- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Getting Started with TypeScript Classes
Discover how to structure your code better with object-oriented programming principles.
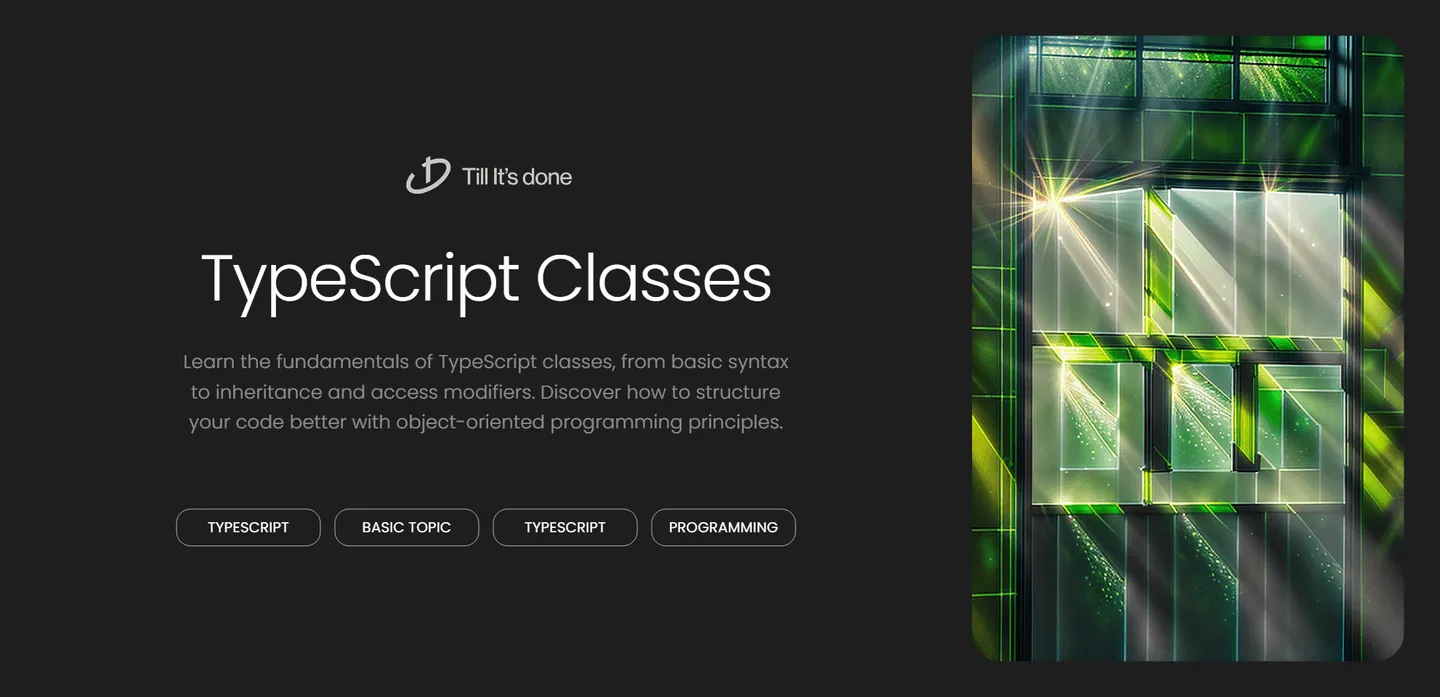
Getting Started with TypeScript Classes
TypeScript classes have revolutionized how we structure our code, bringing object-oriented programming principles to the JavaScript ecosystem. If you’re just starting with TypeScript, understanding classes is a crucial step in your journey. Let’s dive into the fundamentals and explore how classes can make your code more organized and maintainable.
What Are TypeScript Classes?
Think of a class as a blueprint for creating objects. Just like an architect’s blueprint details every aspect of a building, a TypeScript class defines the structure and behavior of objects we want to create. It’s a powerful way to encapsulate related data and functions into a single, organized unit.
Creating Your First Class
Let’s start with a simple example. Imagine we’re building a library management system:
class Book { title: string; author: string; pages: number;
constructor(title: string, author: string, pages: number) { this.title = title; this.author = author; this.pages = pages; }
getInfo(): string { return `${this.title} by ${this.author} has ${this.pages} pages`; }}
This class definition includes properties (title, author, pages) and methods (constructor and getInfo). It’s like creating a custom data type that can also perform actions.
Working with Class Inheritance
One of the most powerful features of classes is inheritance. It allows us to create new classes based on existing ones, sharing and extending functionality.
class EBook extends Book { format: string;
constructor(title: string, author: string, pages: number, format: string) { super(title, author, pages); this.format = format; }
getInfo(): string { return `${super.getInfo()} - Available in ${this.format} format`; }}
Access Modifiers and Encapsulation
TypeScript classes support access modifiers (public, private, protected) to control how properties and methods can be accessed. This helps us build more secure and maintainable applications by hiding implementation details.
class BankAccount { private balance: number; protected accountNumber: string;
constructor(initialBalance: number) { this.balance = initialBalance; this.accountNumber = Math.random().toString(36).slice(-8); }
public getBalance(): number { return this.balance; }}
Classes are fundamental to object-oriented programming in TypeScript, providing a clean and organized way to structure your code. As you continue your TypeScript journey, you’ll discover how classes can help you build more robust and maintainable applications.
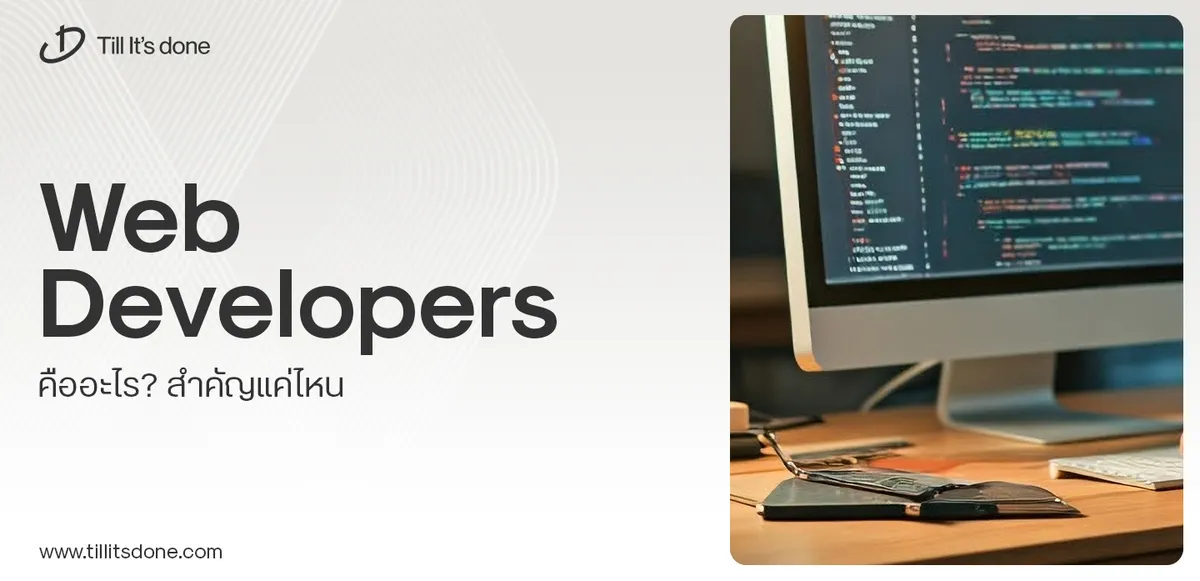
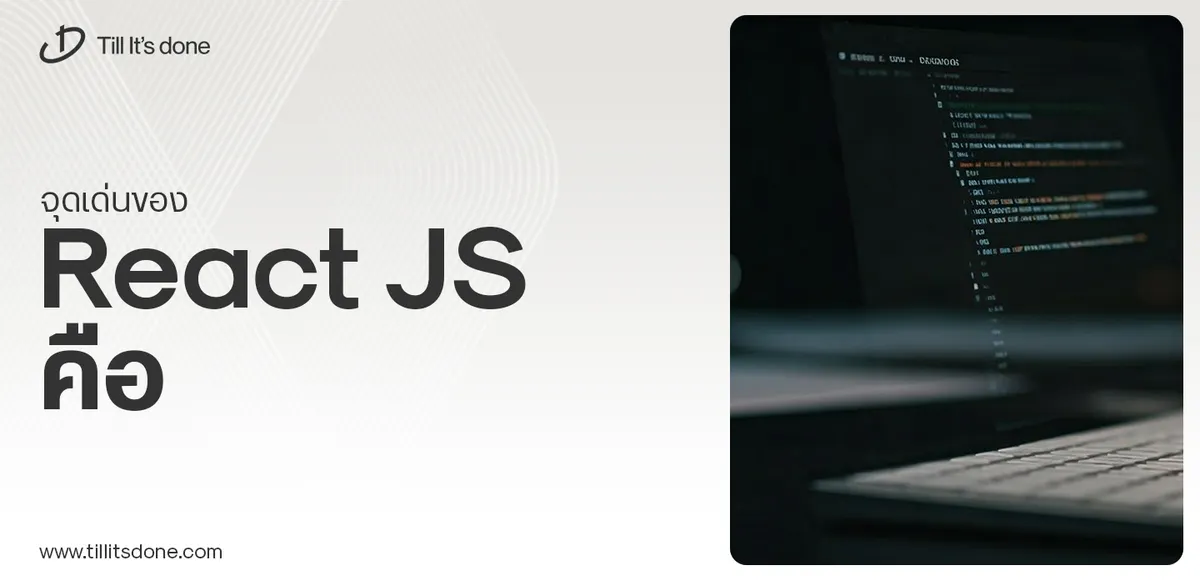
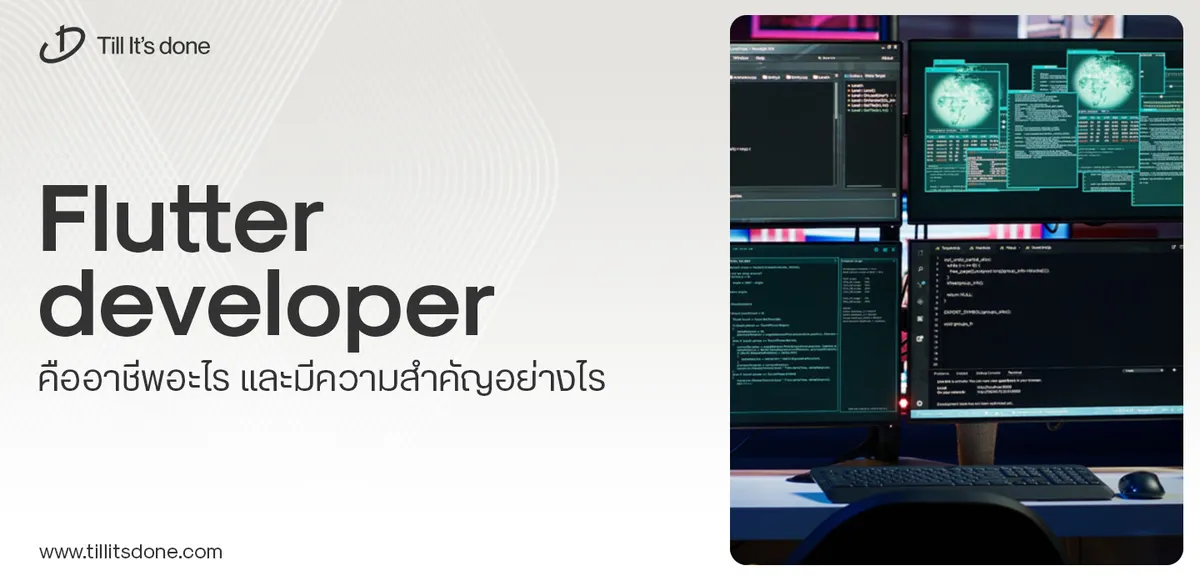
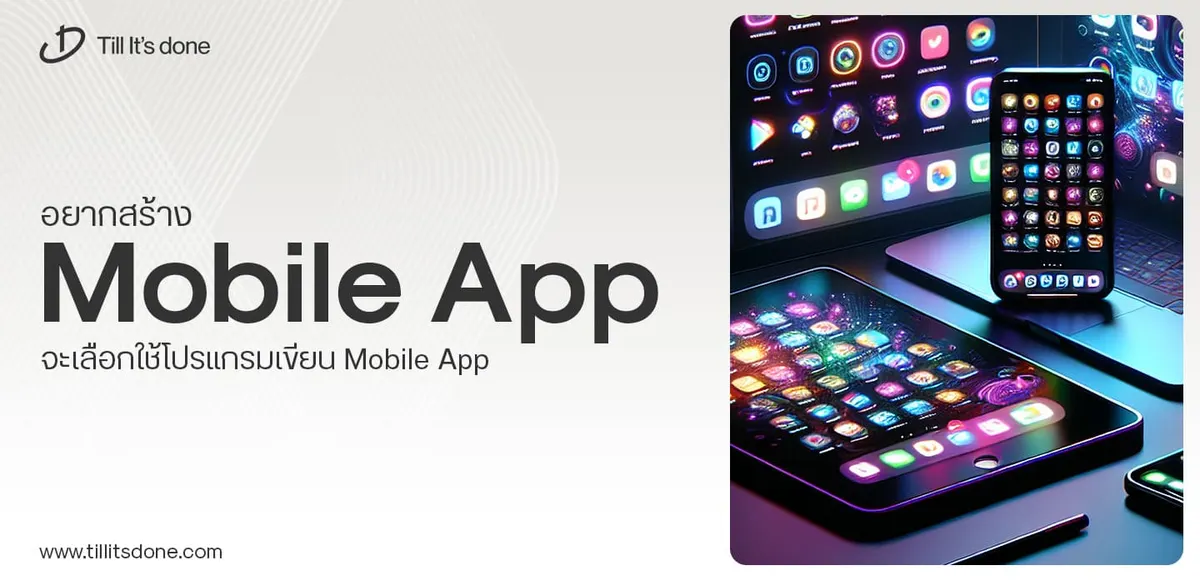
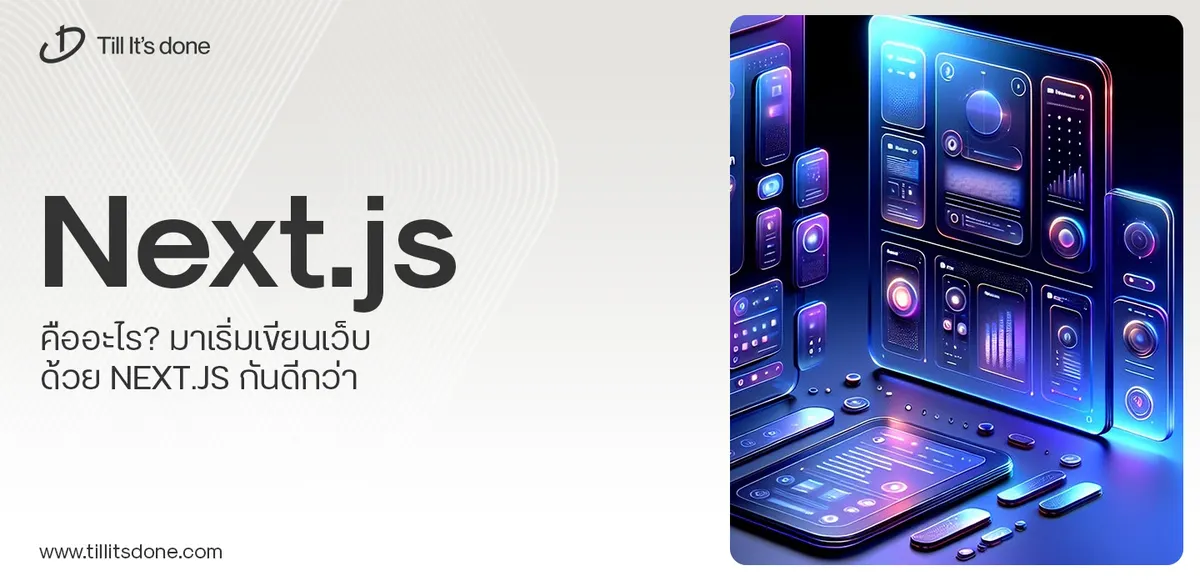
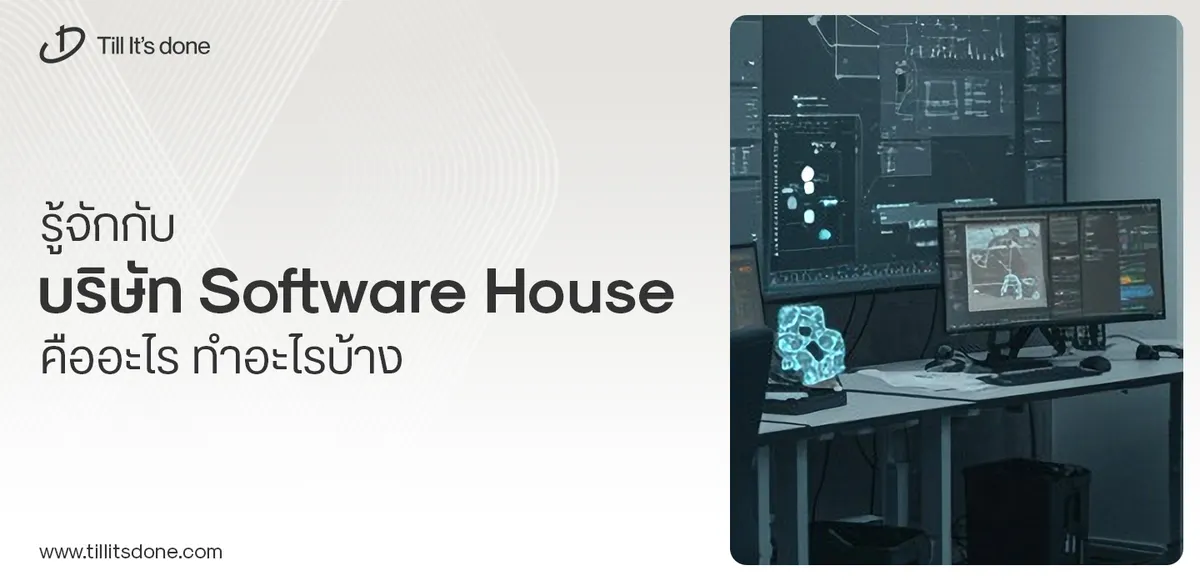
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.