- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Understanding TypeScript Function Types
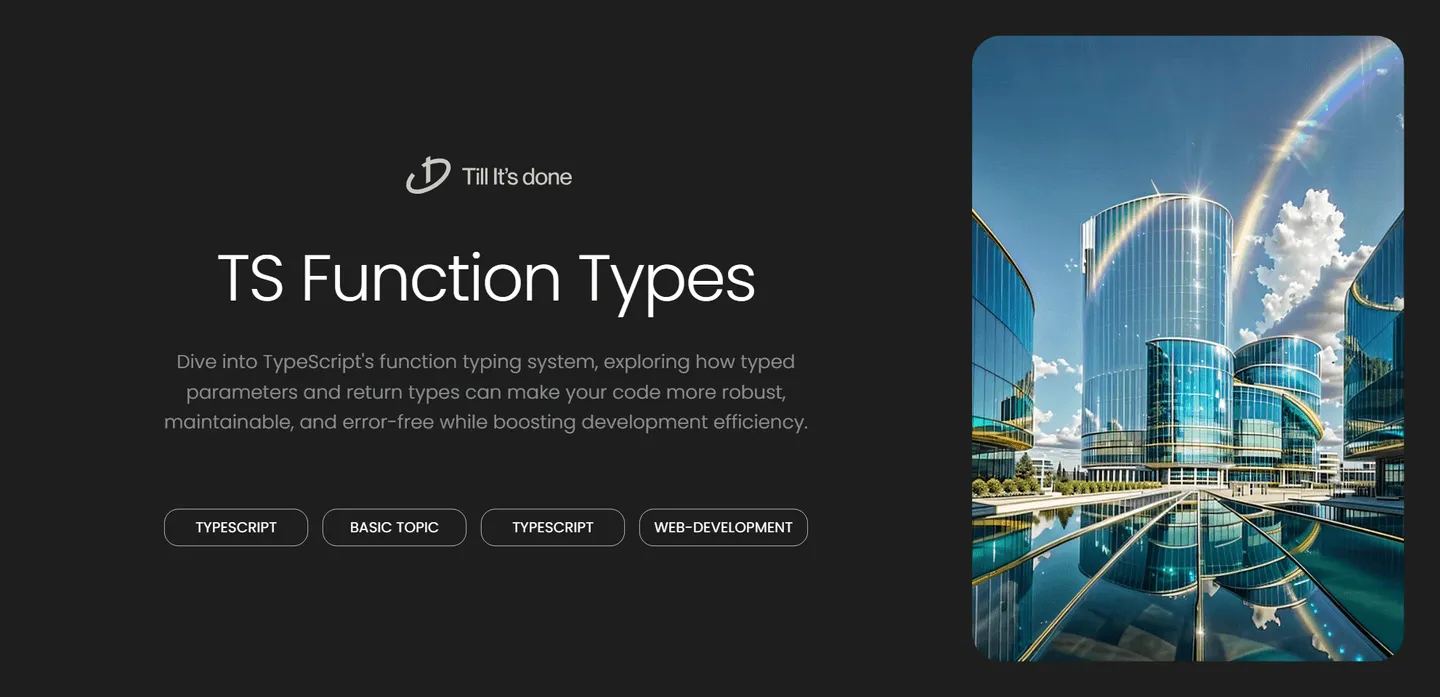
Exploring TypeScript Functions: Typed Parameters and Return Types
Hey fellow developers! Today, let’s dive into one of TypeScript’s most powerful features - functions with typed parameters and return types. If you’re coming from JavaScript, this will be a game-changer in how you write and maintain your code.
Understanding the Basics
Think of TypeScript types as guardrails for your functions. They help prevent bugs before they happen and make your code more predictable. Let’s start with a simple example:
function greetUser(name: string): string { return `Hello, ${name}!`;}
Why Use Typed Parameters?
In the real world, functions often handle complex data. Typed parameters act like a contract, ensuring you’re passing the right data every time. Here’s a practical example:
interface UserProfile { id: number; email: string; age: number;}
function validateUser(profile: UserProfile): boolean { return profile.age >= 18 && profile.email.includes('@');}
Return Types Matter
Return types aren’t just extra syntax - they’re your first line of defense against unexpected outputs. They make your functions more reliable and self-documenting:
function calculateDiscount(price: number, percentage: number): number { const discount = price * (percentage / 100); return Number(discount.toFixed(2));}
Advanced Function Types
TypeScript really shines when dealing with more complex scenarios. Let’s look at function overloads and optional parameters:
function processData(data: string): string;function processData(data: number): number;function processData(data: string | number): string | number { if (typeof data === 'string') { return data.toUpperCase(); } return data * 2;}
Best Practices
Remember these key points:
- Always specify return types explicitly
- Use interfaces for complex parameter types
- Consider making parameters optional when appropriate
- Leverage union types for flexibility
- Use type inference wisely
Wrapping Up
TypeScript’s type system for functions might seem like extra work at first, but it’s an investment that pays off in code quality and maintainability. Start small, and gradually incorporate more advanced typing as you get comfortable.
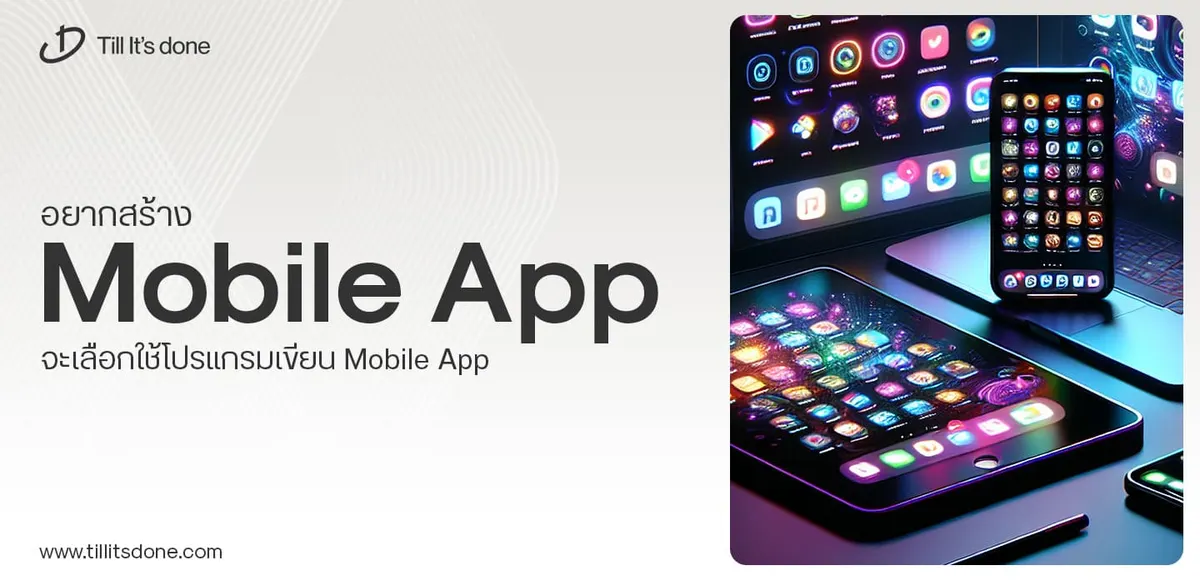
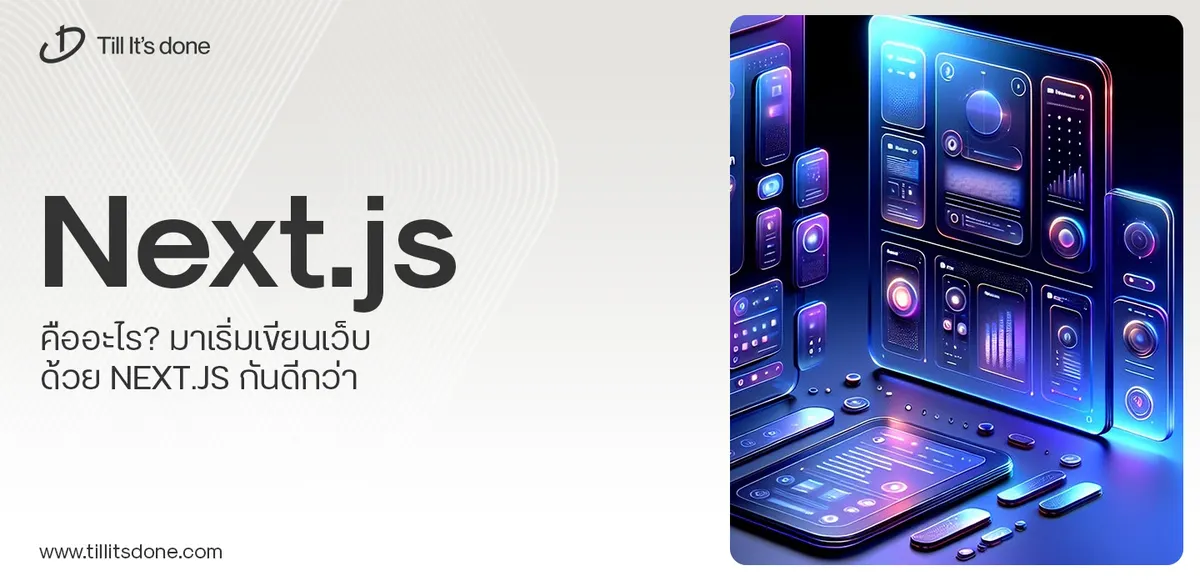
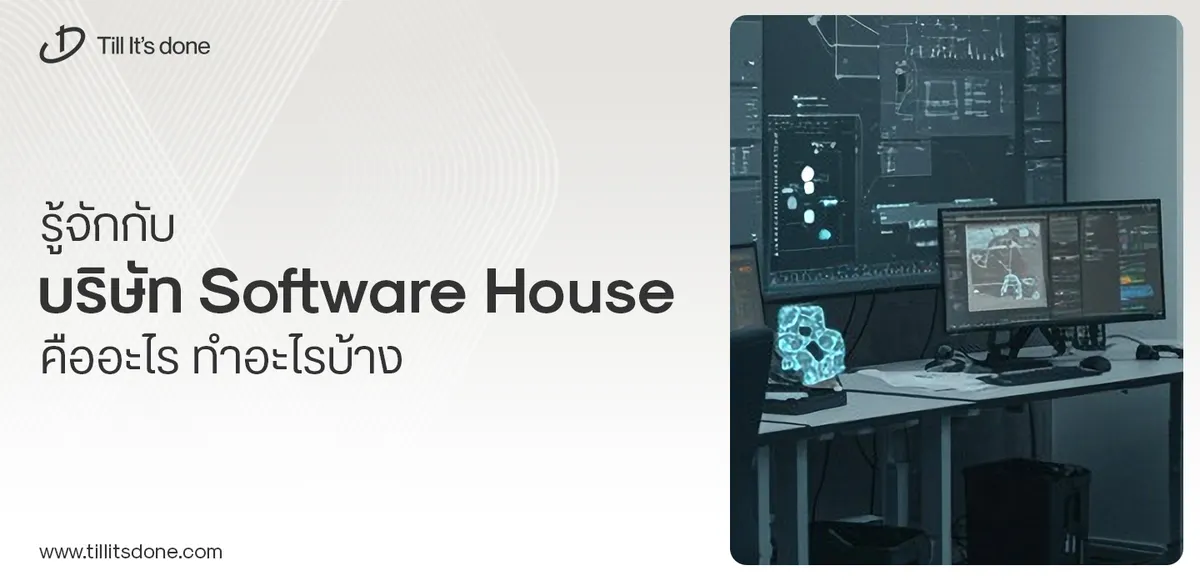
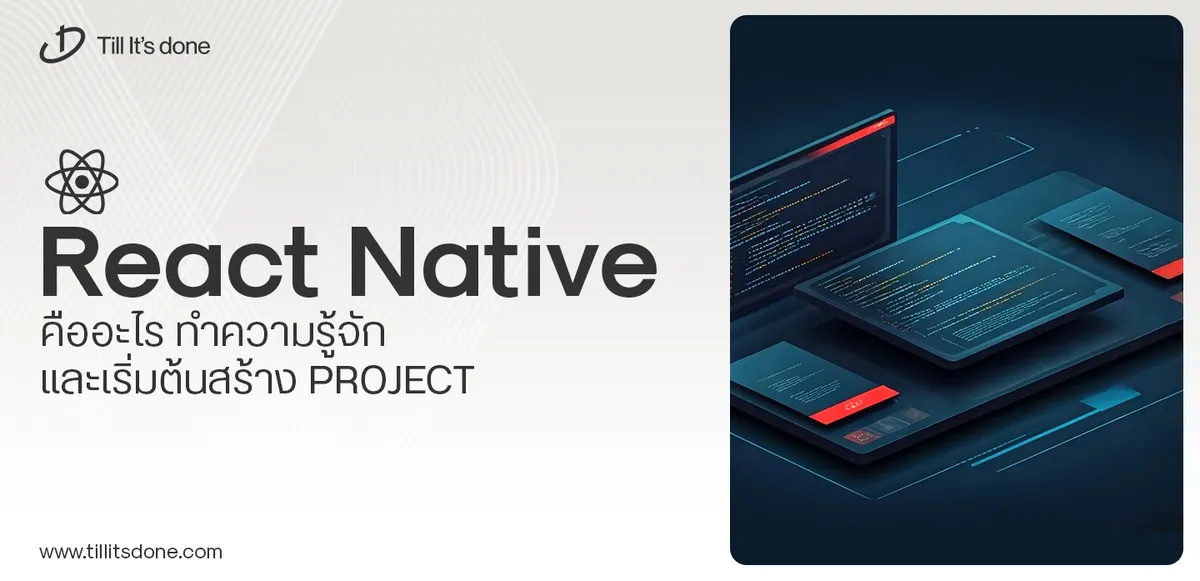
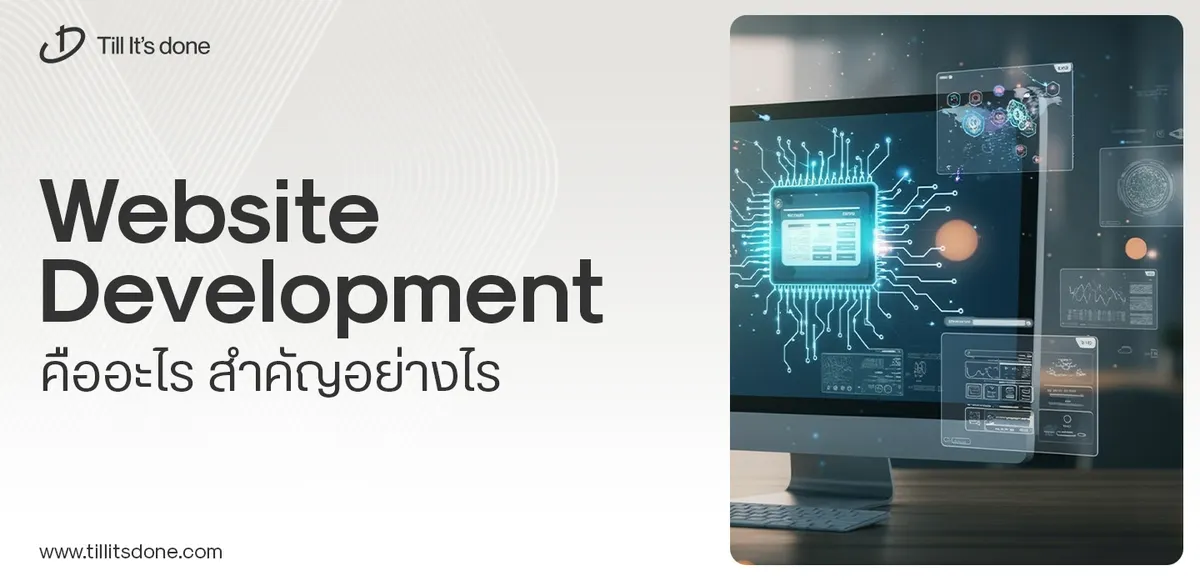
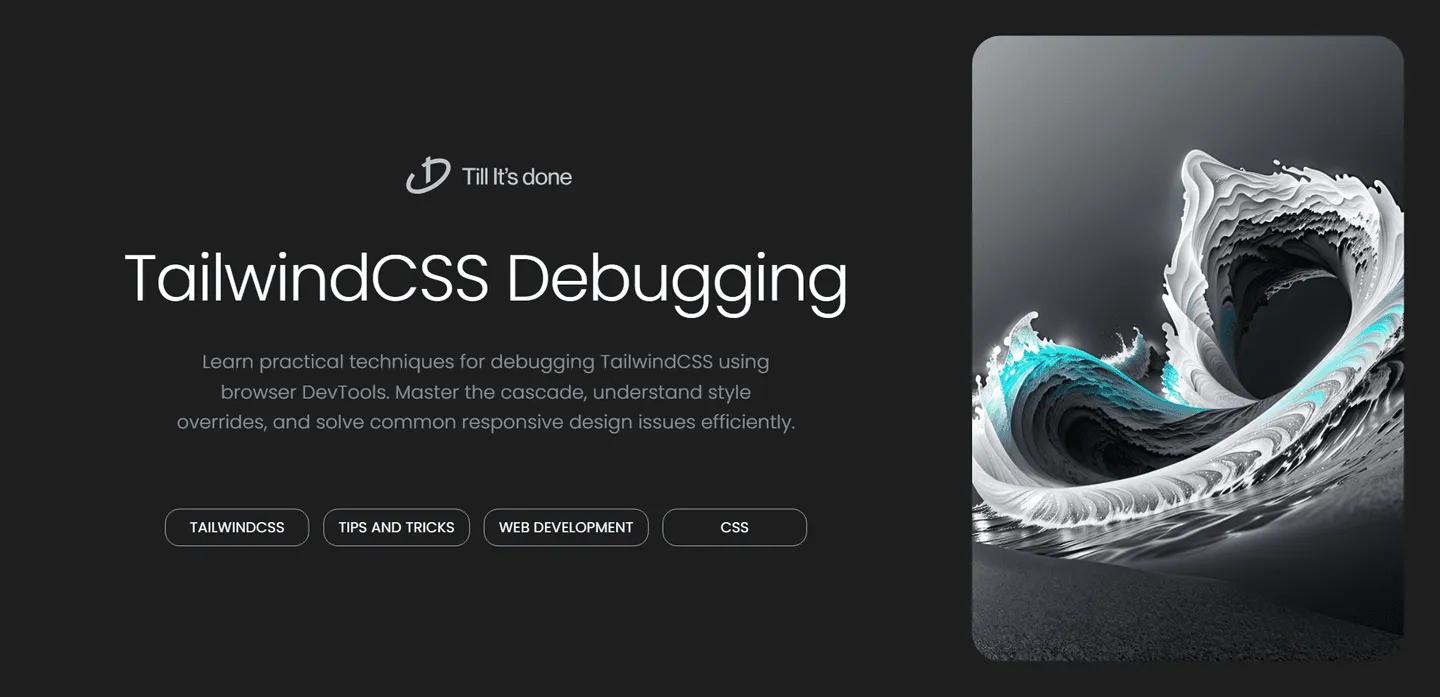
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.