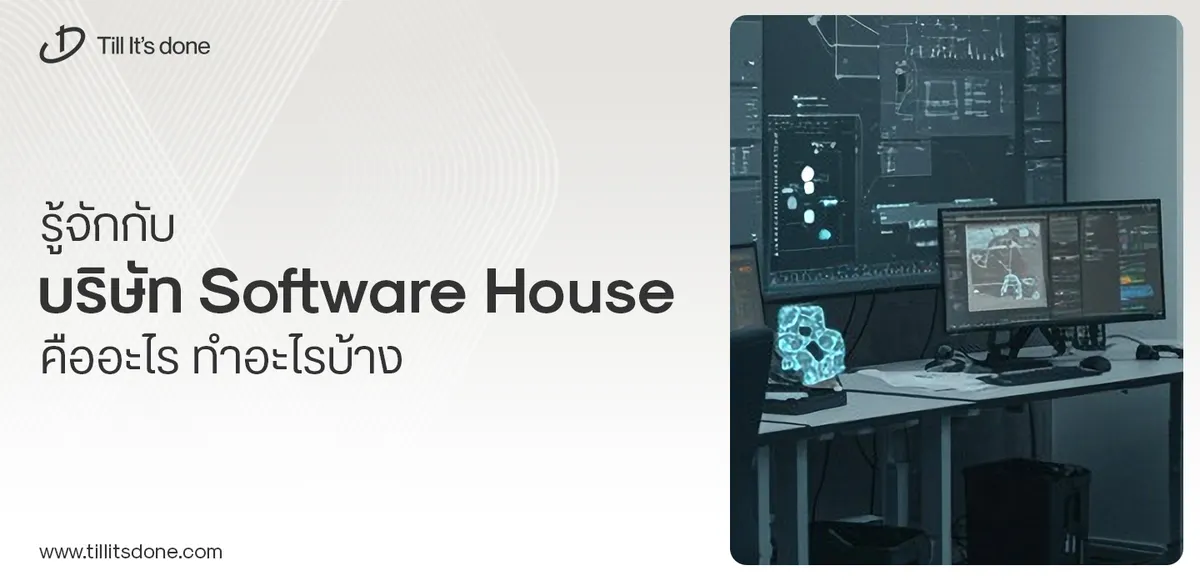
พูดคุยกับซีอีโอ
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.