- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Zustand + TypeScript: State Management Guide
Learn best practices, performance optimization tips, and advanced patterns for building scalable applications.
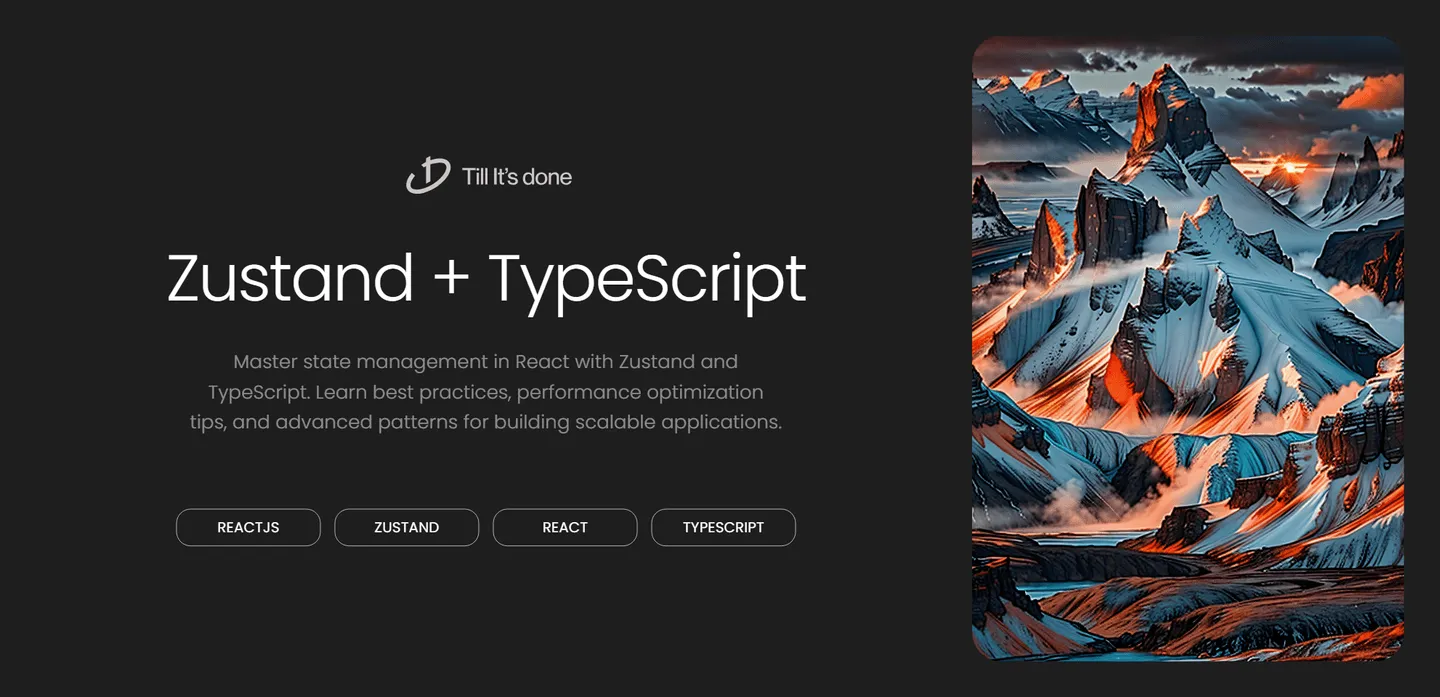
Using Zustand in a TypeScript Project: Tips and Best Practices
Have you ever found yourself wrestling with complex state management in your React applications? While Redux has been the go-to solution for many developers, Zustand has emerged as a refreshingly simple yet powerful alternative. In this guide, we’ll explore how to effectively use Zustand with TypeScript to create maintainable and type-safe state management solutions.
Why Choose Zustand?
Zustand’s philosophy is simple: provide powerful state management without the boilerplate. Unlike traditional state management solutions, Zustand offers a minimalist API that feels natural in TypeScript projects. It’s lightweight, has zero dependencies, and doesn’t require providers wrapping your React tree.
Setting Up Your Store
One of Zustand’s strengths is its straightforward TypeScript integration. Let’s look at how to create a properly typed store:
import create from 'zustand'
interface TodoState { todos: string[] addTodo: (todo: string) => void removeTodo: (index: number) => void}
const useTodoStore = create<TodoState>((set) => ({ todos: [], addTodo: (todo) => set((state) => ({ todos: [...state.todos, todo] })), removeTodo: (index) => set((state) => ({ todos: state.todos.filter((_, i) => i !== index) }))}))
Best Practices for Scaling
When your application grows, organizing your store becomes crucial. Here are some tried-and-true patterns I’ve found effective:
-
Split Your Store: Rather than creating one massive store, split it into logical modules. This makes maintenance and testing much easier.
-
Use TypeScript’s Utility Types: Leverage
Partial\<T\>
andPick\<T\>
to create more flexible and reusable store slices. -
Implement Computed Values: Zustand works beautifully with derived state.
Advanced Patterns
One pattern I particularly love is combining multiple stores when needed:
const useComputedStore = create<ComputedState>((set, get) => ({ // ... store implementation derivedValue: () => { const value = get().someValue return someComputation(value) }}))
Performance Optimization Tips
Remember these key points to keep your Zustand stores performing optimally:
- Use shallow selectors for basic state selection
- Implement memoization for complex computations
- Keep your store structure flat when possible
- Utilize TypeScript’s strict mode for better type safety
Conclusion
Zustand’s simplicity, combined with TypeScript’s type safety, creates a powerful duo for state management. By following these patterns and best practices, you’ll be well-equipped to build scalable and maintainable React applications.
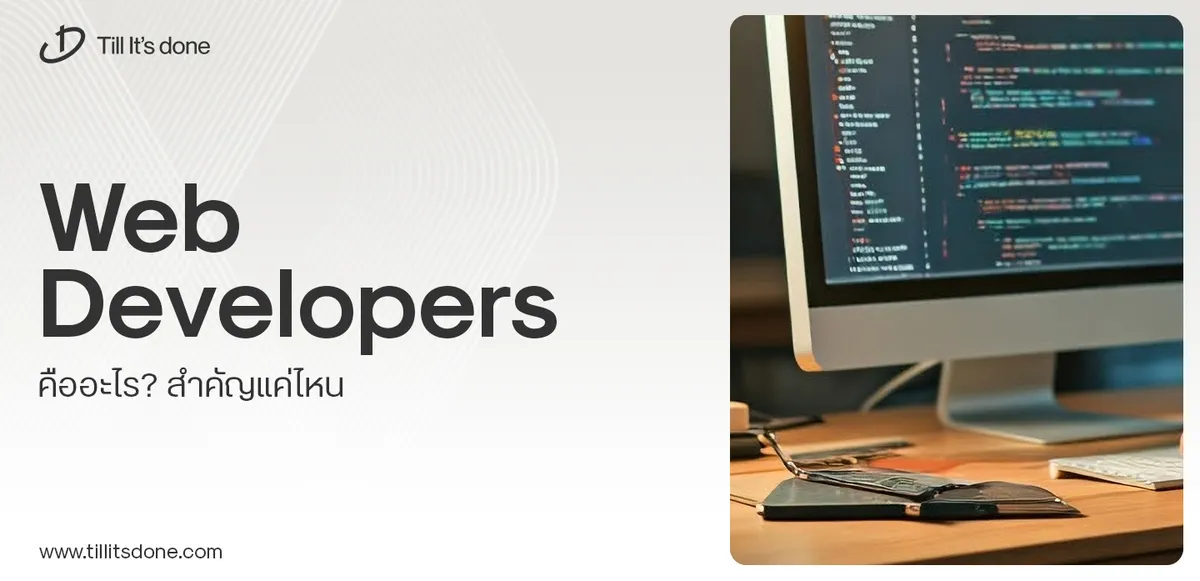
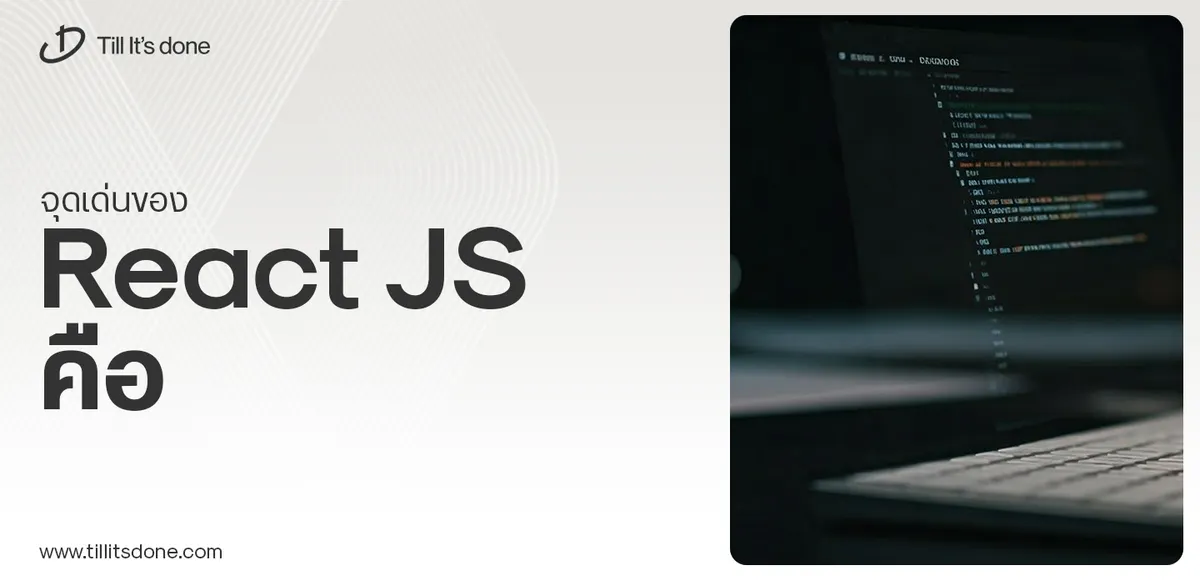
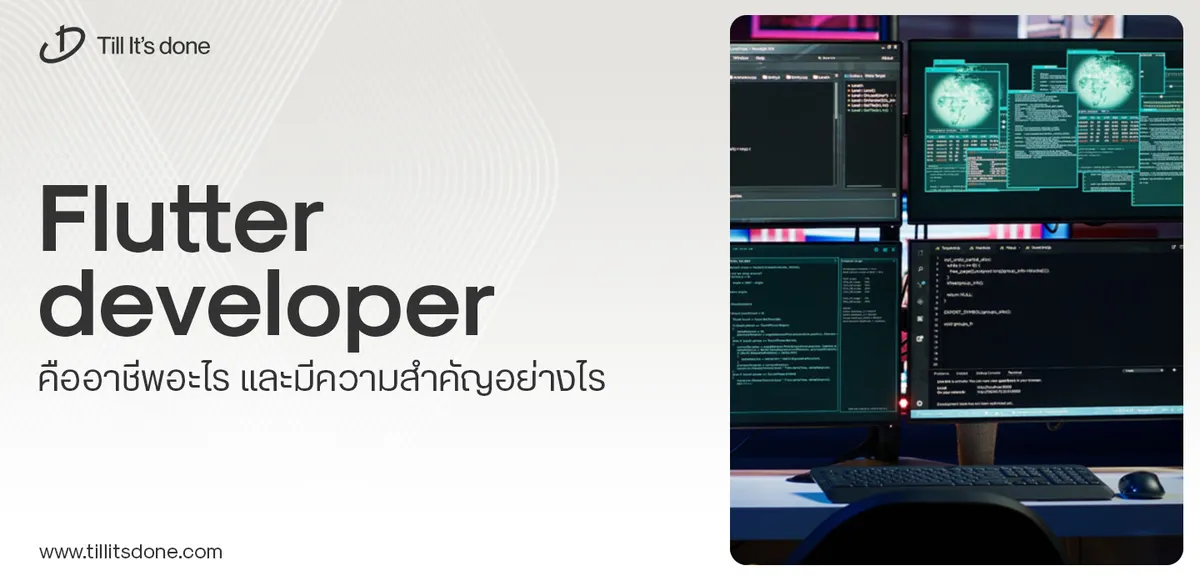
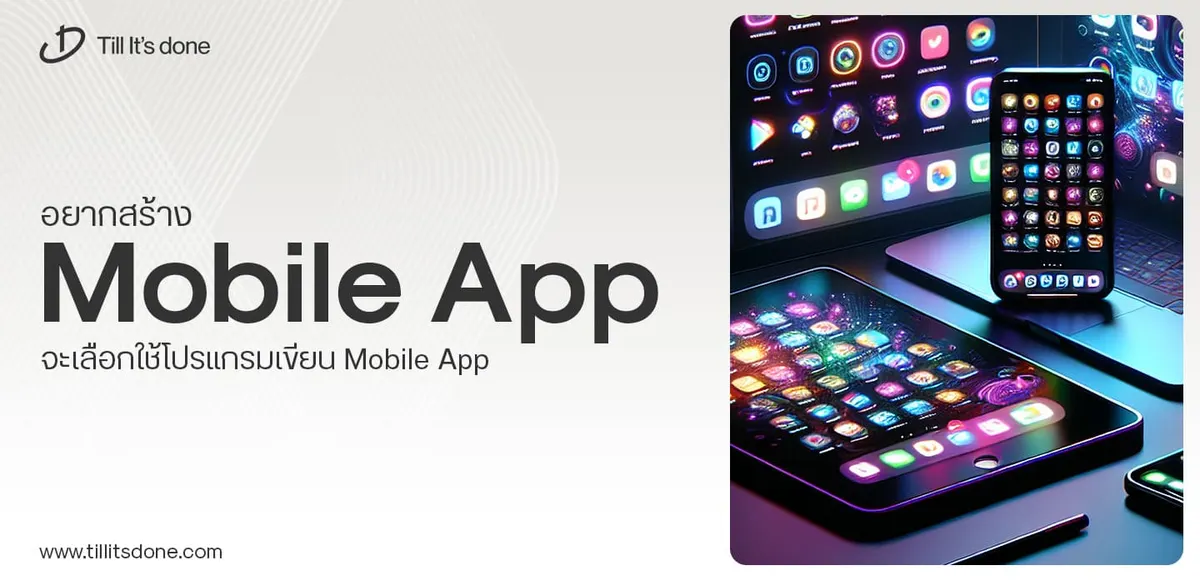
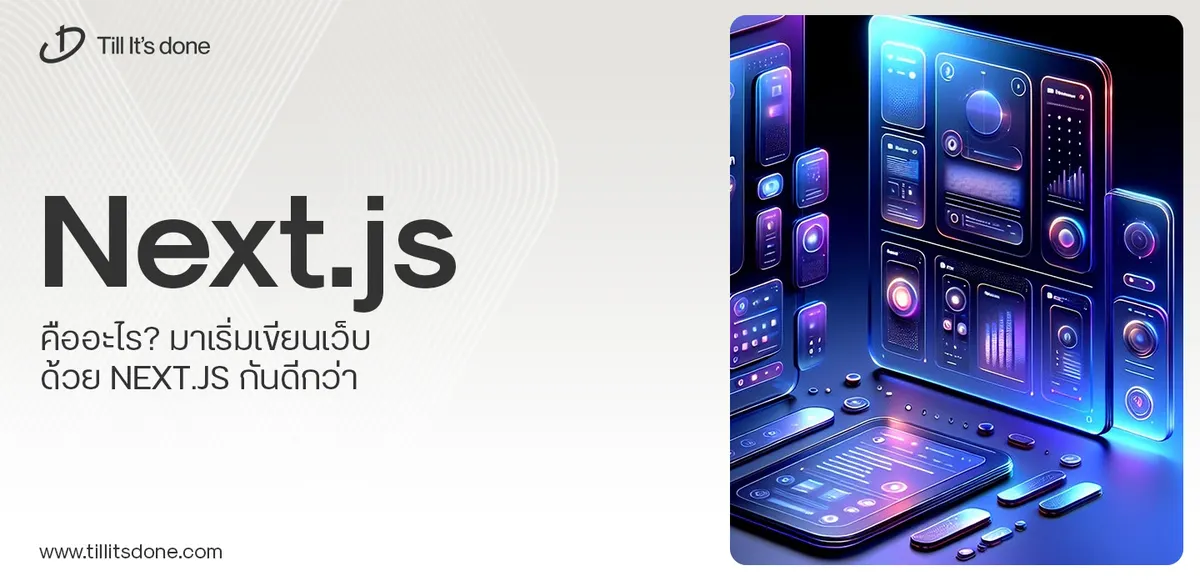
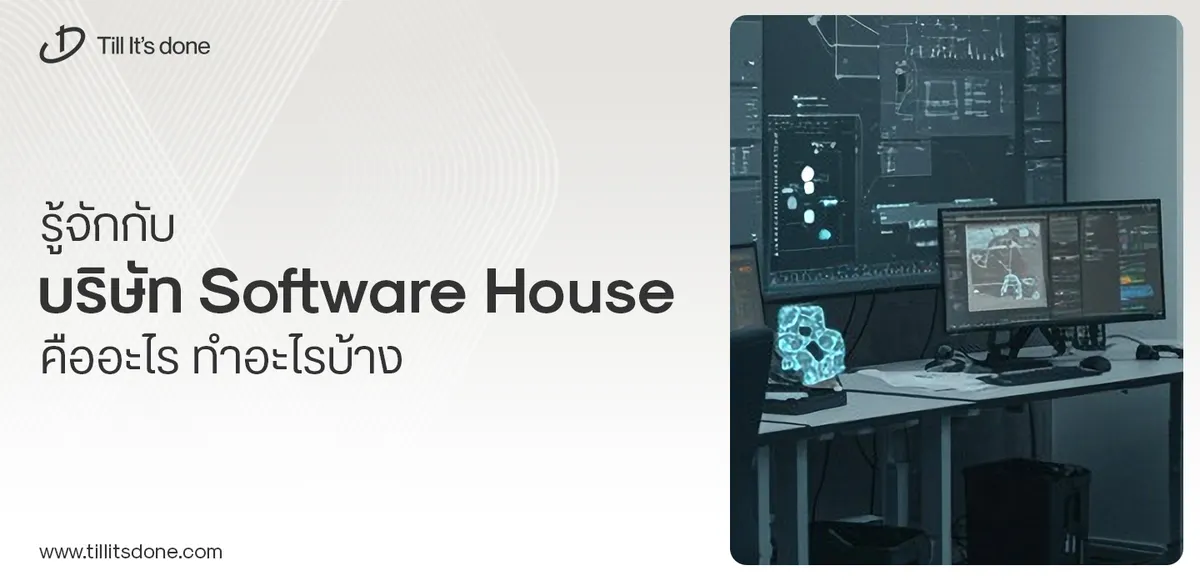
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.