- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to Zod in Node.js: Schema Validation
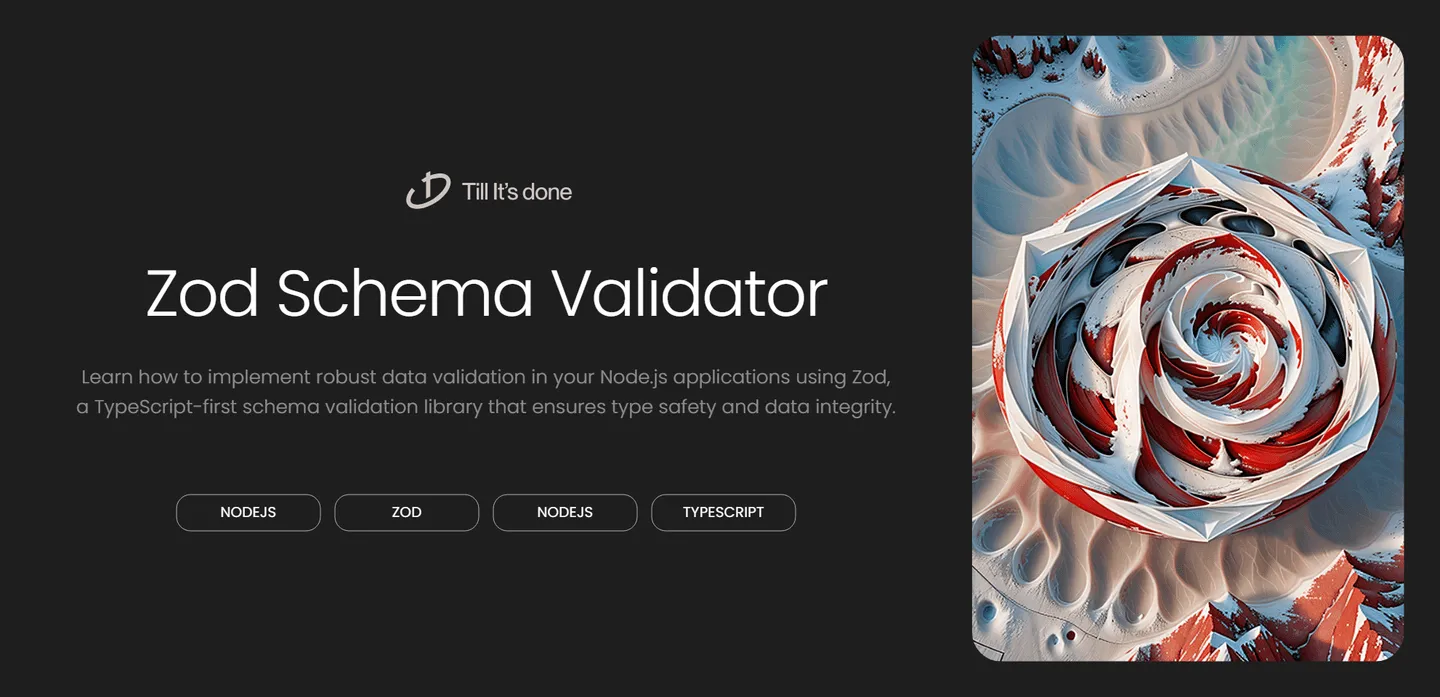
Ever found yourself wrestling with data validation in your Node.js applications? We’ve all been there – checking input types, handling edge cases, and ensuring data consistency. That’s where Zod steps in as a game-changer in the world of schema validation.
What is Zod?
Zod is a TypeScript-first schema declaration and validation library that lets you create complex type-safe validations with minimal effort. Think of it as your data’s bouncer – nothing gets through without meeting the exact specifications you set.
Getting Started with Zod
First things first, let’s get Zod into your project:
npm install zod
Here’s a simple example to get your feet wet:
import { z } from 'zod';
// Define a schema for a userconst userSchema = z.object({ username: z.string().min(3).max(20), email: z.string().email(), age: z.number().min(18).optional(),});
// Validate datatry { const userData = userSchema.parse({ username: "john_doe", email: "john@example.com", age: 25 }); console.log("Validation successful:", userData);} catch (error) { console.error("Validation failed:", error);}
Why Zod Stands Out?
Unlike traditional validation libraries, Zod brings several unique advantages to the table. The schema definitions are incredibly intuitive, and the TypeScript integration is seamless. You get type inference out of the box – meaning your IDE can provide autocomplete and type checking based on your schema definitions.
Real-World Applications
Zod really shines when handling complex data structures. Let’s look at a more advanced example:
const orderSchema = z.object({ orderId: z.string().uuid(), items: z.array(z.object({ productId: z.number(), quantity: z.number().positive(), price: z.number().positive() })), customer: z.object({ name: z.string(), address: z.string(), email: z.string().email() }), status: z.enum(['pending', 'processing', 'shipped', 'delivered'])});
Best Practices and Tips
- Always define your schemas outside request handlers for better reusability
- Use
.safeParse()
instead of.parse()
when you want to handle validation errors gracefully - Leverage Zod’s built-in error formatting for consistent error messages
- Combine schemas using
.extend()
for flexible schema composition
Remember, good validation isn’t just about preventing bugs – it’s about creating a robust foundation for your application’s data flow.
Conclusion
Zod is more than just a validation library – it’s a powerful tool that brings together type safety and runtime validation in an elegant package. By incorporating Zod into your Node.js applications, you’re not just validating data; you’re building a more reliable and maintainable codebase.
Whether you’re building a small API or a large-scale application, Zod’s flexibility and TypeScript-first approach make it an excellent choice for handling your validation needs. Start small, experiment with its features, and watch as it transforms how you think about data validation in your applications.
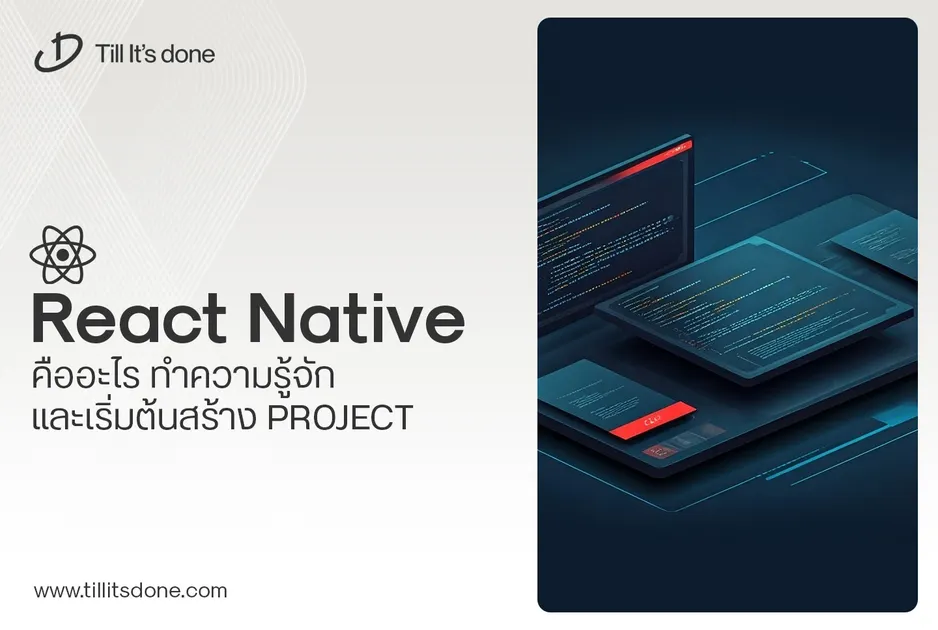
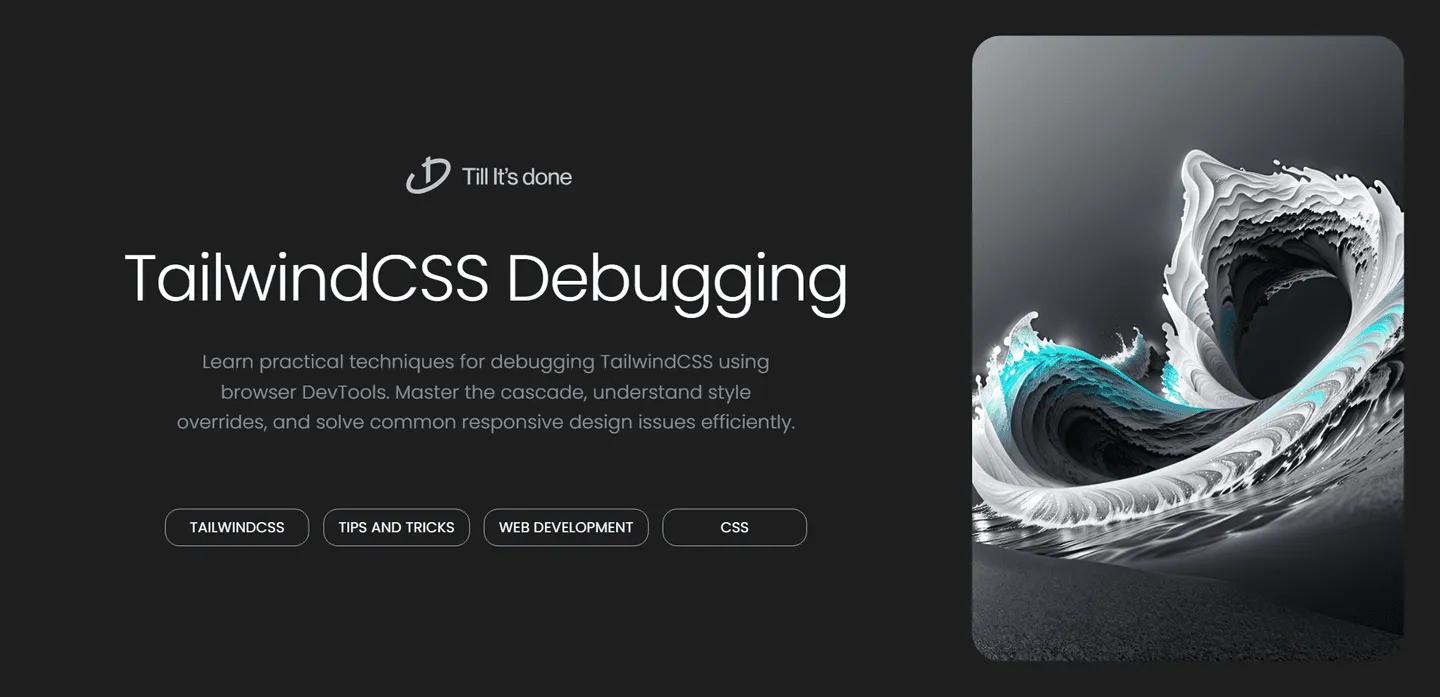
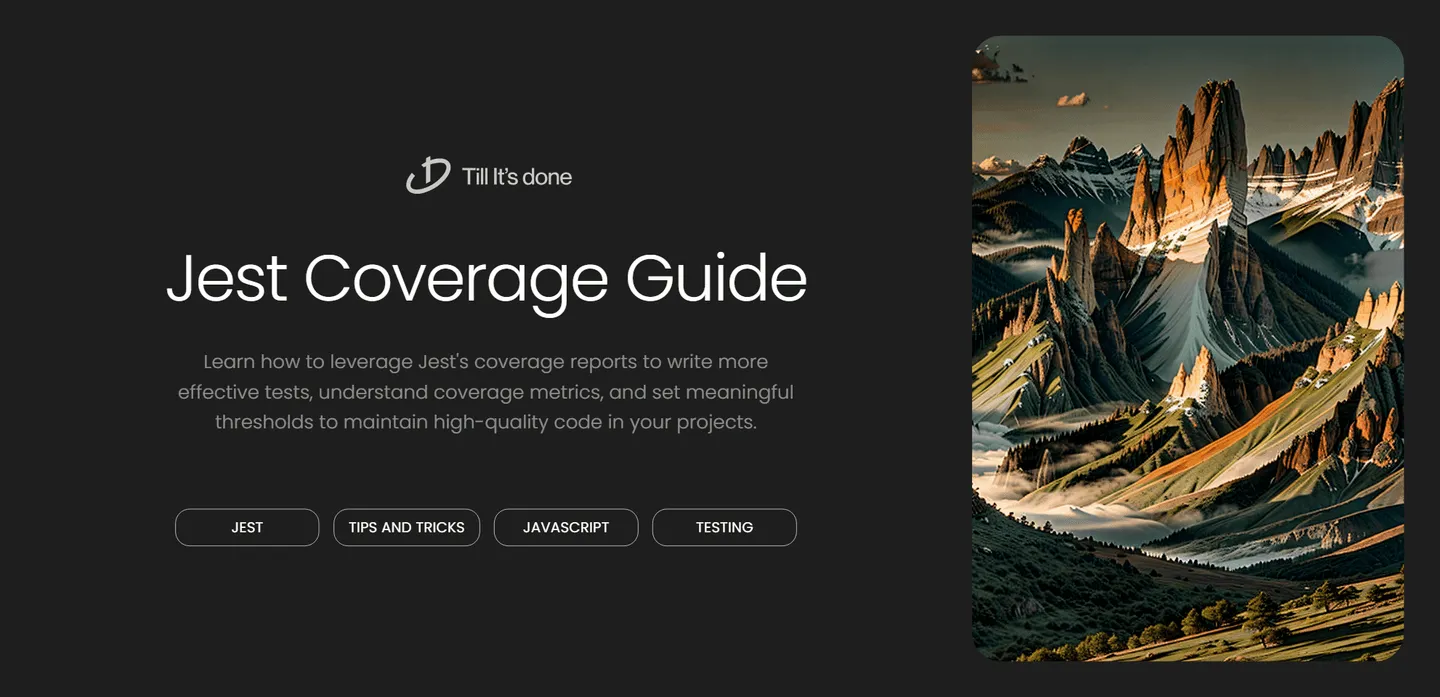
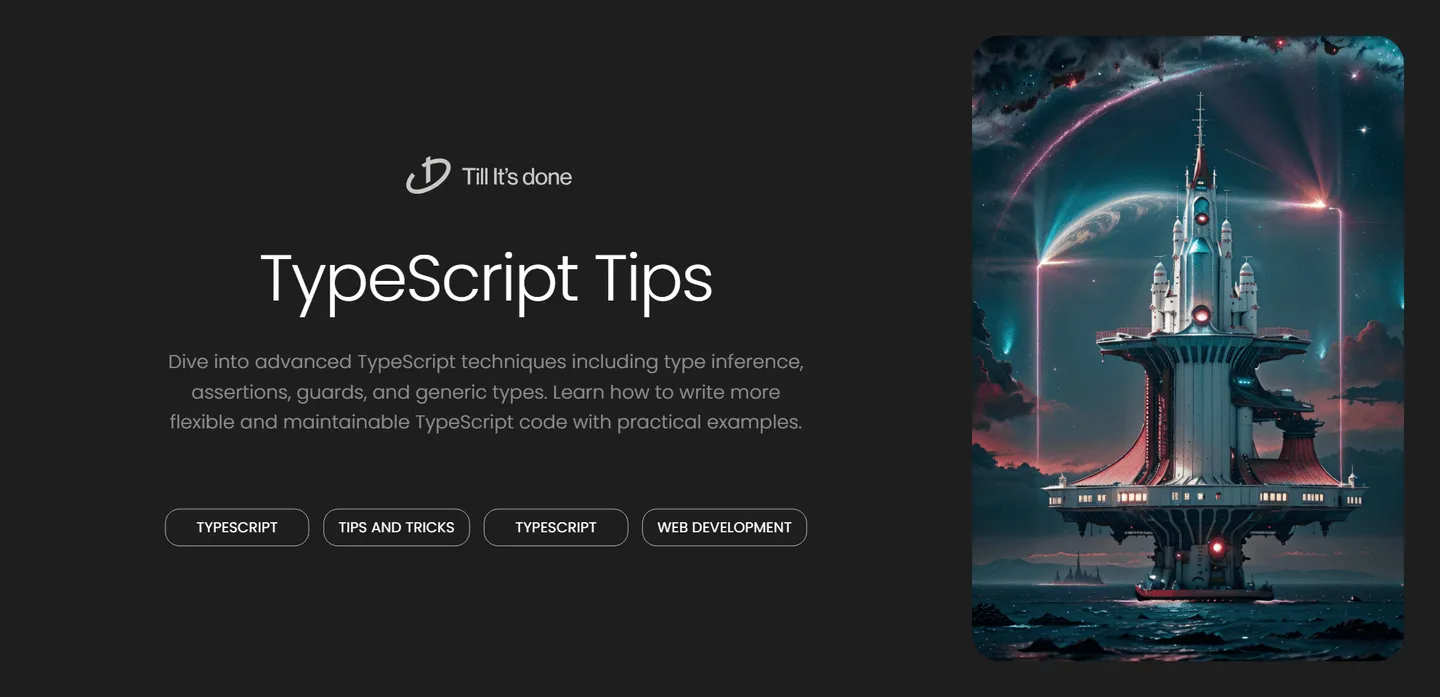
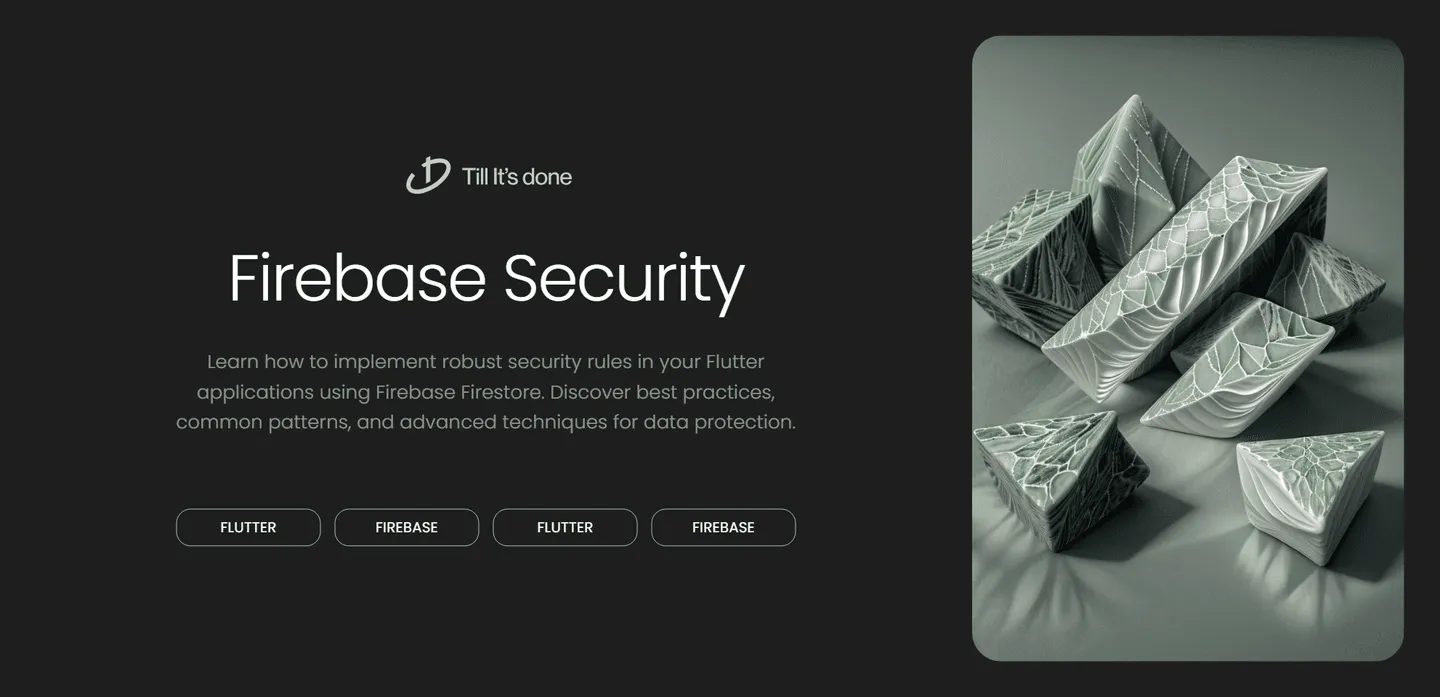
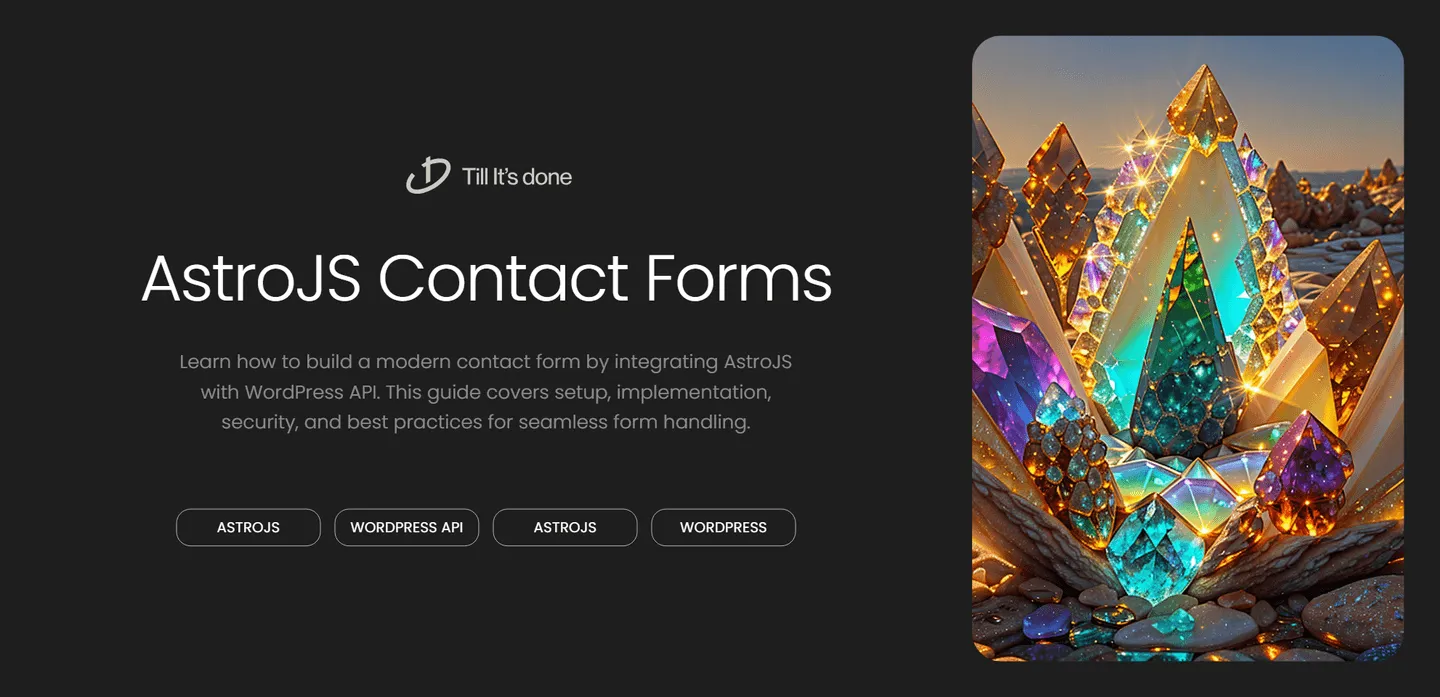
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.