- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building a Simple To-Do App with Zustand
Discover the power of simple, efficient state handling.

Building a Simple To-Do App with Zustand
Ever felt overwhelmed by Redux’s boilerplate code? Meet Zustand – the tiny, fast, and scaleable state management solution that’s taking the React world by storm. Today, we’ll build a simple to-do app that showcases just how easy state management can be.
Why Zustand?
Before we dive in, let’s talk about why Zustand might be your next favorite state management library. Unlike Redux, Zustand follows a minimalistic approach – no reducers, no action types, no dispatch. Just pure, simple state management that feels natural to use.
Setting Up Our Project
First things first, let’s create a new React project and install Zustand:
npm create vite@latest todo-zustand -- --template reactcd todo-zustandnpm install zustand
Creating Our Store
The beauty of Zustand lies in its simplicity. Here’s how we create our todo store:
import create from 'zustand'
const useStore = create((set) => ({ todos: [], addTodo: (text) => set((state) => ({ todos: [...state.todos, { id: Date.now(), text, completed: false }] })), toggleTodo: (id) => set((state) => ({ todos: state.todos.map(todo => todo.id === id ? { ...todo, completed: !todo.completed } : todo ) })), removeTodo: (id) => set((state) => ({ todos: state.todos.filter(todo => todo.id !== id) }))}))
Building the Components
Now, let’s create our todo components. The great thing about Zustand is how cleanly it integrates with React components:
function TodoApp() { const { todos, addTodo, toggleTodo, removeTodo } = useStore() const [text, setText] = useState('')
const handleSubmit = (e) => { e.preventDefault() if (!text.trim()) return addTodo(text) setText('') }
return ( <div> <form onSubmit={handleSubmit}> <input value={text} onChange={(e) => setText(e.target.value)} placeholder="What needs to be done?" /> <button type="submit">Add Todo</button> </form>
<ul> {todos.map(todo => ( <li key={todo.id}> <input type="checkbox" checked={todo.completed} onChange={() => toggleTodo(todo.id)} /> <span style={{ textDecoration: todo.completed ? 'line-through' : 'none' }}> {todo.text} </span> <button onClick={() => removeTodo(todo.id)}>Delete</button> </li> ))} </ul> </div> )}
What Makes This Approach Special?
- No Context Provider Needed: Unlike Redux or React Context, you don’t need to wrap your app in any providers.
- Automatic Re-rendering: Zustand only re-renders components when their specific subscribed state changes.
- TypeScript Ready: Zustand works great with TypeScript out of the box.
- DevTools Support: You can use Redux DevTools to debug your Zustand store.
Performance Considerations
Zustand is incredibly lightweight and performs really well out of the box. However, here are some tips to make your app even more efficient:
- Use selectors to subscribe to specific parts of the state
- Implement memo when needed for complex components
- Split your store into multiple stores if it grows too large
Conclusion
Building a todo app with Zustand shows just how straightforward modern state management can be. No complex setups, no confusing patterns – just simple, effective state management that gets out of your way and lets you focus on building features.
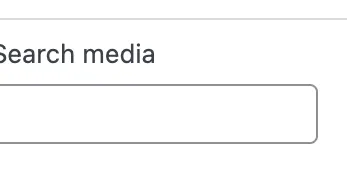





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.