- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Integrating Viper with Config Formats in Golang
Master configuration management with practical examples and best practices.

Integrating Viper with JSON, YAML, and TOML Configuration Formats
Managing configuration in Go applications can be tricky, especially when dealing with multiple config formats. That’s where Viper comes to the rescue! In this post, I’ll walk you through how to seamlessly integrate Viper with JSON, YAML, and TOML formats, making your configuration management a breeze.
Why Choose Viper?
Before diving in, let’s talk about why Viper is such a fantastic choice. Think of Viper as a Swiss Army knife for configuration management. It not only supports multiple formats but also handles environment variables, command-line flags, and remote configuration systems. Pretty cool, right?
Setting Up Viper in Your Project
First things first, let’s get Viper into your project. It’s as simple as running:
go get github.com/spf13/viper
Working with Different Config Formats
JSON Configuration
JSON is probably the most familiar format for many developers. Here’s how you can use it with Viper:
viper.SetConfigName("config") // name of config file (without extension)viper.SetConfigType("json") // REQUIRED if the config file does not have the extension in the nameviper.AddConfigPath(".") // path to look for the config file inerr := viper.ReadInConfig() // Find and read the config file
Your JSON config might look something like this:
{ "database": { "host": "localhost", "port": 5432, "name": "myapp" }, "server": { "port": 8080 }}
YAML Configuration
YAML is great for its readability. Here’s how to implement it:
viper.SetConfigName("config")viper.SetConfigType("yaml")viper.AddConfigPath(".")err := viper.ReadInConfig()
And your YAML config might look like:
database: host: localhost port: 5432 name: myappserver: port: 8080
TOML Configuration
TOML strikes a nice balance between readability and features:
viper.SetConfigName("config")viper.SetConfigType("toml")viper.AddConfigPath(".")err := viper.ReadInConfig()
Your TOML config would look like:
[database]host = "localhost"port = 5432name = "myapp"
[server]port = 8080
Pro Tips for Working with Viper
- You can set default values before loading the config:
viper.SetDefault("database.port", 5432)
- Watch for config changes in real-time:
viper.WatchConfig()viper.OnConfigChange(func(e fsnotify.Event) { fmt.Println("Config file changed:", e.Name)})
- Access nested values easily:
dbPort := viper.GetInt("database.port")
Best Practices
- Keep your configuration files in a dedicated directory
- Use environment variables for sensitive information
- Implement validation for your configuration values
- Include example configuration files in your repository
- Document all available configuration options
Wrapping Up
Viper makes configuration management in Go a joy to work with. Whether you prefer JSON, YAML, or TOML, you can easily integrate it into your application. The flexibility to switch between formats without changing your application code is a massive win for maintainability and user preference.
Happy coding!



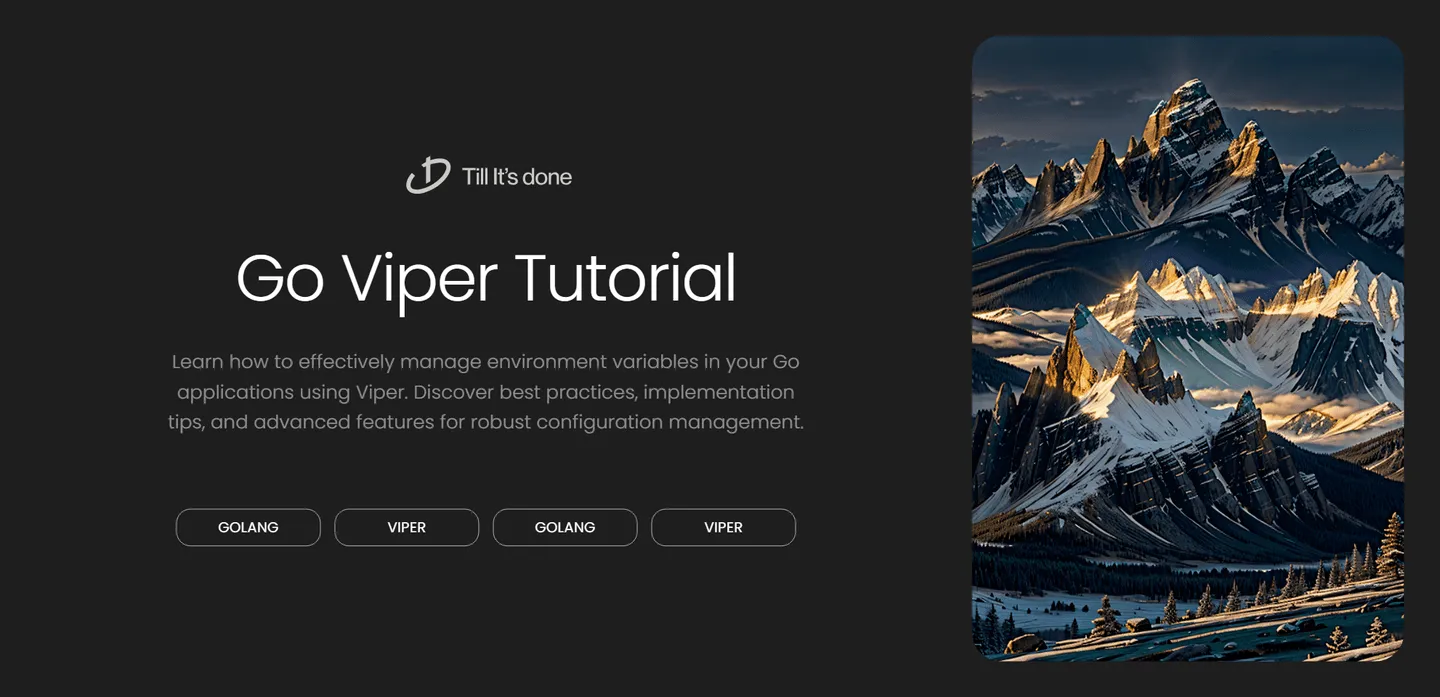
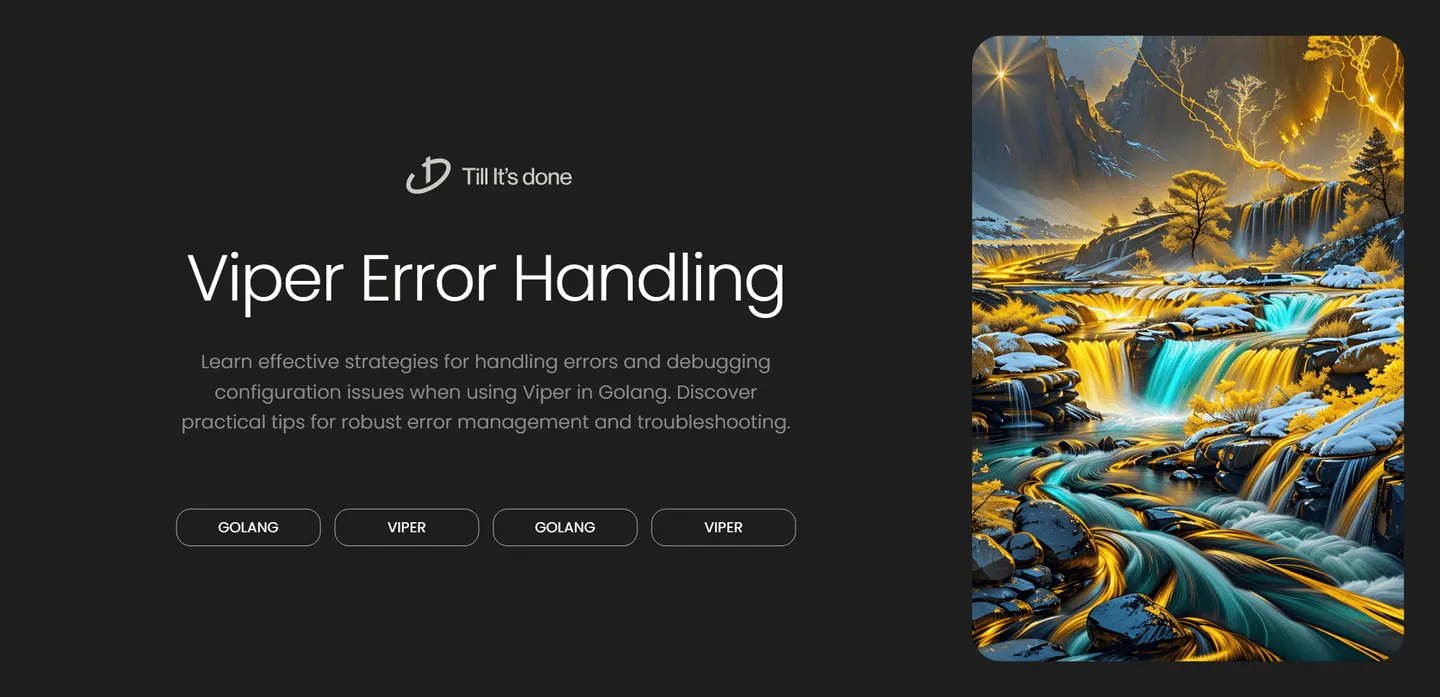

Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.