- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
How to Use Interfaces in TypeScript Guide
Includes practical examples and best practices.
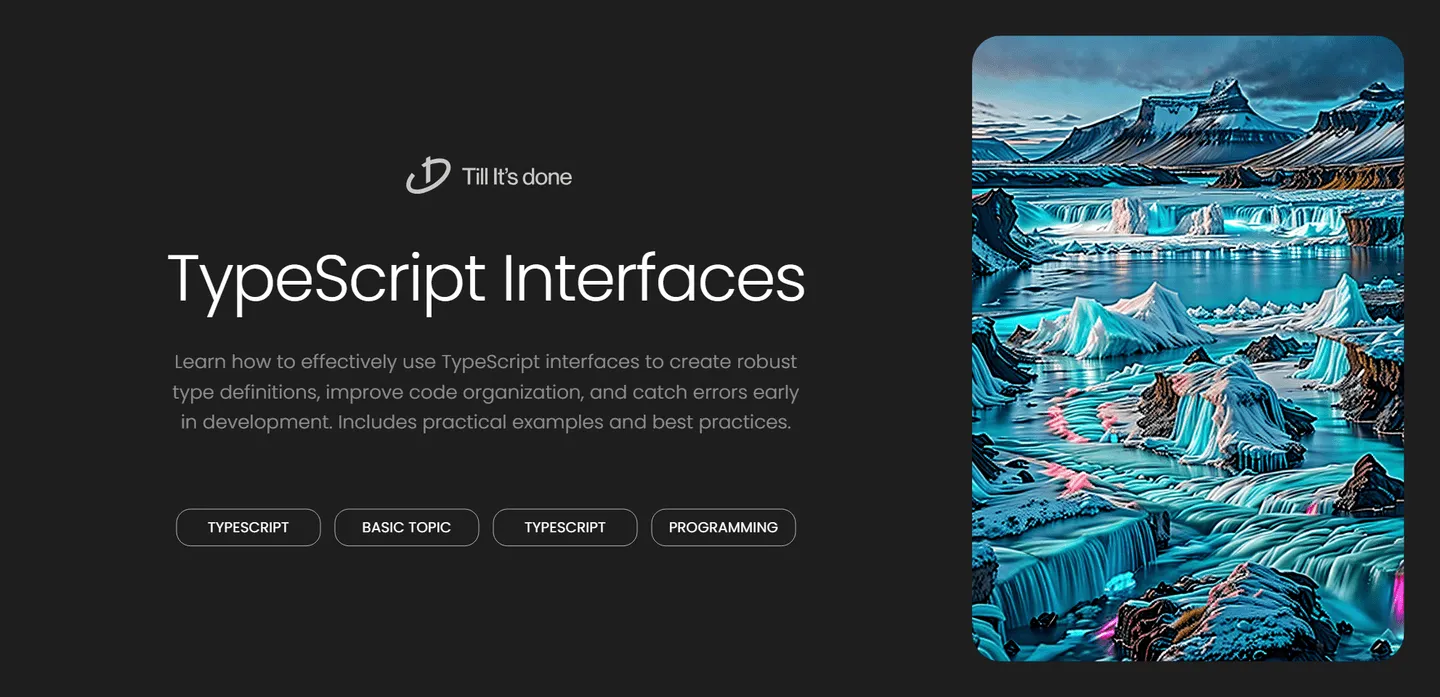
How to Use Interfaces in TypeScript: A Practical Guide
TypeScript interfaces are like blueprints for objects, helping us write cleaner and more maintainable code. Let’s dive into how they work and why they’re so powerful.
Understanding TypeScript Interfaces
Think of interfaces as contracts. When you create an interface, you’re defining a structure that objects must follow. It’s like creating a template that ensures consistency throughout your codebase.
interface User { name: string; email: string; age: number;}
Basic Interface Usage
Let’s see how we can use interfaces in practice. When you define an object that should follow an interface, TypeScript helps ensure you’re following the rules:
interface Product { id: number; name: string; price: number; inStock?: boolean; // Optional property}
const laptop: Product = { id: 1, name: "MacBook Pro", price: 1299 // inStock is optional, so we can skip it};
Advanced Interface Features
Interfaces can do more than just define object shapes. They can extend other interfaces, describe function types, and even define indexed properties.
Extending Interfaces
interface Animal { name: string; age: number;}
interface Dog extends Animal { breed: string; bark(): void;}
Readonly Properties
Sometimes you want to prevent properties from being changed after they’re initially set:
interface Config { readonly apiKey: string; readonly baseUrl: string;}
const config: Config = { apiKey: "abc123", baseUrl: "https://api.example.com"};
// This would cause an error:// config.apiKey = "xyz789";
Best Practices and Tips
- Name your interfaces using PascalCase
- Use descriptive names that reflect the purpose
- Keep interfaces focused and single-purpose
- Consider using readonly when properties shouldn’t change
- Use optional properties sparingly
Real-World Example
Here’s a practical example combining multiple interface concepts:
interface BaseEntity { id: number; createdAt: Date; updatedAt: Date;}
interface UserProfile extends BaseEntity { firstName: string; lastName: string; email: string; preferences: { theme: 'light' | 'dark'; notifications: boolean; };}
// Using the interfaceconst userProfile: UserProfile = { id: 1, createdAt: new Date(), updatedAt: new Date(), firstName: "John", lastName: "Doe", email: "john@example.com", preferences: { theme: "dark", notifications: true }};
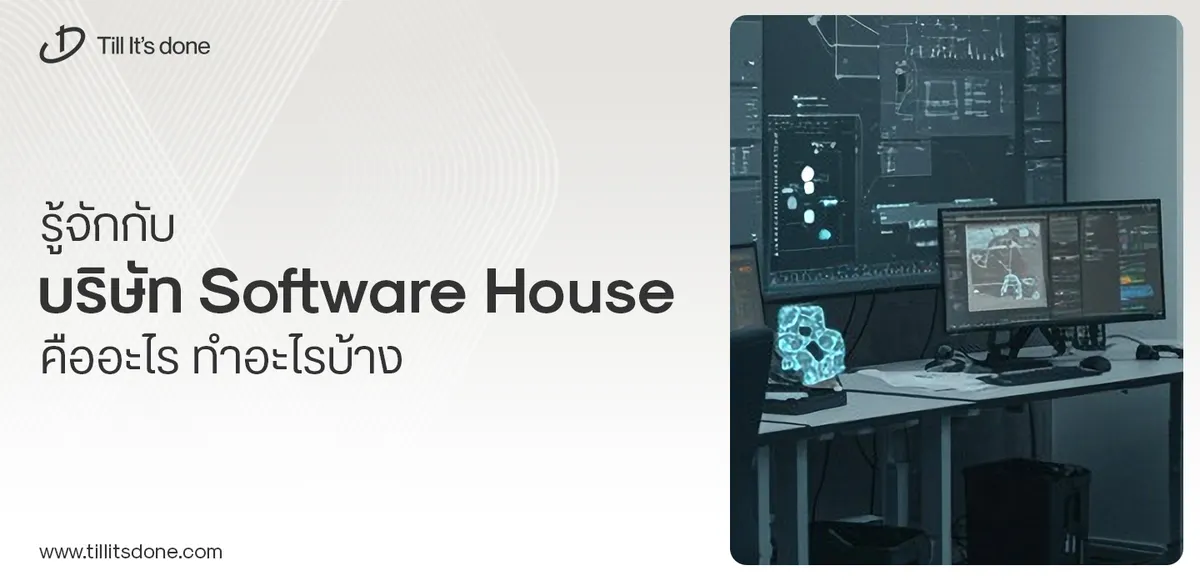
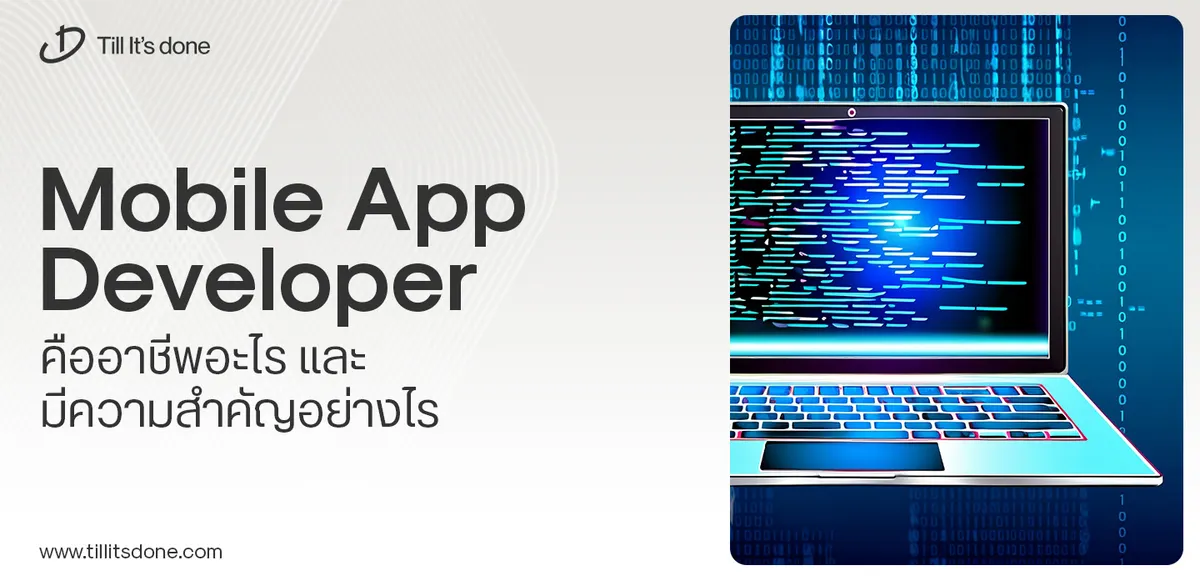
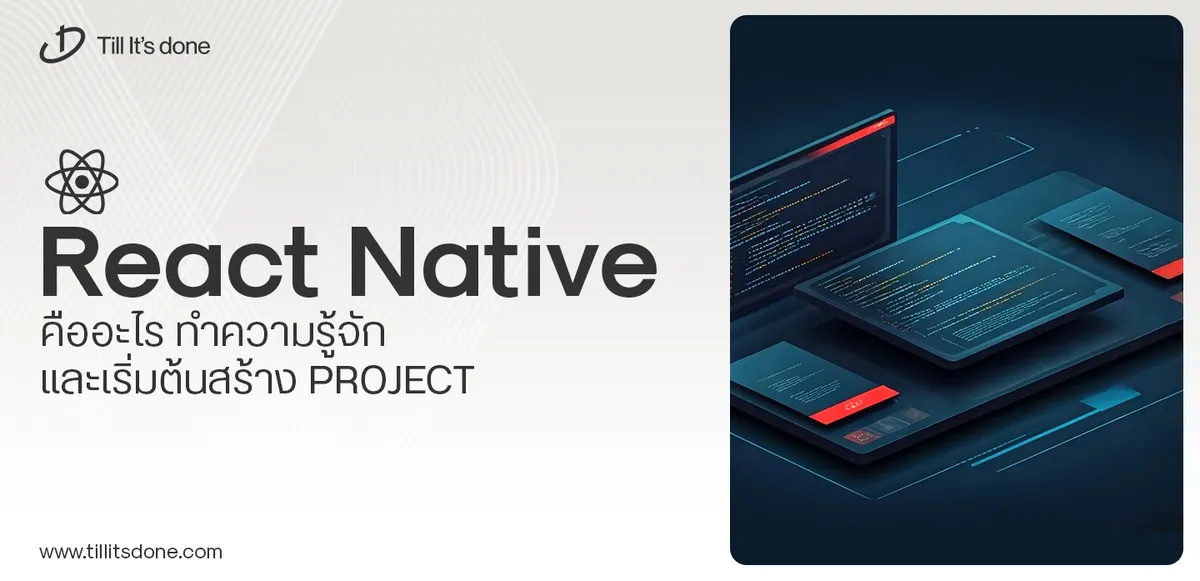
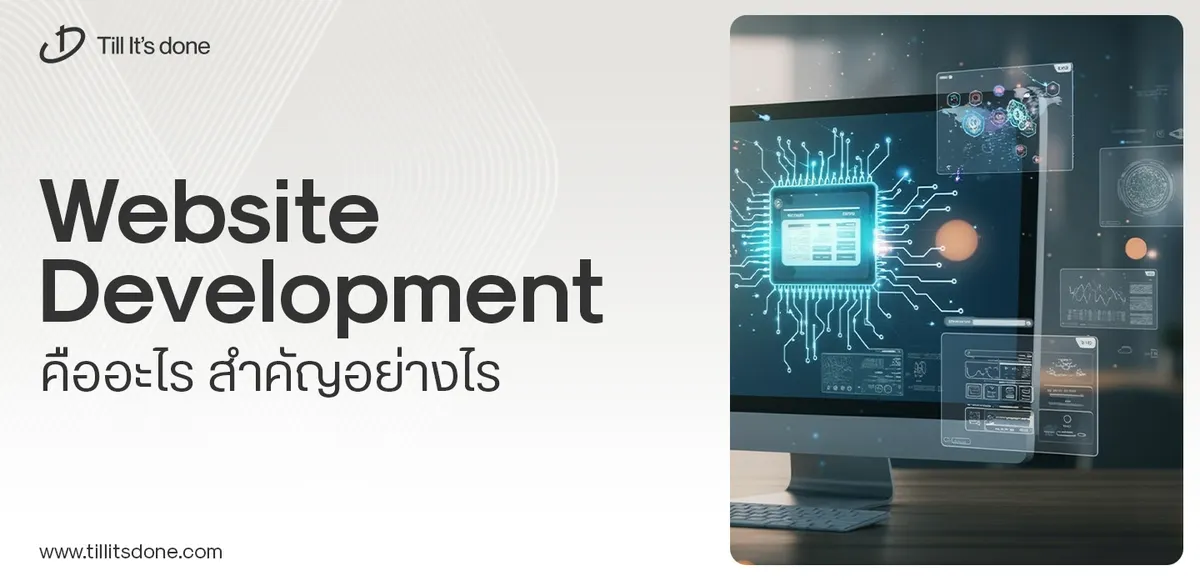
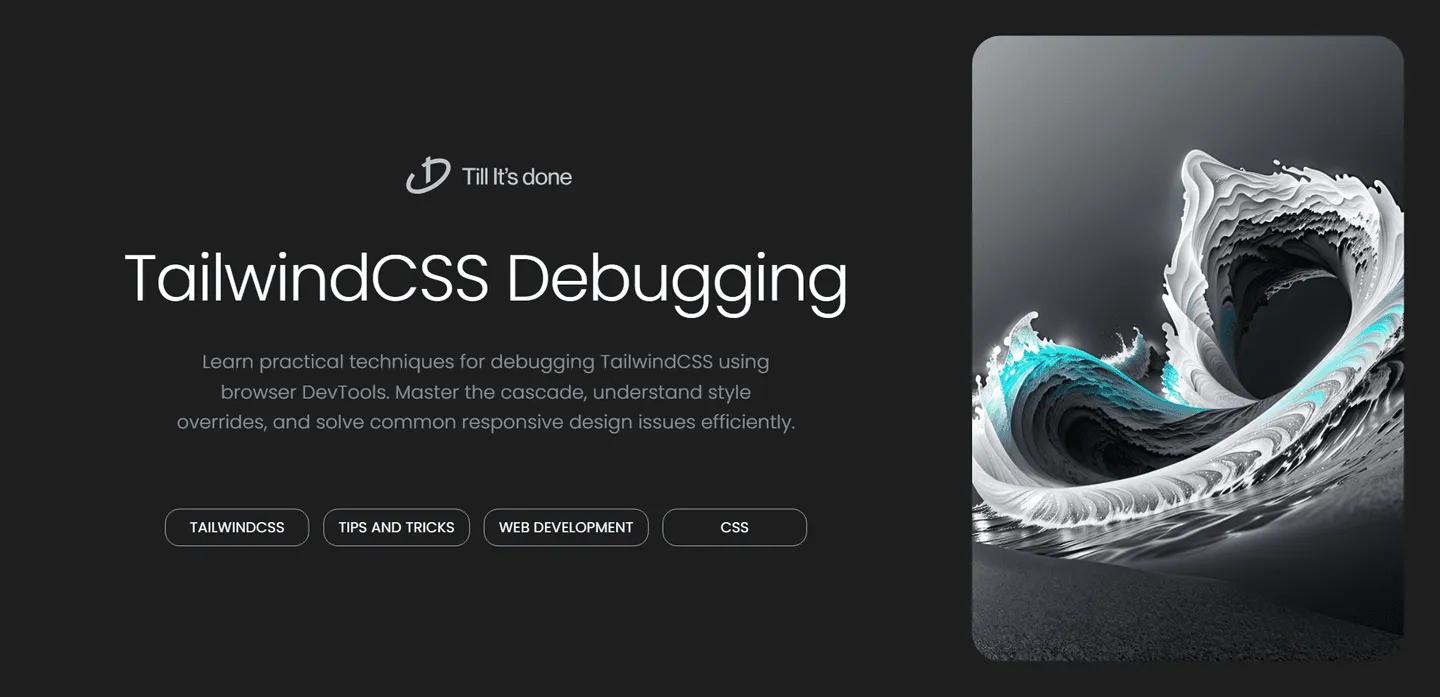
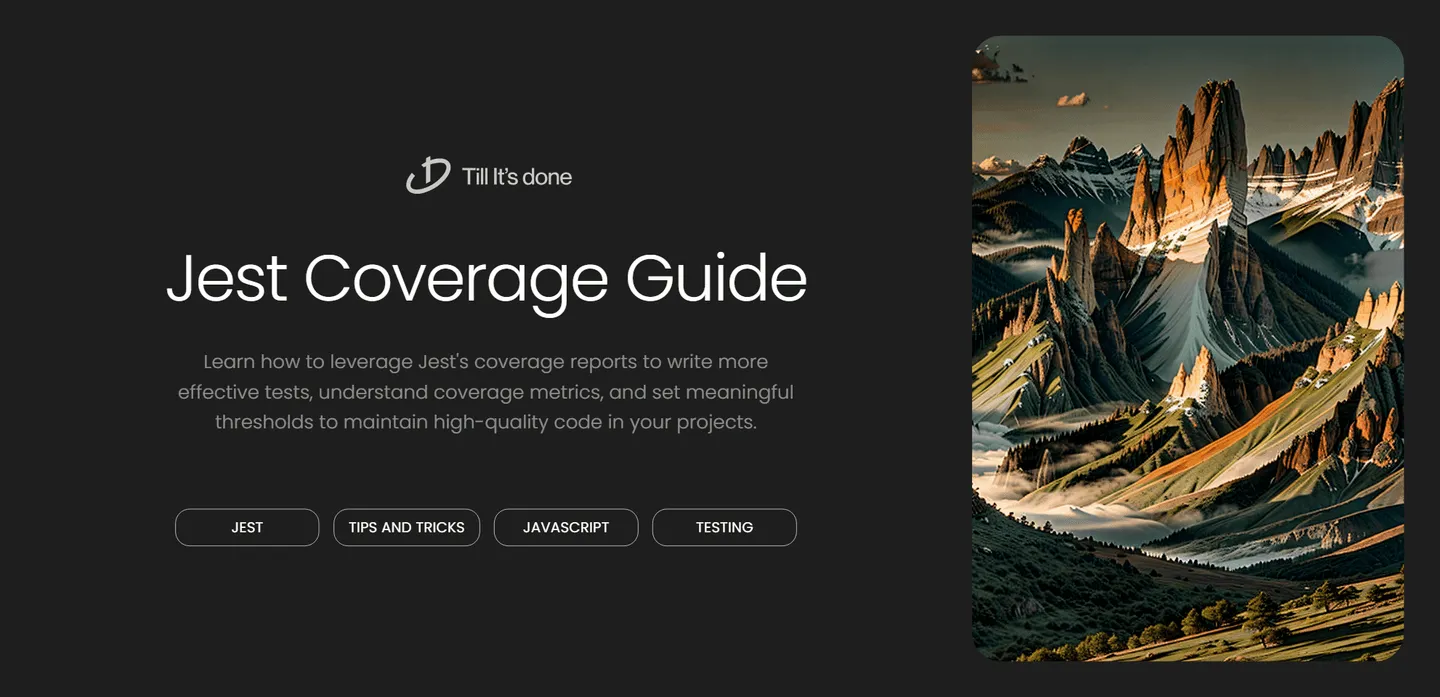
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.