- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
TypeScript Decorators: A Deep Dive into Advanced TS
Learn about class, method, property, parameter, and accessor decorators, with practical examples and best practices.
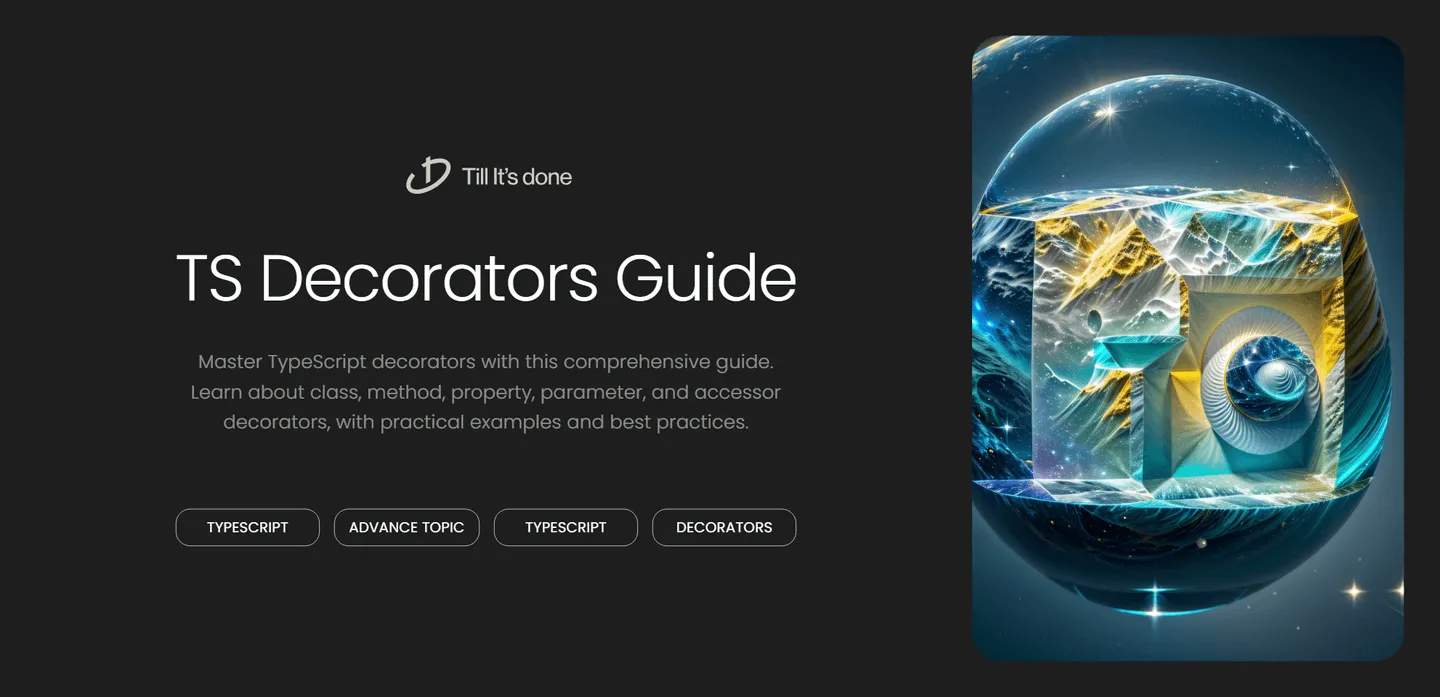
TypeScript Decorators: Deep Dive
Ever found yourself writing repetitive code patterns in TypeScript and wished there was a more elegant way to handle them? Enter TypeScript decorators – a powerful feature that allows you to add metadata, modify behavior, or transform your code in a clean, declarative way.
Understanding the Basics
Decorators are special kinds of declarations that can be attached to class declarations, methods, properties, or parameters. Think of them as “wrappers” that can modify or enhance the behavior of your code. They’re prefixed with the @
symbol and can be used to implement cross-cutting concerns like logging, validation, or dependency injection.
Types of Decorators
Let’s explore the five main types of decorators in TypeScript:
1. Class Decorators
Class decorators are applied to the constructor of the class and can be used to observe, modify, or replace class definitions. They’re perfect for implementing patterns like dependency injection or adding metadata to your classes.
function Logger(logString: string) { return function(constructor: Function) { console.log(logString); console.log(constructor); };}
@Logger('Logging - Person')class Person { name = 'Max';
constructor() { console.log('Creating person object...'); }}
2. Method Decorators
Method decorators allow you to observe, modify, or replace a method definition. They’re commonly used for logging, measuring performance, or adding validation logic.
function Log(target: any, propertyName: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value;
descriptor.value = function(...args: any[]) { console.log(`Calling ${propertyName} with args: ${args}`); const result = originalMethod.apply(this, args); console.log(`Result: ${result}`); return result; };}
class Calculator { @Log multiply(a: number, b: number) { return a * b; }}
3. Property Decorators
Property decorators let you observe or modify property definitions. They’re useful for implementing validation rules or transforming property values.
function ValidateLength(min: number, max: number) { return function(target: any, propertyName: string) { let value: string;
const descriptor: PropertyDescriptor = { get() { return value; }, set(newValue: string) { if (newValue.length < min || newValue.length > max) { throw new Error(`${propertyName} must be between ${min} and ${max} characters`); } value = newValue; } };
Object.defineProperty(target, propertyName, descriptor); };}
class User { @ValidateLength(3, 10) name: string;}
4. Parameter Decorators
Parameter decorators are applied to the parameters of a method or constructor. They’re perfect for dependency injection or parameter validation scenarios.
5. Accessor Decorators
These decorators are specifically for getters and setters. They can be used to modify how properties are accessed or to add validation logic.
Best Practices and Tips
- Keep decorators focused and single-purpose
- Use decorator factories when you need configuration
- Consider performance implications
- Document your decorators thoroughly
- Test decorator behavior independently
Real-world Applications
Decorators shine in many practical scenarios:
- API route handling in frameworks like NestJS
- Validation and type checking
- Dependency injection
- Logging and monitoring
- Caching implementations
- Authentication and authorization
Conclusion
TypeScript decorators are more than just syntactic sugar – they’re powerful tools that can make your code more maintainable, readable, and efficient. While they might seem complex at first, understanding and implementing them can significantly improve your TypeScript applications.
Remember to use them judiciously and always consider whether a decorator is the best solution for your specific use case. Happy coding!
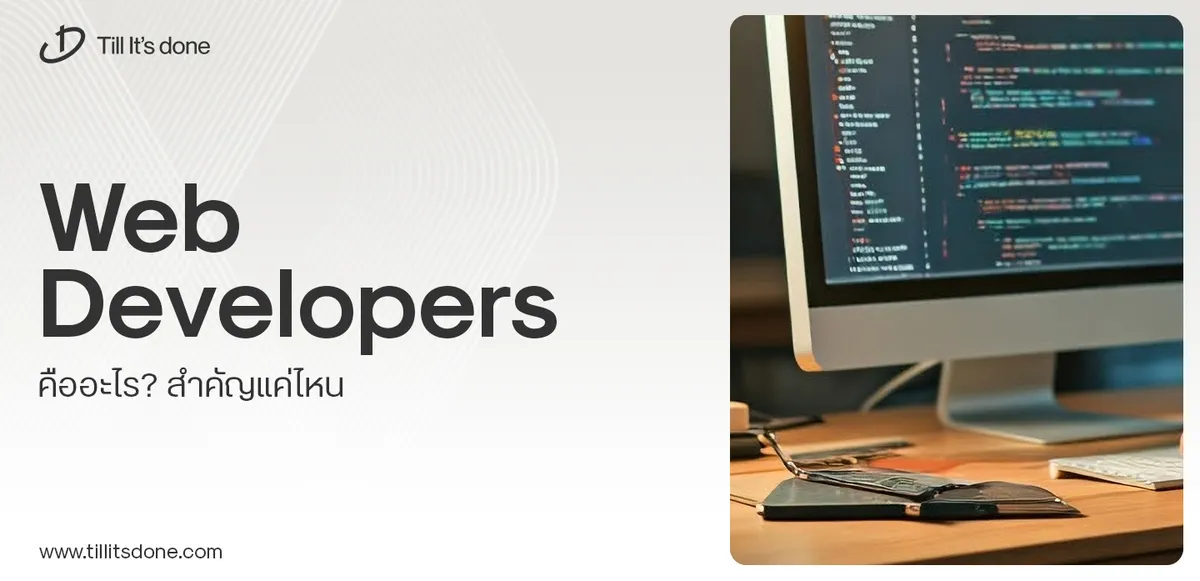
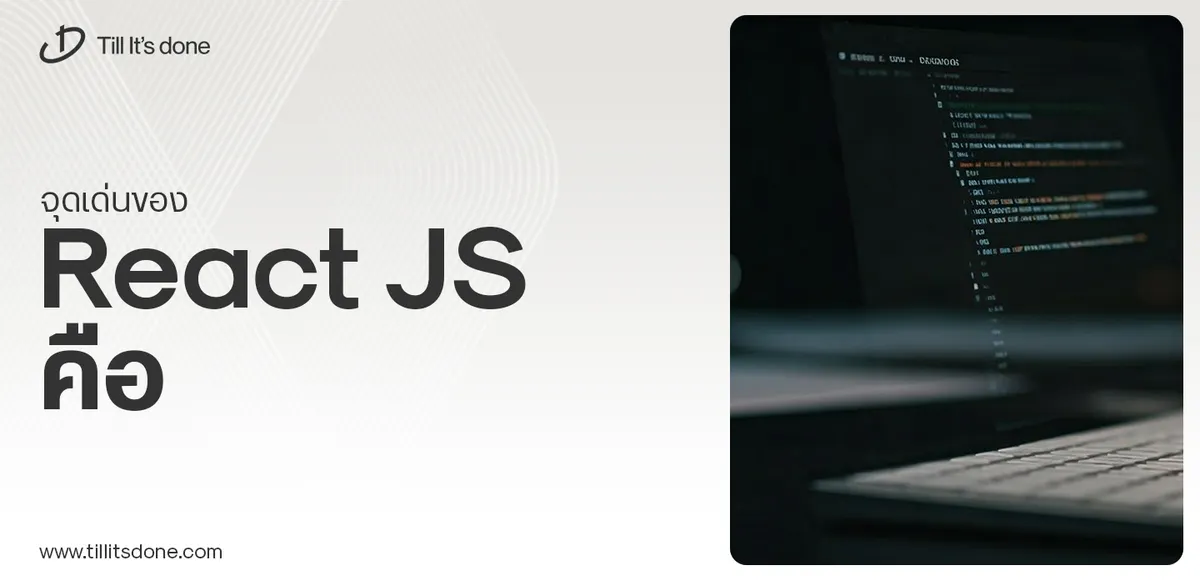
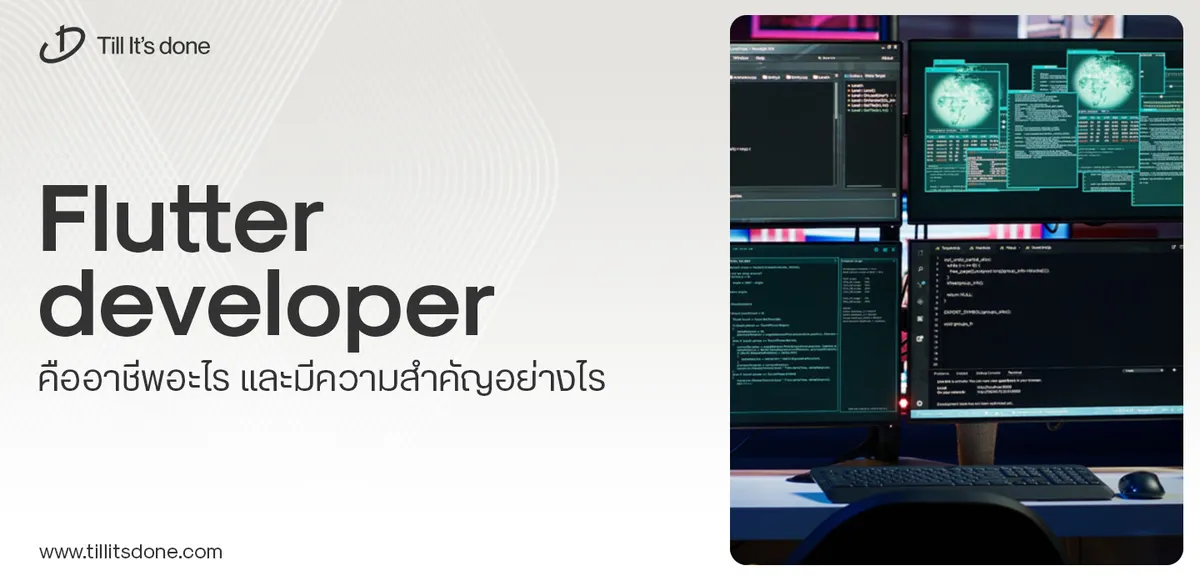
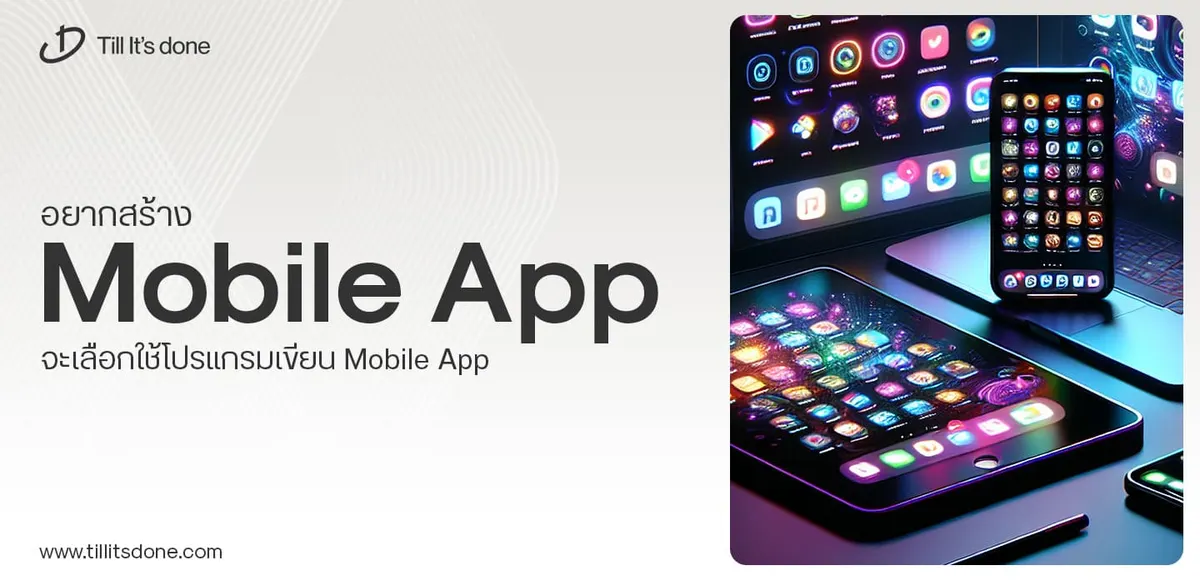
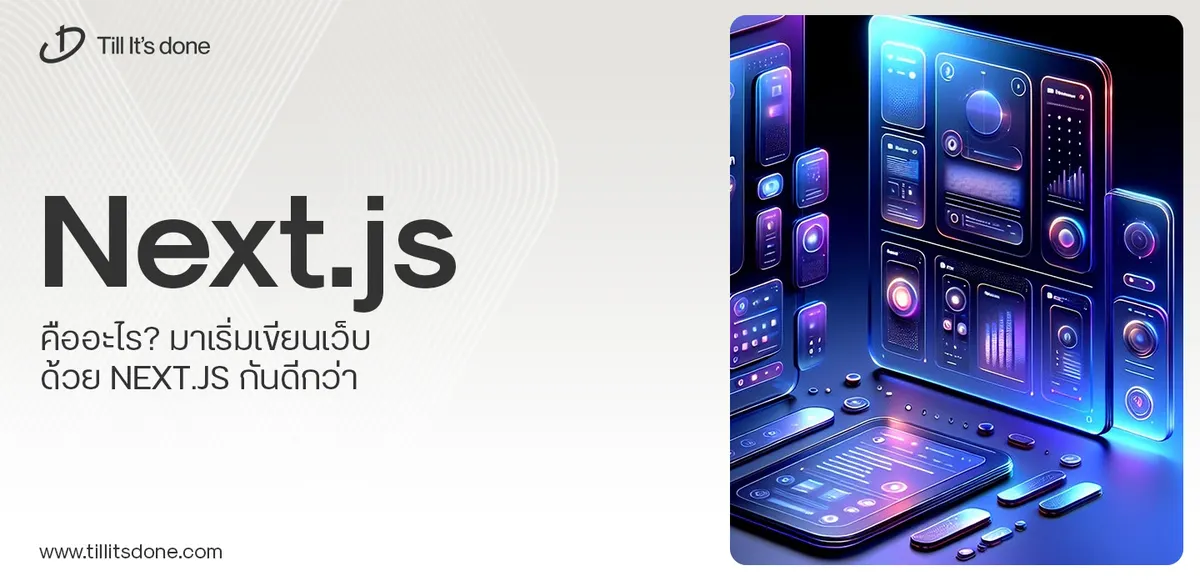
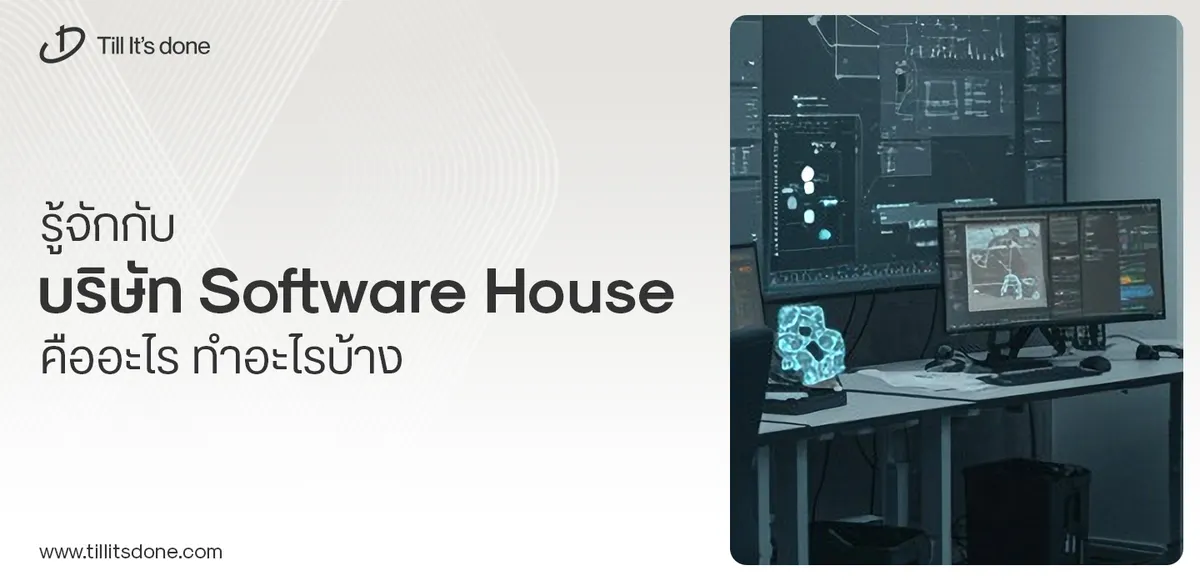
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.