- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Build a Web Crawler with Node.js & Cheerio
This step-by-step guide shows you how to extract data from websites efficiently and handle web scraping like a pro.
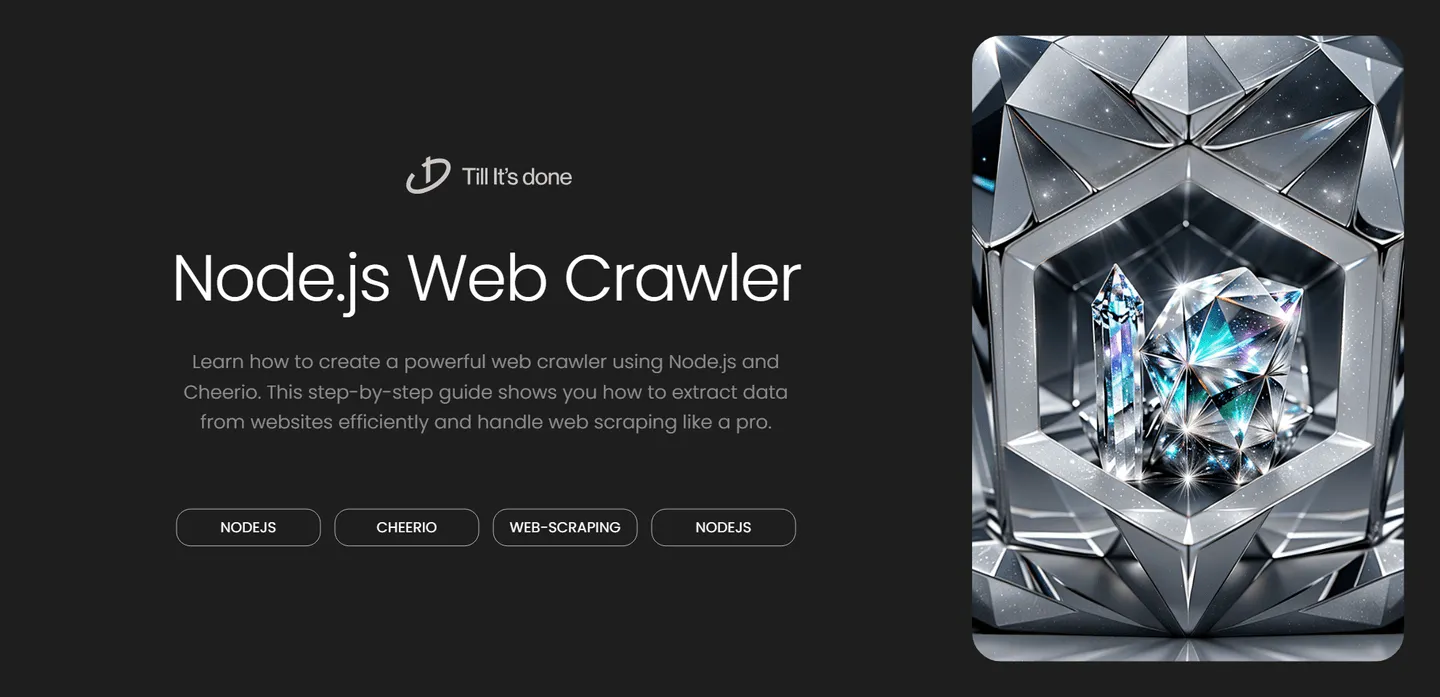
Building a Simple Web Crawler with Node.js and Cheerio
Web crawling is like being a digital explorer, systematically navigating through websites to gather information. Today, we’ll embark on an exciting journey to build our own web crawler using Node.js and Cheerio, a powerful combination that makes web scraping a breeze.
Understanding the Basics
Before we dive in, let’s understand what makes web crawling possible. Think of Cheerio as your digital Swiss Army knife – it lets you parse HTML just like jQuery, but on the server side. It’s lightweight, blazing fast, and incredibly flexible.
Setting Up Our Project
First things first, we need to set up our project. Create a new directory and initialize it with npm. We’ll need two essential packages: cheerio
for HTML parsing and axios
for making HTTP requests.
mkdir web-crawlercd web-crawlernpm init -ynpm install cheerio axios
Creating Our First Crawler
Let’s create a simple crawler that visits a website and extracts all the links from it. Here’s how we can do it:
const cheerio = require('cheerio');const axios = require('axios');
async function crawl(url) { try { // Fetch the HTML content const response = await axios.get(url); const html = response.data;
// Load the HTML into cheerio const $ = cheerio.load(html);
// Extract all links const links = []; $('a').each((i, link) => { links.push($(link).attr('href')); });
return links; } catch (error) { console.error('Error:', error.message); return []; }}
Making it More Powerful
Now that we have our basic crawler, let’s enhance it to gather more information. We can modify our code to extract specific data like titles, descriptions, or any other HTML elements we’re interested in:
async function enhancedCrawl(url) { try { const response = await axios.get(url); const $ = cheerio.load(response.data);
return { title: $('title').text(), links: $('a').map((_, link) => $(link).attr('href')).get(), headings: $('h1, h2').map((_, h) => $(h).text()).get() }; } catch (error) { console.error('Error:', error.message); return null; }}
Best Practices and Considerations
When building your crawler, remember to:
- Respect robots.txt files
- Add delays between requests to avoid overwhelming servers
- Handle errors gracefully
- Store your data efficiently
- Keep track of visited URLs to avoid infinite loops
Conclusion
Web crawling opens up a world of possibilities for data collection and analysis. With Node.js and Cheerio, you have powerful tools at your disposal to explore the web programmatically. Start small, experiment, and gradually build more complex crawlers as you become comfortable with the basics.
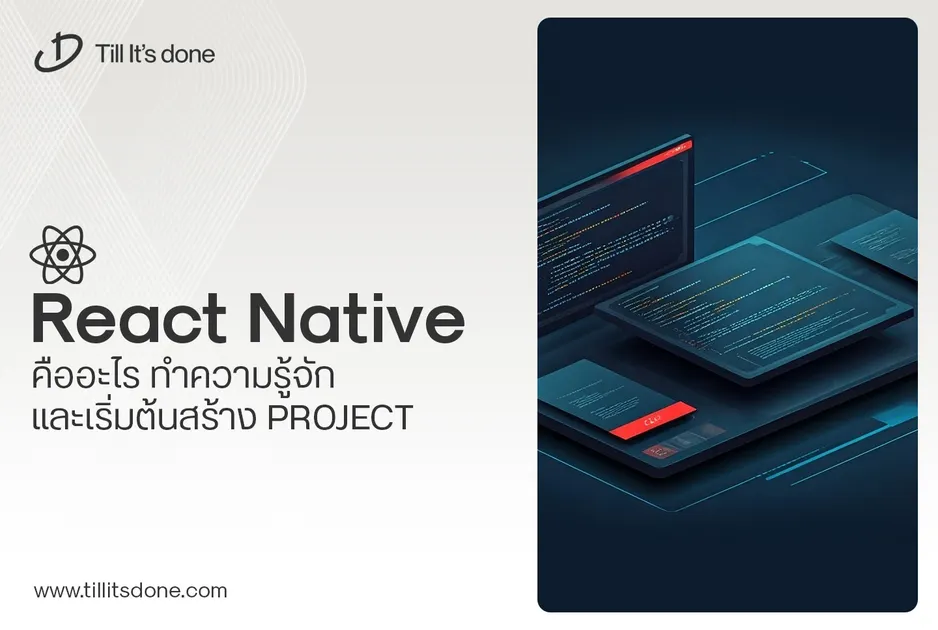
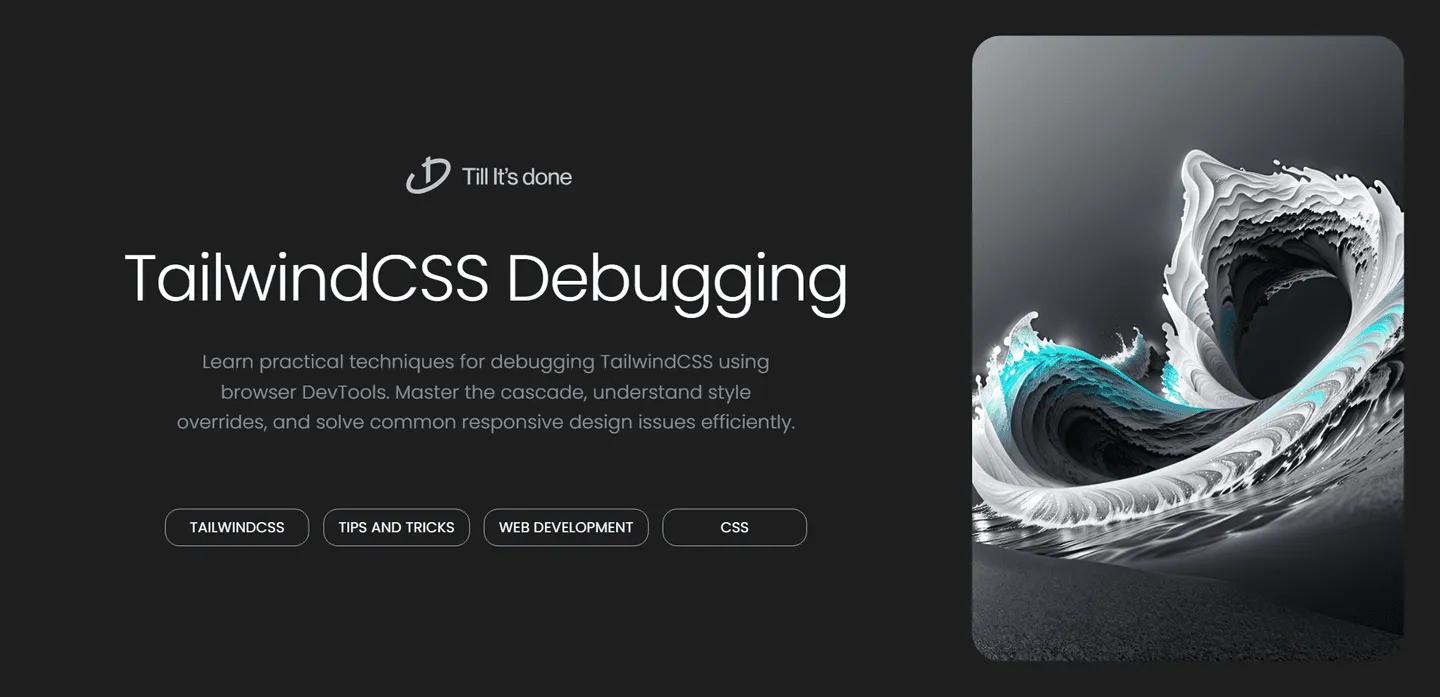
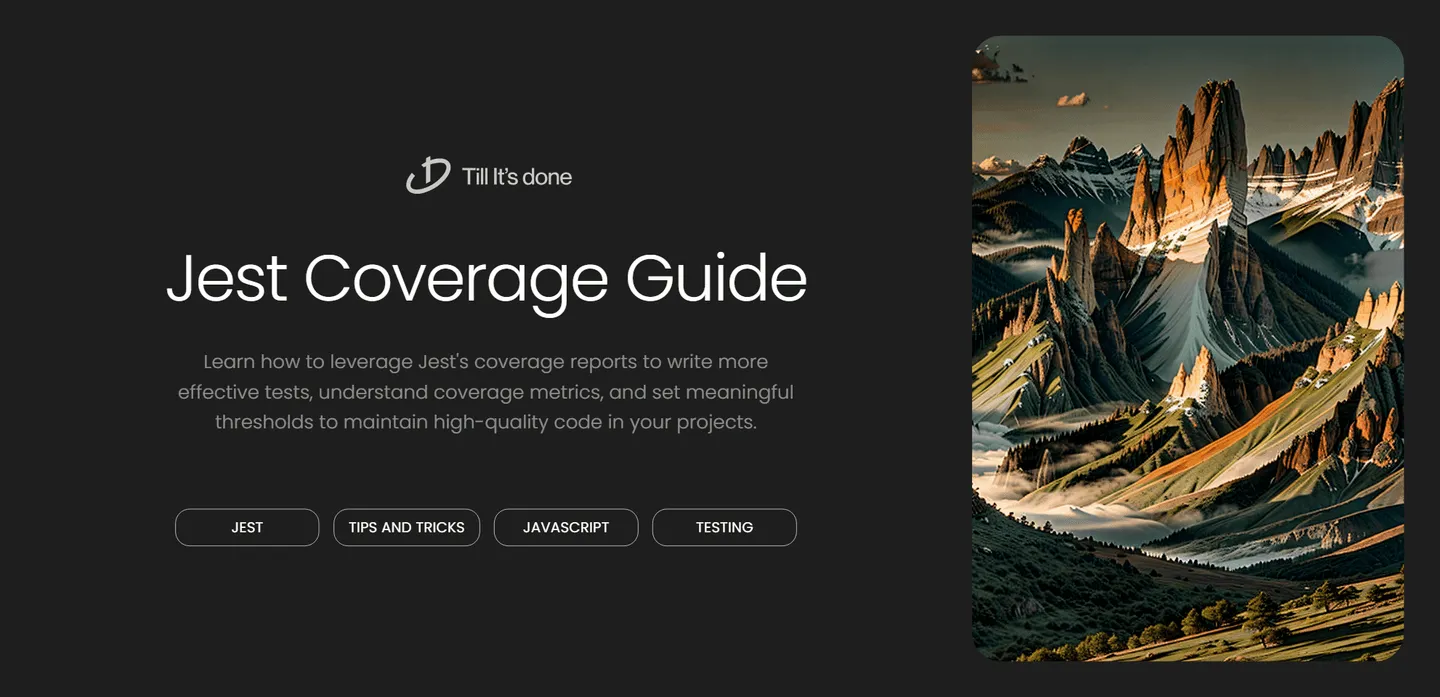
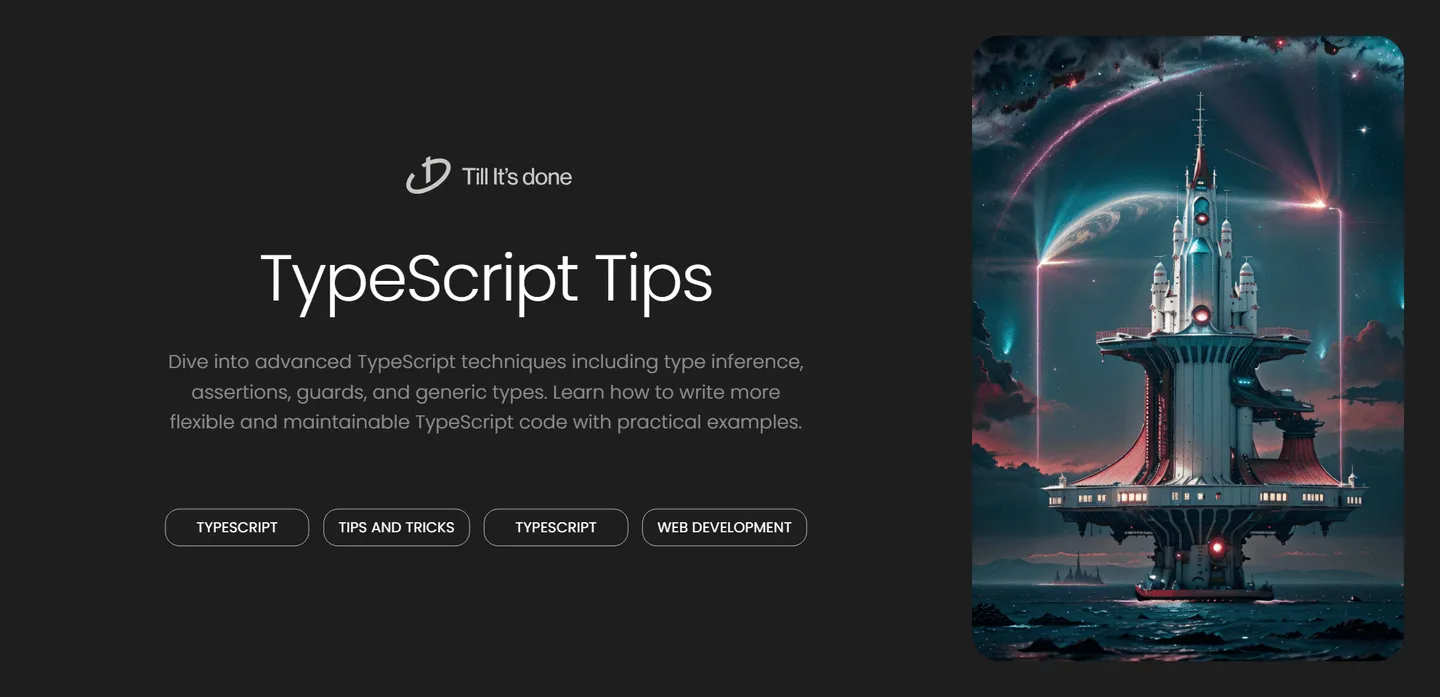
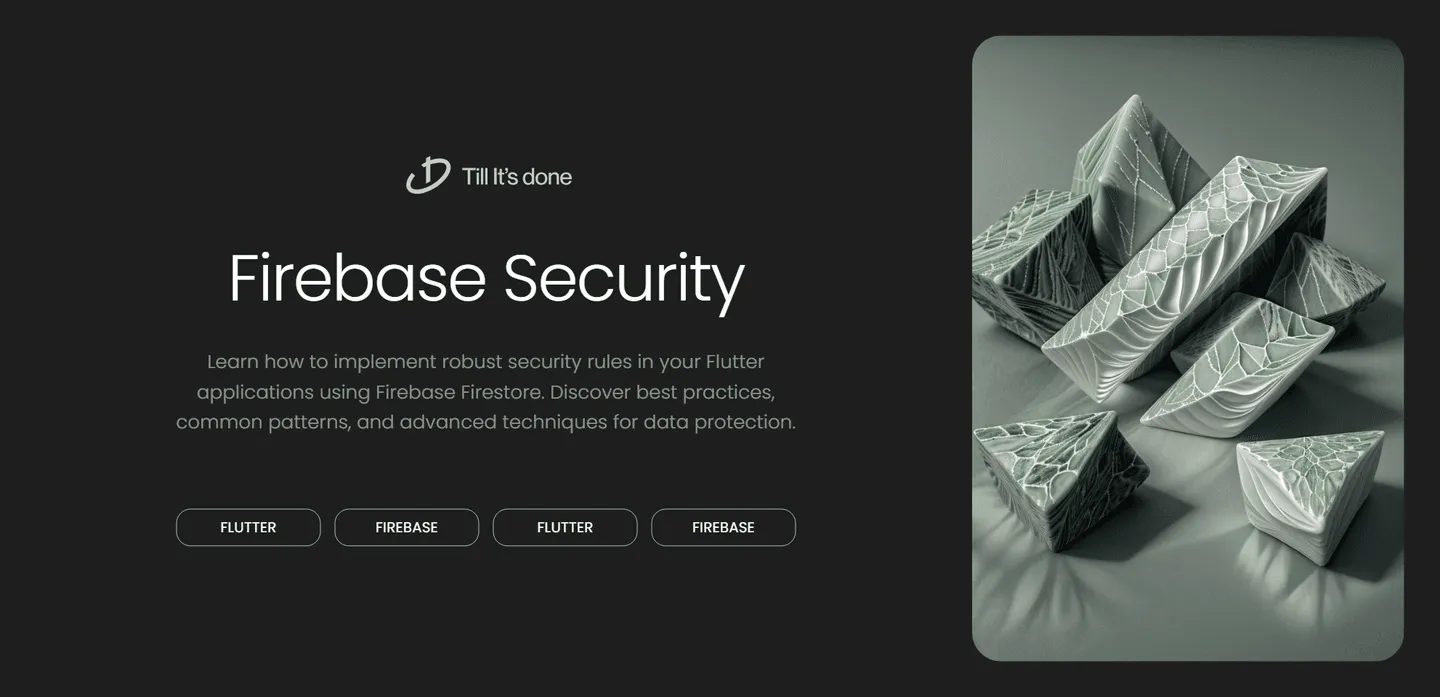
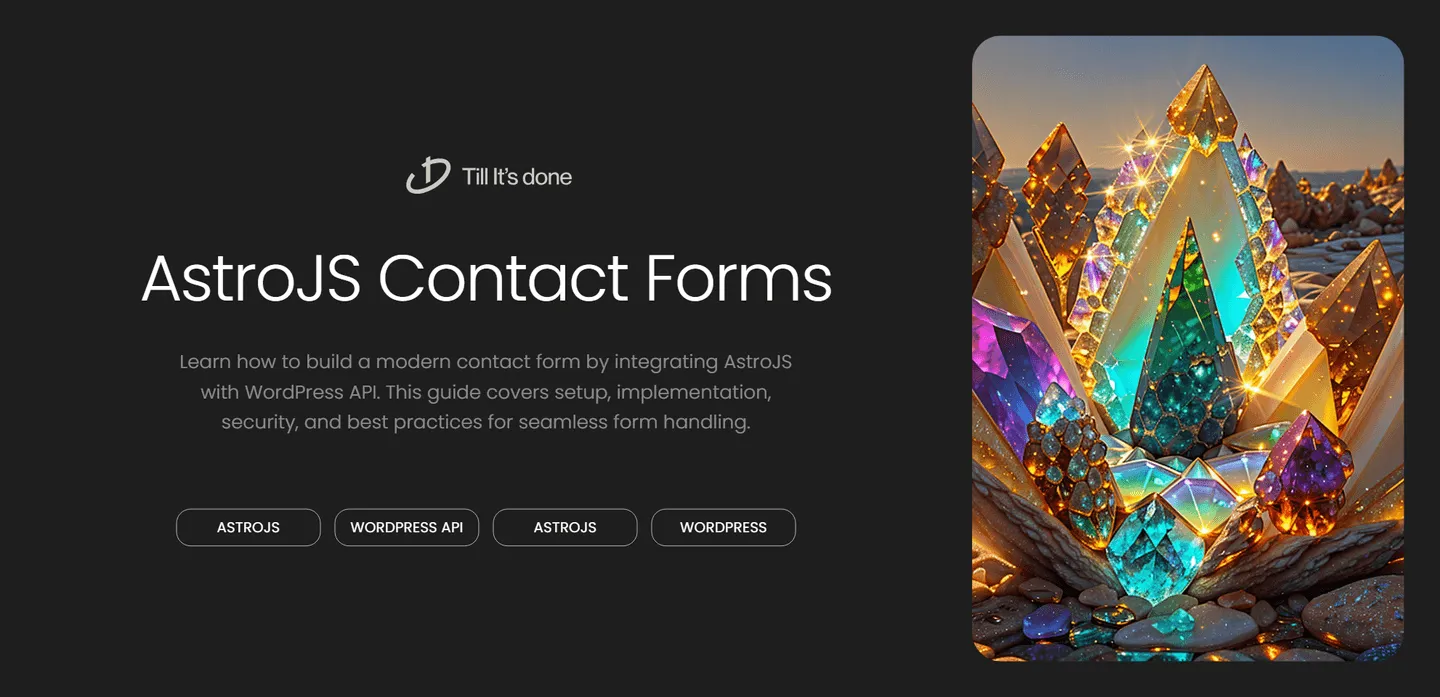
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.