- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Error Handling & Debugging with Viper in Go
Discover practical tips for robust error management and troubleshooting.
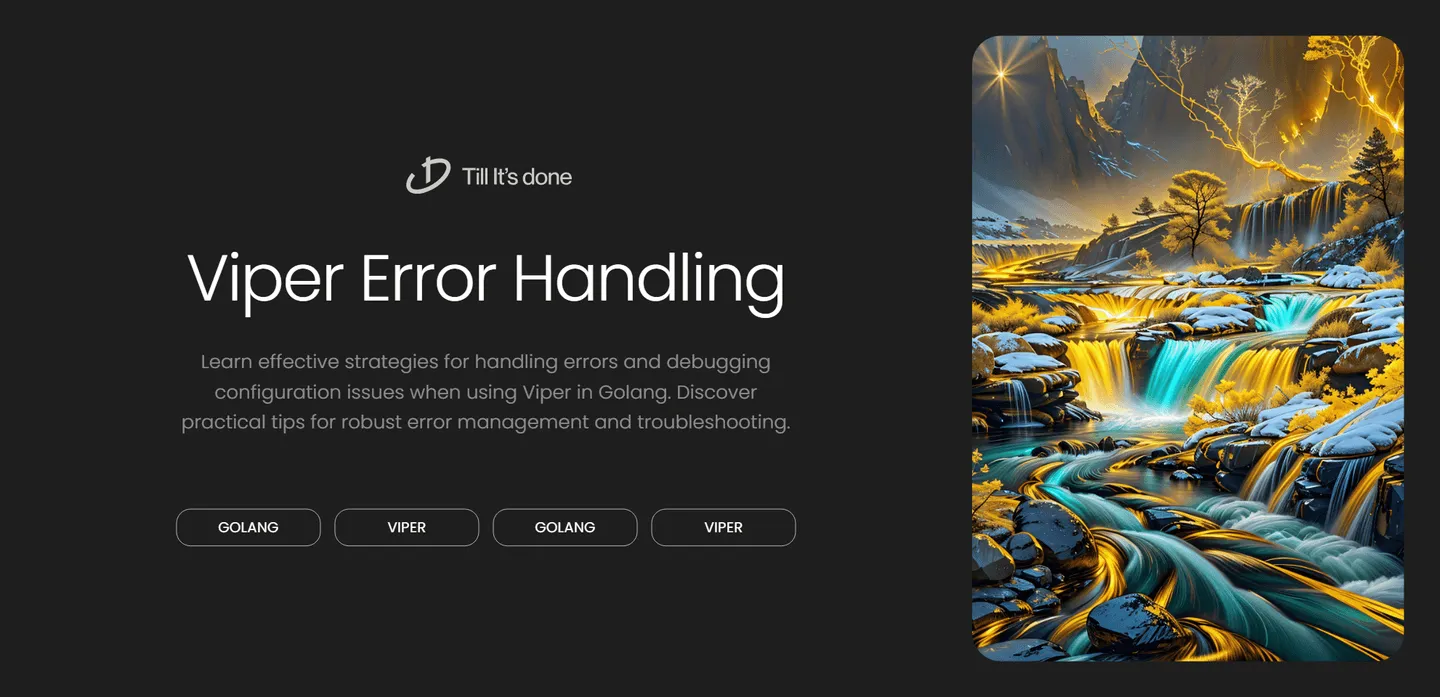
Error Handling and Debugging Configuration Issues with Viper
Working with configuration in Go applications can sometimes feel like navigating a maze. While Viper makes our lives easier, we still need to handle errors gracefully and debug configuration issues effectively. Let’s dive into some practical approaches to tackle these challenges.
Common Configuration Issues
One of the most frustrating moments in development is when your application fails due to configuration errors. With Viper, these usually fall into a few categories: missing files, incorrect paths, or malformed configuration data.
Here’s the thing - these issues are totally normal! Think of configuration as a bridge between your application and its environment. Like any bridge, it needs proper maintenance and error checking.
Essential Error Handling Patterns
When working with Viper, implementing robust error handling isn’t just good practice - it’s crucial for maintaining sanity during development and production.
Let’s look at some practical error handling patterns:
func loadConfig() error { viper.SetConfigName("config") viper.AddConfigPath(".")
if err := viper.ReadInConfig(); err != nil { if _, ok := err.(viper.ConfigFileNotFoundError); ok { return fmt.Errorf("config file not found: %v", err) } return fmt.Errorf("failed to read config: %v", err) }
// Validate required settings requiredKeys := []string{"database.host", "api.port"} for _, key := range requiredKeys { if !viper.IsSet(key) { return fmt.Errorf("required config key missing: %s", key) } }
return nil}
Debugging Techniques
When things go wrong (and they will), having a solid debugging strategy is your best friend. Consider these approaches:
- Enable Viper’s debug logging:
viper.Debug()
- Implement configuration dumping:
func dumpConfig() { settings := viper.AllSettings() out, _ := json.MarshalIndent(settings, "", " ") fmt.Printf("Current configuration: %s\n", string(out))}
Best Practices for Production
Remember to implement environment-specific configuration handling. You might want different behavior in development versus production. Here’s a pattern I’ve found particularly useful:
func initConfig() error { env := os.Getenv("APP_ENV") if env == "" { env = "development" }
viper.SetConfigName(fmt.Sprintf("config.%s", env)) // ... rest of configuration logic}
Conclusion
Error handling and debugging in Viper doesn’t have to be overwhelming. By implementing proper error handling patterns, maintaining good debugging practices, and following environment-specific configurations, you can build more robust and maintainable applications.
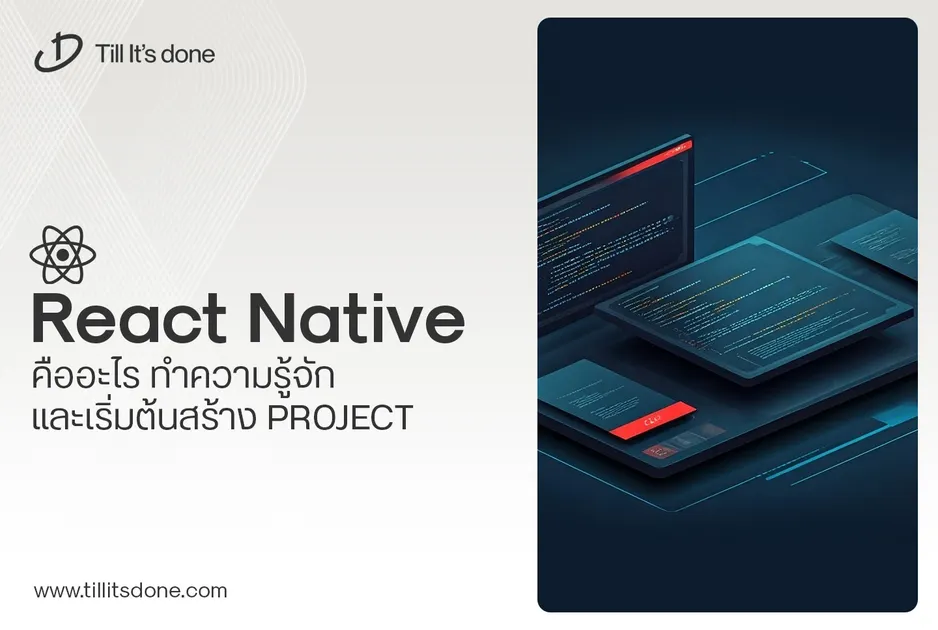
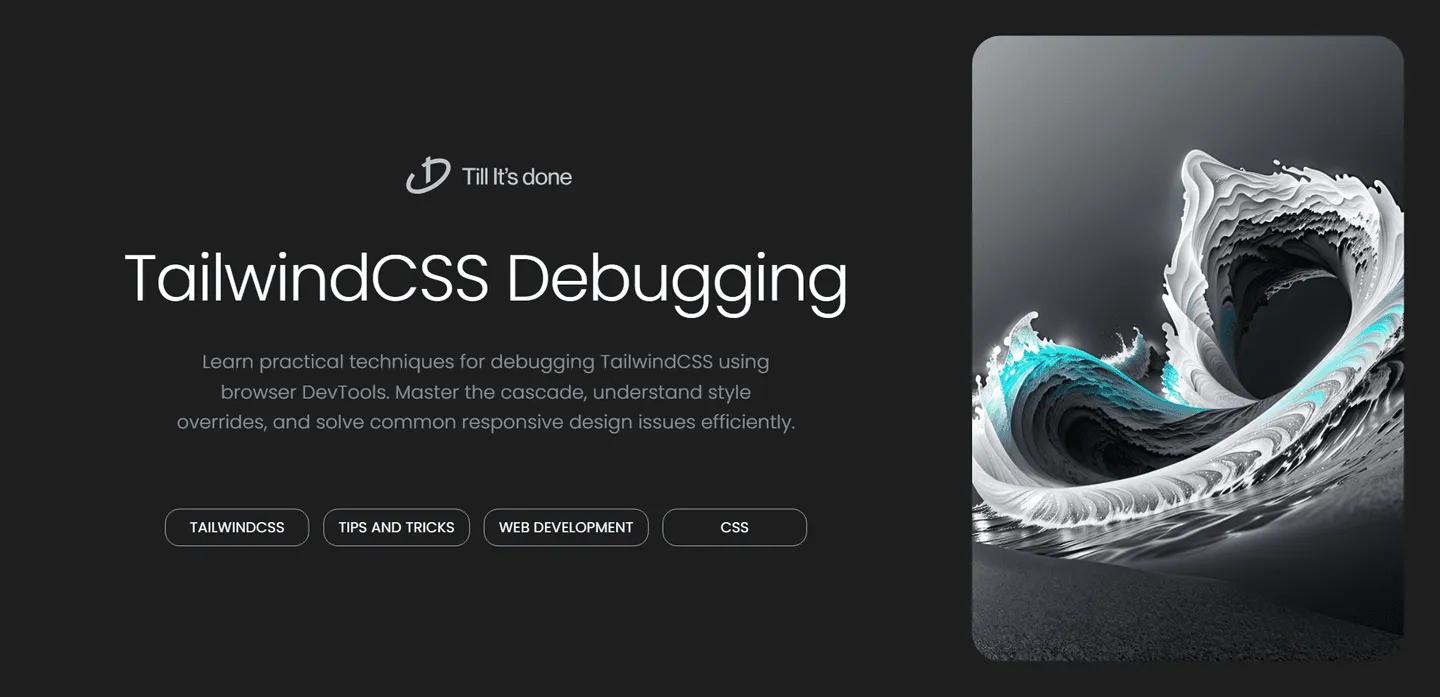
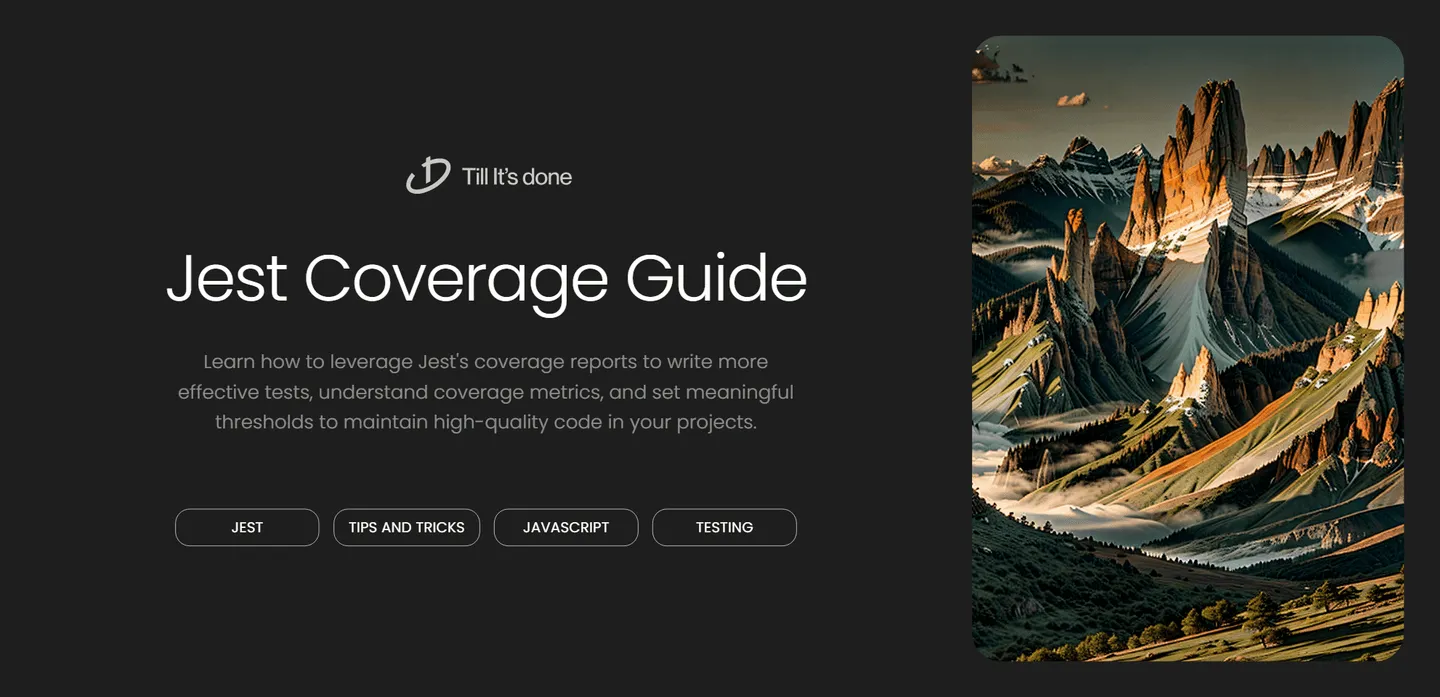
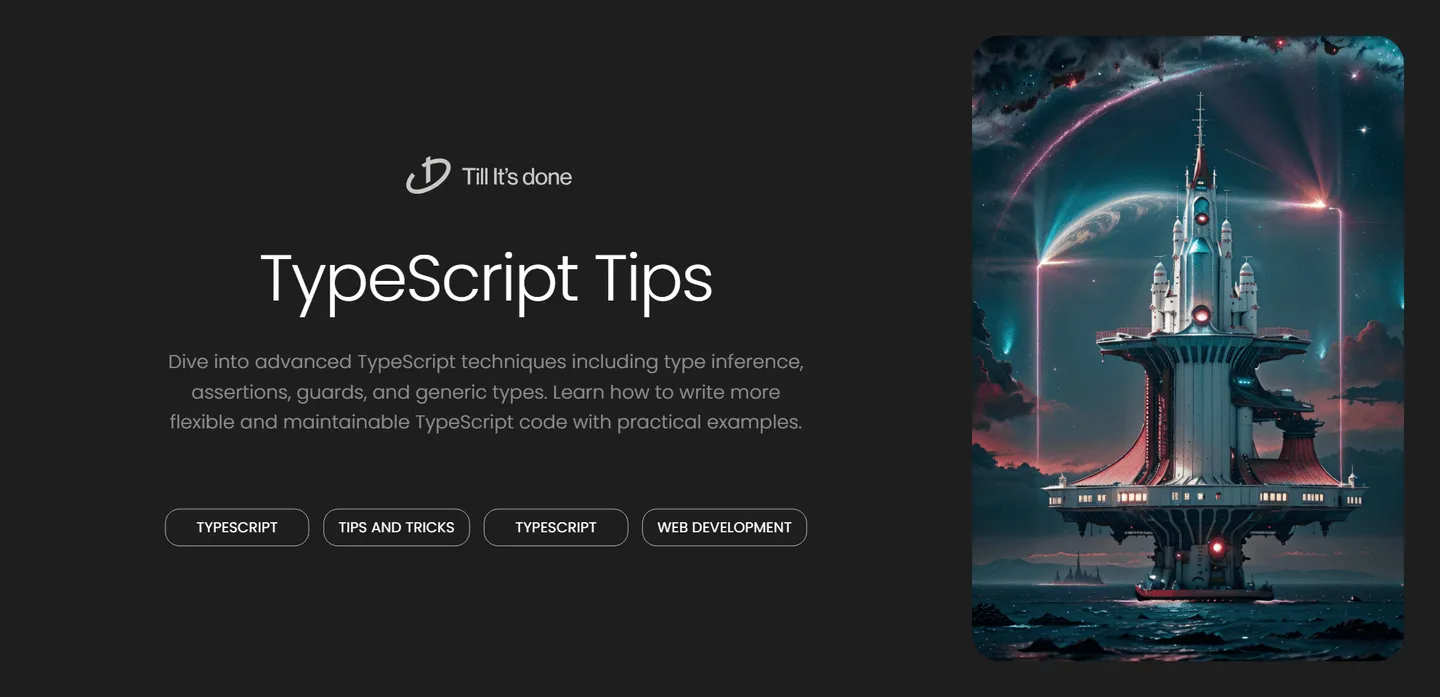
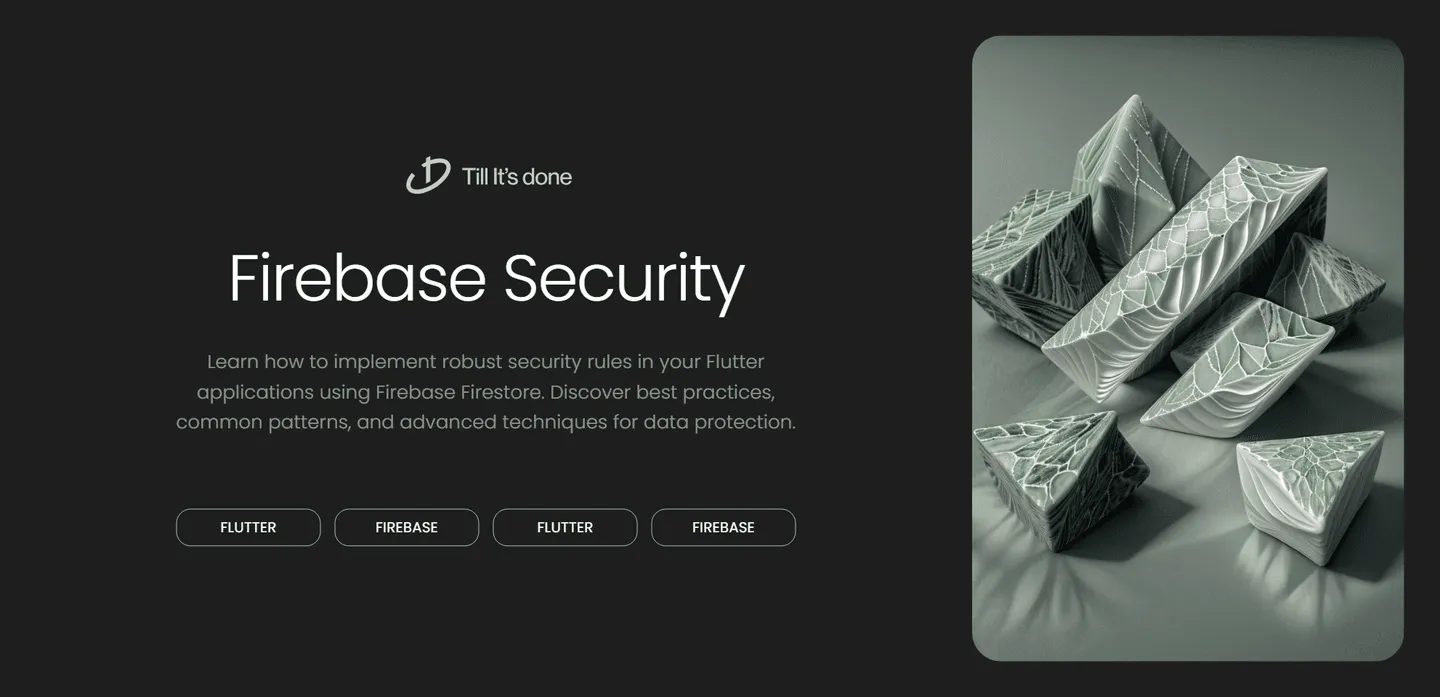
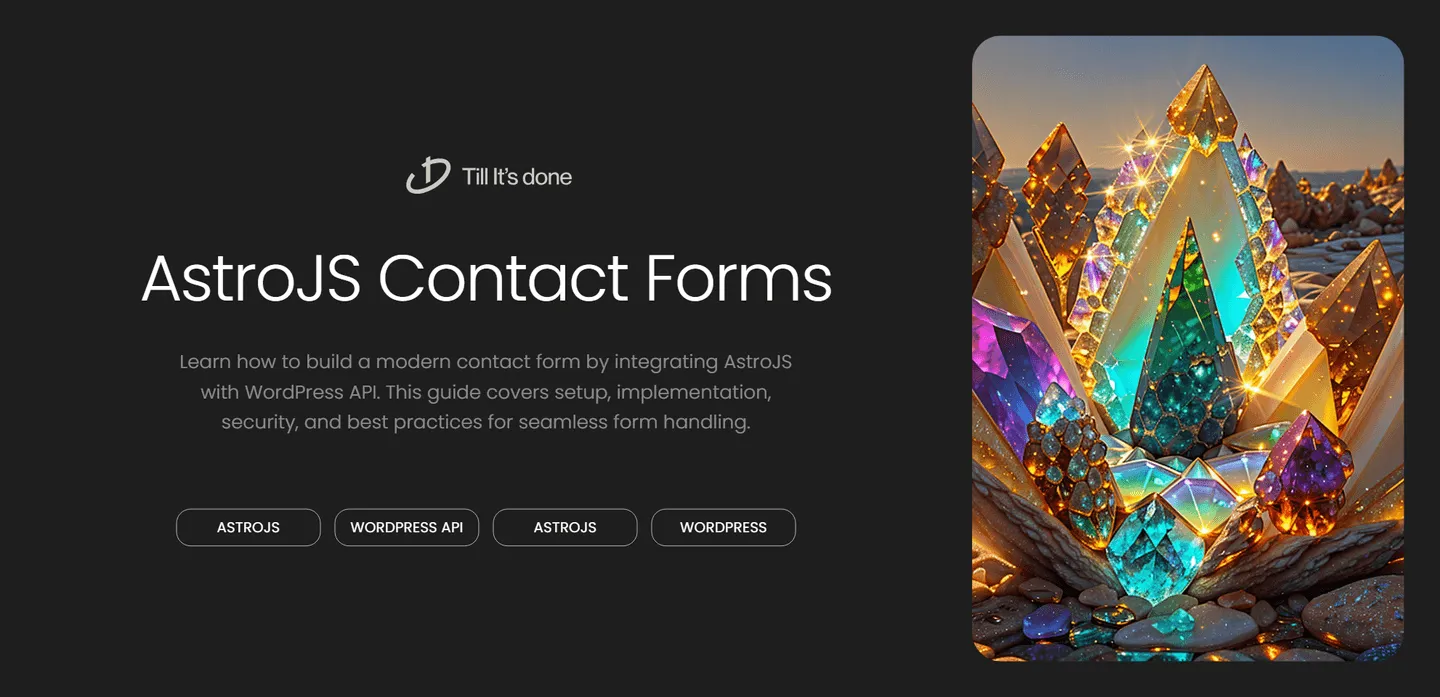
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.