- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Common TypeScript Pitfalls and How to Avoid Them
Learn best practices for writing safer, more maintainable TypeScript code.

Common TypeScript Pitfalls and How to Avoid Them
Hey there, fellow developers! 👋 If you’ve been working with TypeScript, you know it’s an amazing tool that helps us write safer, more maintainable code. But let’s be honest – we’ve all fallen into some common traps along the way. Today, I’m going to share some TypeScript pitfalls I’ve encountered and show you how to dodge them like a pro.
The Type Assertion Temptation
One of the biggest traps I see developers falling into is overusing type assertions (the as
keyword). It’s tempting to silence TypeScript’s complaints with a quick type assertion, but this can lead to runtime errors.
// 🚫 Don't do thisconst userInput: any = getUserInput();const username = userInput as string;
// ✅ Do this insteadconst userInput = getUserInput();if (typeof userInput === 'string') { const username = userInput;} else { handleInvalidInput();}
The Optional Chaining Oversight
Sometimes we get too excited about optional chaining and nullish coalescing operators, forgetting that they might mask underlying issues in our code structure.
// 🚫 Potentially masking design problemsconst displayName = user?.profile?.settings?.displayName ?? 'Anonymous';
// ✅ Better approach with proper type definitionsinterface UserSettings { displayName: string;}
interface UserProfile { settings: UserSettings;}
interface User { profile: UserProfile;}
The Generic Type Confusion
Here’s something that trips up even experienced developers: not constraining generic types properly. This can lead to confusing error messages and unexpected behavior.
// 🚫 Too permissivefunction merge<T, U>(obj1: T, obj2: U) { return { ...obj1, ...obj2 };}
// ✅ Properly constrainedfunction merge<T extends object, U extends object>(obj1: T, obj2: U) { return { ...obj1, ...obj2 };}
The Interface vs Type Alias Debate
While both interfaces and type aliases can define object types, choosing the wrong one for your use case can impact code maintainability and compilation performance.
// Choose interfaces when you need:interface User { name: string; age: number;}
interface User { email: string; // Declaration merging works!}
// Choose type aliases when you need:type Status = 'pending' | 'approved' | 'rejected';type HttpMethod = 'GET' | 'POST' | 'PUT' | 'DELETE';
The Strict Mode Avoidance
I get it – strict mode can feel like fighting with the compiler. But disabling strict mode or using // @ts-ignore
is like taking the batteries out of your smoke detector because it keeps beeping!
// In your tsconfig.json{ "compilerOptions": { "strict": true, "noImplicitAny": true, "strictNullChecks": true // Keep these enabled! }}
Conclusion
Remember, TypeScript is here to help us write better code, not to make our lives difficult. By understanding and avoiding these common pitfalls, you’ll be able to leverage TypeScript’s full potential and write more maintainable applications.
Happy coding! 🚀
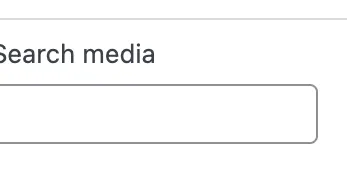





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.