- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
TypeScript Decorators: Tips for Cleaner Code
Learn best practices, common use cases, and performance optimization techniques for better development.

TypeScript Decorators: Tips for Cleaner Code
As TypeScript continues to evolve, decorators have emerged as a powerful feature for writing more elegant and maintainable code. Whether you’re building a complex enterprise application or a simple side project, understanding decorators can significantly improve your development experience.
What Are TypeScript Decorators?
Think of decorators as special wrappers that can modify your classes, methods, properties, or parameters. Like adding sprinkles to a cupcake, decorators add extra functionality to your code without changing its core structure.
Common Use Cases
Method Decorators
One of the most practical applications of decorators is logging method execution. Here’s a simple example:
function log(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value;
descriptor.value = function(...args: any[]) { console.log(`Calling ${propertyKey} with arguments: ${args}`); const result = originalMethod.apply(this, args); console.log(`Method ${propertyKey} returned: ${result}`); return result; };
return descriptor;}
class Calculator { @log multiply(a: number, b: number) { return a * b; }}
Property Decorators
Property decorators are fantastic for implementing validation rules:
function validateEmail(target: any, propertyKey: string) { let value: string;
const getter = function() { return value; };
const setter = function(newVal: string) { if (!newVal.includes('@')) { throw new Error('Invalid email format'); } value = newVal; };
Object.defineProperty(target, propertyKey, { get: getter, set: setter });}
class User { @validateEmail email: string;}
Best Practices
- Keep decorators focused and single-purpose
- Use meaningful names that clearly indicate their function
- Consider composition when combining multiple decorators
- Document your decorators thoroughly
- Handle errors gracefully within decorators
Performance Considerations
While decorators are powerful, they do add a layer of complexity to your code execution. Here are some tips to keep your decorated code performant:
- Cache results when possible
- Avoid heavy computations in decorator functions
- Consider using factory decorators for customization
- Profile your application to identify potential bottlenecks
Remember, the best decorator is often the simplest one that solves your specific problem.
Start small with decorators and gradually expand their use as you become more comfortable with the pattern. They’re a powerful tool in your TypeScript arsenal, but like any tool, they should be used judiciously and with purpose.

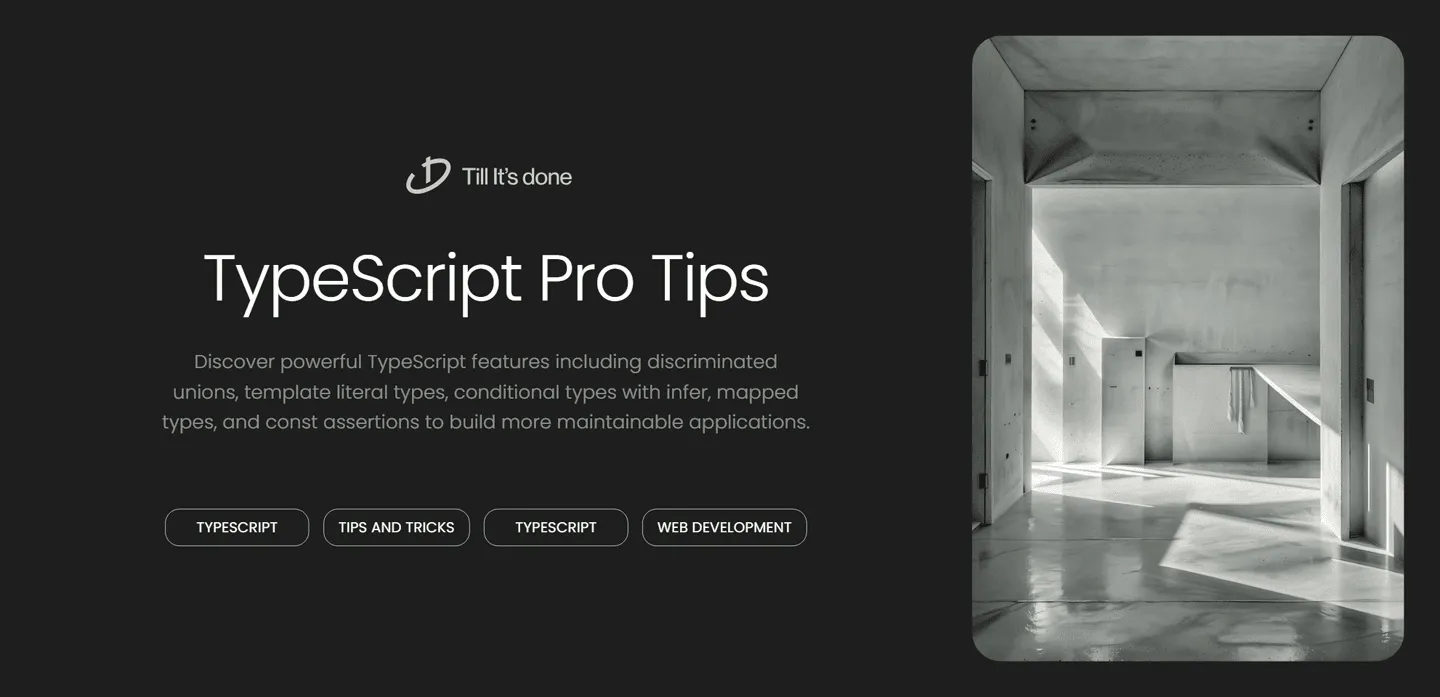
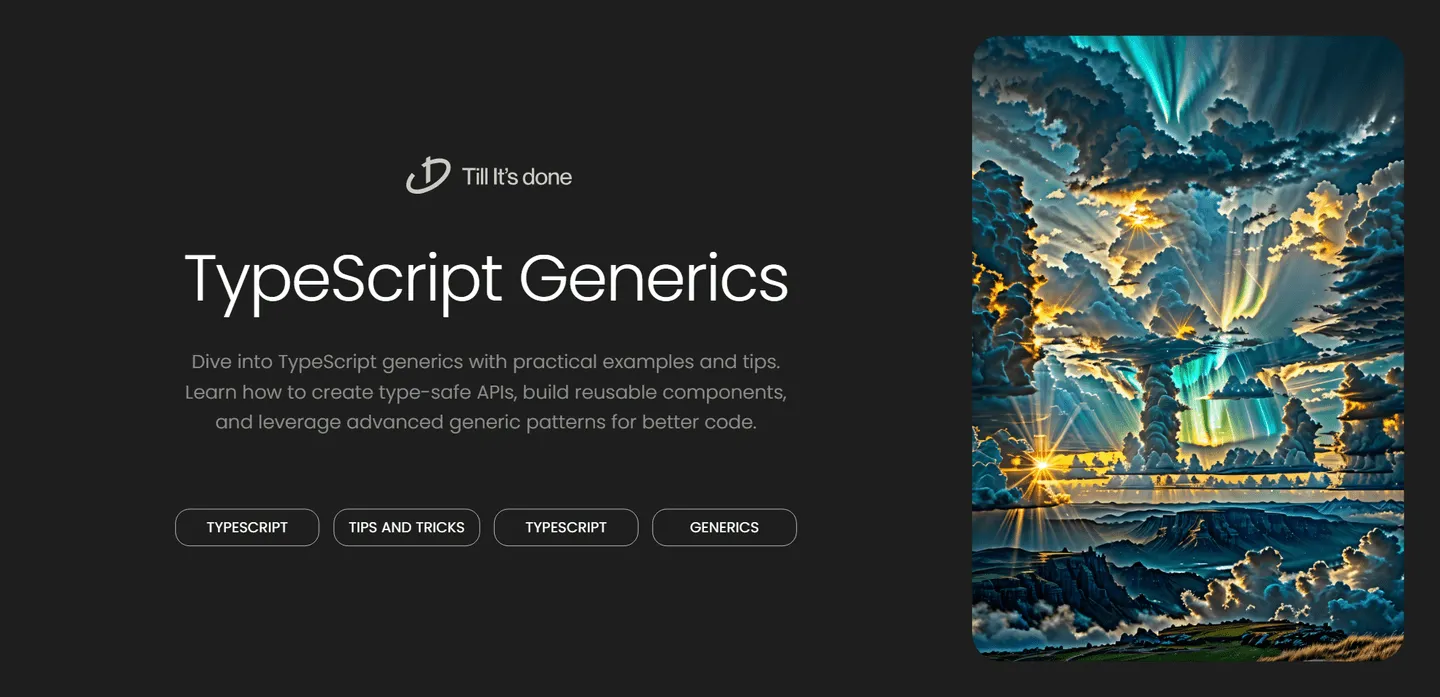



Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.