- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Advanced Patterns with TypeScript's infer Keyword
Learn how to write cleaner, type-safe code with real-world applications.
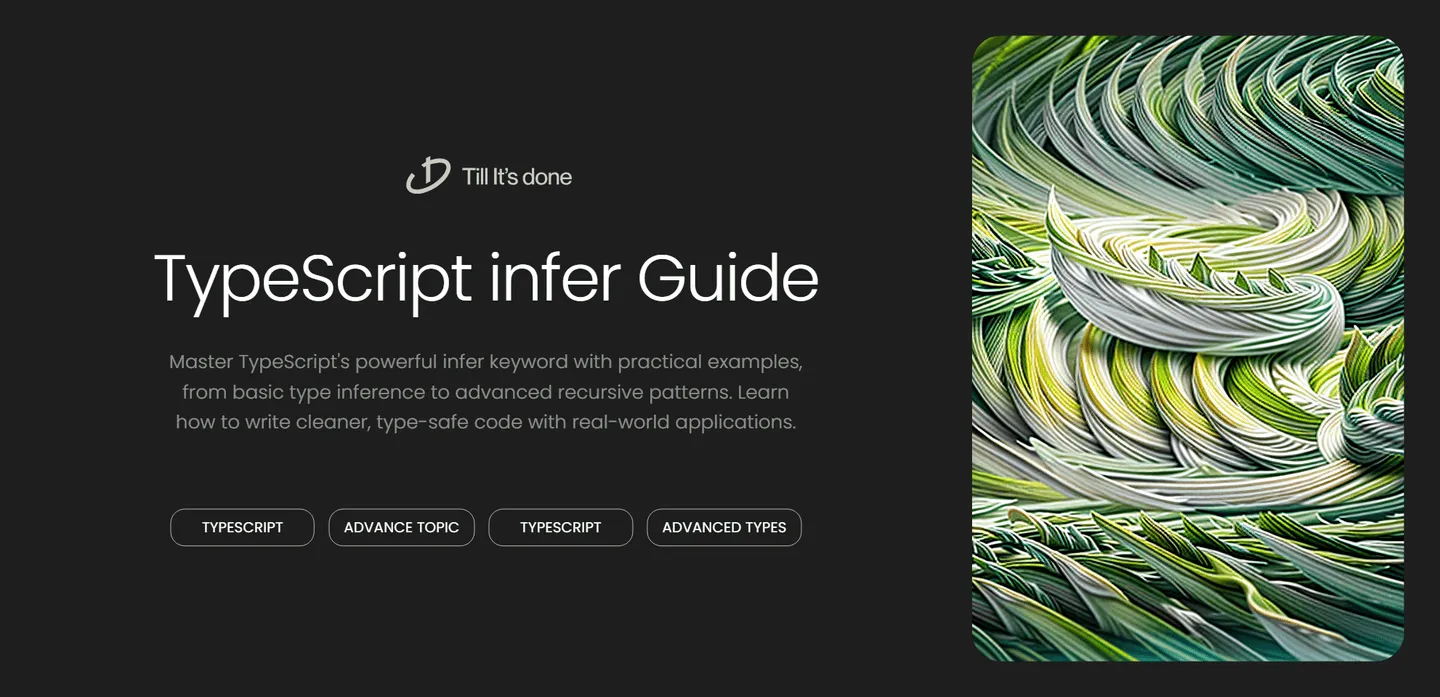
Implementing Advanced Patterns with TypeScript’s infer Keyword
Have you ever found yourself trying to extract types from complex TypeScript structures and wished there was a more elegant way? Enter the infer
keyword – one of TypeScript’s most powerful yet often misunderstood features. Today, we’ll dive deep into advanced patterns that leverage infer
to write more expressive and maintainable type-level code.
Understanding the Magic of infer
At its core, infer
is like a type-level variable declaration. It allows us to capture and reference types within conditional types. Think of it as pattern matching for types – when TypeScript sees a match, it extracts and stores the matched type for later use.
Let’s start with a practical example:
type UnwrapPromise\<T\> = T extends Promise<infer U> ? U : T;
// Usagetype Result1 = UnwrapPromise<Promise<string>>; // stringtype Result2 = UnwrapPromise<number>; // number
Advanced Inference Patterns
1. Function Parameter Inference
One of the most powerful applications of infer
is extracting parameter types from functions:
type FirstParameter\<T\> = T extends (first: infer First, ...args: any[]) => any ? First : never;
// Real-world exampletype EventHandler = (event: MouseEvent, context: any) => void;type EventType = FirstParameter<EventHandler>; // MouseEvent
2. Recursive Type Inference
We can combine infer
with recursive types to handle nested structures:
type UnwrapArray\<T\> = T extends Array<infer U> ? UnwrapArray<U> : T;
// Usagetype NestedArray = number[][][];type FlatType = UnwrapArray<NestedArray>; // number
3. String Pattern Matching
infer
isn’t just for complex types – it’s also fantastic for string manipulation:
type ExtractDomain<T extends string> = T extends `https://${infer Domain}/${string}` ? Domain : never;
// Usagetype Website = ExtractDomain<"https://typescript-rocks.com/blog">; // "typescript-rocks.com"
Best Practices and Tips
- Always consider readability first. Just because you can use
infer
doesn’t always mean you should. - Break down complex inference patterns into smaller, reusable types.
- Use meaningful names for inferred type parameters – future you will thank present you.
- Document your complex type inference patterns with examples.
Real-World Applications
The true power of infer
shines when building robust type utilities:
type PropType<T, Path extends string> = Path extends keyof T ? T[Path] : Path extends `${infer Key}.${infer Rest}` ? Key extends keyof T ? PropType<T[Key], Rest> : never : never;
// Usage with nested objectsinterface User { profile: { name: string; settings: { theme: 'light' | 'dark'; }; };}
type Theme = PropType<User, 'profile.settings.theme'>; // 'light' | 'dark'
The infer
keyword opens up a world of possibilities in TypeScript’s type system. While it might seem daunting at first, mastering these patterns will elevate your TypeScript game to new heights. Remember, the goal isn’t to write the most clever type definitions, but to create maintainable and understandable code that helps catch errors before they happen.
Keep experimenting with these patterns, and you’ll discover even more creative ways to leverage TypeScript’s type system to your advantage. Happy coding!
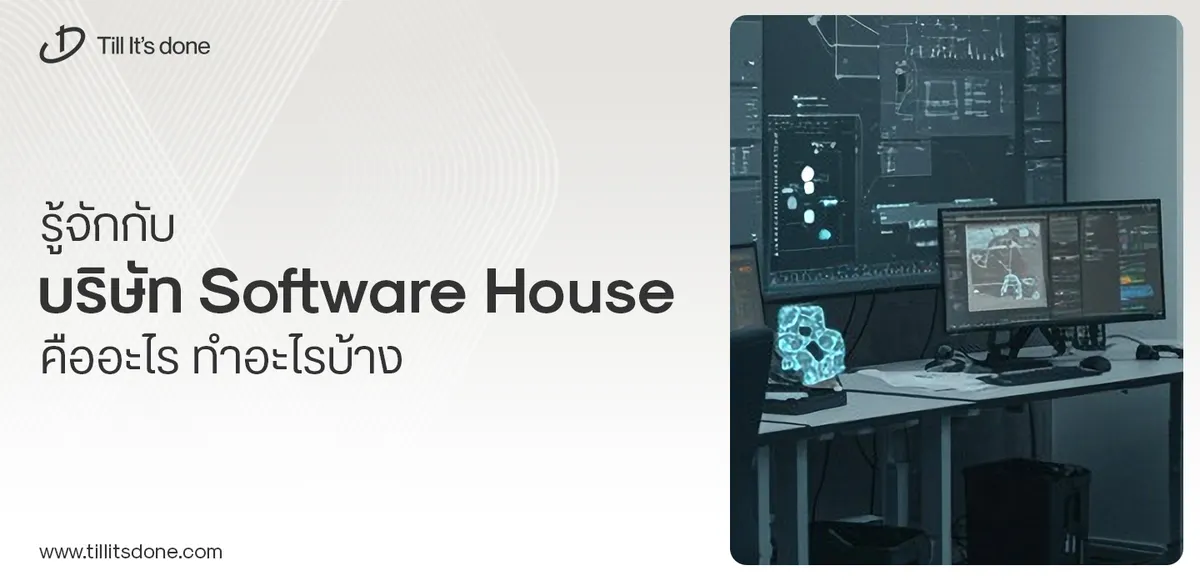
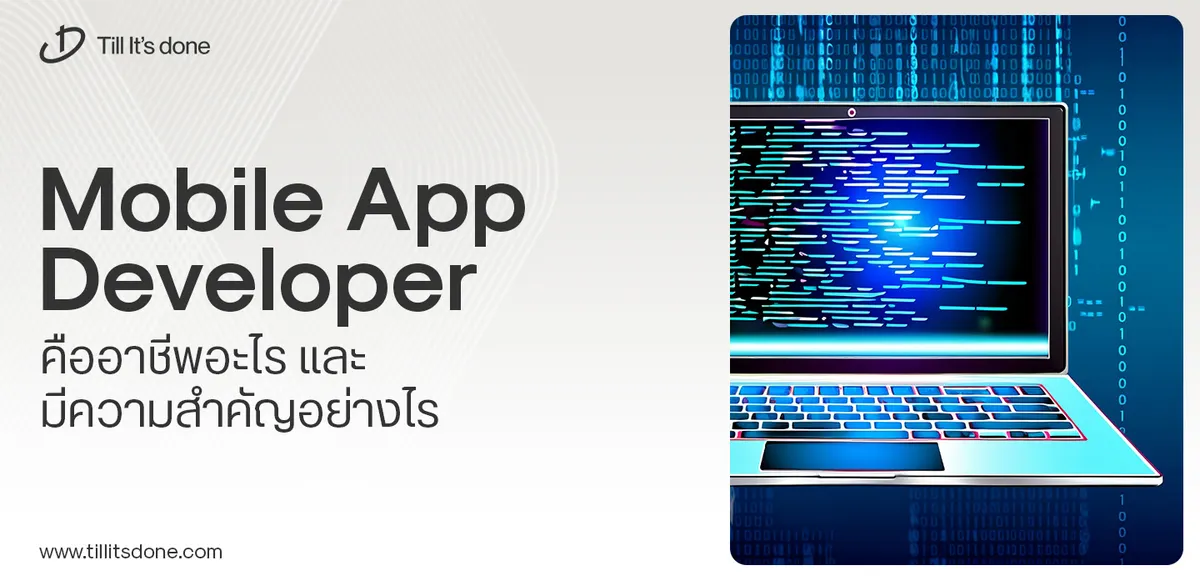
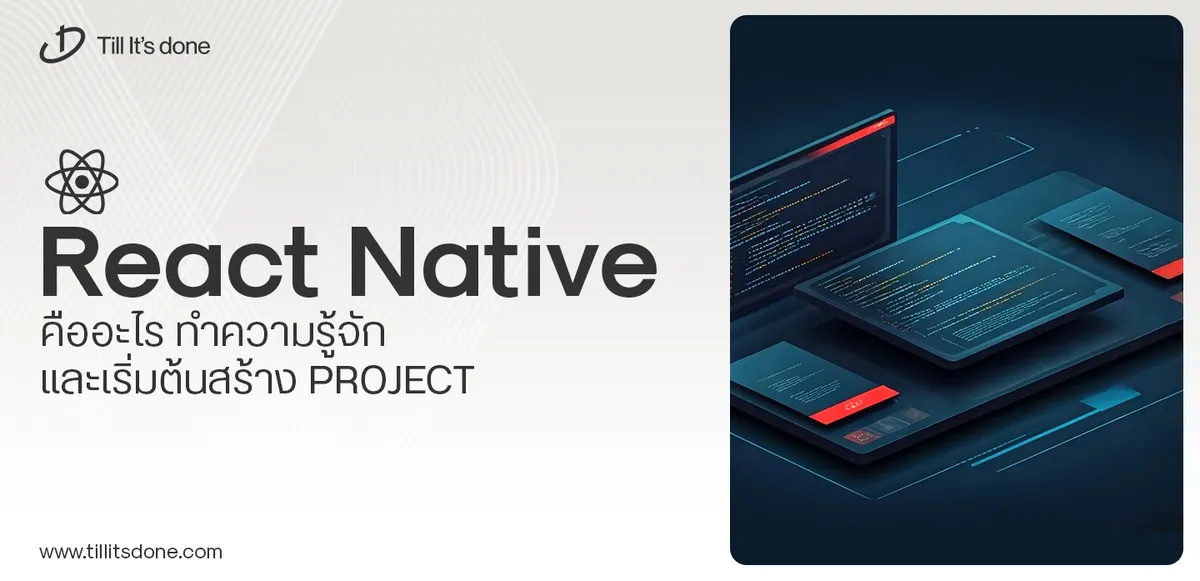
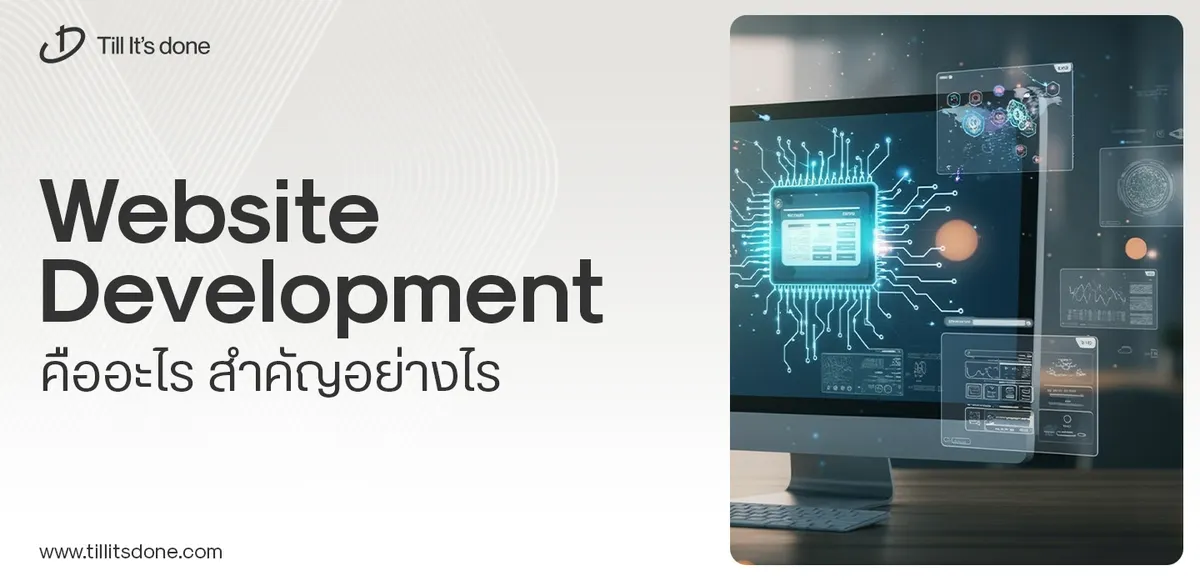
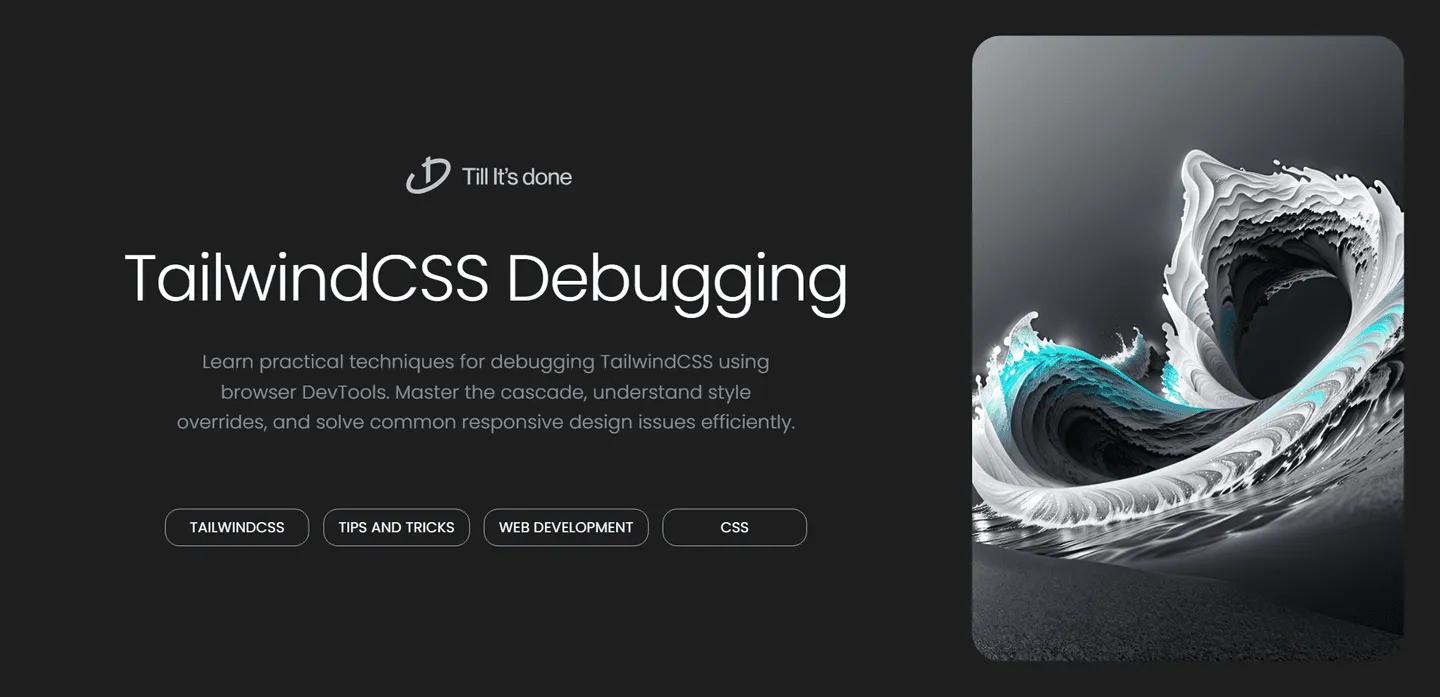
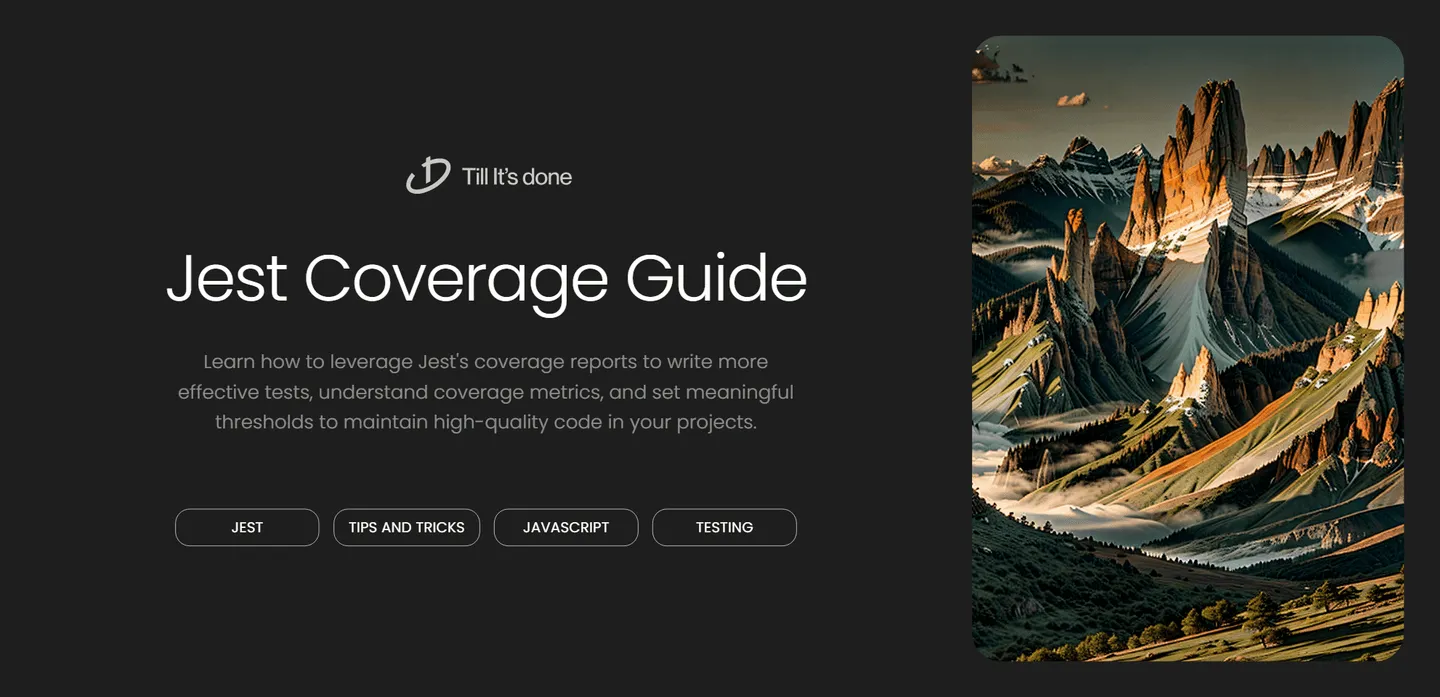
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.