- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Leveraging TypeScript Interfaces for Scale
Learn essential patterns and best practices for building maintainable, type-safe, and scalable systems.
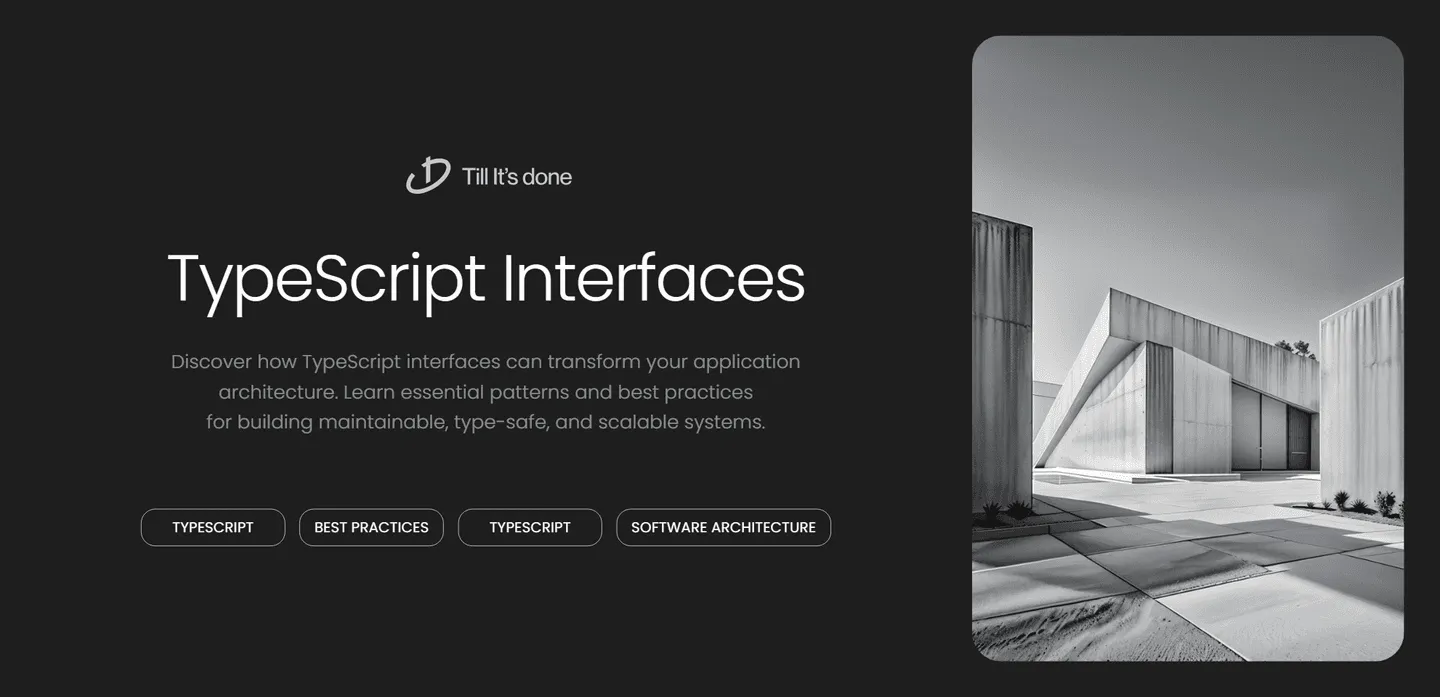
Leveraging TypeScript Interfaces for Scalable Applications
In the ever-evolving landscape of modern web development, building scalable applications has become more crucial than ever. TypeScript stands as a powerful ally in this journey, and at its heart lies one of its most valuable features: interfaces. Let’s dive into how we can harness the power of TypeScript interfaces to create more maintainable and scalable applications.
The Foundation: Understanding Interfaces
Think of interfaces as blueprints for your objects. They define the contract that your code must follow, ensuring consistency across your application. Unlike traditional JavaScript, where object shapes are implicit, TypeScript interfaces make these structures explicit and enforceable.
interface User { id: string; username: string; email: string; isActive: boolean; lastLogin?: Date;}
Building Blocks for Scalability
1. Interface Extension
One of the most powerful aspects of interfaces is their ability to extend other interfaces. This promotes code reuse and creates clear hierarchies in your data structures.
interface BaseEntity { id: string; createdAt: Date; updatedAt: Date;}
interface Product extends BaseEntity { name: string; price: number; category: string;}
2. Interface Composition
Rather than creating monolithic interfaces, break them down into smaller, focused pieces that you can compose together. This approach follows the Interface Segregation Principle from SOLID principles.
interface Timestampable { createdAt: Date; updatedAt: Date;}
interface Addressable { street: string; city: string; country: string;}
interface Customer extends Timestampable, Addressable { name: string; email: string;}
Best Practices for Interface Design
-
Keep Interfaces Focused: Each interface should have a single responsibility. If an interface grows too large, consider breaking it down into smaller, more manageable pieces.
-
Use Readonly When Appropriate: TypeScript’s readonly modifier can help prevent accidental mutations and make your code more predictable.
interface ConfigOptions { readonly apiKey: string; readonly endpoint: string; readonly timeout: number;}
- Leverage Generic Interfaces: Create flexible, reusable interfaces using generics to handle different types while maintaining type safety.
interface ApiResponse\<T\> { data: T; status: number; message: string; timestamp: Date;}
Real-World Implementation Strategies
Consider using interfaces for:
- API response types
- Component props in React/Vue applications
- State management structures
- Database models
- Configuration objects
By consistently using interfaces, you create a strong foundation for your application’s type system, making it easier to:
- Catch errors during development
- Implement new features confidently
- Onboard new team members effectively
- Refactor code with confidence
Advanced Patterns
Index Signatures
Use index signatures when you need flexible object structures while maintaining type safety:
interface DynamicConfig { [key: string]: string | number | boolean;}
Utility Types with Interfaces
Combine interfaces with TypeScript’s utility types for more powerful type definitions:
interface UserProfile { name: string; email: string; phone: string; address: string;}
type UpdateableUserProfile = Partial<UserProfile>;type ReadOnlyUser = Readonly<UserProfile>;
Conclusion
TypeScript interfaces are more than just type definitions – they’re a powerful tool for building scalable, maintainable applications. By following these patterns and best practices, you can create robust applications that can grow and evolve with your needs.
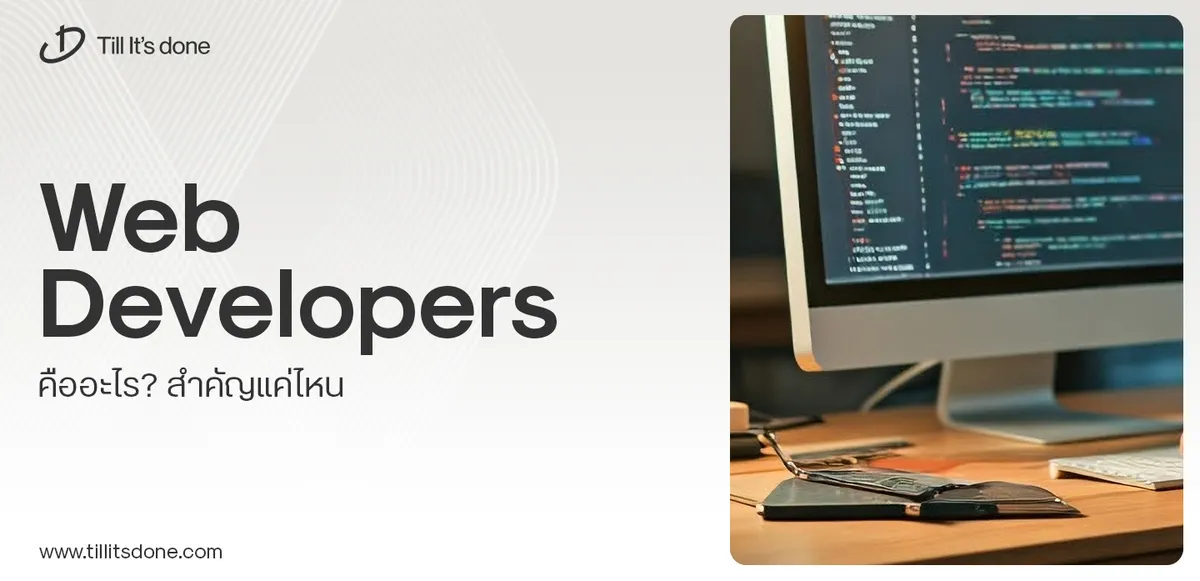
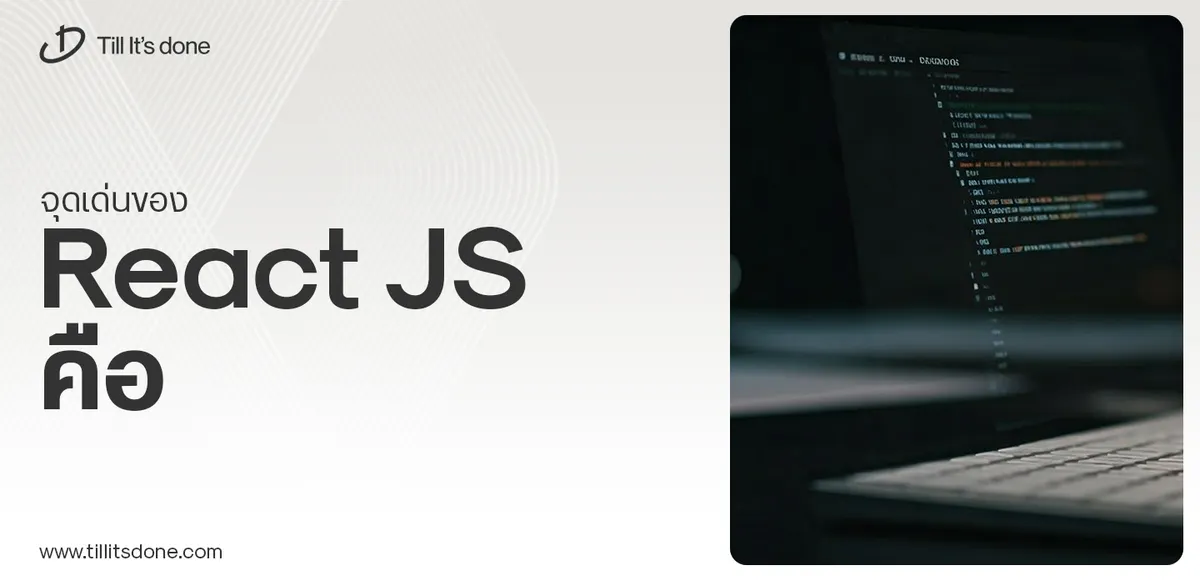
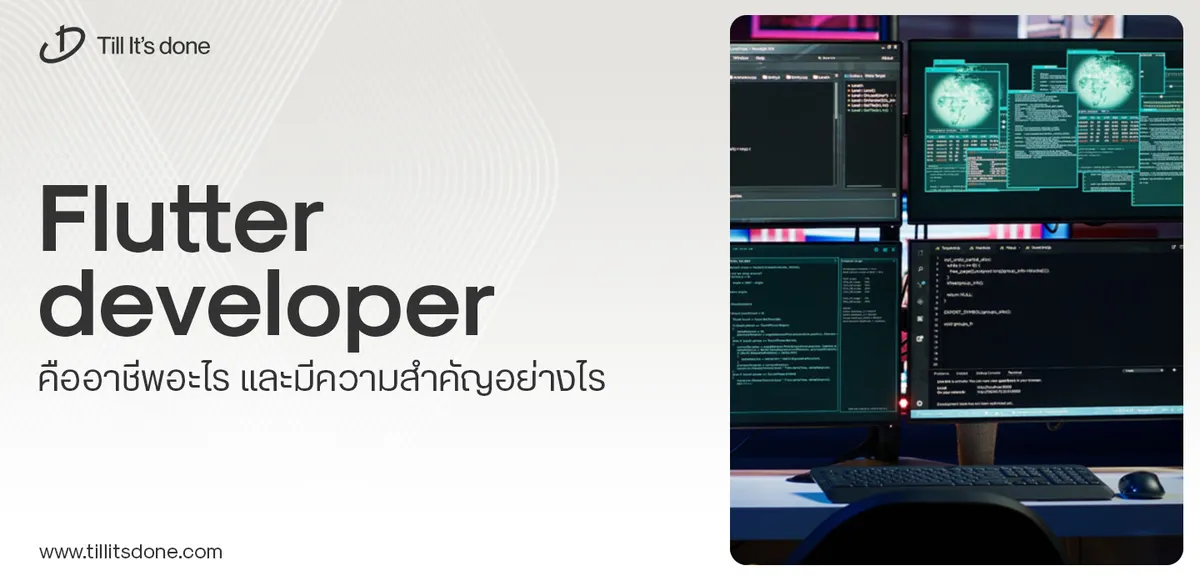
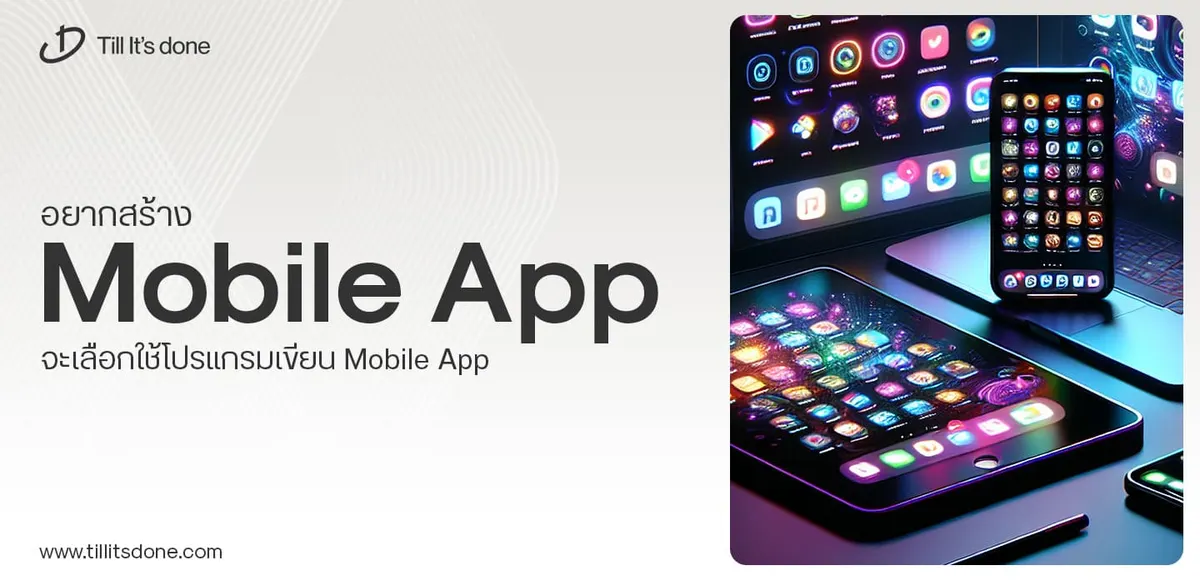
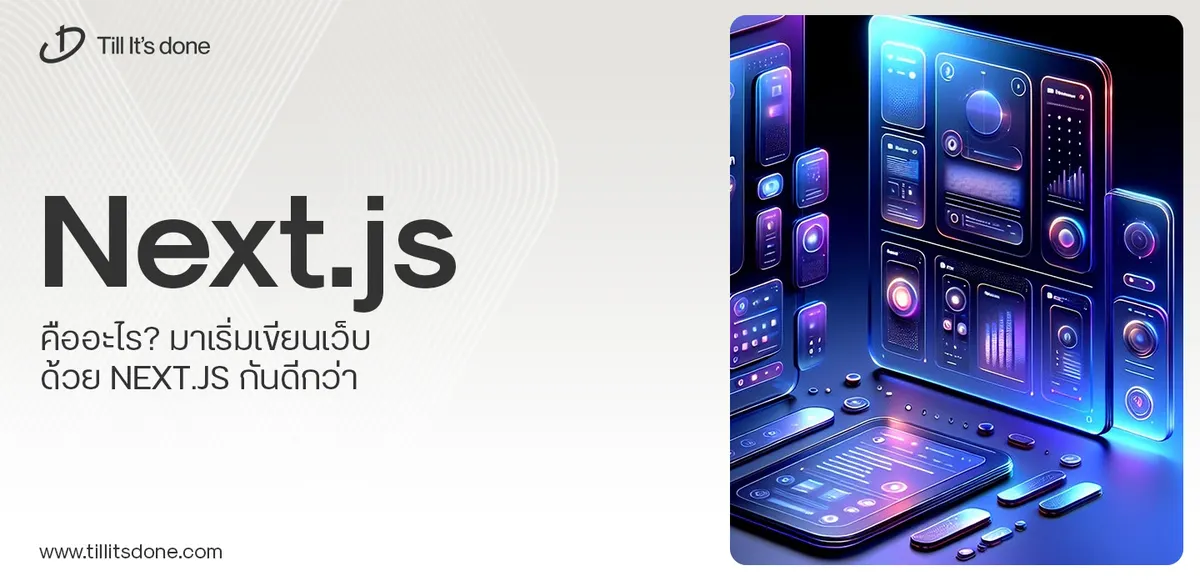
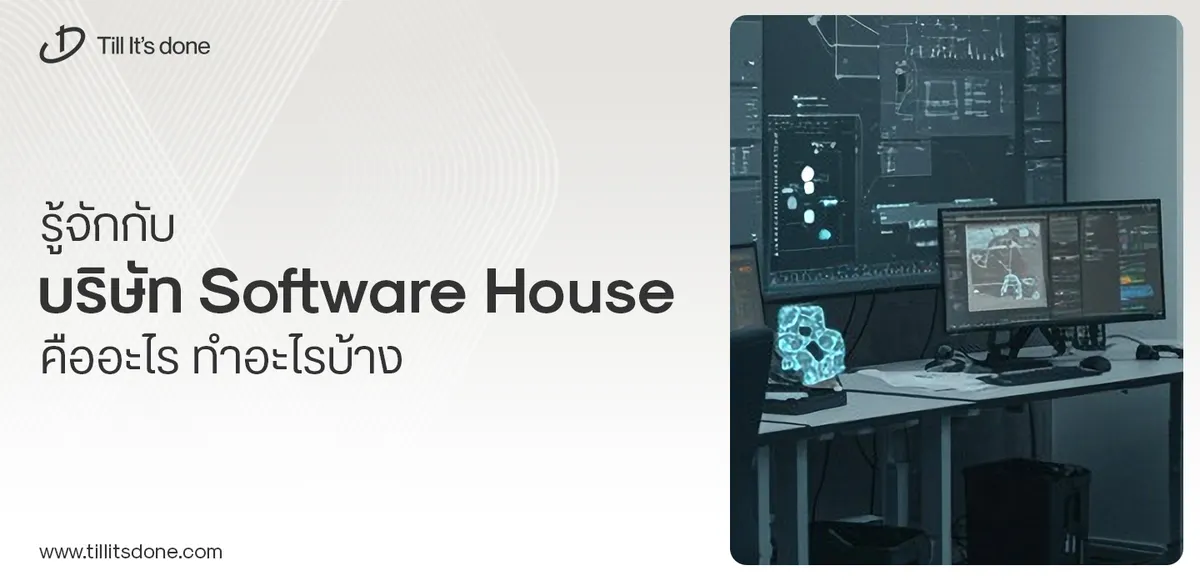
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.