- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
TypeScript Best Practices: Avoiding Common Pitfalls
Learn how to avoid common pitfalls, implement proper type annotations, and write more maintainable code with practical examples.
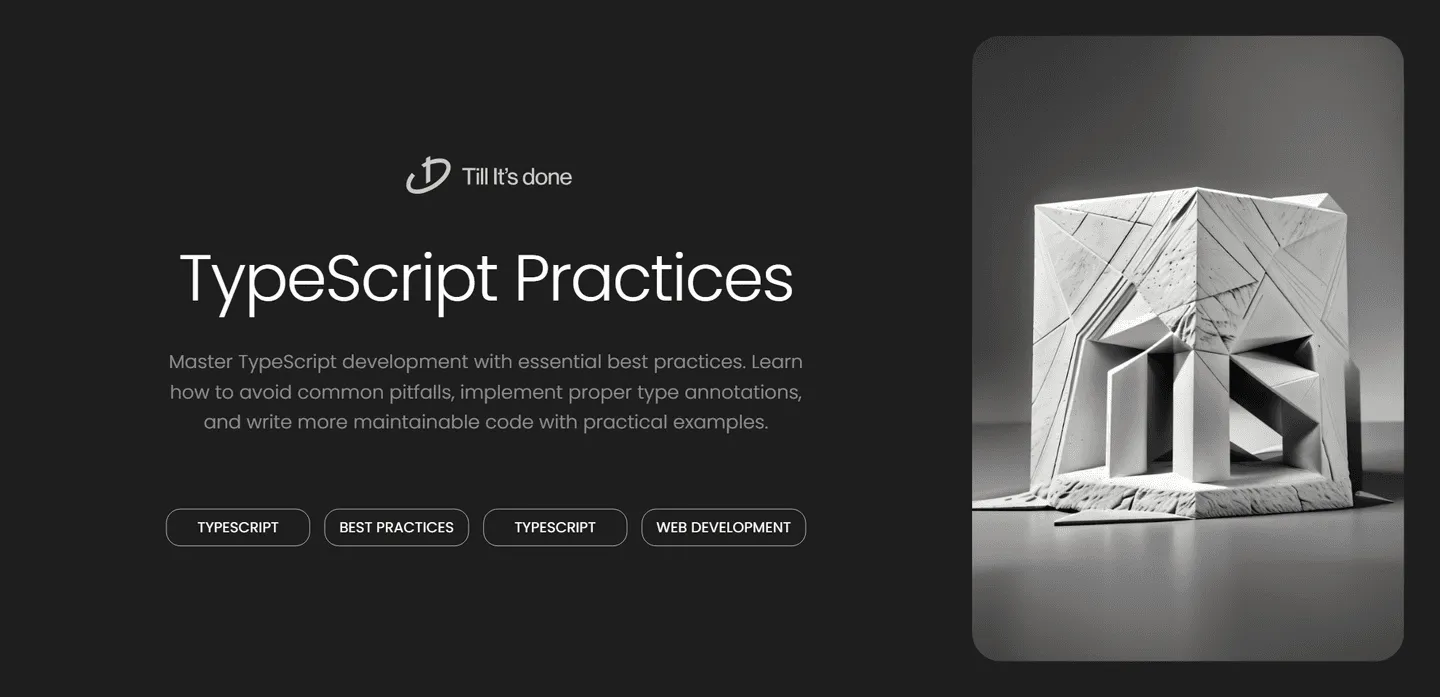
TypeScript Best Practices: Avoiding Common Pitfalls
As TypeScript continues to gain popularity in the development world, understanding its best practices becomes crucial for writing maintainable and robust code. In this guide, we’ll explore common pitfalls developers face and how to avoid them effectively.
1. Proper Type Annotations
One of the most common mistakes developers make is relying too heavily on TypeScript’s type inference. While type inference is powerful, explicit type annotations can make your code more maintainable and self-documenting.
Instead of:
let user = { name: "John", age: 30 };
Do this:
interface User { name: string; age: number;}
let user: User = { name: "John", age: 30 };
2. Avoiding the ‘any’ Type
The ‘any’ type might seem like a quick solution, but it defeats the purpose of using TypeScript. It’s like turning off your car’s safety features because you’re in a hurry.
When dealing with unknown types, consider using ‘unknown’ instead:
function processData(data: unknown) { if (typeof data === "string") { return data.toUpperCase(); } return String(data);}
3. Leveraging Union Types and Type Guards
Union types are a powerful feature that can help you write more flexible and type-safe code. Combined with type guards, they provide robust type checking.
type Response = Success | Error;
interface Success { type: 'success'; data: string;}
interface Error { type: 'error'; message: string;}
function handleResponse(response: Response) { if (response.type === 'success') { console.log(response.data); } else { console.error(response.message); }}
4. Strict Mode Configuration
Enable strict mode in your TypeScript configuration. It might seem daunting at first, but it helps catch potential issues early in development.
{ "compilerOptions": { "strict": true, "noImplicitAny": true, "strictNullChecks": true, "strictFunctionTypes": true }}
5. Using Generics Effectively
Generics can make your code more reusable while maintaining type safety. However, keep them simple and meaningful.
function firstElement\<T\>(arr: T[]): T | undefined { return arr[0];}
6. Proper Error Handling
TypeScript can help you handle errors more effectively with custom error types and proper type checking.
class NetworkError extends Error { constructor(public statusCode: number, message: string) { super(message); this.name = 'NetworkError'; }}
Conclusion
Following these TypeScript best practices will help you write more maintainable, robust, and error-free code. Remember, TypeScript is a tool to help you write better JavaScript, but it’s only as good as how you use it.
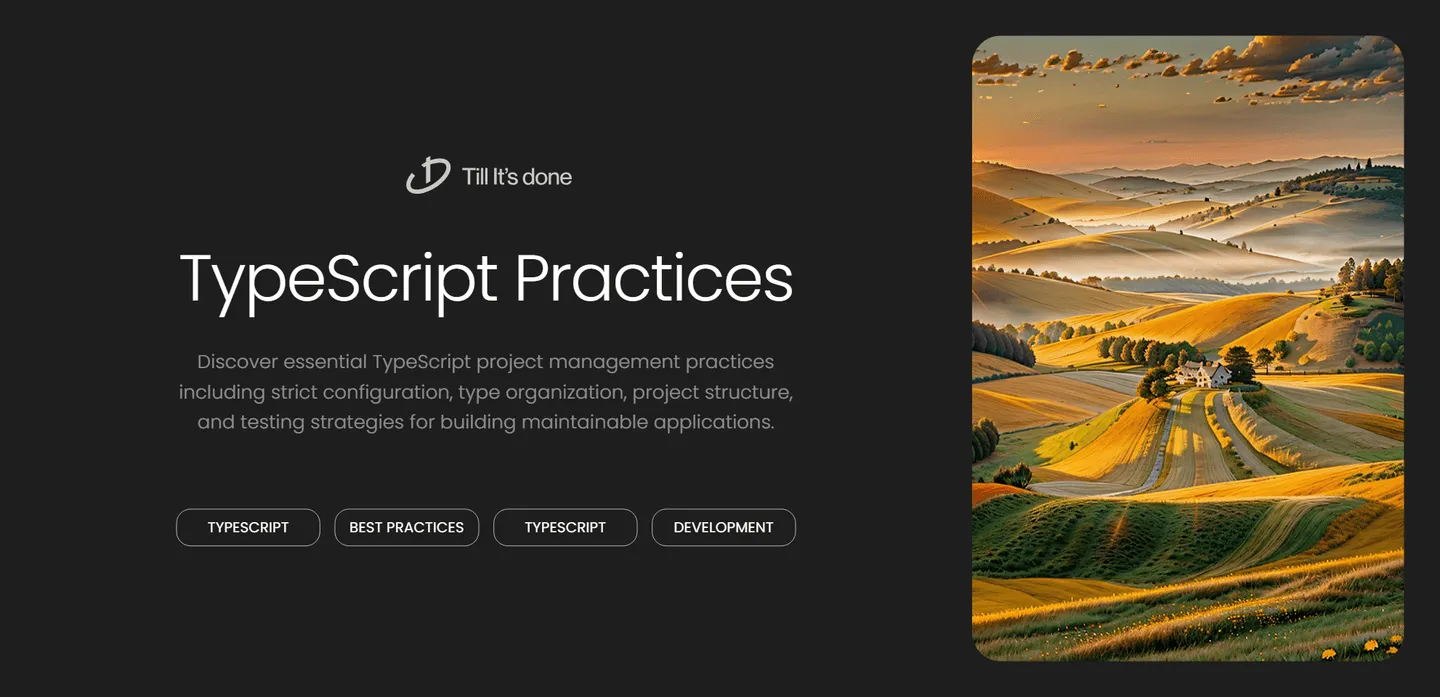
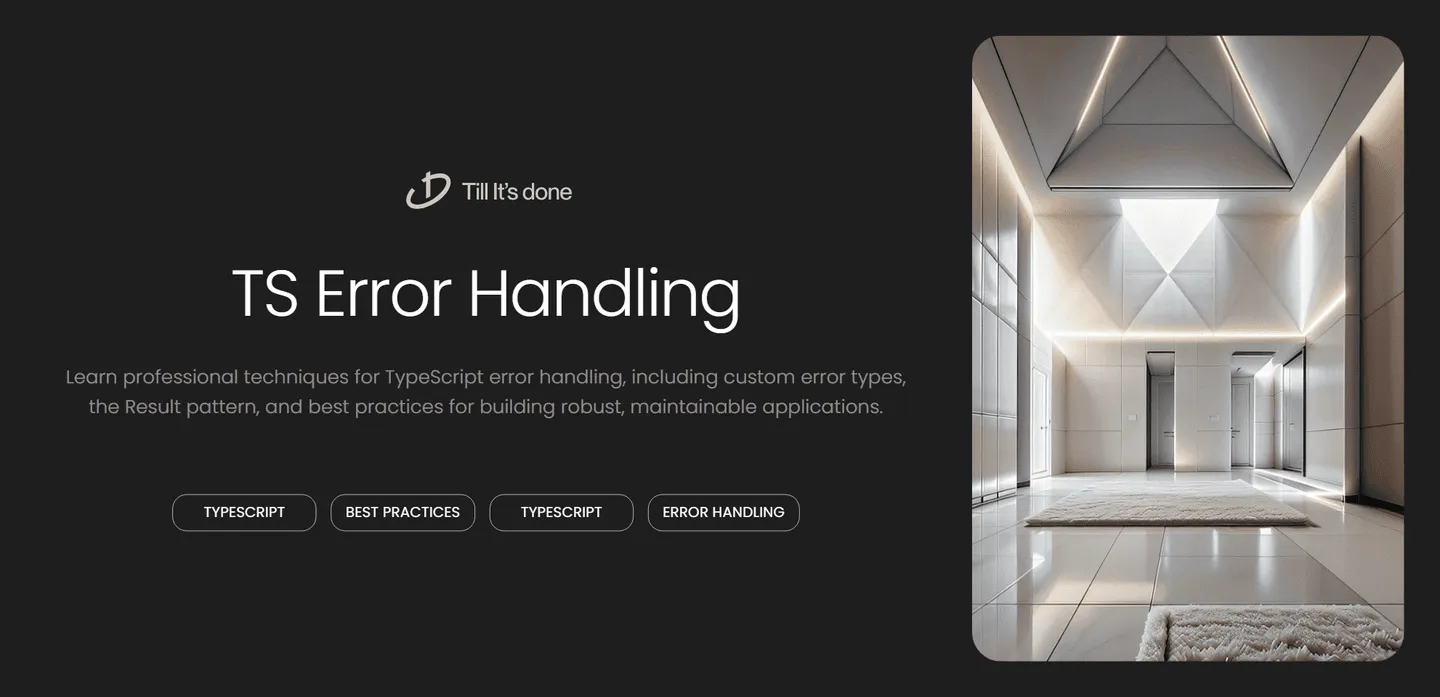
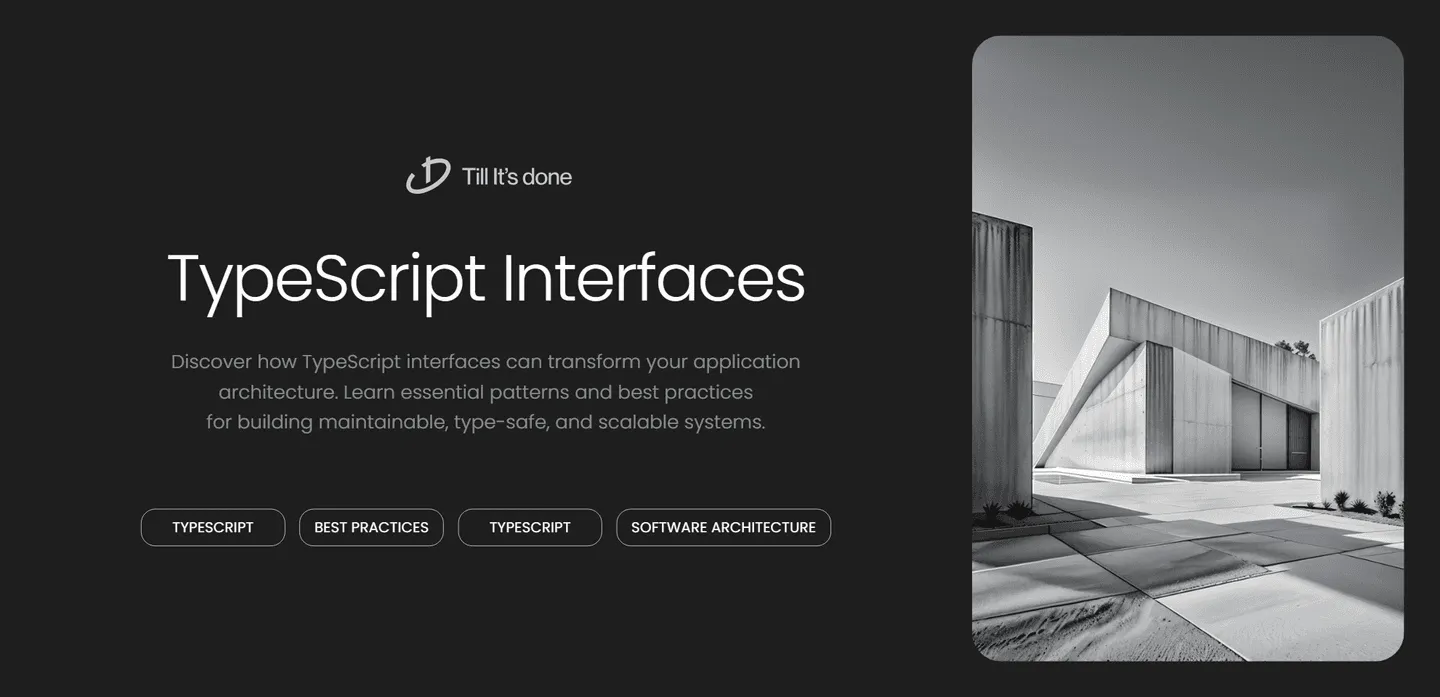
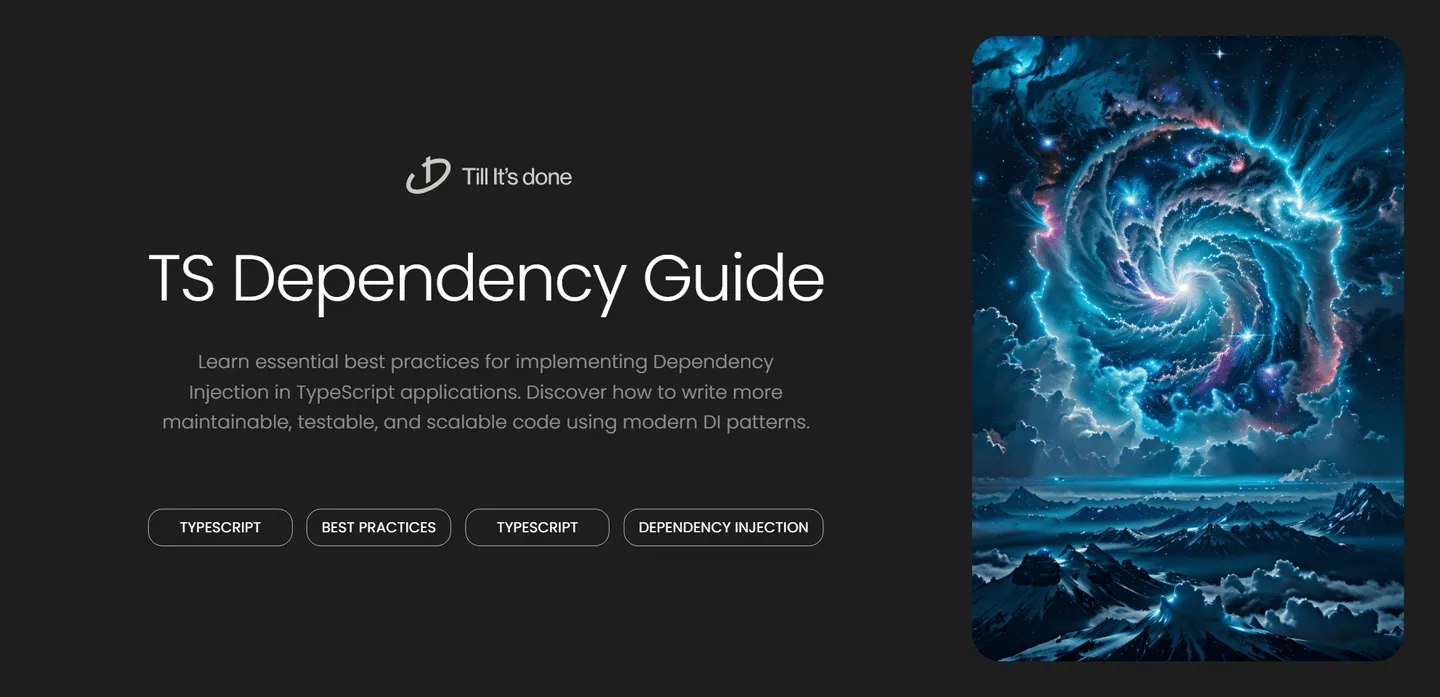
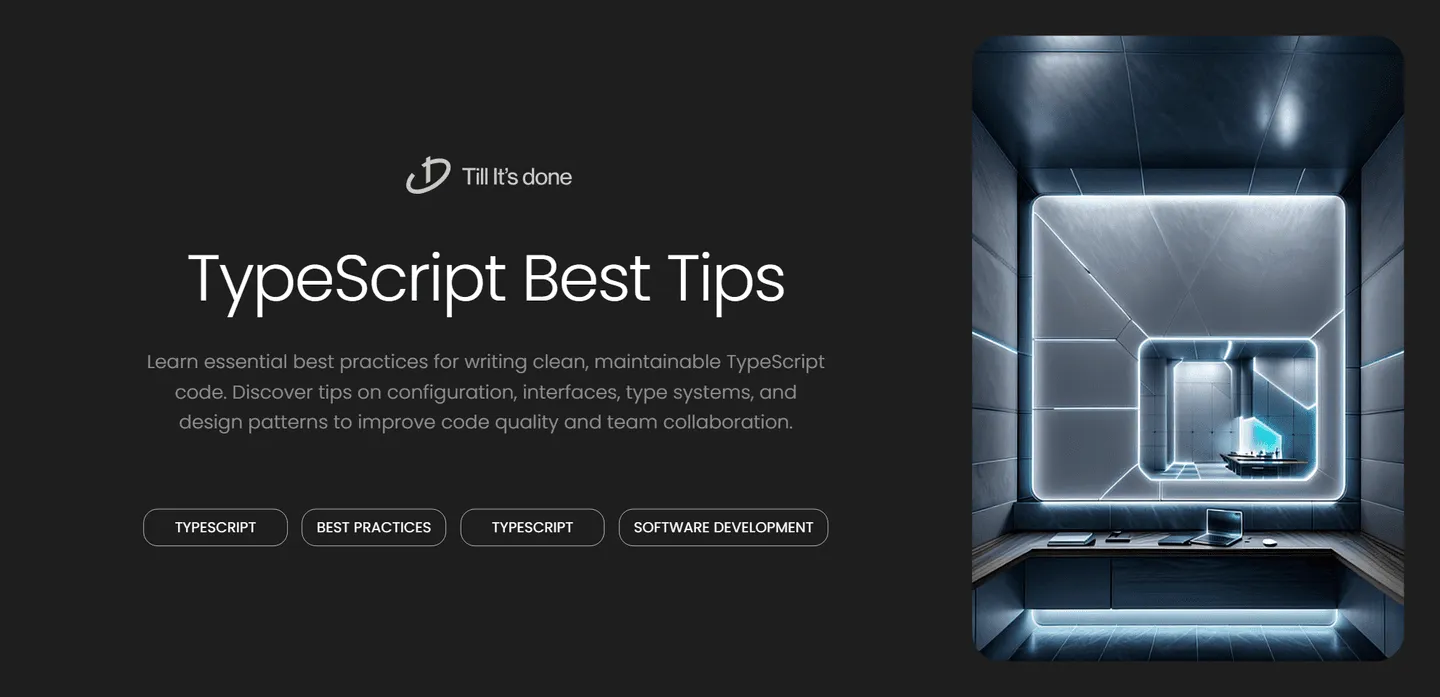
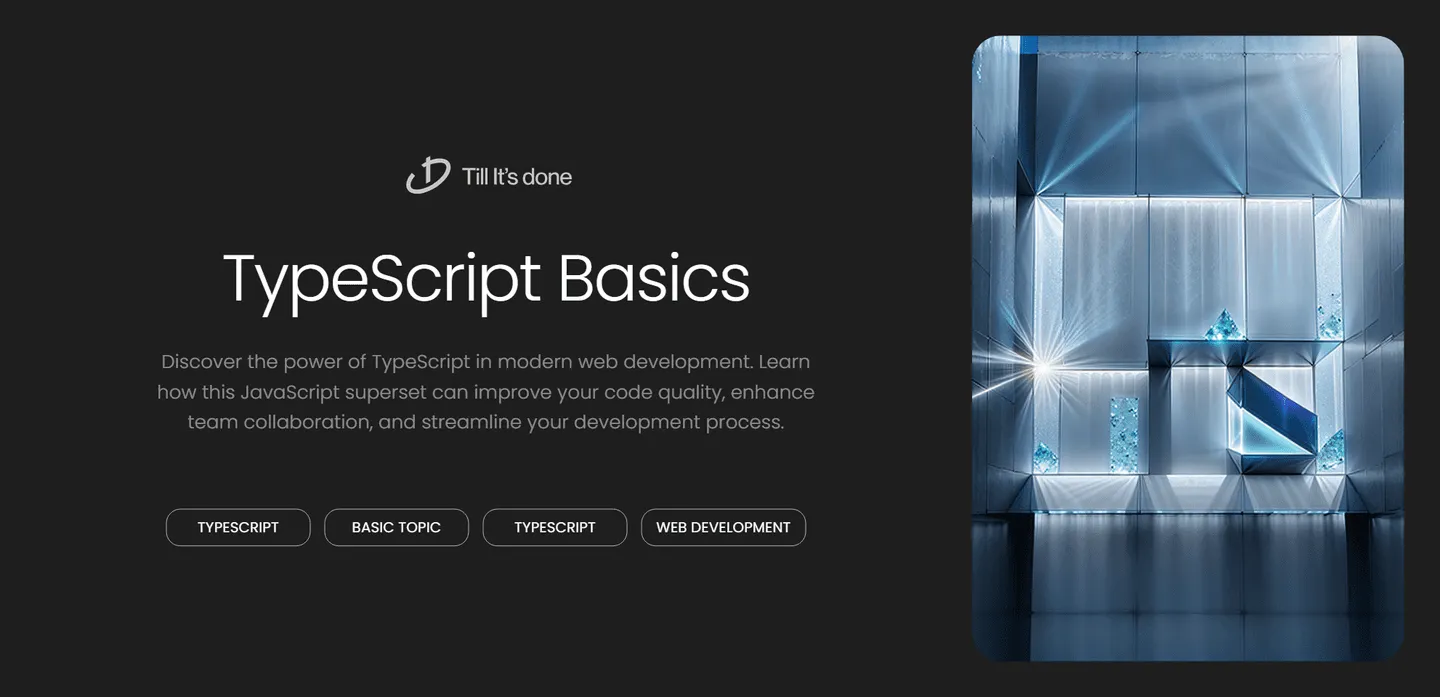
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.