- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Integrate Zod with Astro.js for Type Safety
This guide covers setup, schema creation, form validation, API routes, and best practices for type safety.
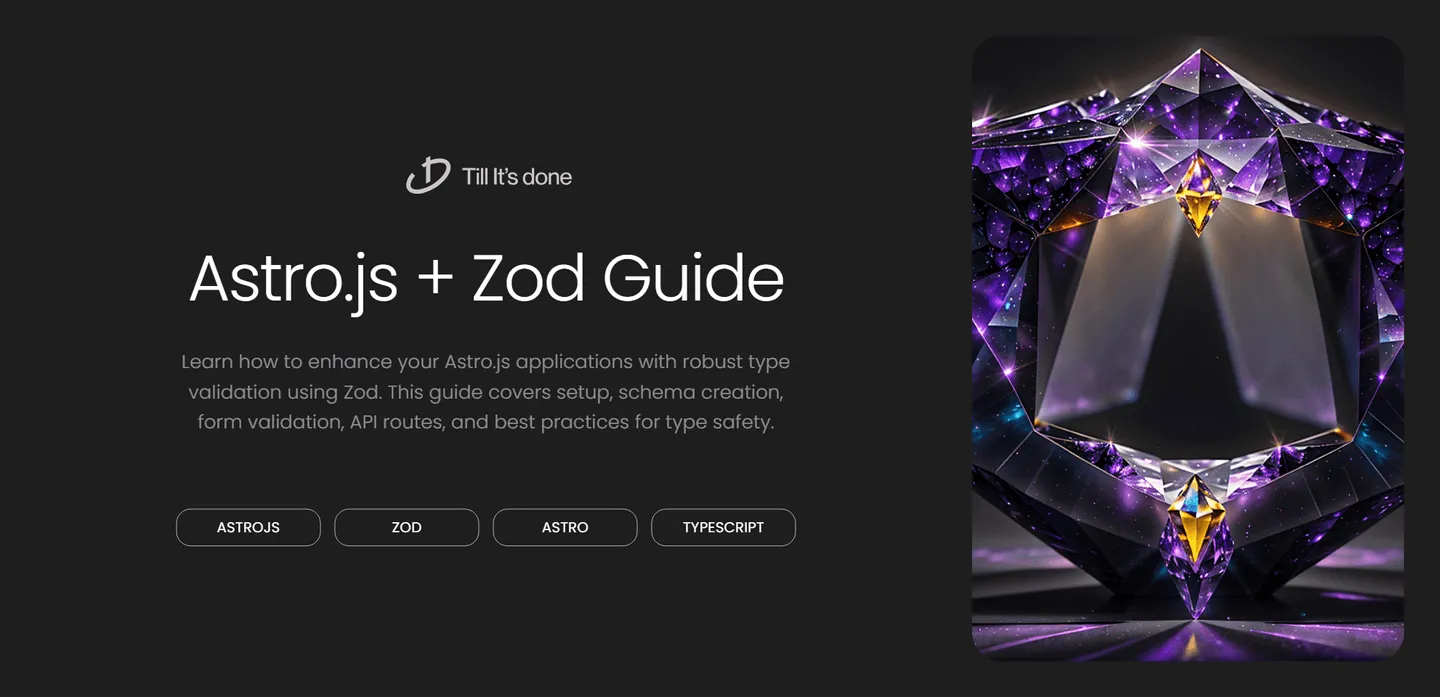
How to Integrate Zod with Astro.js for Type Safety
Type safety is crucial for building robust web applications, and when working with Astro.js, Zod provides an excellent solution for runtime type validation. Today, I’ll walk you through integrating Zod with your Astro.js projects to create more reliable and maintainable applications.
Why Zod?
Before diving into the implementation, let’s understand why Zod is such a powerful choice. As a TypeScript-first schema validation library, Zod ensures your data maintains its expected shape not just during development, but also at runtime. This is particularly valuable in Astro.js applications where we often work with various data sources and user inputs.
Setting Up Zod in Your Astro Project
First, let’s add Zod to your Astro project. It’s as simple as running:
npm install zod
Creating Your First Schema
Let’s start with a practical example. Imagine we’re building a blog with a structured content model. Here’s how you might define your post schema:
import { z } from 'zod';
export const PostSchema = z.object({ title: z.string().min(1).max(100), slug: z.string().min(1), content: z.string(), publishedDate: z.date(), tags: z.array(z.string()).default([])});
export type Post = z.infer<typeof PostSchema>;
Practical Use Cases
Form Validation
One of the most common use cases is form validation in Astro components:
---import { z } from 'zod';
const ContactSchema = z.object({ email: z.string().email(), message: z.string().min(10)});
const formData = await Astro.request.formData();const result = ContactSchema.safeParse({ email: formData.get('email'), message: formData.get('message')});---
API Route Validation
Zod really shines when validating API endpoints in Astro:
import type { APIRoute } from 'astro';import { PostSchema } from '../schemas';
export const post: APIRoute = async ({ request }) => { try { const data = await request.json(); const validatedData = PostSchema.parse(data);
// Process validated data... return new Response(JSON.stringify({ success: true }), { status: 200 }); } catch (error) { return new Response(JSON.stringify({ error: 'Invalid data' }), { status: 400 }); }};
Best Practices
- Reuse Schemas: Define your schemas in a central location and import them where needed.
- Custom Error Messages: Customize your error messages for better user experience:
const UserSchema = z.object({ username: z.string().min(3, { message: "Username must be at least 3 characters long" })});
- Transform Data: Use Zod’s transform method to modify data during validation:
const DateSchema = z.string().transform((str) => new Date(str));
Advanced Tips
Composing Schemas
You can compose complex schemas from simpler ones:
const BasePostSchema = z.object({ title: z.string(), content: z.string()});
const PublishedPostSchema = BasePostSchema.extend({ publishedAt: z.date(), author: z.string()});
Optional Fields and Defaults
Zod makes it easy to handle optional fields and default values:
const ConfigSchema = z.object({ theme: z.enum(['light', 'dark']).default('light'), notifications: z.boolean().optional()});
Conclusion
Integrating Zod with Astro.js provides a robust foundation for type-safe applications. By validating data at runtime, you can catch errors early and provide better feedback to users. Remember to start simple and gradually build up your validation logic as your application grows.
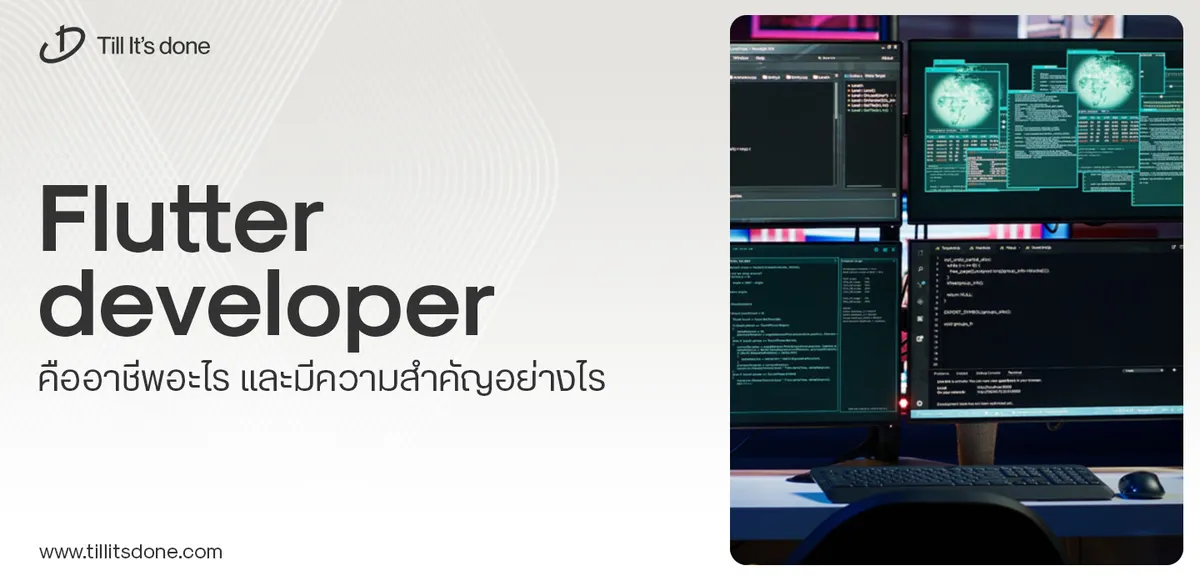
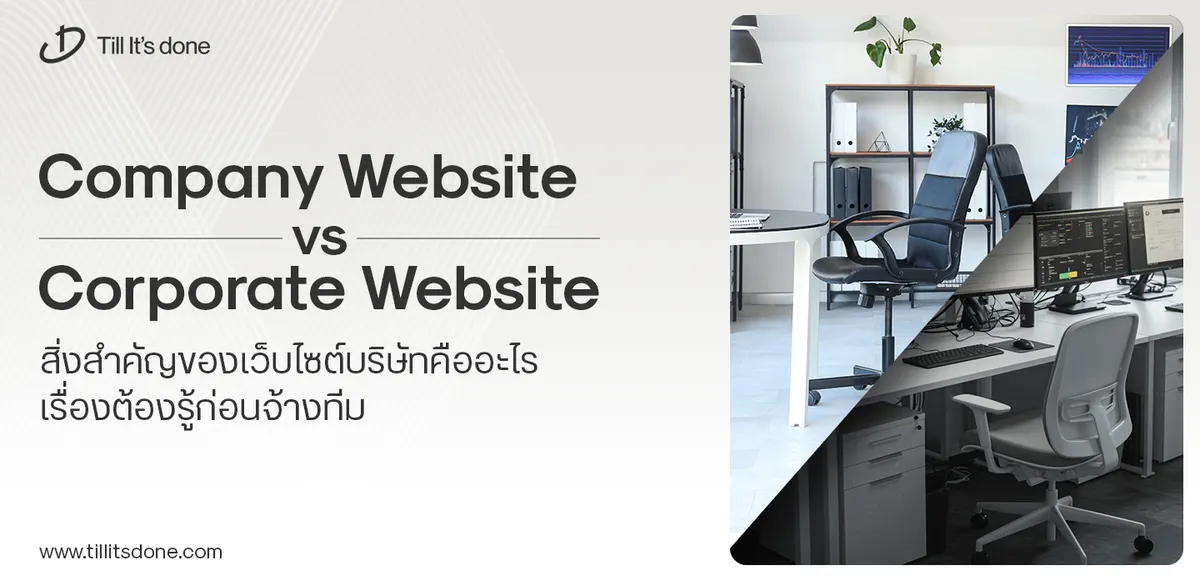
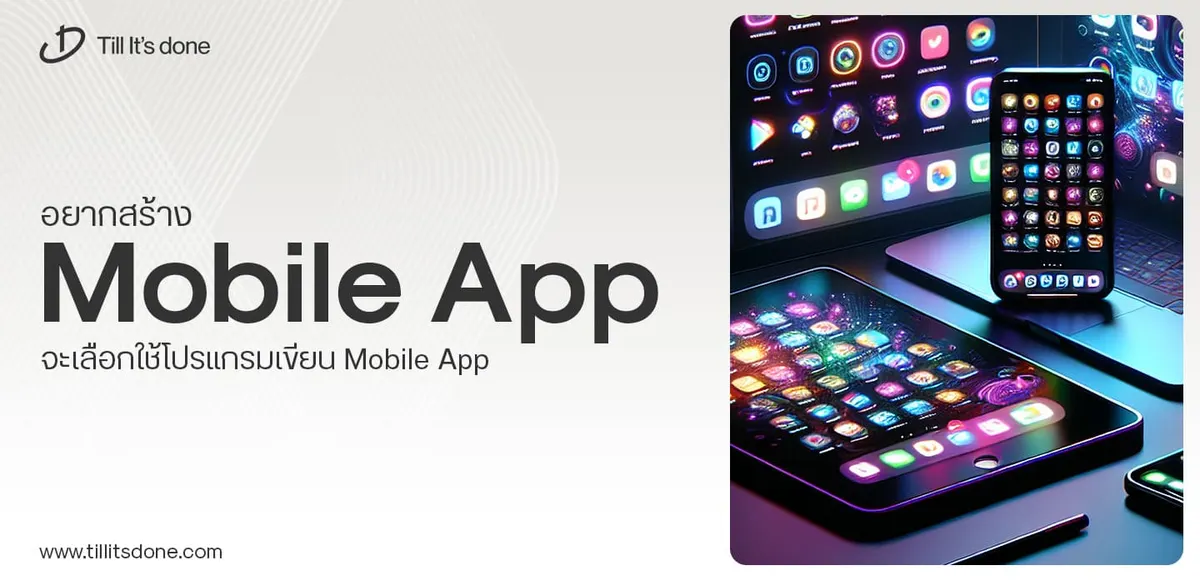
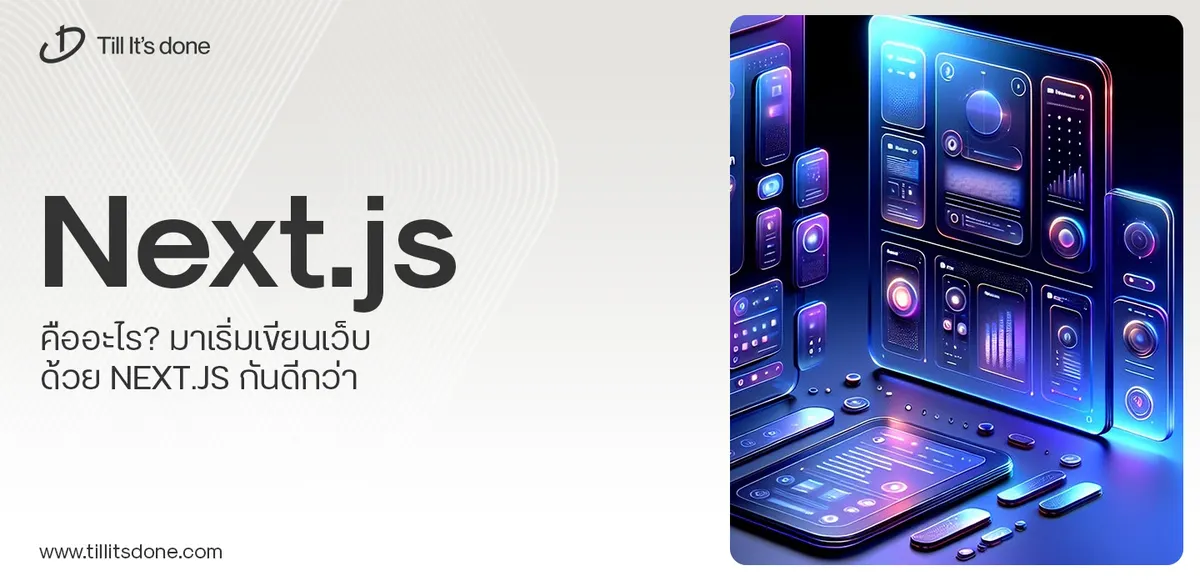
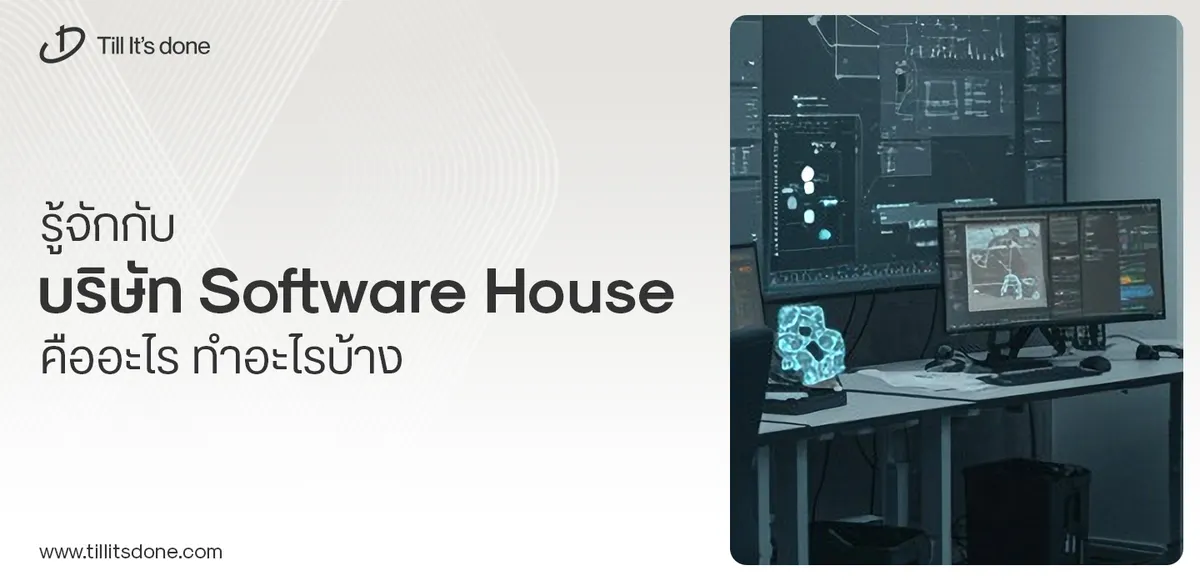
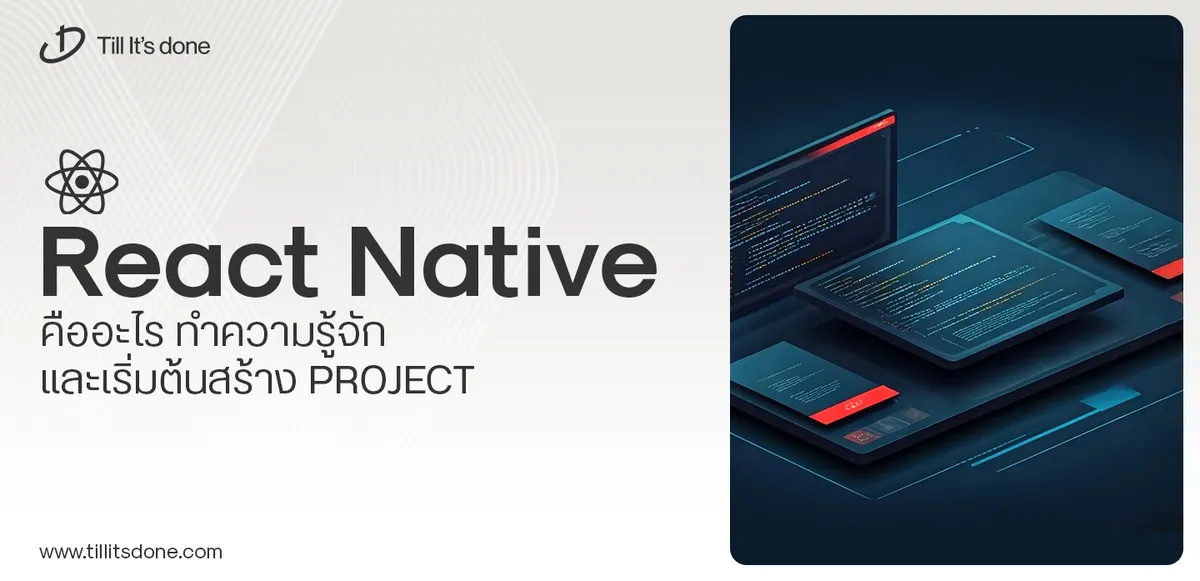
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.