- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building a Type-Safe API Client with TypeScript
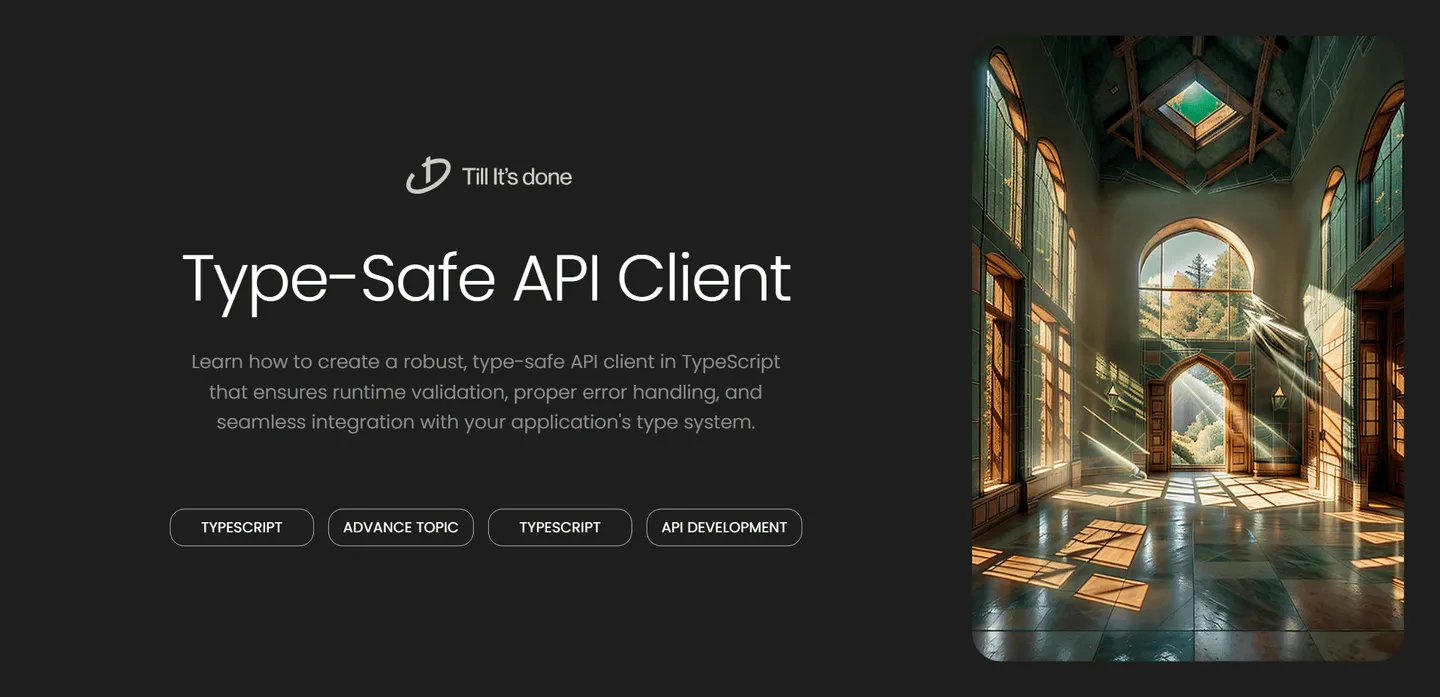
Building a Type-Safe API Client with TypeScript
Ever found yourself wrestling with API calls in TypeScript, never quite sure if you’re handling the responses correctly? You’re not alone. Today, we’ll dive into creating a robust, type-safe API client that’ll make your development experience smoother and more reliable.
The Problem with Traditional API Clients
Let’s be honest - we’ve all been there. You’re making API calls using fetch or axios, crossing your fingers that the response matches what you expect. Maybe you’ve even encountered that dreaded runtime error when the API response doesn’t quite match your types. It’s frustrating, time-consuming, and exactly what we’re going to fix.
Building Our Type-Safe Foundation
First, let’s create a foundation that ensures type safety from the ground up. We’ll start by defining our API response types:
interface ApiResponse\<T\> { data: T; status: number; message: string;}
interface User { id: number; name: string; email: string;}
Now, here’s where it gets interesting. Instead of creating a simple client, we’ll build one that validates our types at runtime:
class TypeSafeApiClient { private baseUrl: string;
constructor(baseUrl: string) { this.baseUrl = baseUrl; }
async get\<T\>(path: string, validator: (data: unknown) => data is T): Promise<ApiResponse\<T\>> { const response = await fetch(`${this.baseUrl}${path}`); const json = await response.json();
if (!validator(json.data)) { throw new Error('Response validation failed'); }
return json; }}
Implementing Runtime Type Checking
The real magic happens when we combine our type-safe client with runtime type checking. Here’s how we can implement it using a validation library:
import { z } from 'zod';
const userSchema = z.object({ id: z.number(), name: z.string(), email: z.string().email(),});
const apiClient = new TypeSafeApiClient('https://api.example.com');
// Type-safe API callconst getUser = async (id: number) => { return apiClient.get<User>( `/users/${id}`, userSchema.parse );};
Advanced Features and Error Handling
Let’s take it a step further by adding proper error handling and response type inference:
type ApiErrorResponse = { error: string; code: number;};
class TypeSafeApiError extends Error { constructor(public response: ApiErrorResponse) { super(response.error); }}
// Enhanced client methodasync request\<T\>( method: string, path: string, validator: (data: unknown) => data is T, body?: unknown): Promise\<T\> { try { const response = await fetch(`${this.baseUrl}${path}`, { method, body: body ? JSON.stringify(body) : undefined, headers: { 'Content-Type': 'application/json', }, });
const json = await response.json();
if (!response.ok) { throw new TypeSafeApiError(json); }
if (!validator(json)) { throw new Error('Response validation failed'); }
return json; } catch (error) { if (error instanceof TypeSafeApiError) { throw error; } throw new Error('Network error'); }}
When to Use This Pattern
This pattern shines when you’re building large-scale applications where type safety is crucial. It’s particularly valuable when:
- Working with complex API responses
- Dealing with frequently changing API contracts
- Building applications with strict type safety requirements
- Collaborating in large teams where type safety helps prevent bugs
Conclusion
Building a type-safe API client isn’t just about adding types - it’s about creating a robust system that catches errors early and makes your development experience more enjoyable. By combining TypeScript’s static typing with runtime validation, we’ve created a powerful pattern that will help you build more reliable applications.
Remember, type safety isn’t just about catching errors - it’s about building confidence in your code and making your development process smoother and more efficient.
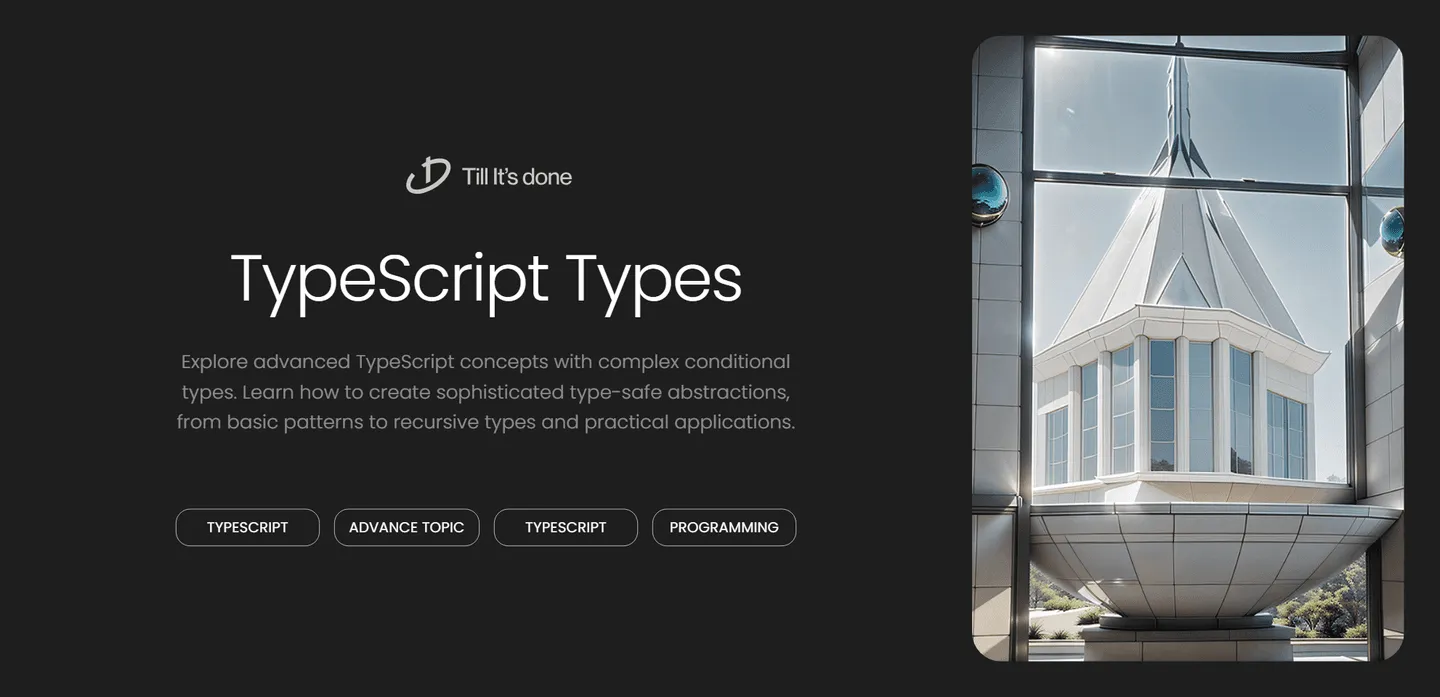
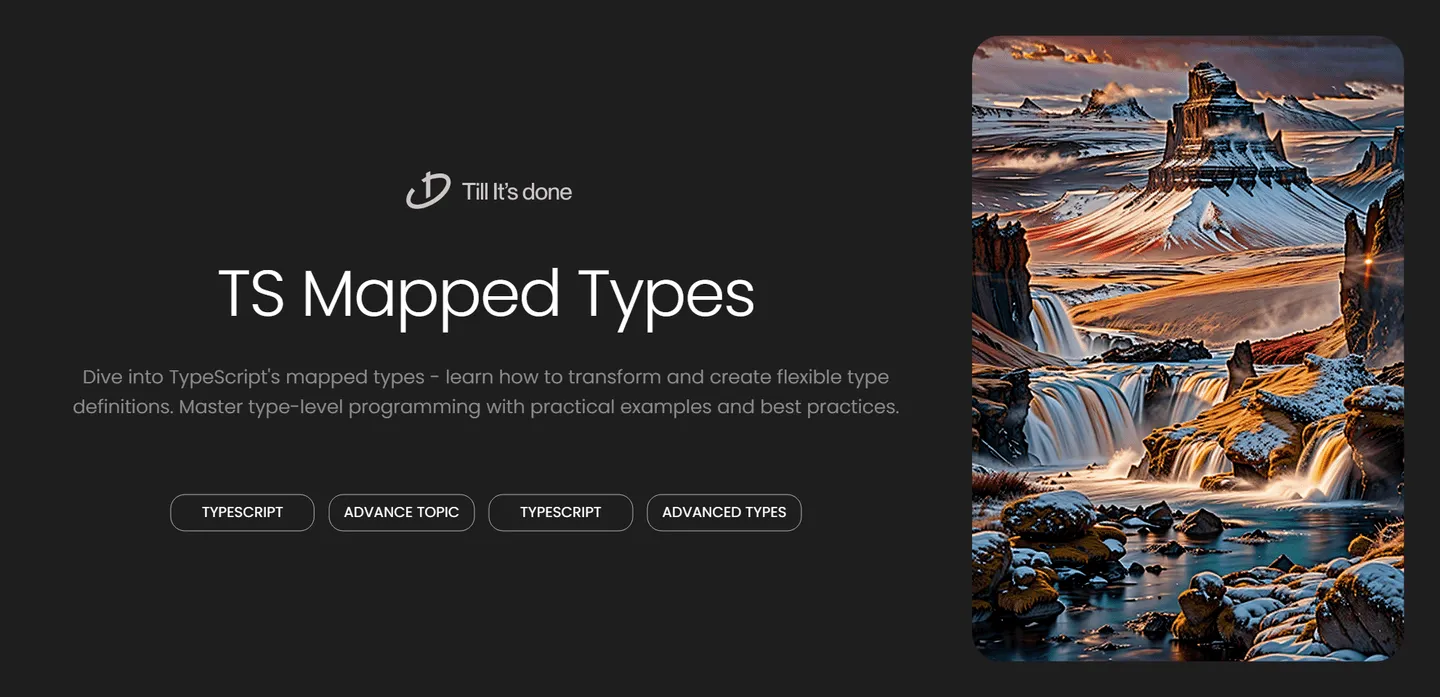
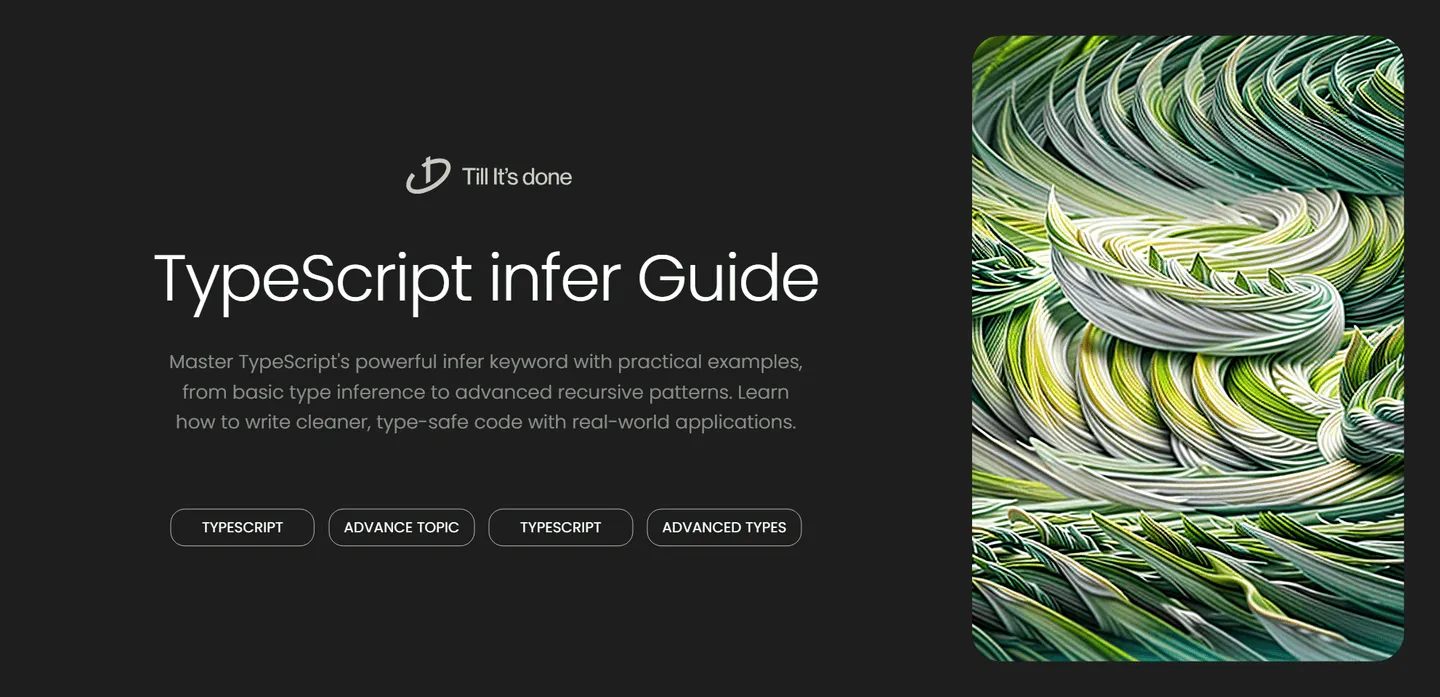
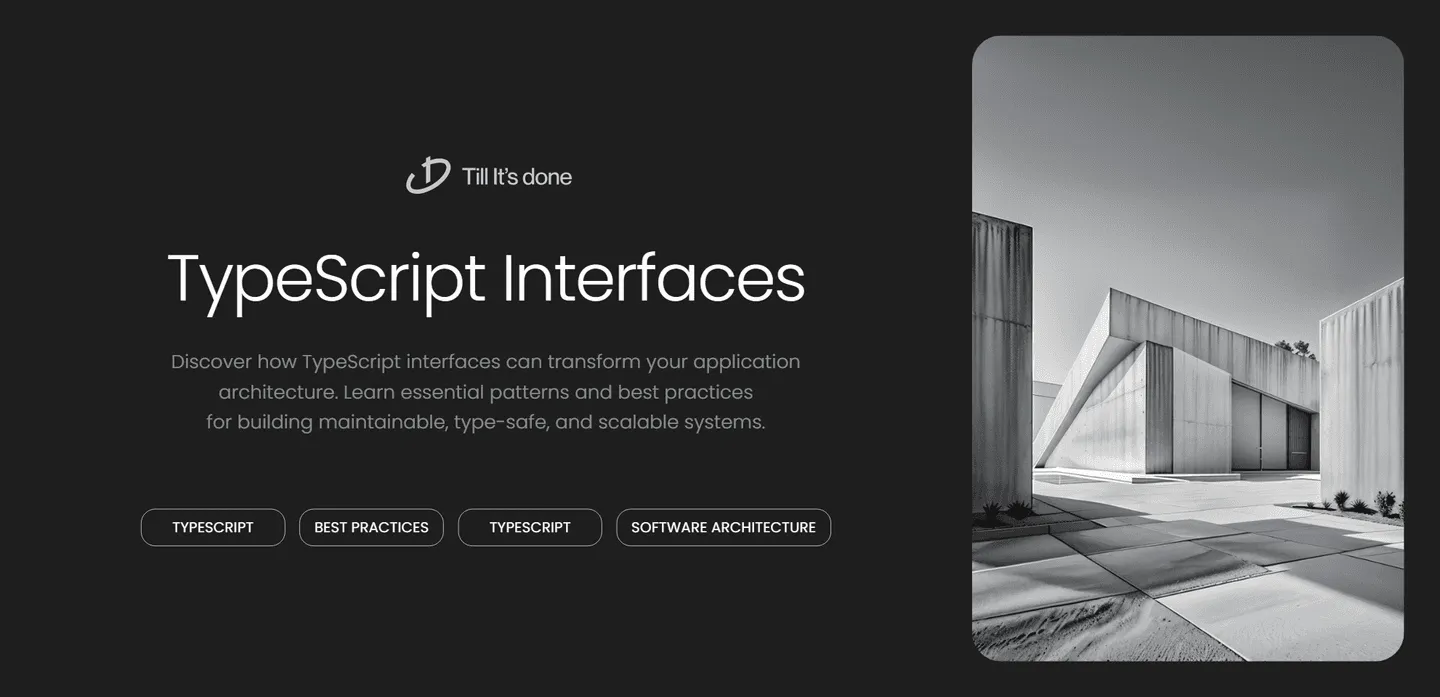
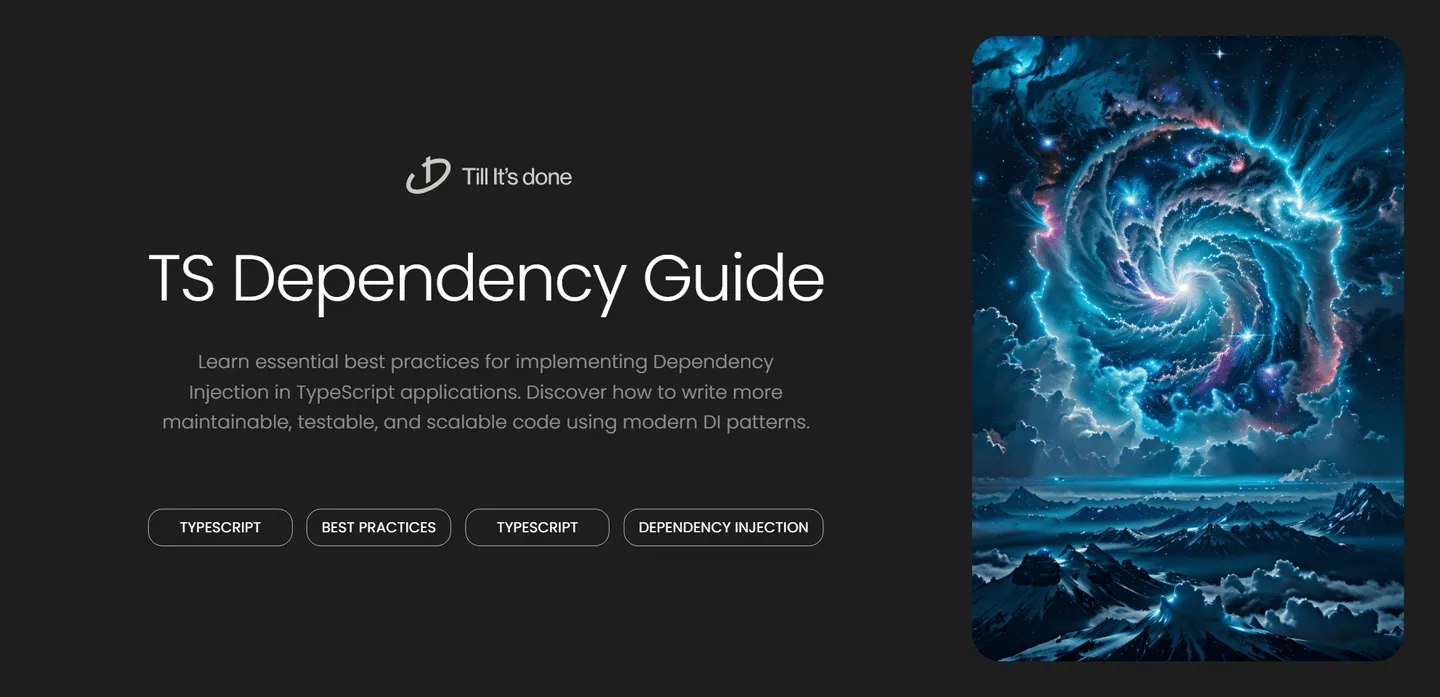
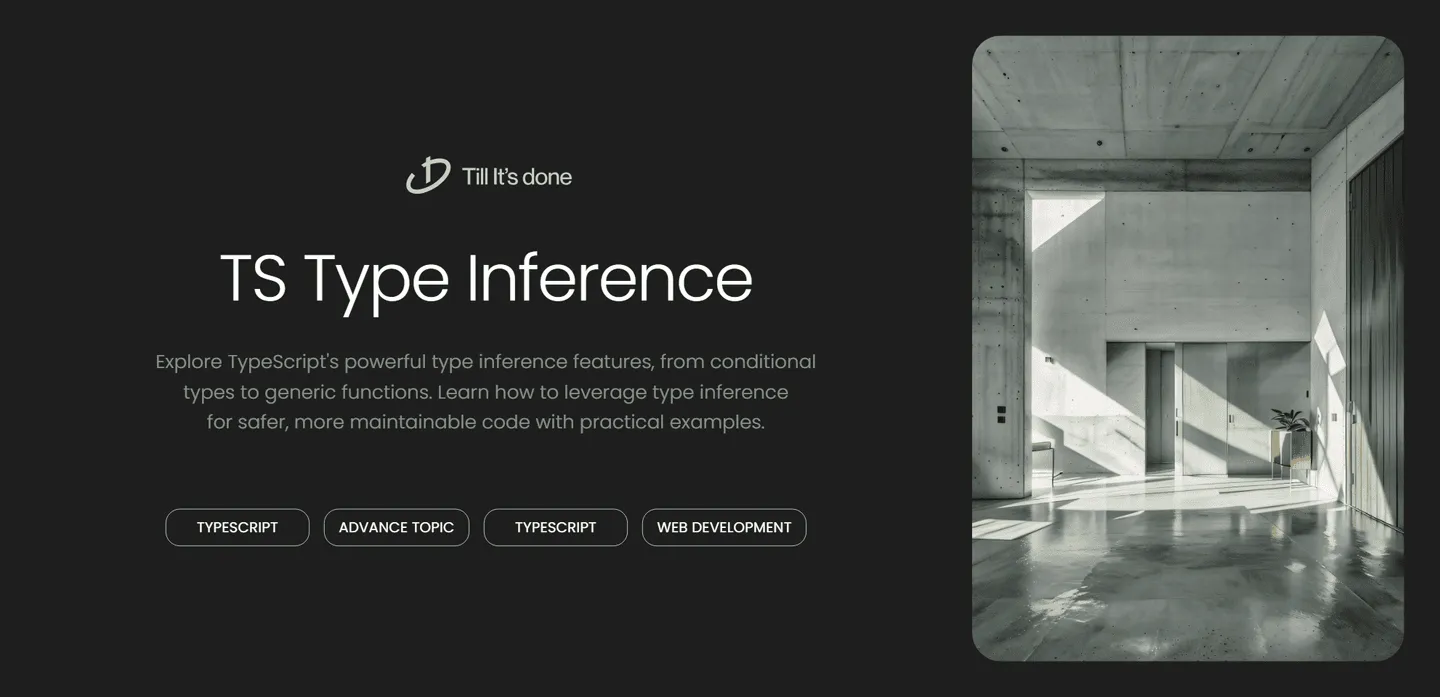
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.