- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Testing React Components with Jest and RTL
This guide covers setup, best practices, and advanced testing scenarios with practical examples.
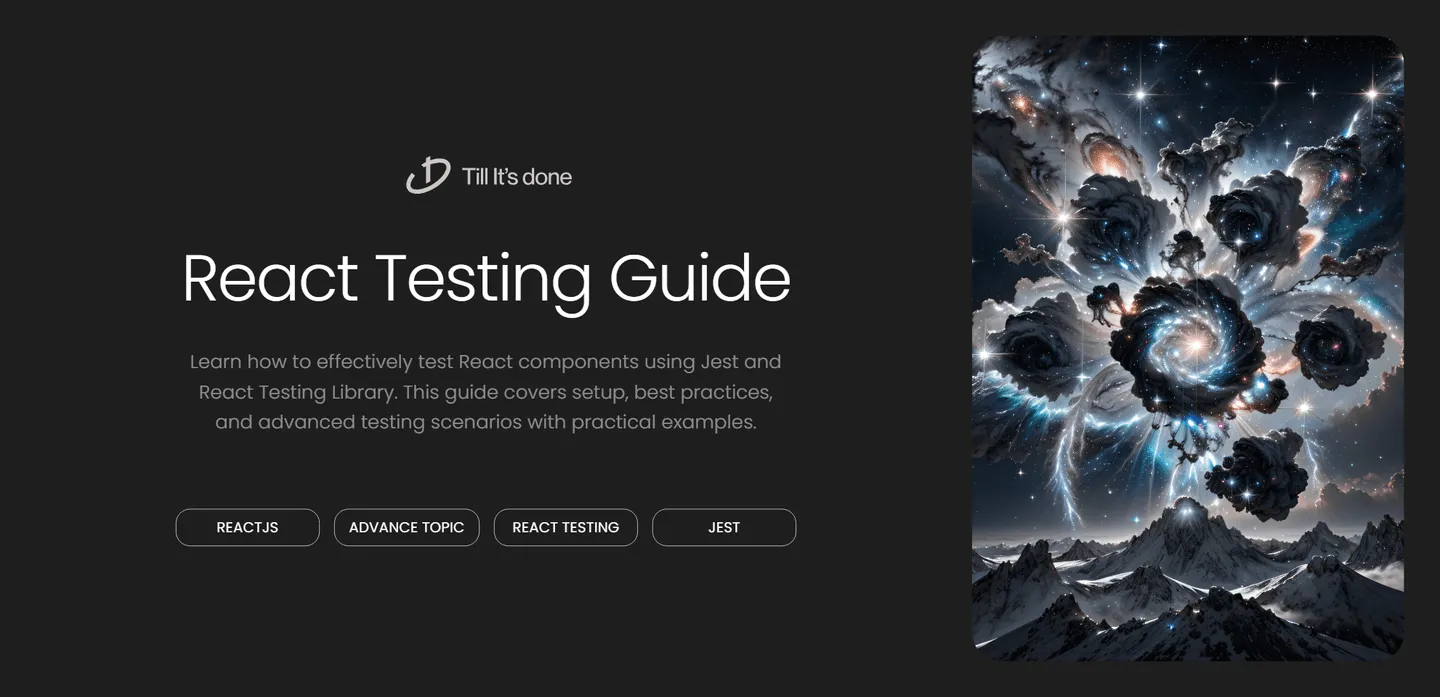
Testing React Components with Jest and React Testing Library: A Comprehensive Guide
Introduction
Testing is a crucial part of developing robust React applications. As our applications grow in complexity, having a solid testing strategy becomes increasingly important. In this guide, we’ll dive deep into testing React components using Jest and React Testing Library, exploring best practices and real-world examples.
Why Jest and React Testing Library?
Jest and React Testing Library have become the go-to testing tools in the React ecosystem. Jest serves as our test runner and assertion library, while React Testing Library enables us to test components in a way that resembles how users actually interact with our application.
Setting Up Your Testing Environment
First, let’s set up our testing environment. If you’re using Create React App, Jest and React Testing Library are included by default. For other setups, you’ll need to install the following packages:
npm install --save-dev @testing-library/react @testing-library/jest-dom jest
Writing Your First Test
Let’s start with a simple example. Consider this basic Button component:
const Button = ({ onClick, children }) => { return ( <button onClick={onClick}> {children} </button> );};
Here’s how we can test it:
import { render, screen, fireEvent } from '@testing-library/react';import Button from './Button';
describe('Button Component', () => { test('renders button with correct text', () => { render(<Button>Click me</Button>); expect(screen.getByText('Click me')).toBeInTheDocument(); });
test('calls onClick handler when clicked', () => { const handleClick = jest.fn(); render(<Button onClick={handleClick}>Click me</Button>); fireEvent.click(screen.getByText('Click me')); expect(handleClick).toHaveBeenCalledTimes(1); });});
Testing Best Practices
1. Test Behavior, Not Implementation
Focus on testing how users interact with your component rather than testing implementation details:
// ❌ Don't test implementation detailstest('should set internal state', () => { const { result } = renderHook(() => useState(false)); expect(result.current[0]).toBe(false);});
// ✅ Do test user behaviortest('should show success message when form is submitted', () => { render(<ContactForm />); fireEvent.click(screen.getByText('Submit')); expect(screen.getByText('Message sent!')).toBeInTheDocument();});
2. Use Proper Queries
React Testing Library provides several queries to find elements. Use them in this order of preference:
- getByRole
- getByLabelText
- getByPlaceholderText
- getByText
- getByTestId
// Example of proper query usagetest('form submission', () => { render(<LoginForm />);
// Using getByRole and getByLabelText const usernameInput = screen.getByLabelText('Username'); const submitButton = screen.getByRole('button', { name: /submit/i });
fireEvent.change(usernameInput, { target: { value: 'testuser' } }); fireEvent.click(submitButton);});
3. Async Testing
When testing asynchronous operations, use waitFor
or findBy
queries:
test('loads and displays user data', async () => { render(<UserProfile userId="123" />);
// Wait for loading to complete await screen.findByText('User Profile');
expect(screen.getByText('John Doe')).toBeInTheDocument();});
Advanced Testing Scenarios
Testing Custom Hooks
Use renderHook
from @testing-library/react-hooks
:
import { renderHook, act } from '@testing-library/react-hooks';import useCounter from './useCounter';
test('should increment counter', () => { const { result } = renderHook(() => useCounter());
act(() => { result.current.increment(); });
expect(result.current.count).toBe(1);});
Testing Context
When testing components that use Context, wrap them in the provider:
const customRender = (ui, { providerProps, ...renderOptions }) => { return render( <ThemeContext.Provider {...providerProps}>{ui}</ThemeContext.Provider>, renderOptions );};
Conclusion
Testing React components effectively requires a good understanding of both Jest and React Testing Library. Remember to focus on testing behavior rather than implementation details, use the right queries, and structure your tests in a way that makes them easy to maintain.
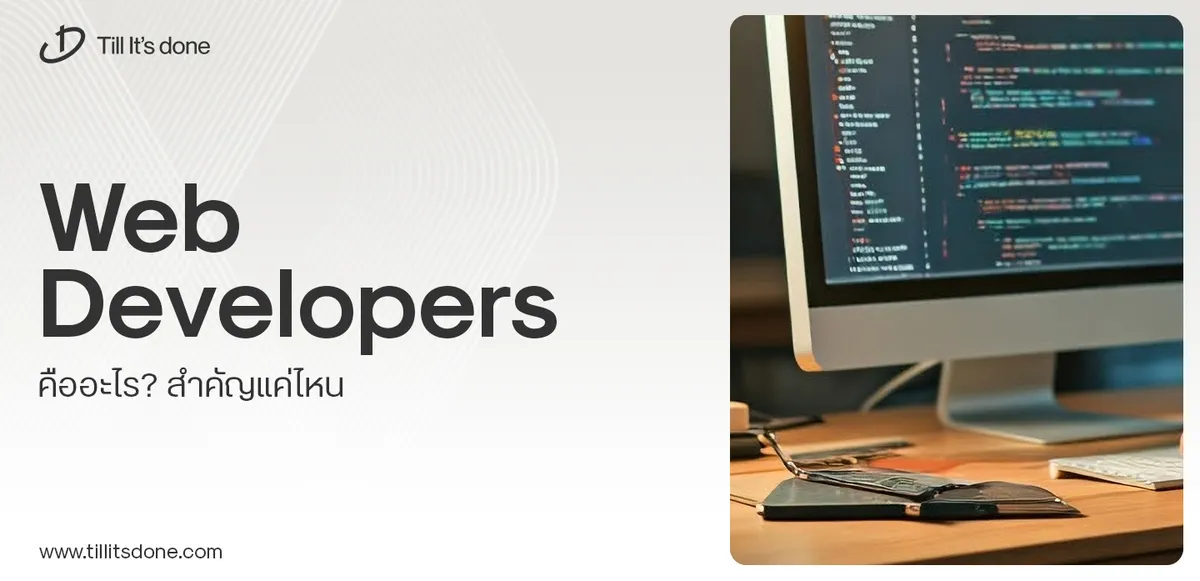
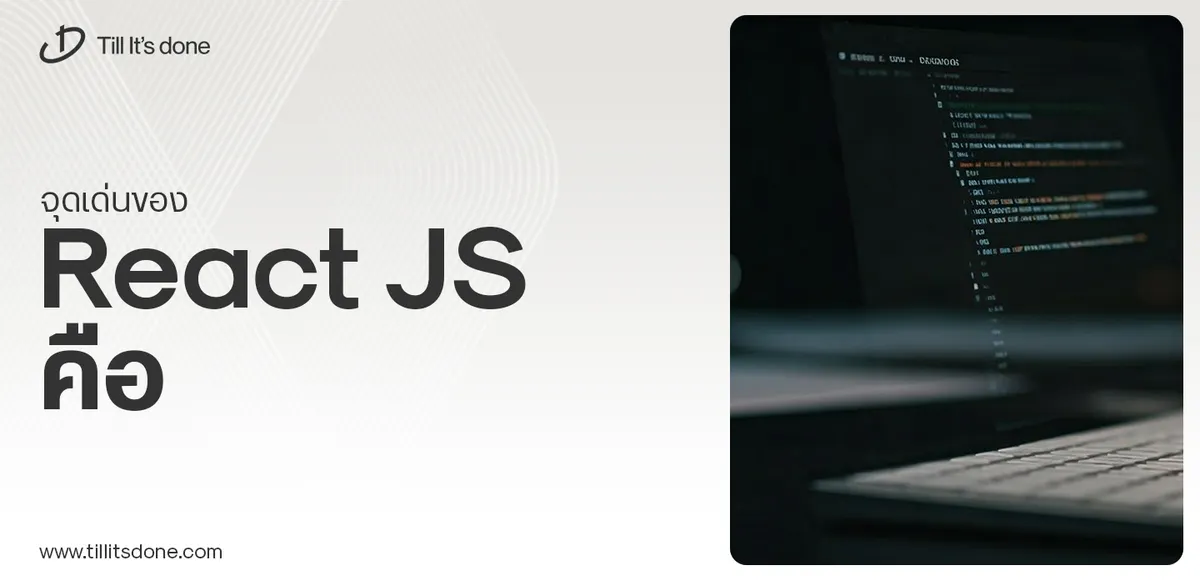
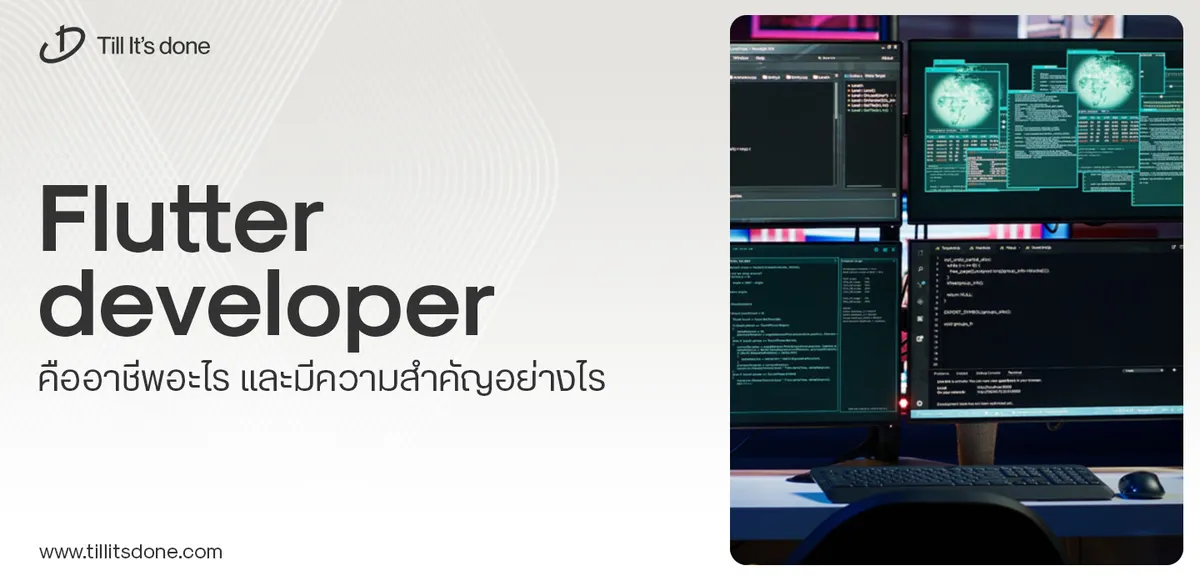
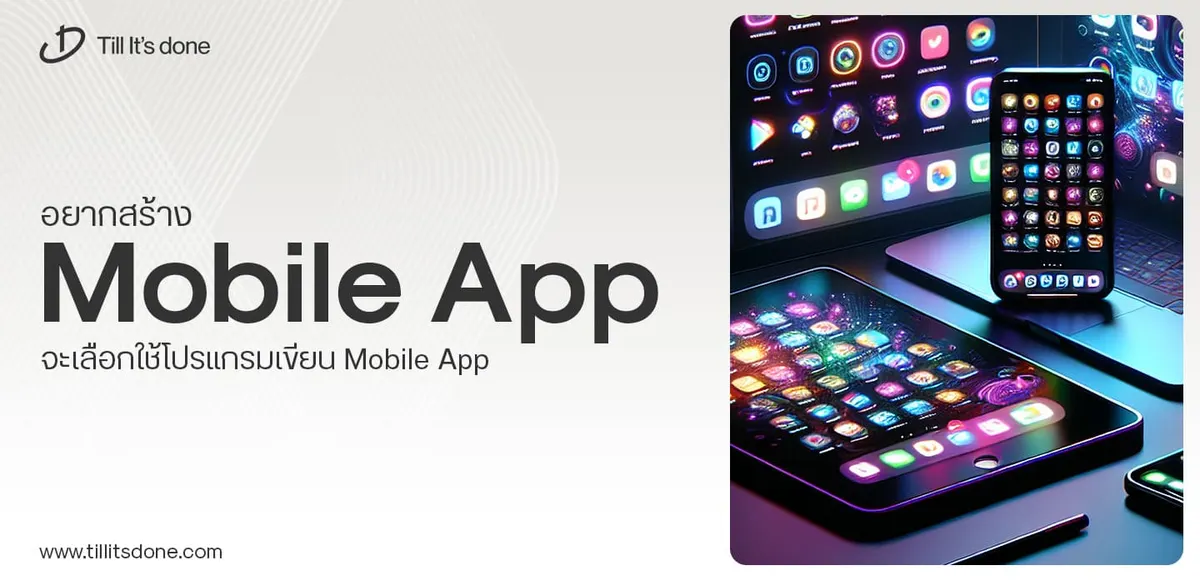
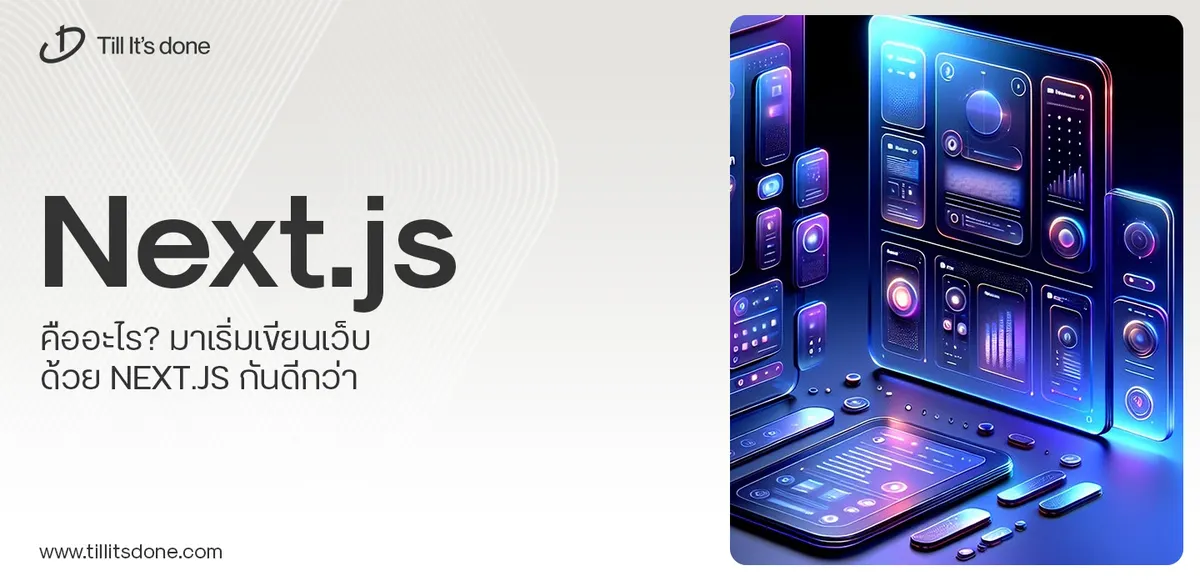
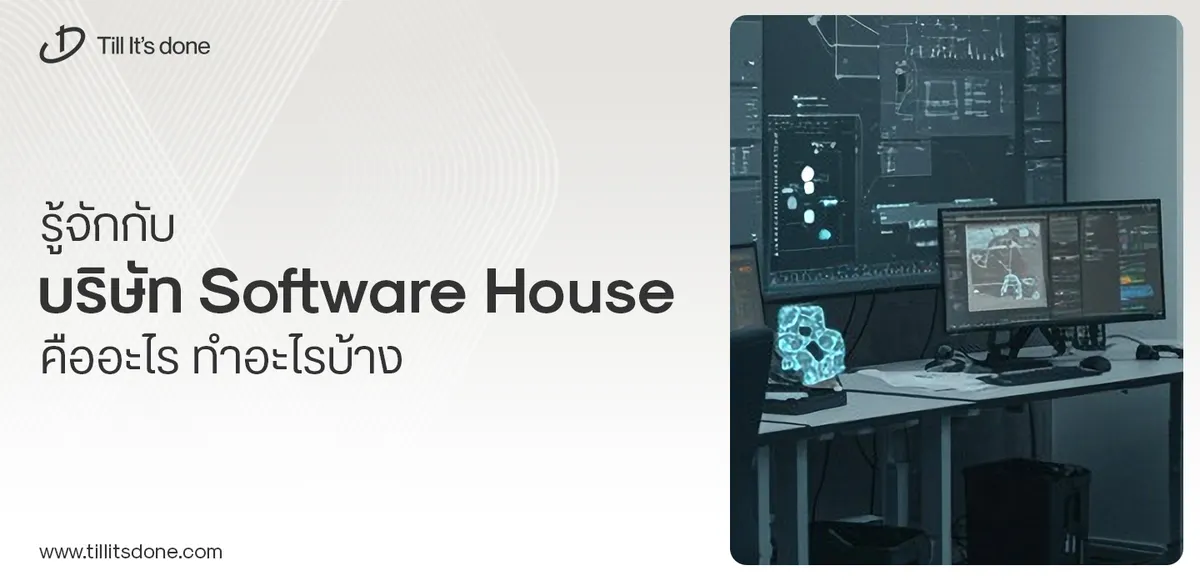
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.