- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Best Practices for TypeScript Applications
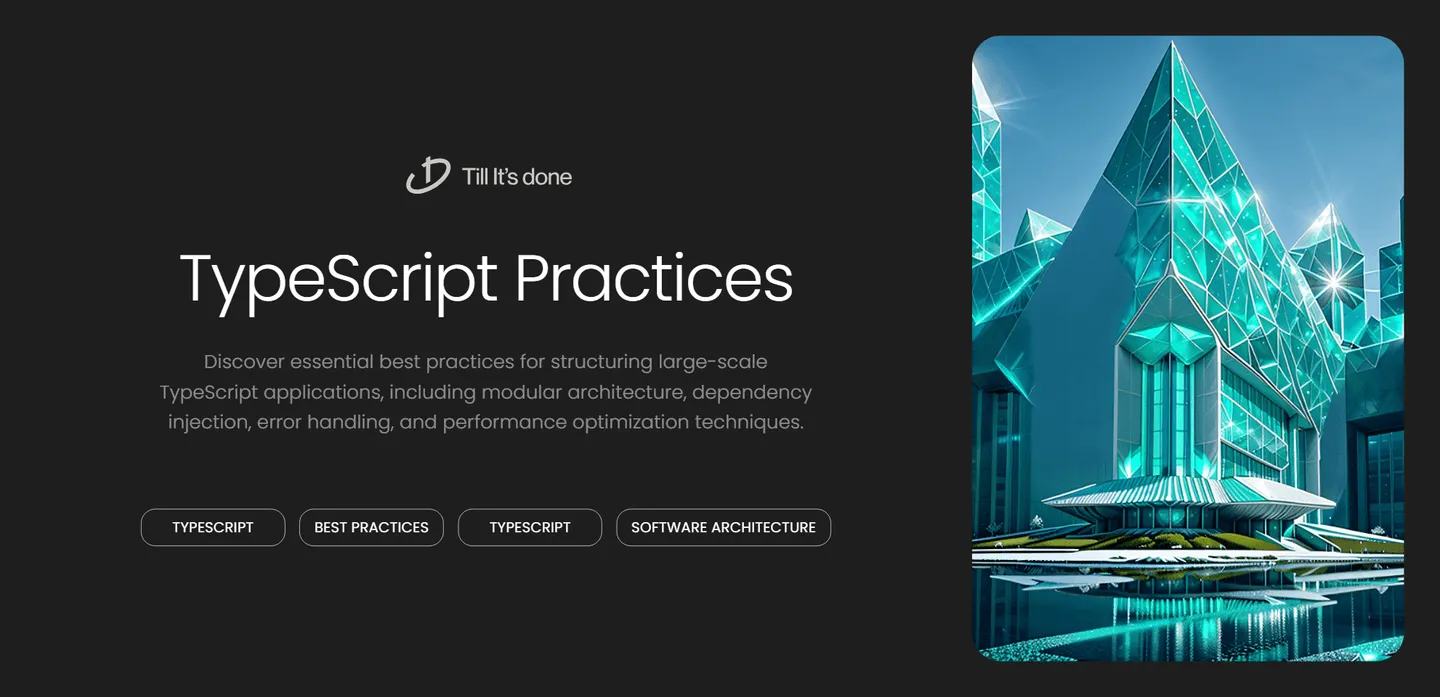
Best Practices for Structuring Large TypeScript Applications
As TypeScript applications grow larger, maintaining a clean and scalable codebase becomes increasingly challenging. Through years of experience and countless refactoring sessions, I’ve discovered that proper structure isn’t just about organizing files – it’s about creating a sustainable ecosystem where your code can evolve gracefully.
The Foundation: Project Architecture
The backbone of any well-structured TypeScript application lies in its architecture. Let’s dive into the key principles that can make or break your project’s maintainability.
Module Organization
Think of your application as a well-organized city. Each district (module) serves a specific purpose, and the streets (interfaces) connecting them are carefully planned. Here’s how I approach it:
src/ ├── core/ // Core business logic ├── features/ // Feature-specific modules ├── shared/ // Shared utilities and components ├── infrastructure/ // External service integrations └── types/ // Global type definitions
The Power of Barrel Files
One practice that’s transformed how I manage imports is using barrel files (index.ts). They act as a single entry point for module exports, making import statements cleaner and more maintainable:
export * from './auth.service';export * from './auth.types';export * from './auth.utils';
Type Safety and Domain Modeling
The real power of TypeScript shines when you leverage its type system effectively. Here are some battle-tested practices:
Strong Domain Types
Instead of using primitive types everywhere, create meaningful domain types that carry business logic:
// Badfunction processUser(name: string, age: number) { ... }
// Goodtype User = { name: string; age: number; role: UserRole;}
function processUser(user: User) { ... }
Dependency Injection
Implement a robust dependency injection system to maintain loose coupling between modules:
interface IUserService { getUser(id: string): Promise<User>;}
class UserController { constructor(private userService: IUserService) {}
async handleUserRequest(id: string) { return await this.userService.getUser(id); }}
Error Handling and Validation
One aspect that often gets overlooked is consistent error handling. I’ve found that creating a unified approach to errors saves countless hours of debugging:
class ApplicationError extends Error { constructor( public code: string, public message: string, public details?: unknown ) { super(message); }}
Performance Considerations
When scaling TypeScript applications, performance becomes crucial. Here are some practices I’ve found invaluable:
- Use lazy loading for modules where possible
- Implement proper memoization for expensive computations
- Keep an eye on bundle size through proper code splitting
- Leverage TypeScript’s const assertions for better compile-time optimizations
Conclusion
Structuring large TypeScript applications is an art that combines technical expertise with architectural vision. By following these practices, you’re not just organizing code – you’re building a foundation that will support your application’s growth for years to come.
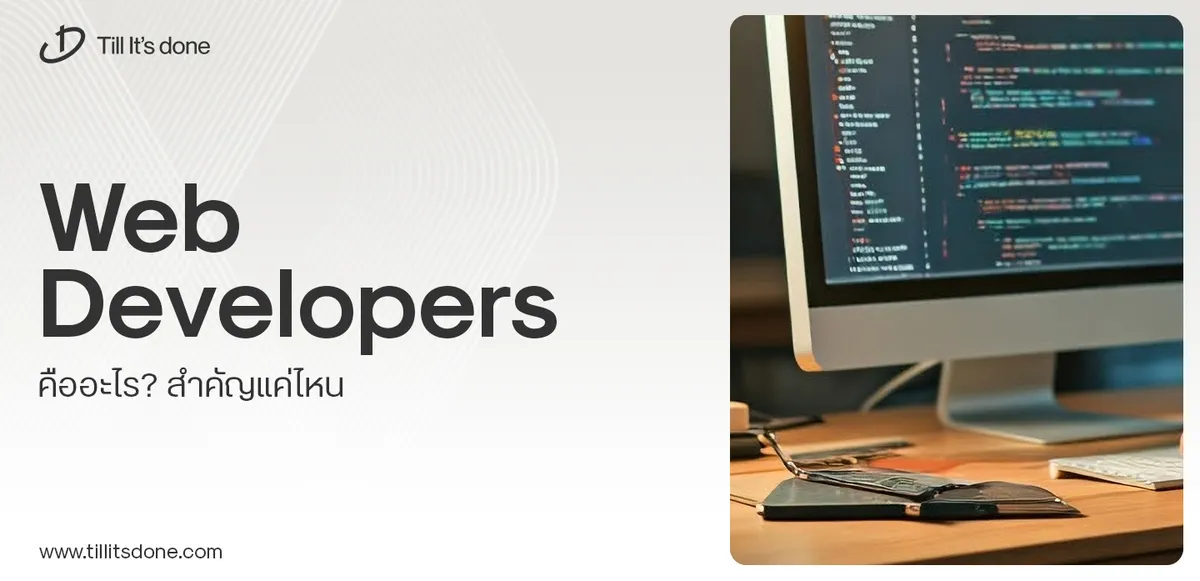
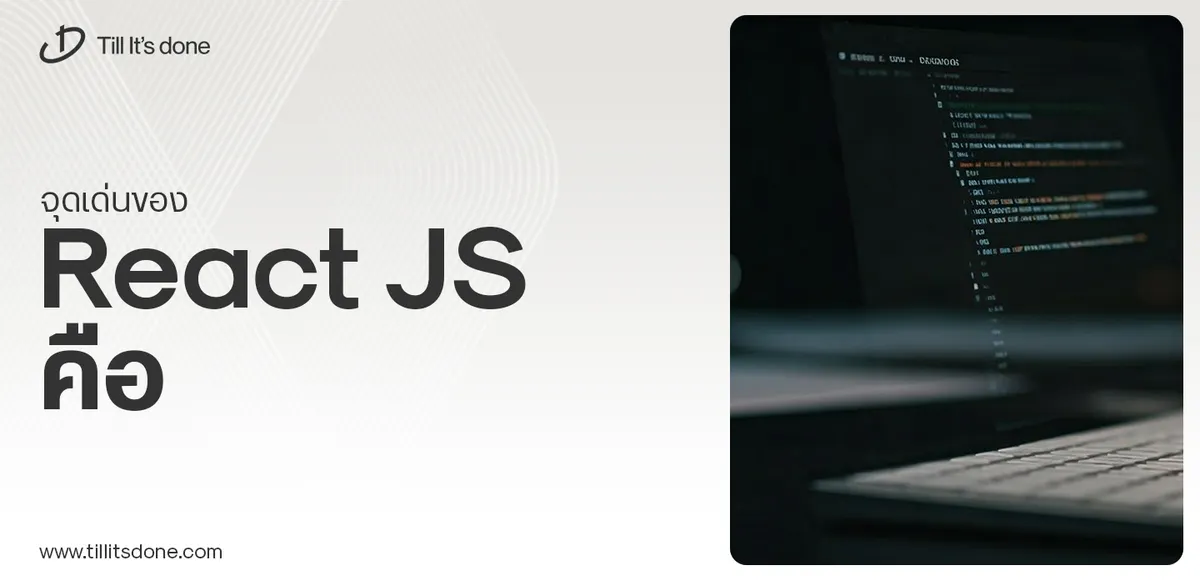
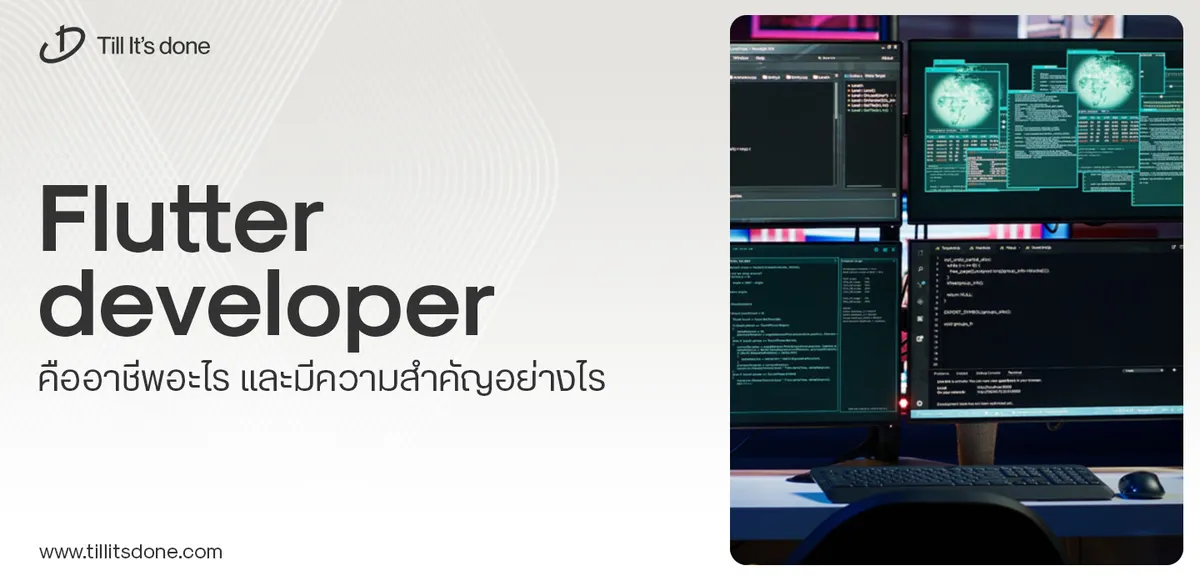
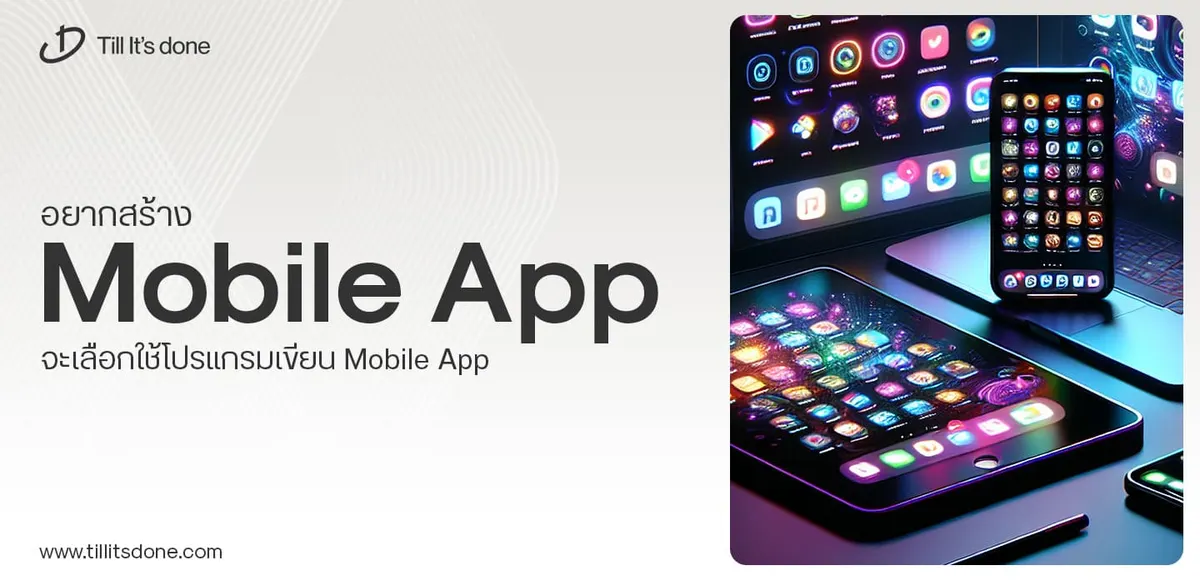
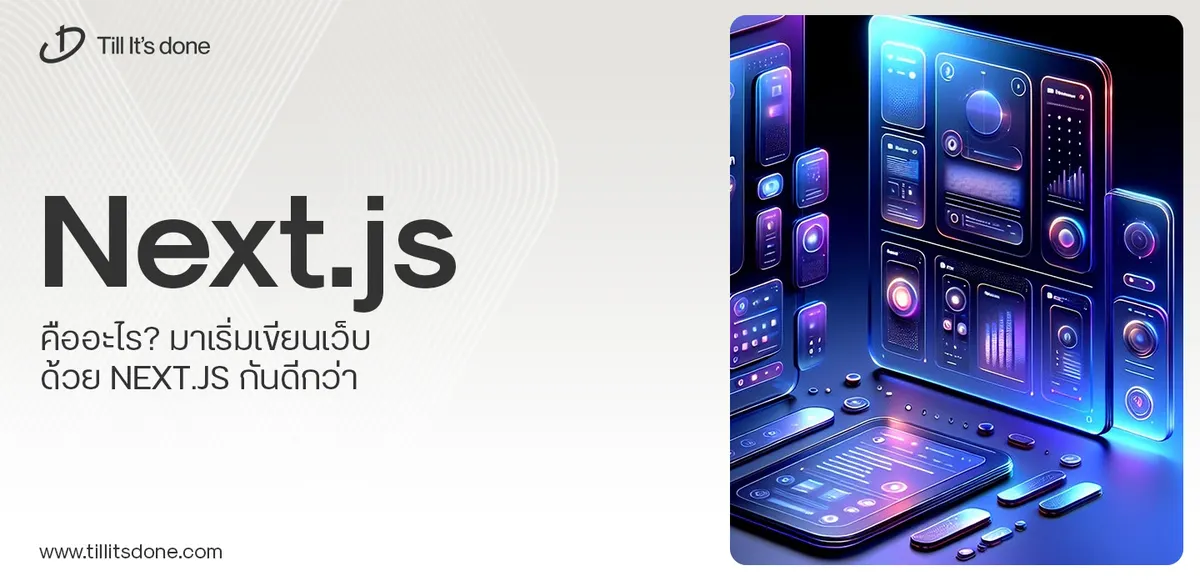
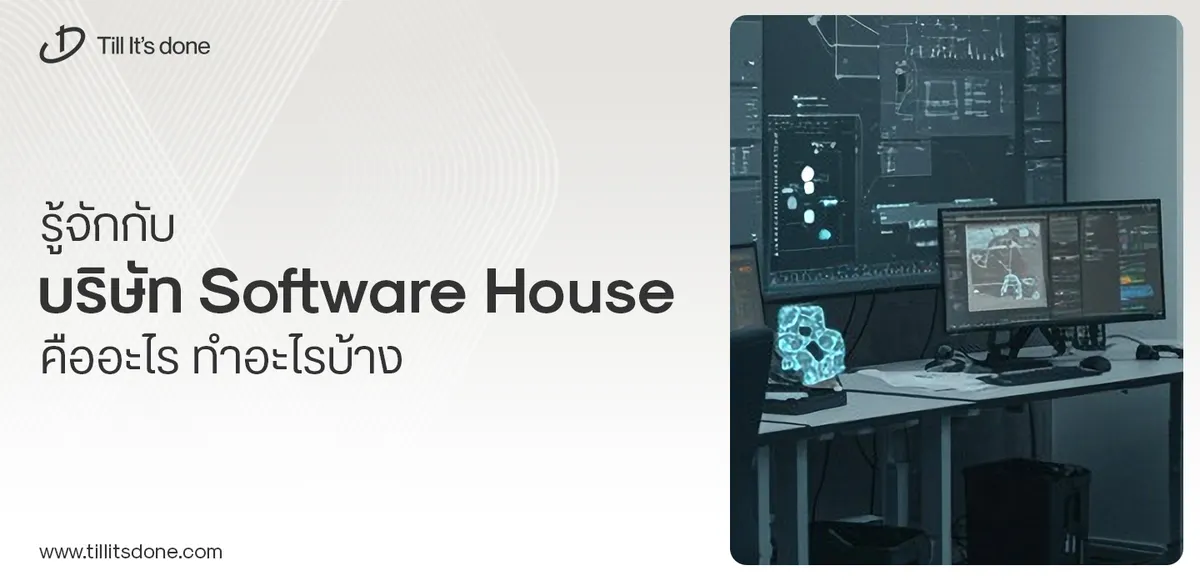
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.