- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
TypeScript Modules and Import/Export Syntax
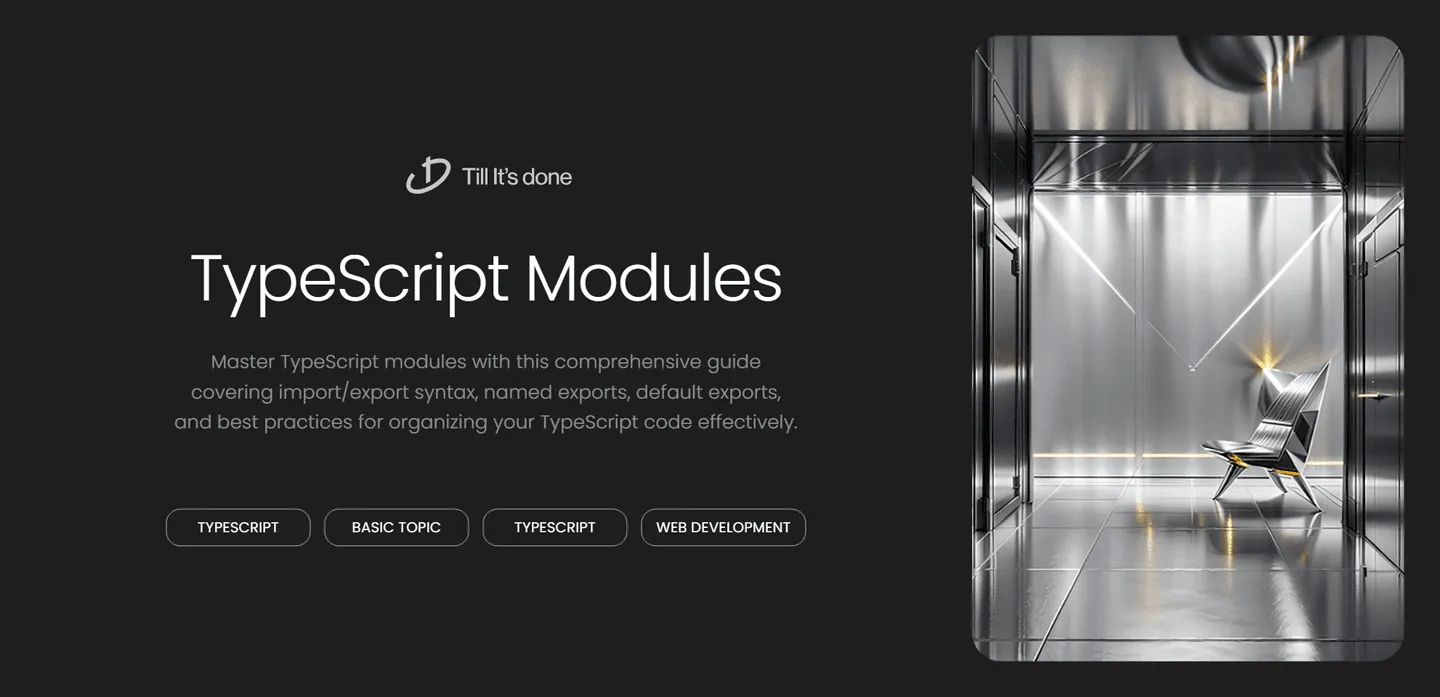
Understanding TypeScript Modules and Import/Export Syntax
If you’re diving into TypeScript, understanding modules and how to structure your code is crucial. Today, let’s explore TypeScript modules and master the import/export syntax in a way that’ll make your code more organized and maintainable.
What are TypeScript Modules?
Think of modules as containers for your code. Just like how we organize our clothes in different drawers, modules help us organize our code into manageable, reusable pieces. Each module can contain functions, classes, interfaces, or variables that we can share between different parts of our application.
Export Syntax: Sharing Your Code
TypeScript provides several ways to export your code. Let’s look at the most common approaches:
Named Exports
The most straightforward way to share your code is through named exports. You can export multiple items from a single module:
export const add = (a: number, b: number): number => a + b;export const subtract = (a: number, b: number): number => a - b;
export interface MathOperation { (x: number, y: number): number;}
Default Exports
When you want to export a single main thing from a module, use a default export:
export default class Calculator { add(a: number, b: number): number { return a + b; }}
Import Syntax: Using Shared Code
Now that we know how to export code, let’s see how to import it:
Importing Named Exports
import { add, subtract, MathOperation } from './math';
const result = add(5, 3);
Importing Default Exports
import Calculator from './calculator';
const calc = new Calculator();
Import Aliases
You can rename imports to avoid naming conflicts:
import { add as addNumbers } from './math';import * as MathUtils from './math';
Best Practices
- Keep one module per file to maintain clarity
- Use meaningful names for your modules
- Prefer named exports for better code discoverability
- Use barrel files (index.ts) to consolidate exports
- Be consistent with your import/export style
Remember, good module organization is like having a well-organized toolbox – it makes your work smoother and more efficient.
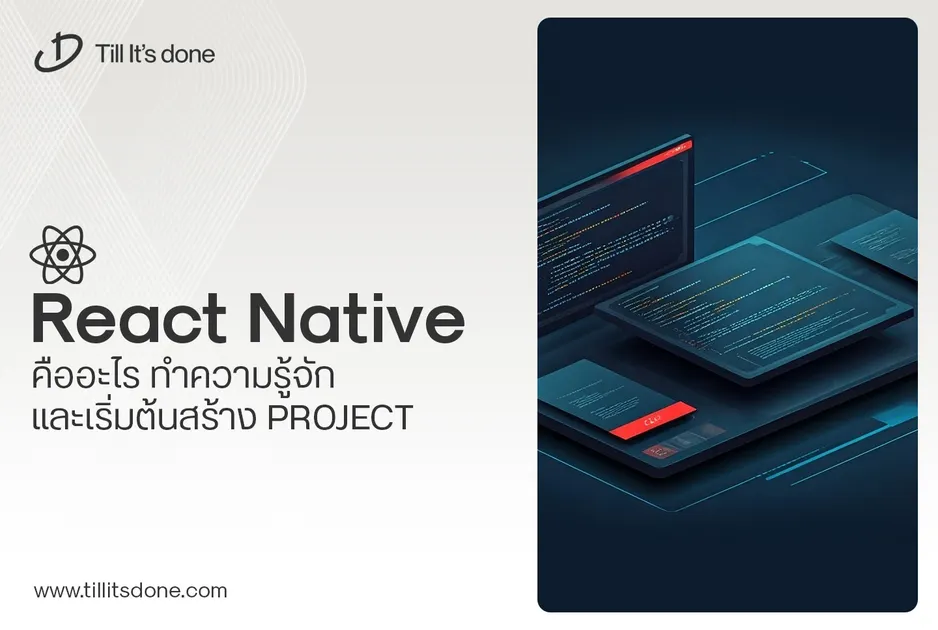
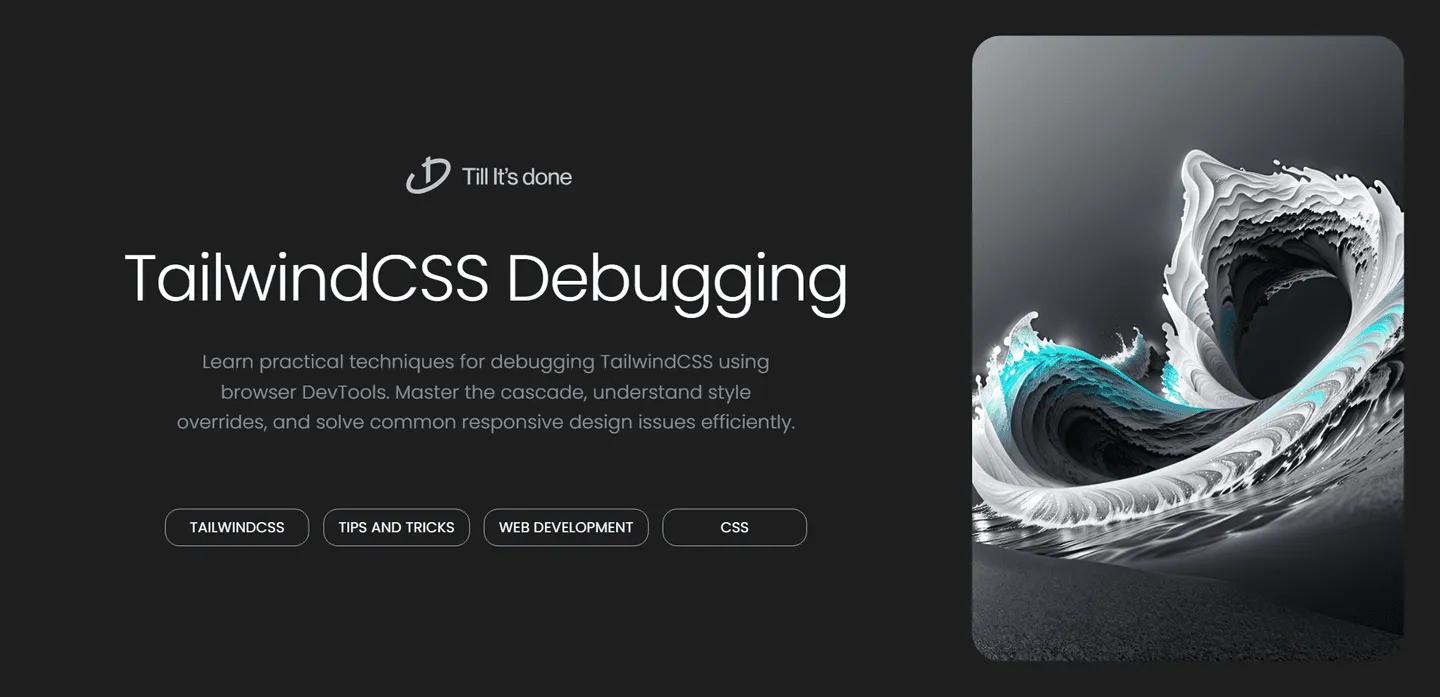
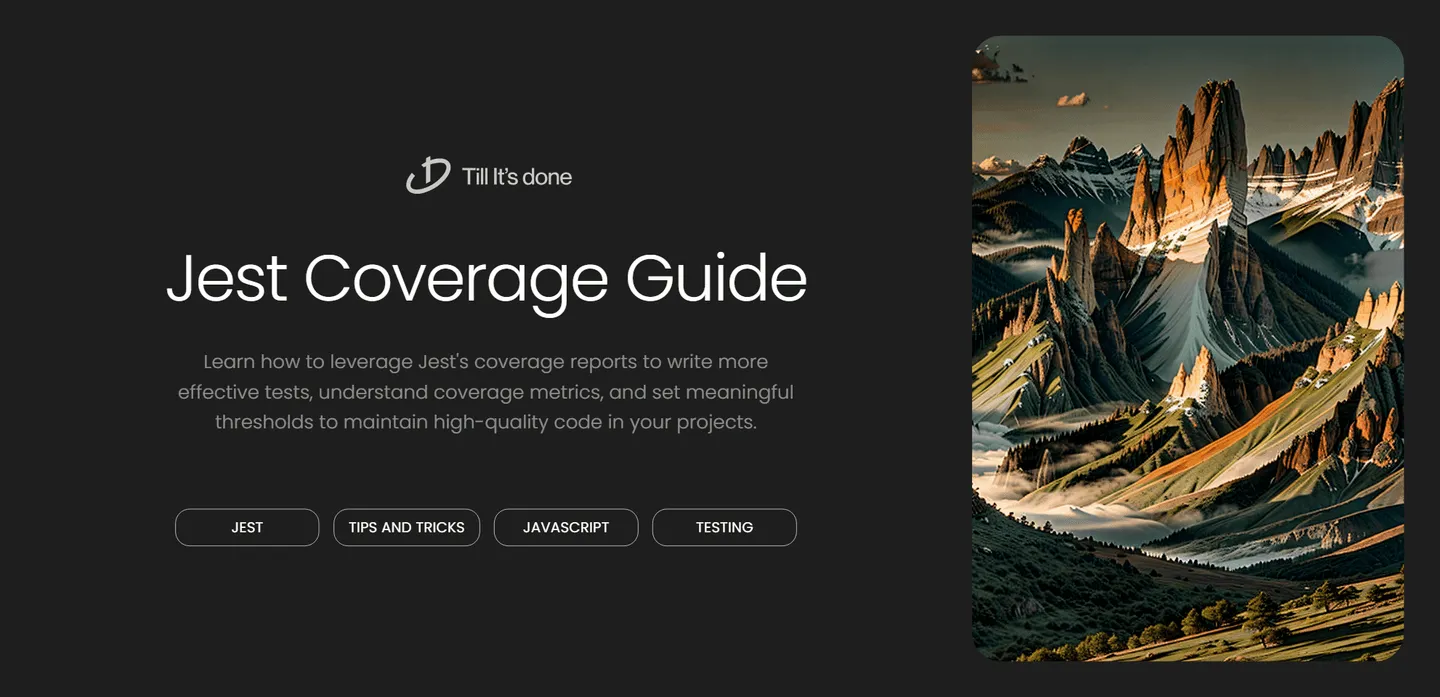
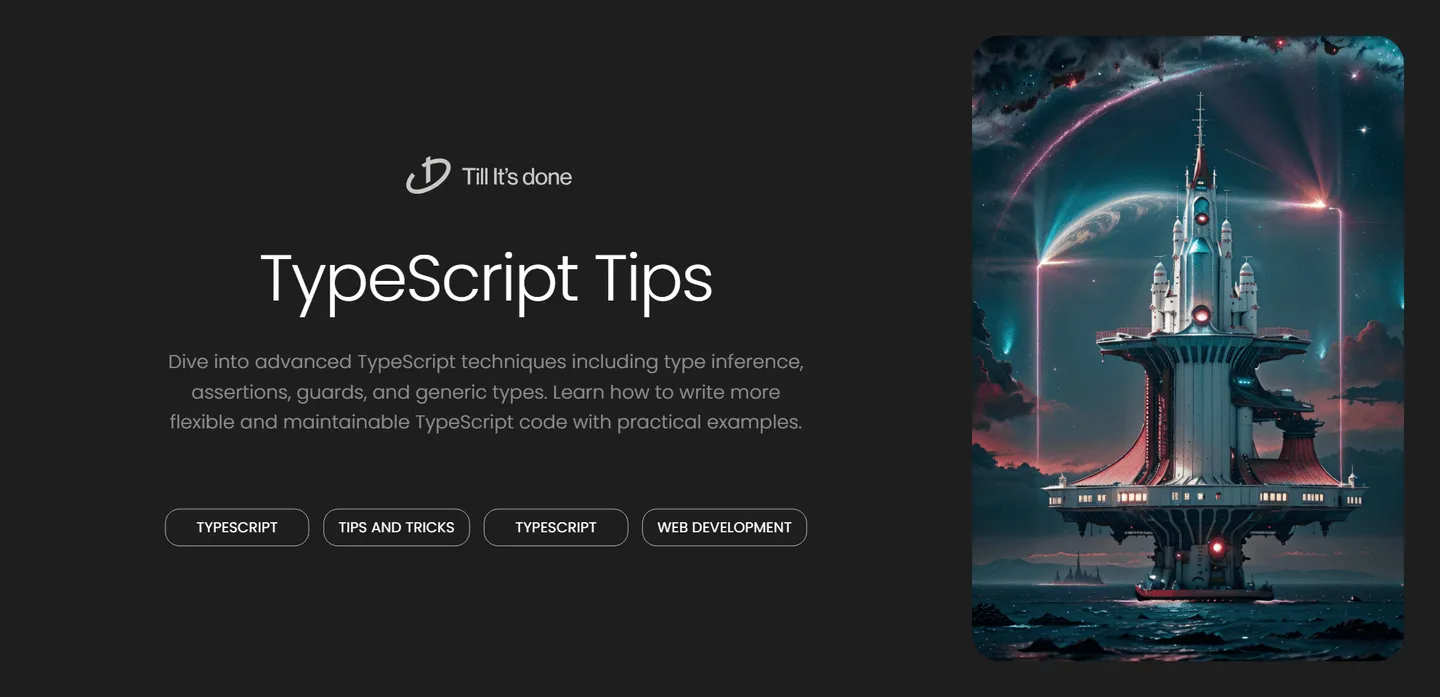
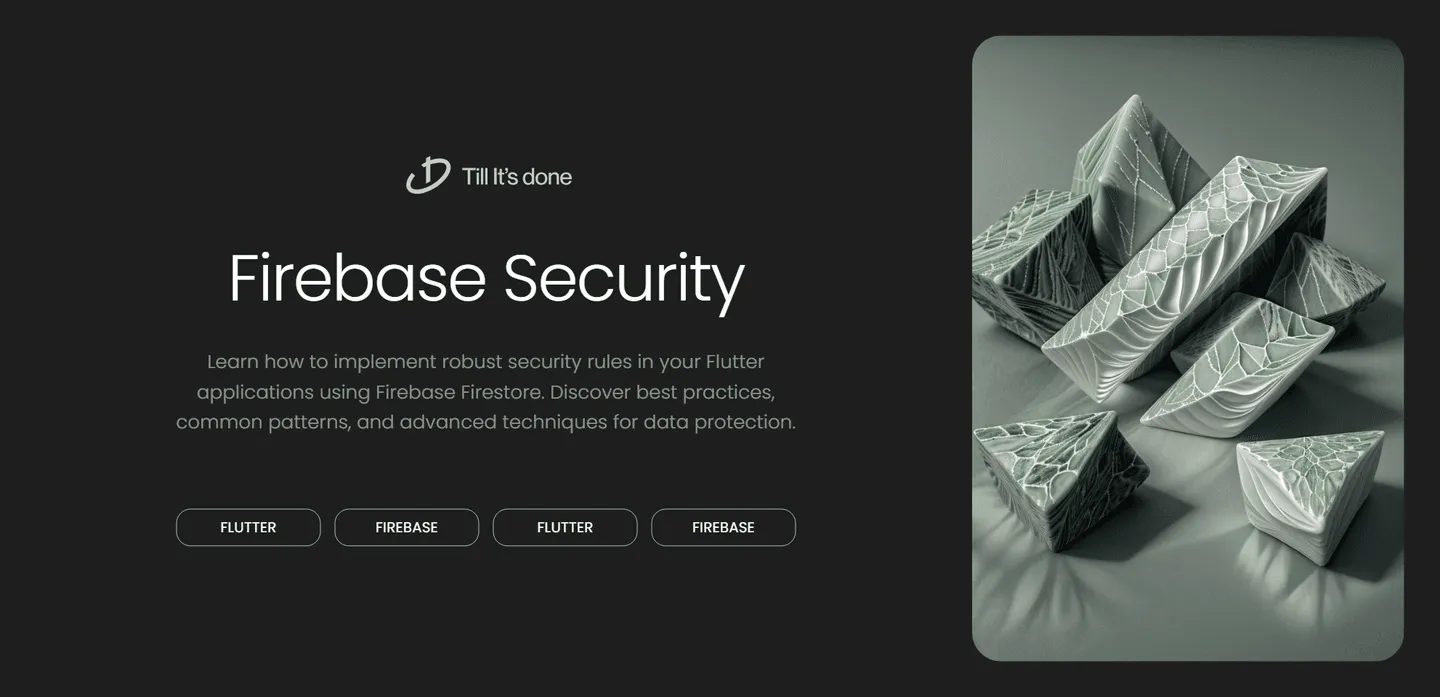
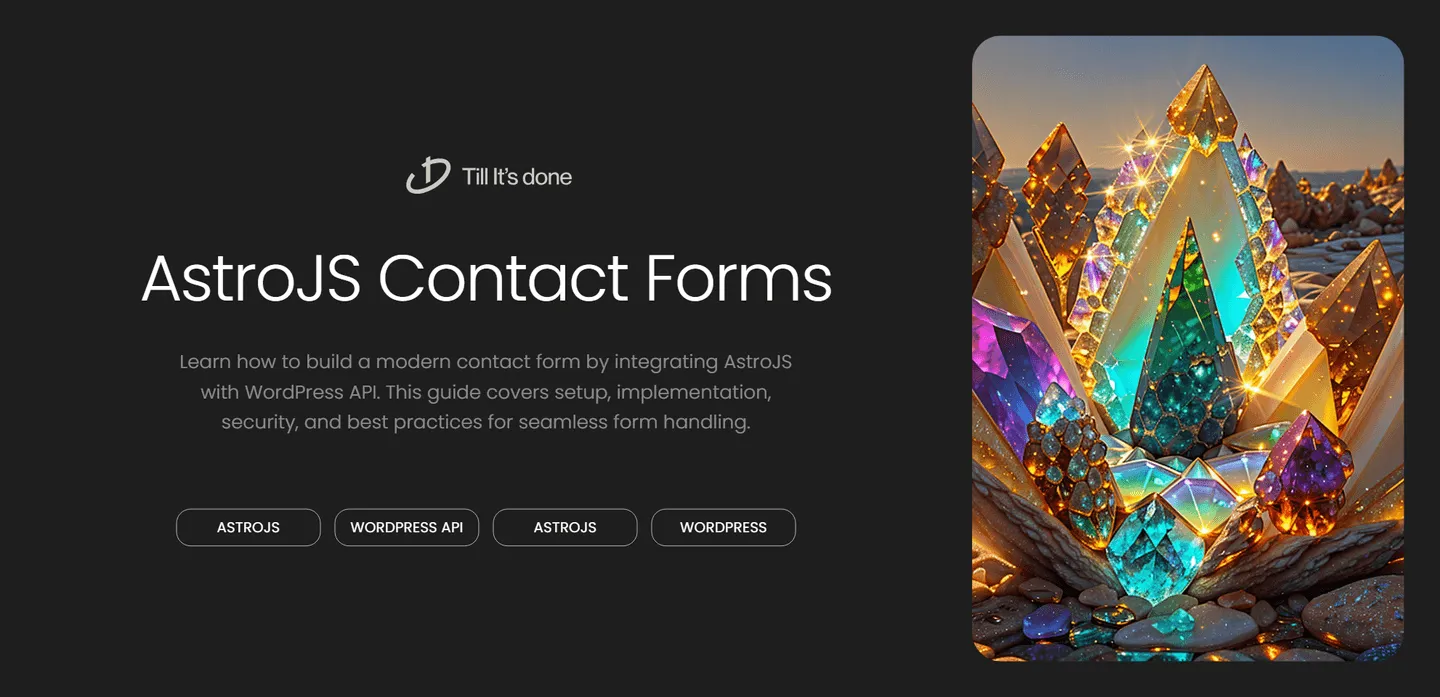
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.