- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Master TypeScript's Type System: Essential Tips
Learn about type inference, union types, generics, and best practices to write more maintainable code.
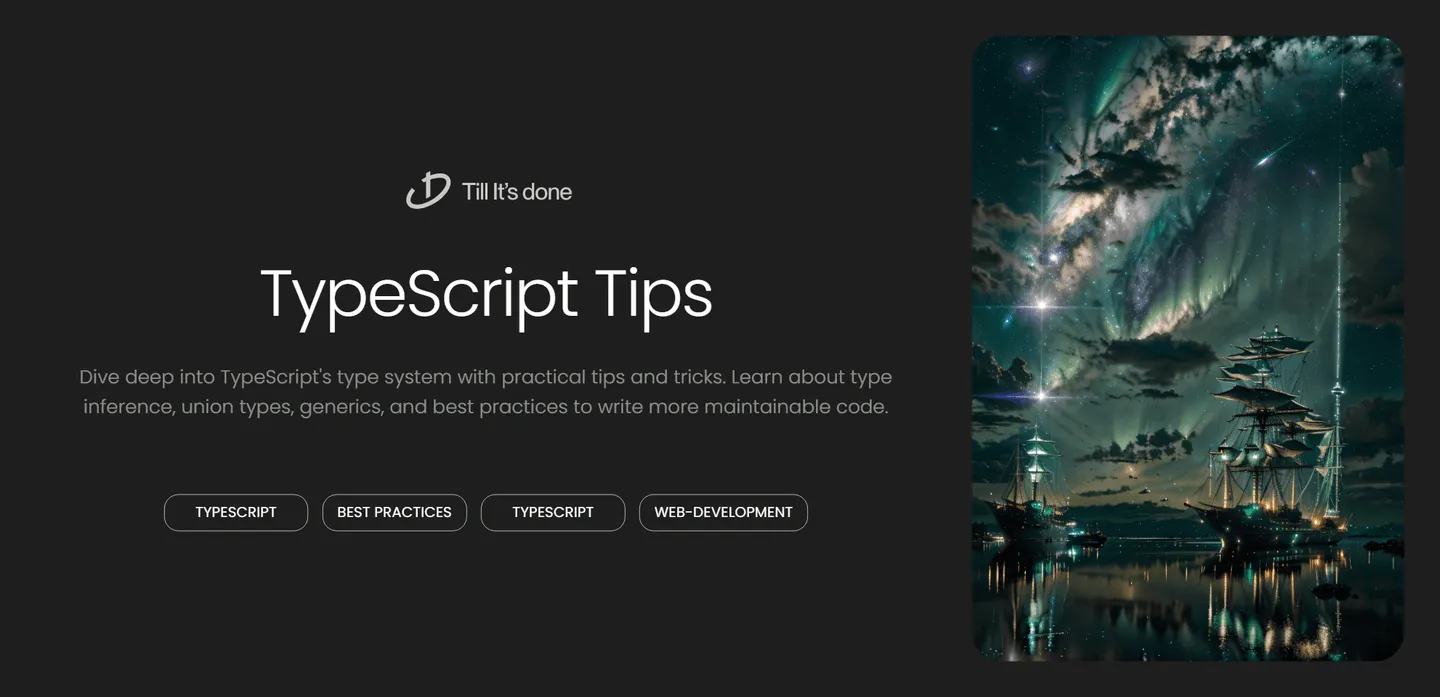
Effective Use of TypeScript’s Type System: Tips and Tricks
TypeScript has revolutionized how we write JavaScript, bringing robust type safety to our everyday coding. But are you truly harnessing its full potential? Let’s dive into some game-changing tips and tricks that’ll level up your TypeScript game.
Understanding Type Inference
One of TypeScript’s most powerful features is its ability to infer types automatically. While it might be tempting to explicitly type everything, sometimes less is more:
// Instead of thisconst numbers: number[] = [1, 2, 3];const user: { name: string; age: number } = { name: 'John', age: 30 };
// Let TypeScript do the heavy liftingconst numbers = [1, 2, 3];const user = { name: 'John', age: 30 };
The Power of Union Types
Union types are your best friends when dealing with multiple possible types. They’re especially useful when handling API responses or form inputs:
type ApiResponse = SuccessResponse | ErrorResponse;
interface SuccessResponse { status: 'success'; data: unknown;}
interface ErrorResponse { status: 'error'; message: string;}
Type Guards: Your Safety Net
Type guards help you narrow down types safely. They’re essential for handling complex data structures:
function processResponse(response: ApiResponse) { if (response.status === 'success') { // TypeScript knows this is SuccessResponse console.log(response.data); } else { // TypeScript knows this is ErrorResponse console.log(response.message); }}
Generics: The Ultimate Flexibility
Generics provide incredible flexibility while maintaining type safety. They’re perfect for creating reusable components and utilities:
function createState\<T\>(initial: T): [T, (newValue: T) => void] { let value = initial; const setValue = (newValue: T) => { value = newValue; }; return [value, setValue];}
// TypeScript knows exactly what types we're working withconst [count, setCount] = createState(0);const [user, setUser] = createState({ name: 'John' });
Best Practices to Live By
- Enable strict mode in your tsconfig.json. It might seem annoying at first, but it’ll save you countless hours of debugging.
- Use type aliases and interfaces to create meaningful, reusable types.
- Leverage mapped types and utility types to reduce code duplication.
- Don’t abuse ‘any’ - it defeats the purpose of using TypeScript.
- Document complex types with JSDoc comments for better team collaboration.
Remember, TypeScript is more than just a type system - it’s a powerful tool for building maintainable and scalable applications. The key is finding the right balance between type safety and code readability.
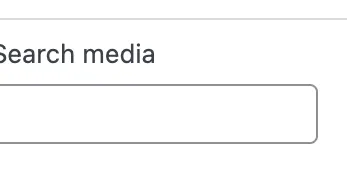





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.