- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Introduction to Zod for Form Validation in Astro.js
Discover best practices and tips for building type-safe forms.
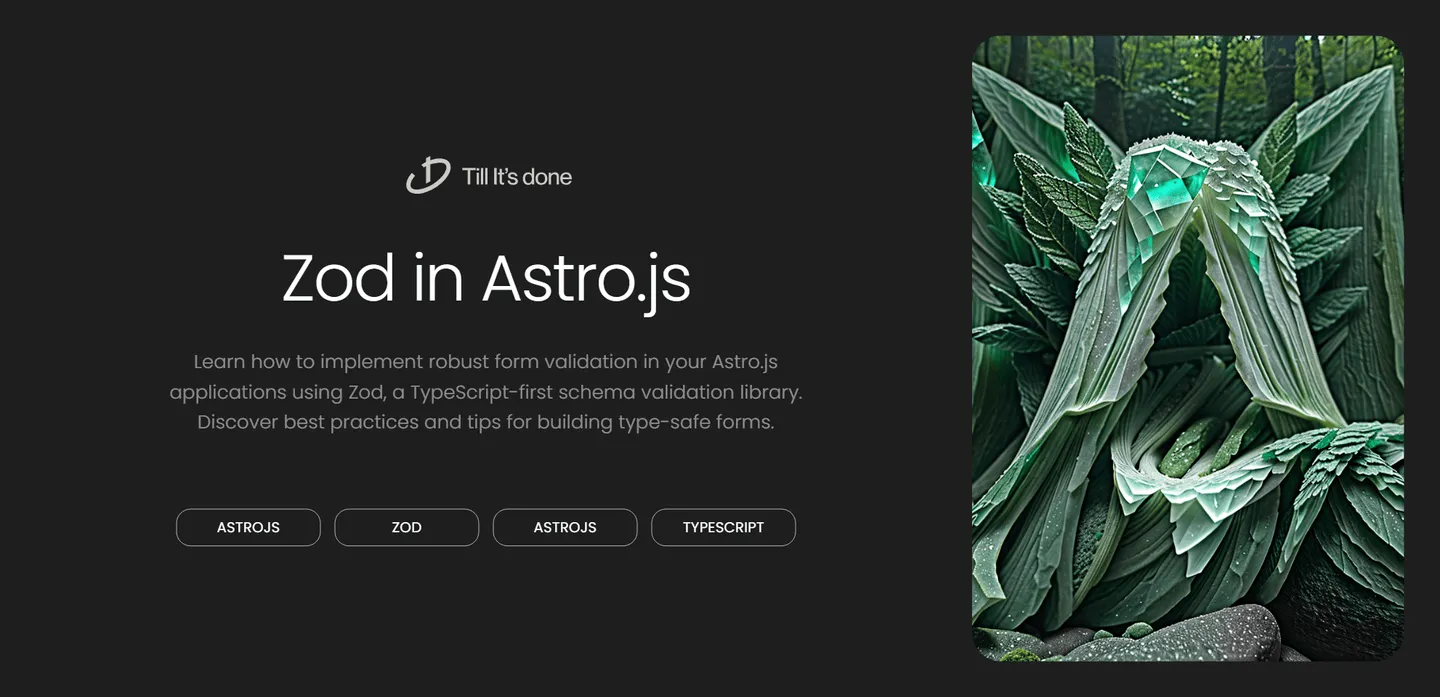
Form validation is a crucial aspect of web development that every developer needs to master. While Astro.js provides an excellent foundation for building static and dynamic websites, adding robust form validation can be challenging. Enter Zod – a TypeScript-first schema validation library that makes form validation both powerful and enjoyable.
Why Choose Zod for Astro.js?
When building forms in Astro.js, you need a validation solution that’s both type-safe and easy to implement. Zod fits perfectly into this niche, offering a declarative way to define your data schemas and validate them with minimal boilerplate.
Getting Started with Zod
First, let’s set up Zod in your Astro.js project. Run the following command in your terminal:
npm install zod
Let’s create a simple contact form schema:
import { z } from 'zod';
const contactSchema = z.object({ name: z.string().min(2, 'Name must be at least 2 characters'), email: z.string().email('Please enter a valid email'), message: z.string().min(10, 'Message must be at least 10 characters')});
Implementing Form Validation
Here’s how you can implement form validation in your Astro component:
---import { z } from 'zod';
let errors = {};let success = false;
if (Astro.request.method === 'POST') { try { const data = await Astro.request.formData(); const formData = { name: data.get('name'), email: data.get('email'), message: data.get('message') };
const validatedData = contactSchema.parse(formData); success = true; // Handle your form submission here
} catch (error) { if (error instanceof z.ZodError) { errors = error.flatten().fieldErrors; } }}---
Best Practices and Tips
-
Custom Error Messages: Make your validation messages user-friendly and specific. Zod allows you to customize error messages for each validation rule.
-
Type Inference: Take advantage of Zod’s TypeScript integration to automatically infer types from your schemas:
type ContactForm = z.infer<typeof contactSchema>;
- Complex Validations: Zod supports advanced validation scenarios, including:
- Conditional validation
- Custom validation functions
- Array and object validation
- Optional and nullable fields
Advanced Usage
You can extend your validation schemas to handle more complex scenarios:
const advancedContactSchema = z.object({ name: z.string().min(2).max(50), email: z.string().email(), subject: z.enum(['support', 'feedback', 'other']), message: z.string().min(10).max(1000), attachments: z.array(z.string()).optional(), newsletter: z.boolean().default(false)});
By implementing Zod validation in your Astro.js applications, you’re not just validating forms – you’re building a type-safe, maintainable, and user-friendly experience. The combination of Astro.js’s performance and Zod’s robust validation capabilities creates a powerful foundation for your web applications.
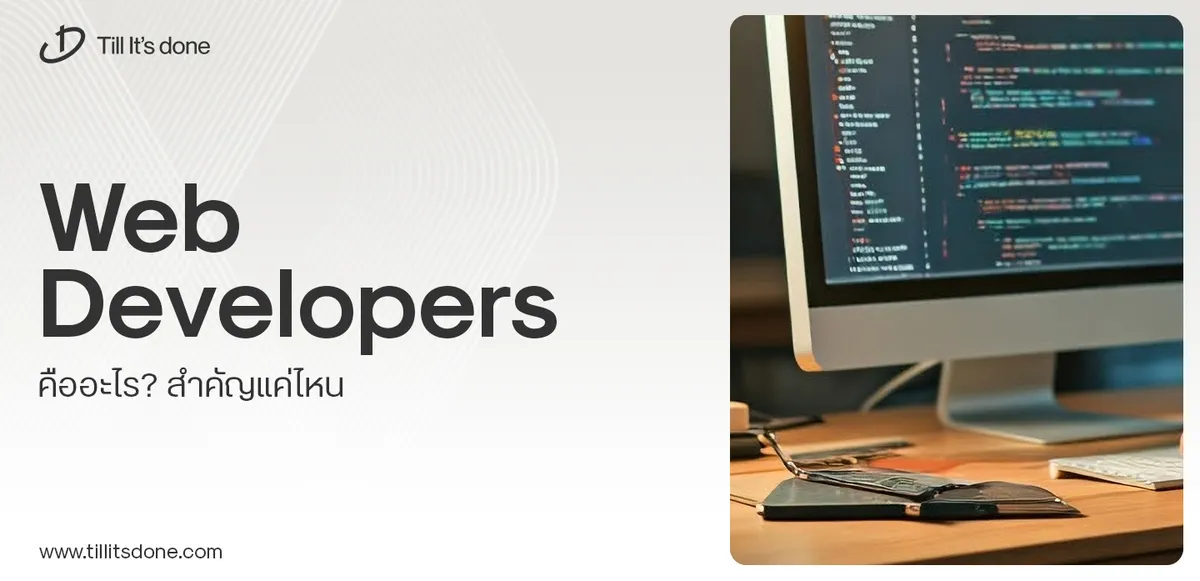
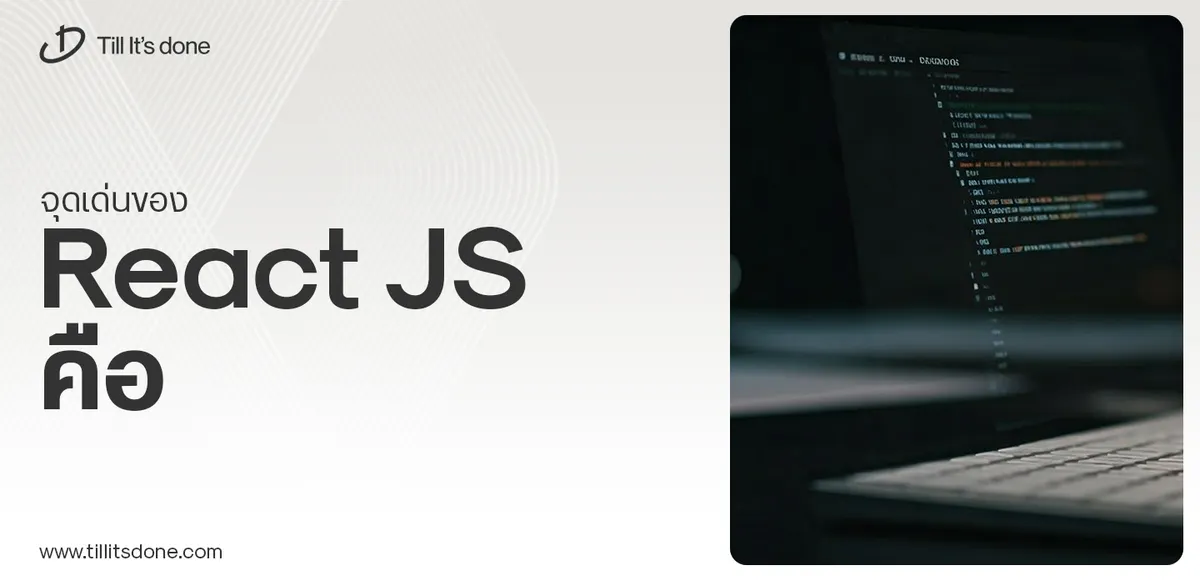
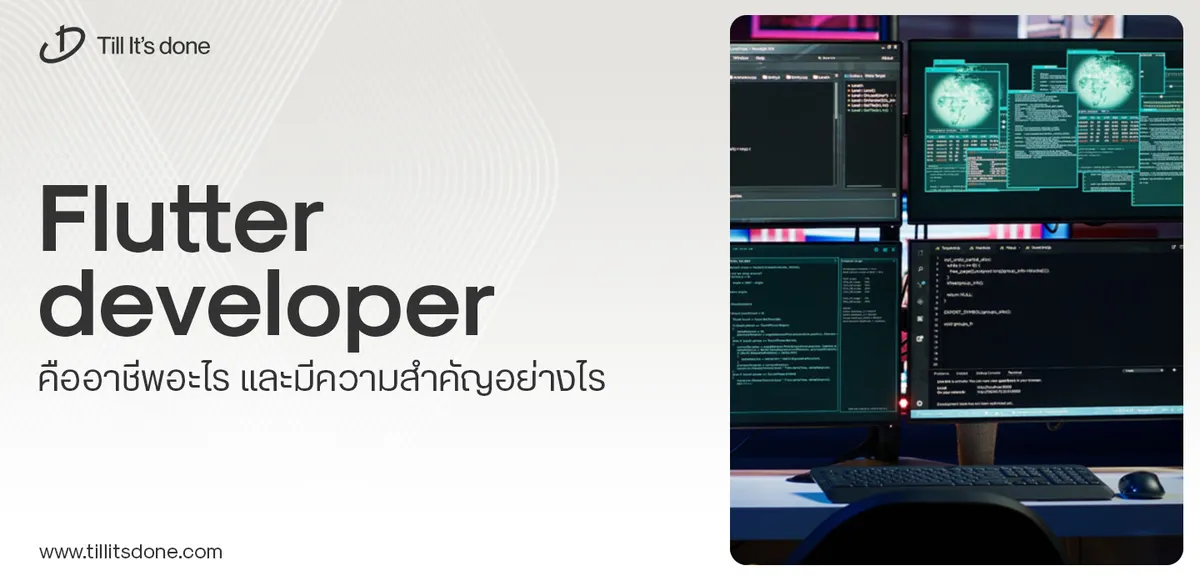
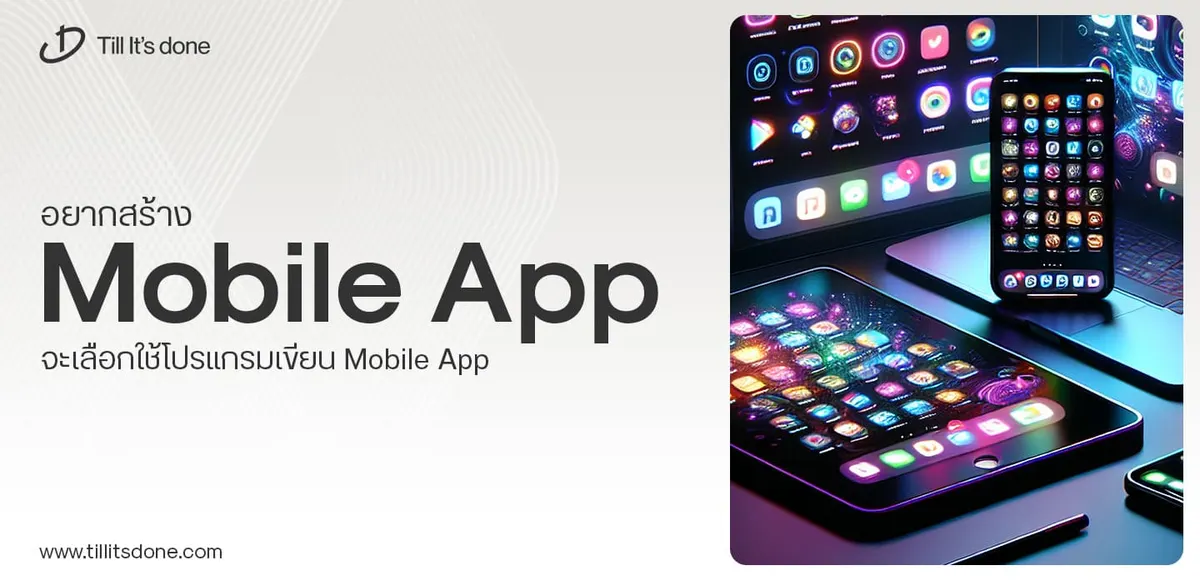
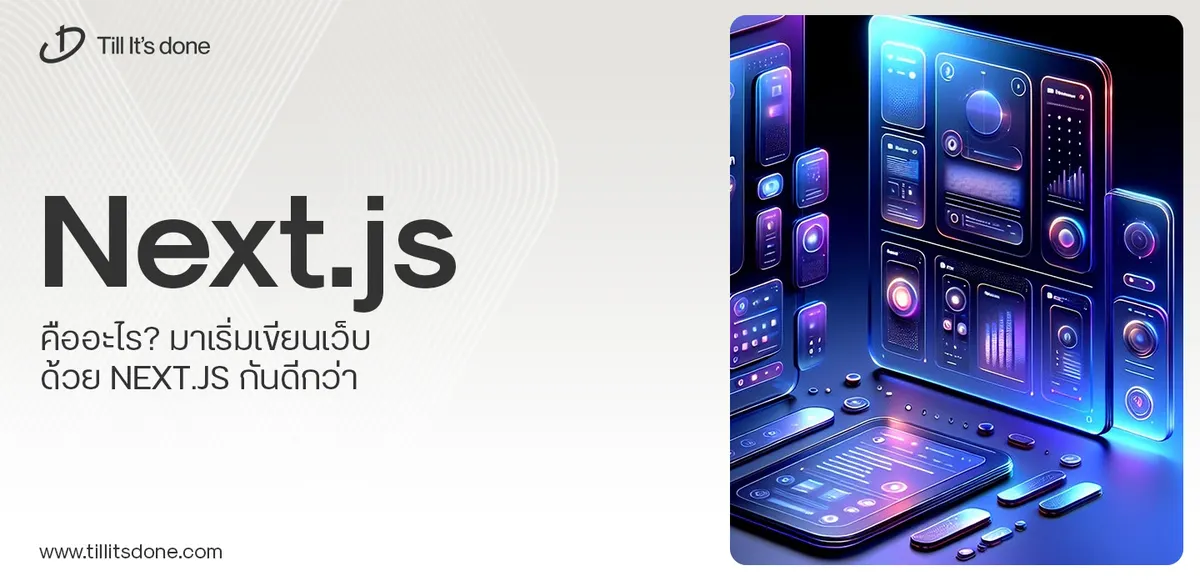
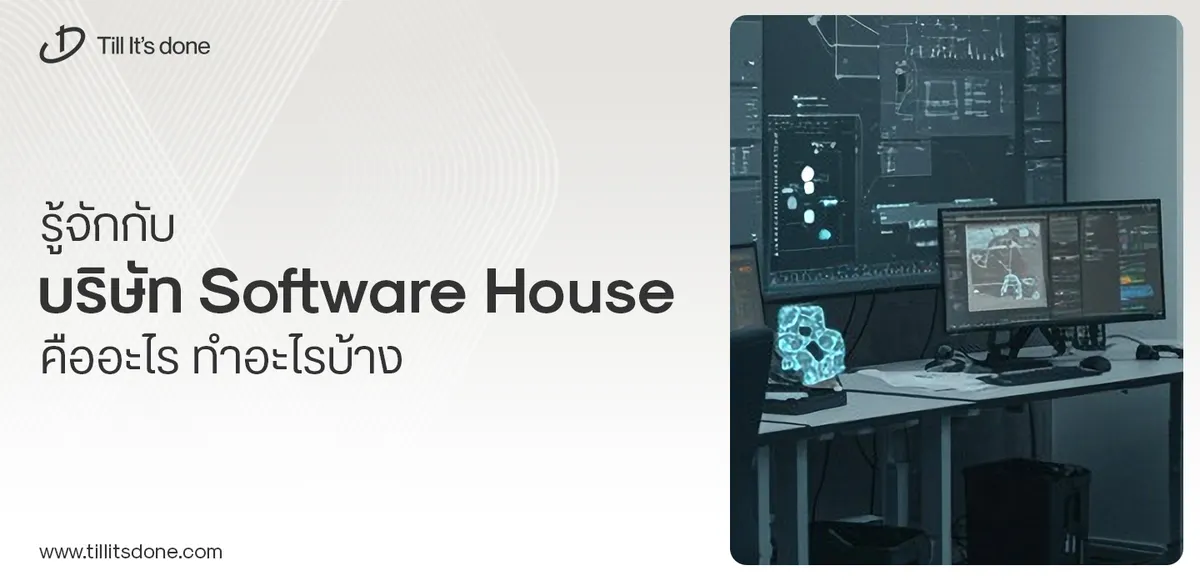
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.