- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Set Up WebSocket Connections in Golang
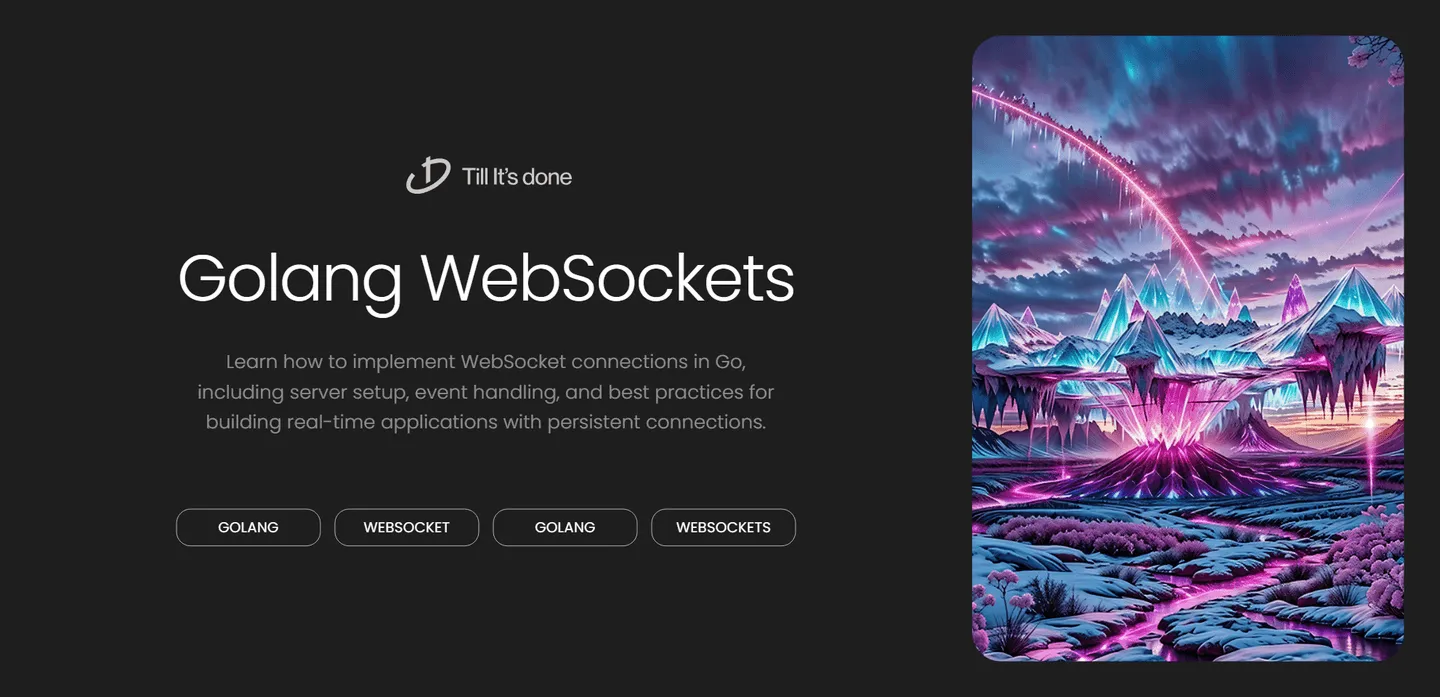
How to Set Up WebSocket Connections in Golang
WebSocket technology has revolutionized real-time web applications by enabling persistent, bidirectional communication between clients and servers. In this guide, we’ll explore how to implement WebSocket connections in Go, making it easy to build interactive applications like chat systems, live dashboards, and gaming platforms.
Understanding WebSocket Basics
Before diving into the implementation, it’s crucial to understand that WebSocket connections start as HTTP requests and then upgrade to maintain a persistent connection. This upgrade process allows for efficient, real-time data exchange without the overhead of establishing new connections repeatedly.
Setting Up a Basic WebSocket Server
Let’s start by creating a simple WebSocket server in Go. We’ll use the popular gorilla/websocket
package, which provides a robust implementation of the WebSocket protocol.
First, install the required package:
go get github.com/gorilla/websocket
Here’s a basic WebSocket server implementation:
package main
import ( "log" "net/http" "github.com/gorilla/websocket")
var upgrader = websocket.Upgrader{ ReadBufferSize: 1024, WriteBufferSize: 1024, CheckOrigin: func(r *http.Request) bool { return true // Be careful with this in production },}
func handleWebSocket(w http.ResponseWriter, r *http.Request) { conn, err := upgrader.Upgrade(w, r, nil) if err != nil { log.Println(err) return } defer conn.Close()
for { messageType, message, err := conn.ReadMessage() if err != nil { log.Println("Error during message reading:", err) break }
// Echo the message back to the client err = conn.WriteMessage(messageType, message) if err != nil { log.Println("Error during message writing:", err) break } }}
func main() { http.HandleFunc("/ws", handleWebSocket) log.Fatal(http.ListenAndServe(":8080", nil))}
Handling WebSocket Events
When working with WebSocket connections, you’ll need to handle various events and manage connection states. Here’s how to implement more advanced functionality:
type Client struct { conn *websocket.Conn send chan []byte}
func (c *Client) handleMessages() { defer func() { c.conn.Close() }()
for { _, message, err := c.conn.ReadMessage() if err != nil { if websocket.IsUnexpectedCloseError(err, websocket.CloseGoingAway, websocket.CloseAbnormalClosure) { log.Printf("error: %v", err) } break } // Process the message c.send <- message }}
Best Practices and Error Handling
- Always implement proper error handling
- Set appropriate timeouts and buffer sizes
- Implement heartbeat mechanisms
- Handle connection closure gracefully
- Consider implementing reconnection logic on the client side
Production Considerations
When deploying your WebSocket application to production, consider these important factors:
- Load balancing and scaling
- Connection persistence
- Security measures including origin checking
- Message size limits
- Rate limiting
- Connection pooling
- Monitoring and logging
Remember to implement proper authentication and authorization mechanisms to secure your WebSocket endpoints. You might want to pass authentication tokens during the initial handshake or use secure WebSocket connections (WSS) in production.
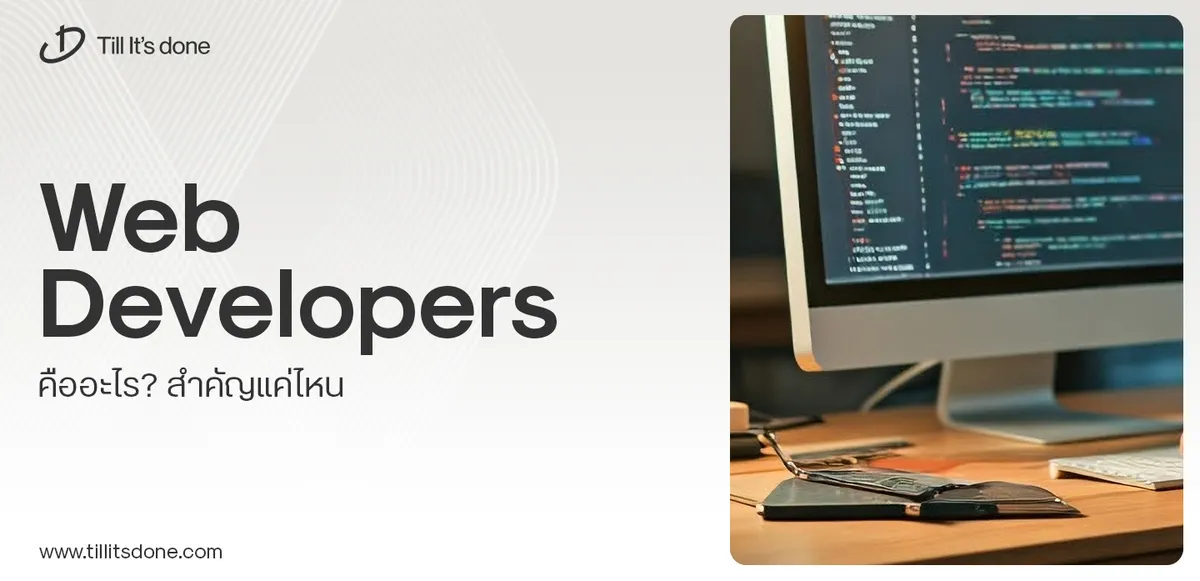
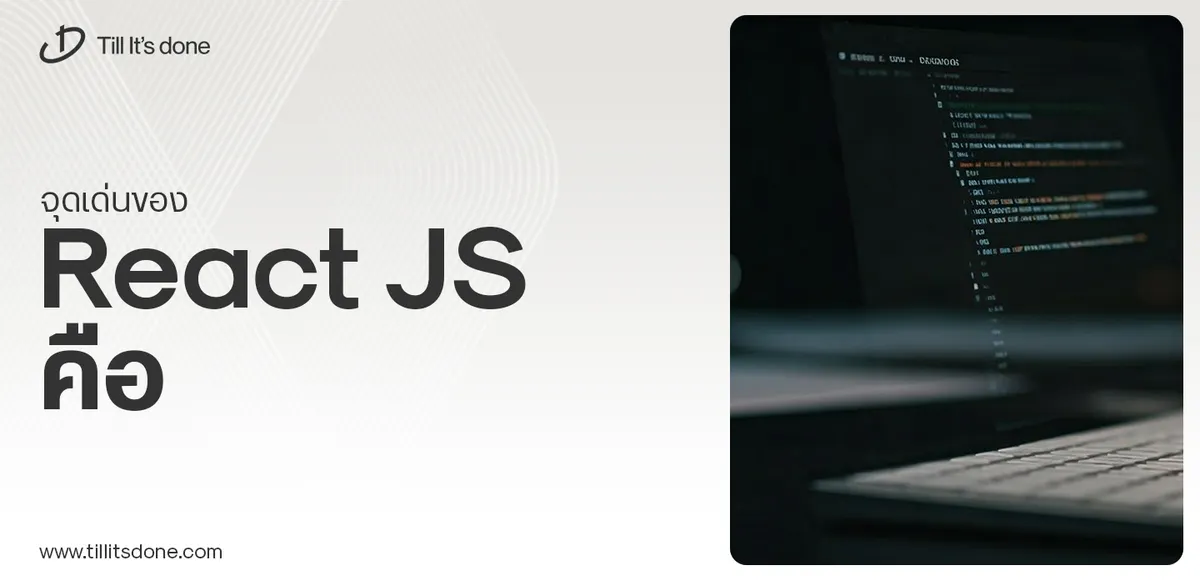
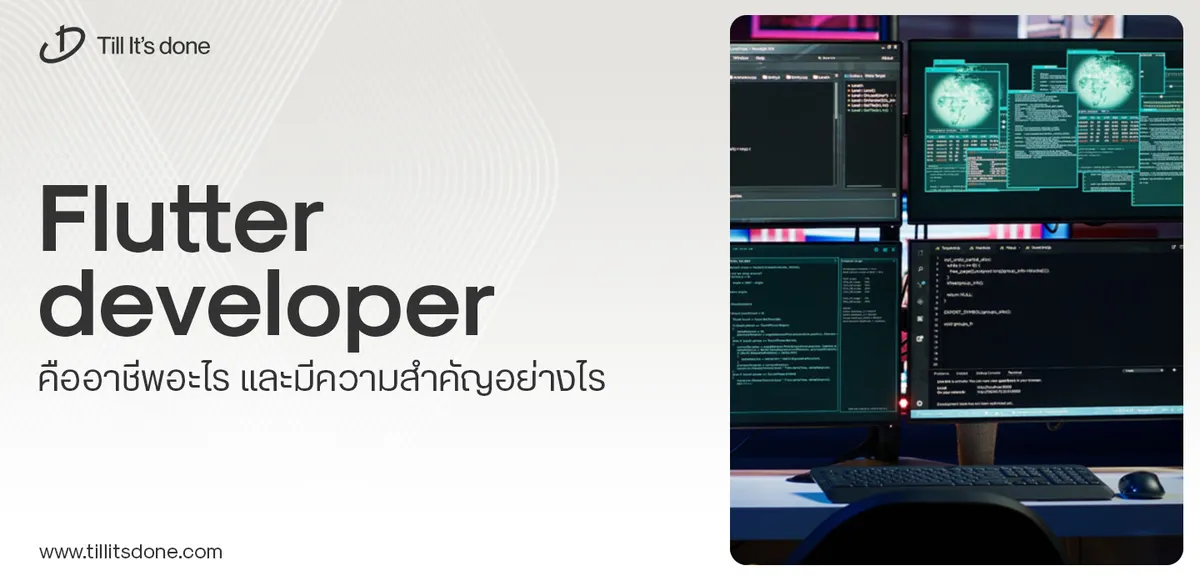
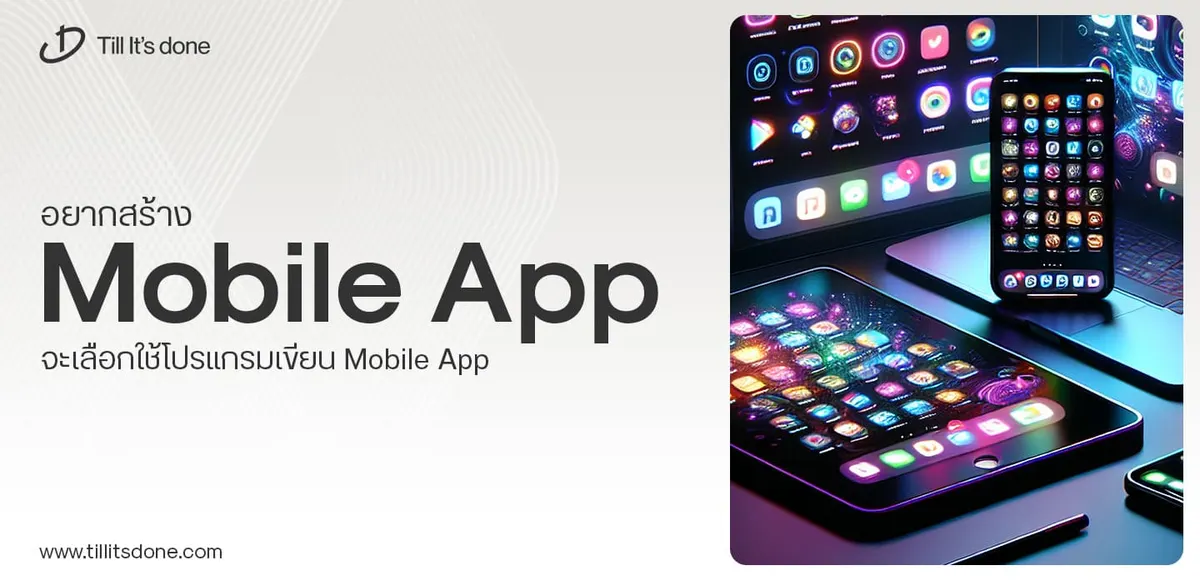
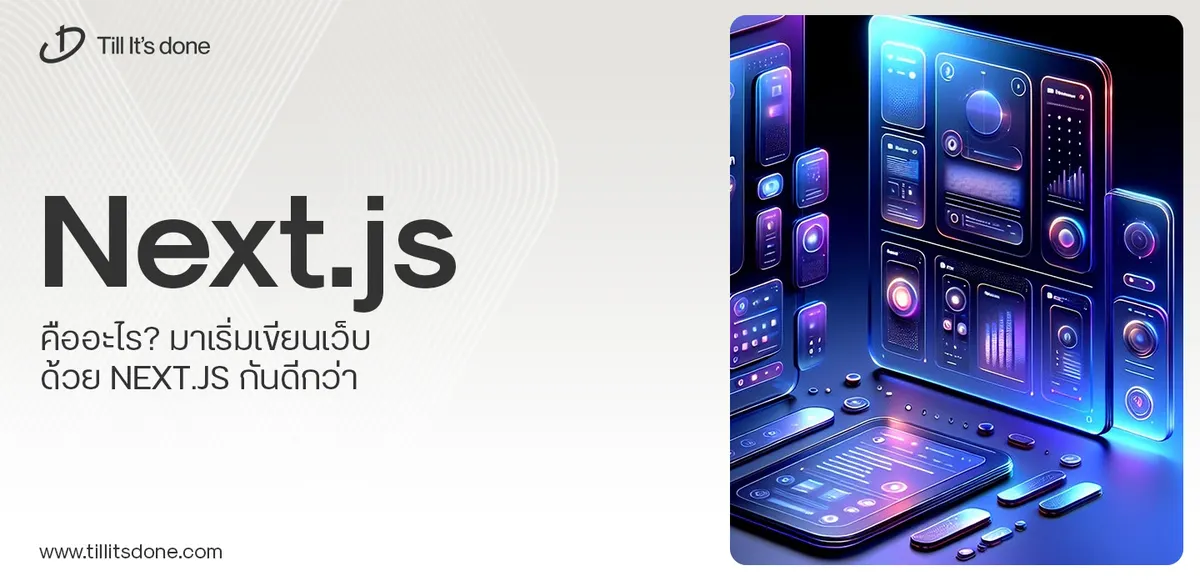
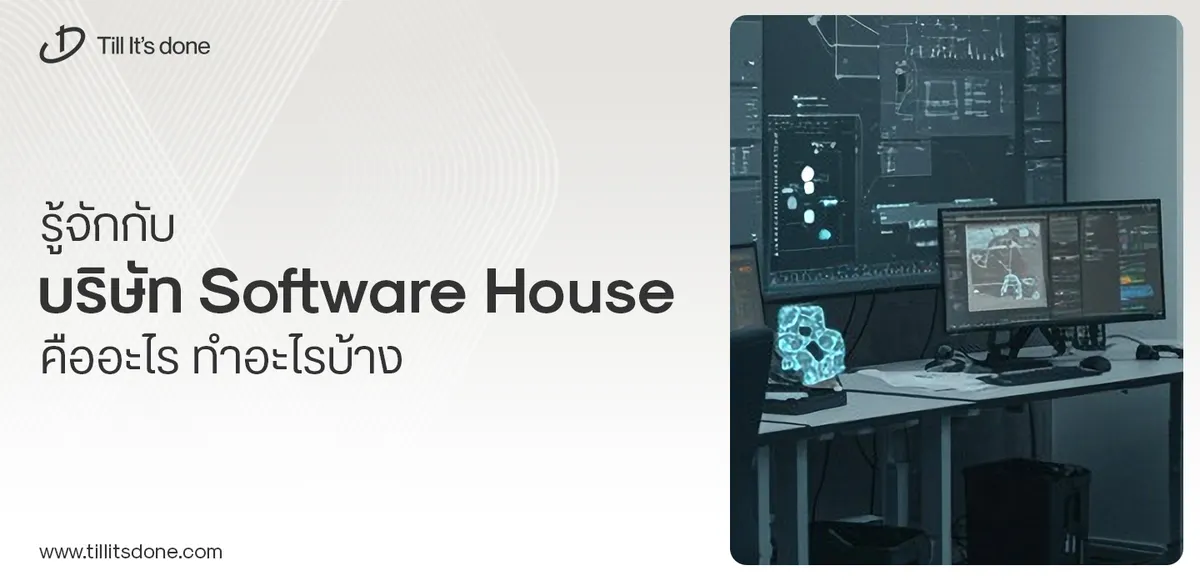
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.