- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master TypeScript Utility Types for Better Code
A practical guide with real-world examples.
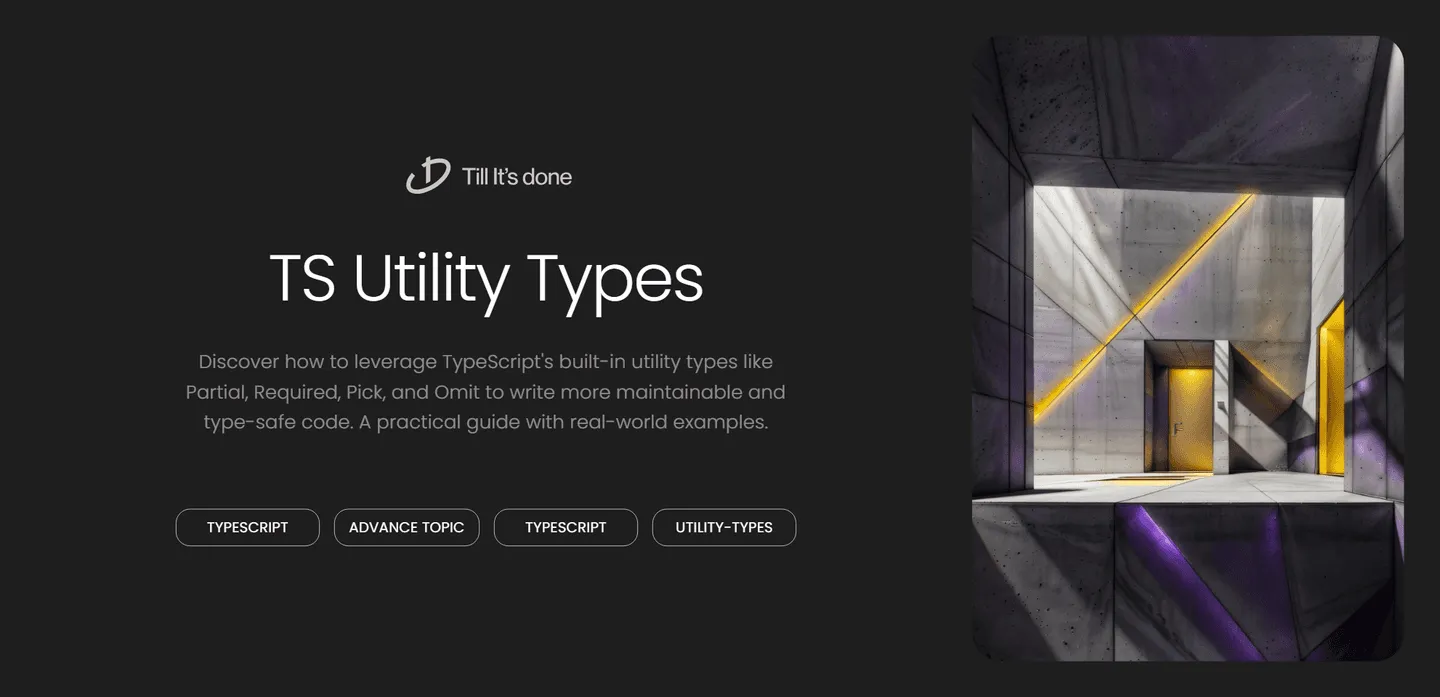
Mastering Utility Types in TypeScript: A Practical Guide
TypeScript’s utility types are like your Swiss Army knife for type transformations. They’re built-in tools that help you modify and manipulate types with ease, making your code more flexible and type-safe. Let’s dive deep into these powerful features that every TypeScript developer should master.
Understanding the Foundations
Before we dive into the more complex utility types, let’s start with the fundamentals. Think of utility types as type transformers - they take an existing type and create a new one with modified properties.
Partial<T>: Making Properties Optional
One of the most commonly used utility types is Partial\<T\>
. Imagine you’re updating a user’s profile, but you only want to update some fields, not all of them. This is where Partial\<T\>
shines:
interface User { name: string; email: string; age: number;}
function updateUser(userId: string, updates: Partial<User>) { // Only some properties of User are required}
// This works perfectly fine!updateUser('123', { name: 'John' });
Required<T>: The Opposite of Partial
Sometimes you need to make all properties required. Required\<T\>
is your go-to utility type for this:
interface ConfigSettings { theme?: string; language?: string; debugMode?: boolean;}
// Now all properties are requiredtype MandatoryConfig = Required<ConfigSettings>;
Advanced Type Manipulations
Let’s explore some more powerful utility types that can transform your types in fascinating ways.
Pick<T, K> and Omit<T, K>: Surgical Precision
These utility types let you create new types by either selecting or removing specific properties:
interface Article { title: string; content: string; publishDate: Date; author: string; tags: string[];}
// Just what we need for a previewtype ArticlePreview = Pick<Article, 'title' | 'author'>;
// Everything except tagstype CleanArticle = Omit<Article, 'tags'>;
Record<K, T>: Type-Safe Dictionaries
Record
is perfect for creating type-safe key-value pairs:
type CategoryCounts = Record<string, number>;
const postsByCategory: CategoryCounts = { technology: 23, lifestyle: 15, sports: 42};
Real-World Applications
Let’s look at some practical scenarios where utility types really shine:
// Combining utility types for powerful transformationstype ReadOnlyUser = Readonly<Pick<User, 'id' | 'email'>>;
// Making nested structures partially mutabletype MutableNestedObject\<T\> = { -readonly [P in keyof T]: T[P] extends object ? MutableNestedObject<T[P]> : T[P];};
Best Practices and Tips
- Chain utility types for complex transformations
- Use conditional types with utility types for dynamic type creation
- Leverage mapped types for consistent type modifications
- Document your custom utility types thoroughly
Remember, TypeScript’s utility types are not just syntactic sugar - they’re powerful tools that can make your codebase more maintainable and type-safe.
Keep exploring and experimenting with these utility types. They’re not just types - they’re the building blocks of robust TypeScript applications. Happy coding!
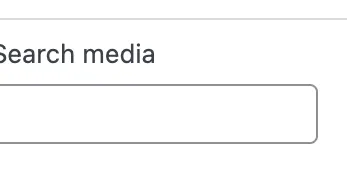





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.