- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Use Zustand with React Context
Discover best practices, implementation tips, and performance optimization techniques.

How to Use Zustand with React Context for Global State Management
State management in React applications can sometimes feel overwhelming, but it doesn’t have to be. Today, let’s explore how to combine the simplicity of Zustand with the power of React Context to create an elegant state management solution that’s both powerful and developer-friendly.
Understanding the Basics
Zustand has been gaining popularity in the React ecosystem, and for good reason. It’s lightweight, has zero dependencies, and doesn’t require complex boilerplate code. When combined with React Context, it becomes even more powerful, allowing us to create a state management system that’s both efficient and easy to maintain.
Let’s dive into how we can implement this combination effectively.
Setting Up the Store
First, let’s create a simple Zustand store. The beauty of Zustand lies in its straightforward API:
import create from 'zustand'
interface BearStore { bears: number increasePopulation: () => void removeAllBears: () => void}
const useStore = create<BearStore>((set) => ({ bears: 0, increasePopulation: () => set((state) => ({ bears: state.bears + 1 })), removeAllBears: () => set({ bears: 0 }),}))
Creating the Context
Now, let’s wrap this store in a React Context to make it available throughout our application:
import { createContext, useContext } from 'react'
const StoreContext = createContext(null)
export const StoreProvider = ({ children }) => ( <StoreContext.Provider value={useStore}> {children} </StoreContext.Provider>)
Using the Combined Solution
The real magic happens when we start using our store throughout the application. Here’s how simple it becomes:
const BearCounter = () => { const bears = useStore((state) => state.bears) return <h1>{bears} bears around here</h1>}
const Controls = () => { const increasePopulation = useStore((state) => state.increasePopulation) return <button onClick={increasePopulation}>Add bear</button>}
Benefits of This Approach
- Simplified State Management: Zustand’s minimal API combined with React Context provides a clean and intuitive way to manage global state.
- Improved Performance: Zustand’s built-in optimization ensures components only re-render when necessary.
- TypeScript Support: Full TypeScript support out of the box makes development more robust and maintainable.
- Easy Testing: The simplicity of the store structure makes it straightforward to test and debug.
Best Practices and Tips
- Keep your store logic separated by concern
- Use selective updates to prevent unnecessary re-renders
- Leverage TypeScript for better type safety
- Consider using middleware for additional functionality
Conclusion
The combination of Zustand and React Context provides a powerful yet simple solution for state management in React applications. It offers the best of both worlds: the simplicity and performance of Zustand with the global accessibility of React Context.




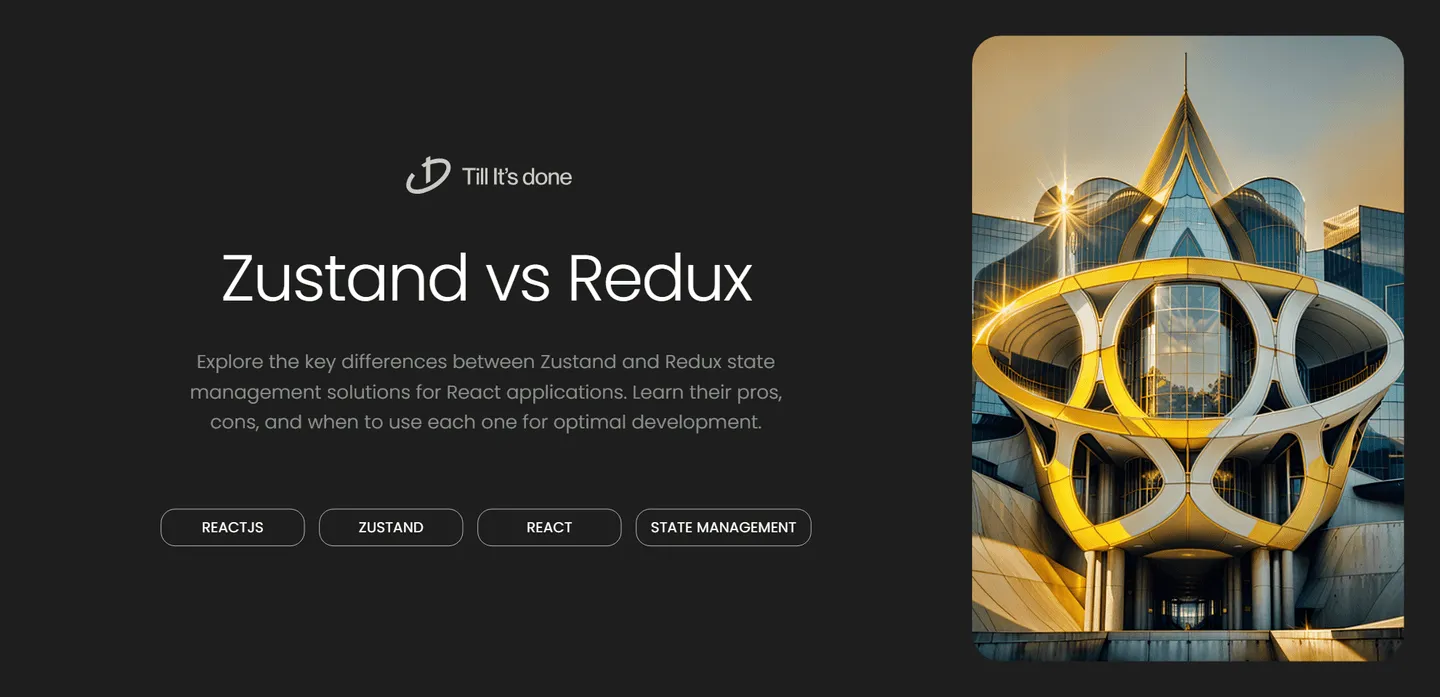

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.