- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Dynamic Configuration Reloading with Viper in Go
Update your app's settings in real-time without restarts, with practical examples and best practices.
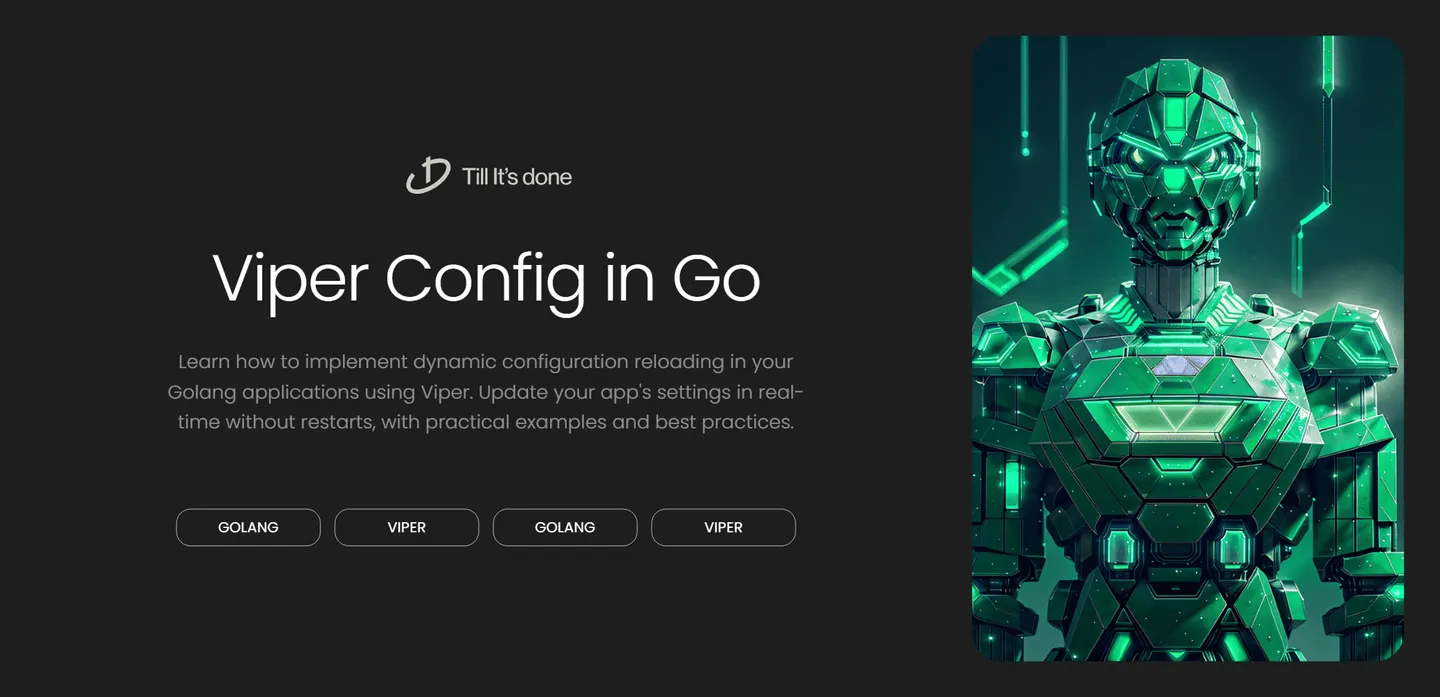
Dynamic Configuration Reloading with Viper in Golang
Have you ever needed to update your Go application’s configuration without restarting it? That’s where Viper’s dynamic configuration reloading comes into play. In this post, we’ll explore how to implement this powerful feature in your Golang applications.
What is Viper?
Viper is like a Swiss Army knife for configuration in Go. It’s a complete configuration solution that supports various formats (JSON, YAML, TOML, etc.), environment variables, and remote configuration systems. But one of its most impressive features is the ability to reload configurations on the fly.
Setting Up Dynamic Configuration
Let’s dive into how we can set up dynamic configuration reloading. The process is surprisingly straightforward, but incredibly powerful. Here’s what you need to know:
- First, we need to set up Viper to watch our configuration file:
viper.SetConfigFile("config.yaml")viper.WatchConfig()
- Then, we can add a callback function that executes whenever the configuration changes:
viper.OnConfigChange(func(e fsnotify.Event) { fmt.Printf("Config file changed: %s \n", e.Name) // Your configuration reload logic here})
Real-World Example
Let’s say we’re building a web server that needs to be able to update its rate limiting rules without downtime. Here’s how we might implement that:
type Config struct { RateLimit int Timeout time.Duration}
var config Config
func loadConfig() { viper.SetConfigName("config") viper.SetConfigType("yaml") viper.AddConfigPath(".")
if err := viper.ReadInConfig(); err != nil { log.Fatalf("Error reading config file: %s", err) }
viper.WatchConfig() viper.OnConfigChange(func(e fsnotify.Event) { fmt.Println("Config file changed, reloading...") var newConfig Config if err := viper.Unmarshal(&newConfig); err != nil { log.Printf("Error unmarshaling config: %s", err) return } config = newConfig })}
Best Practices
When implementing dynamic configuration reloading, keep these tips in mind:
- Always validate your new configuration before applying it
- Consider using atomic operations when updating configuration values
- Implement proper error handling for configuration changes
- Log configuration changes for debugging purposes
- Use mutex locks when accessing shared configuration values
Conclusion
Dynamic configuration reloading with Viper gives your Go applications the flexibility to adapt to changes without interruption. It’s a powerful feature that, when implemented correctly, can make your applications more maintainable and user-friendly.
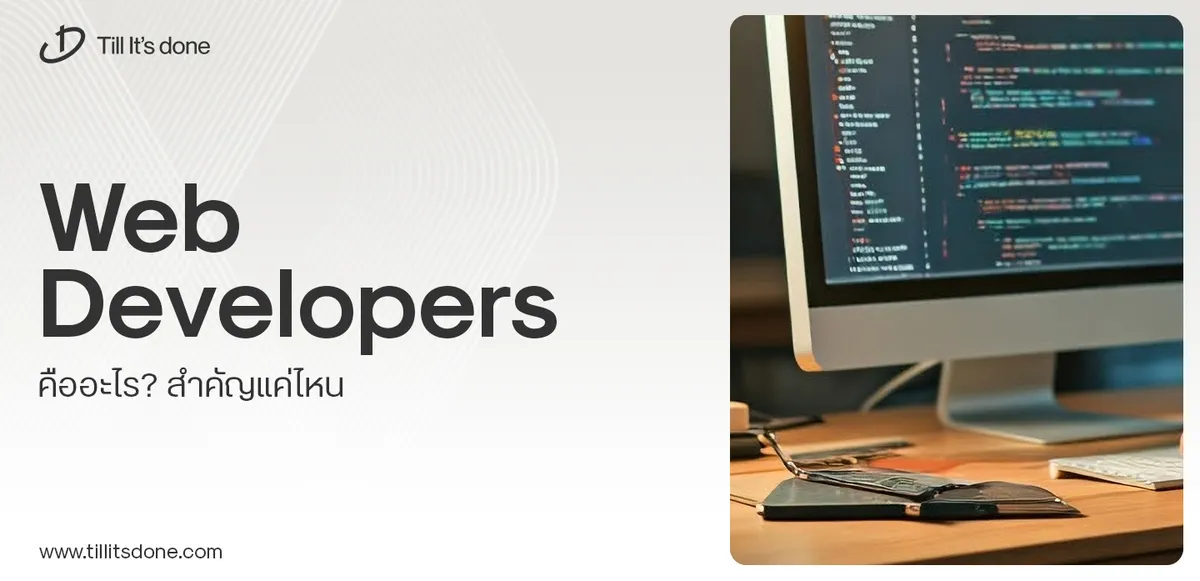
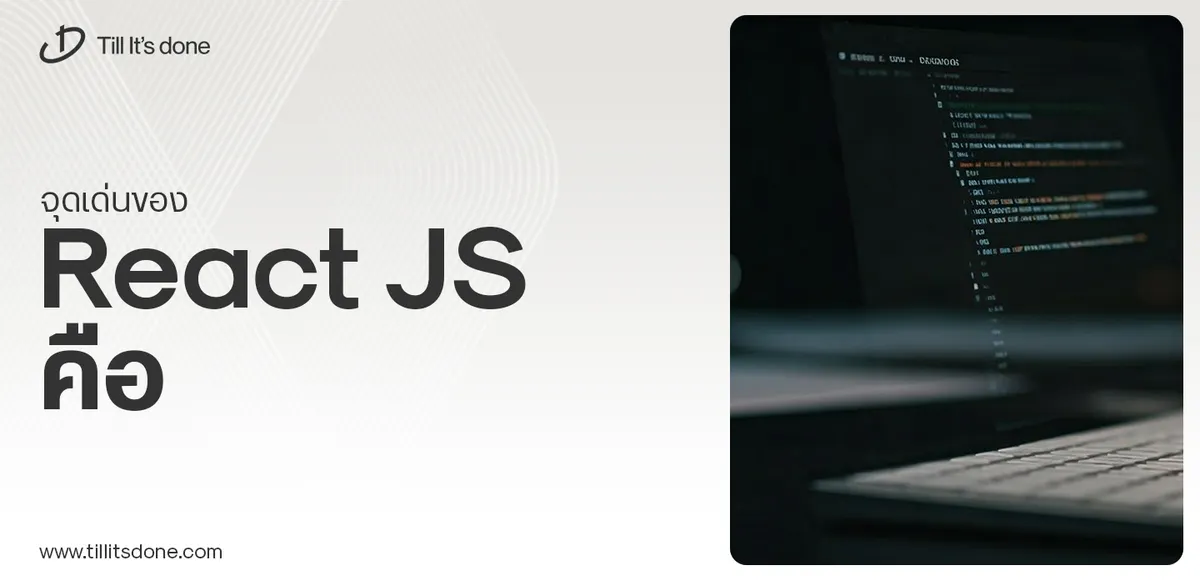
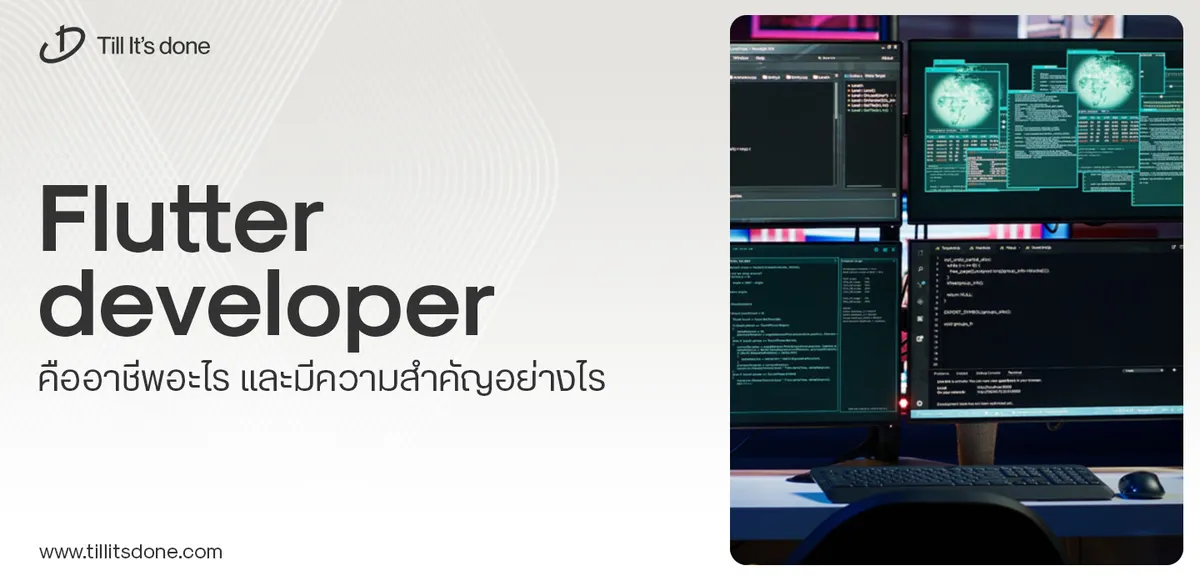
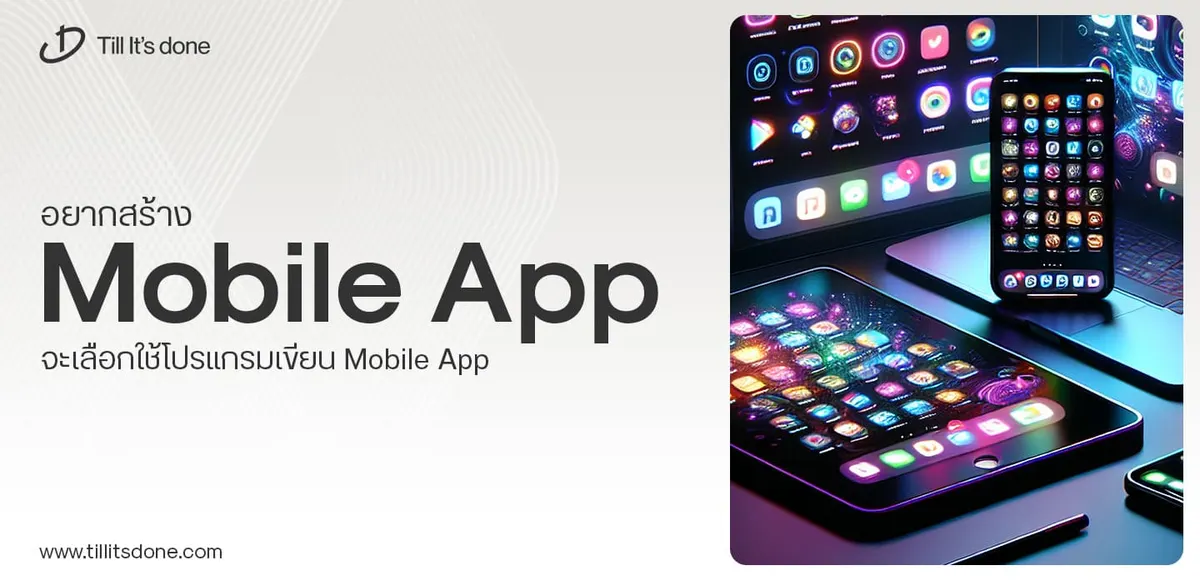
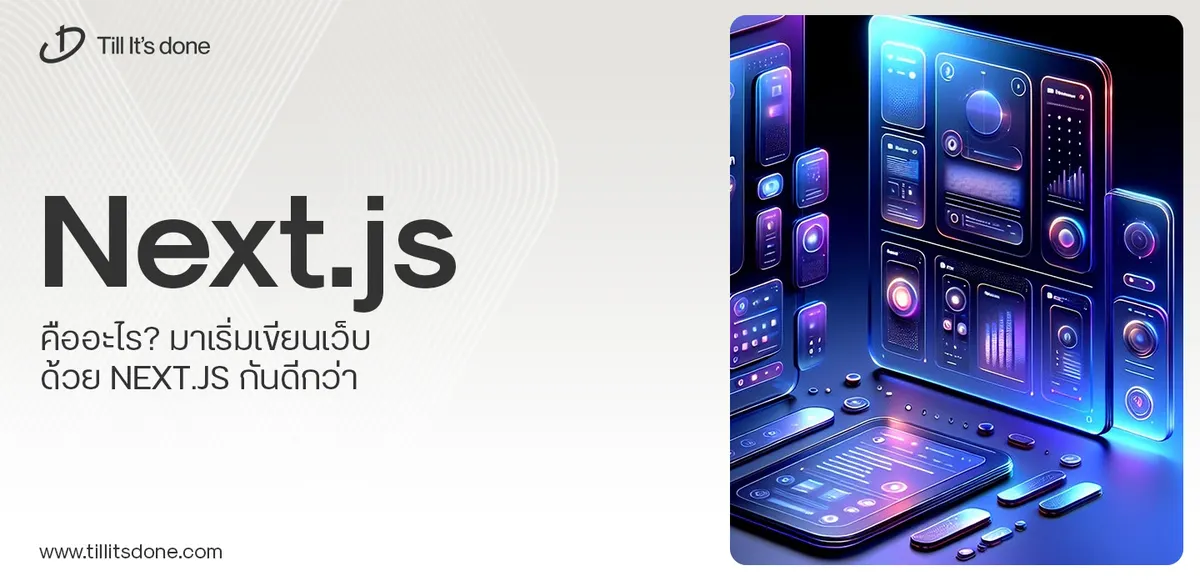
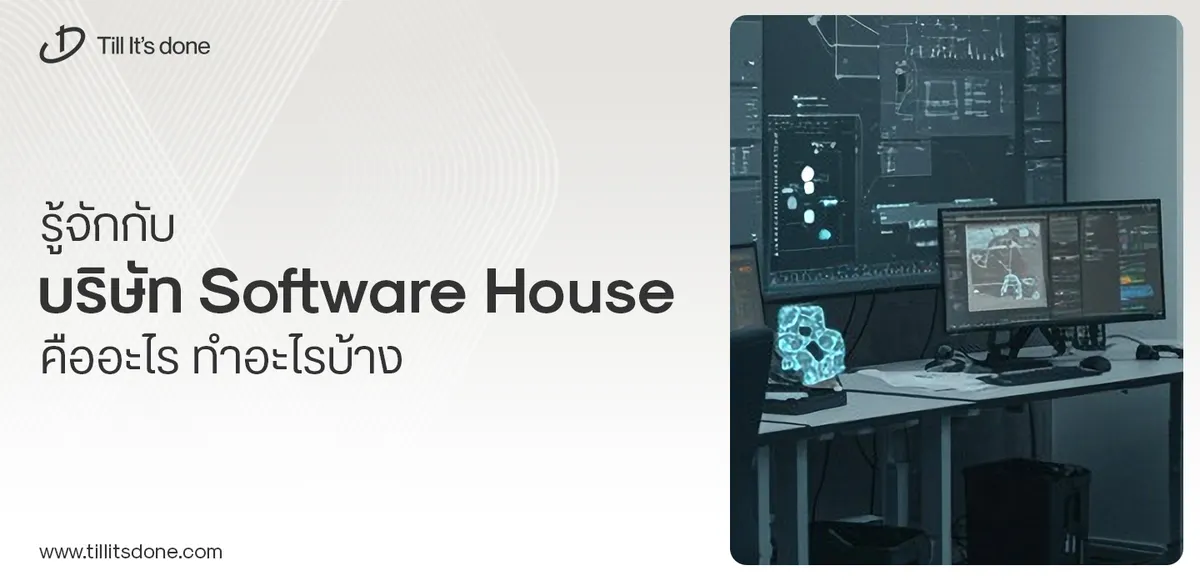
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.