- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Building Custom Hooks for Reusable Logic
Learn best practices, real-world examples, and advanced patterns for building maintainable React code.
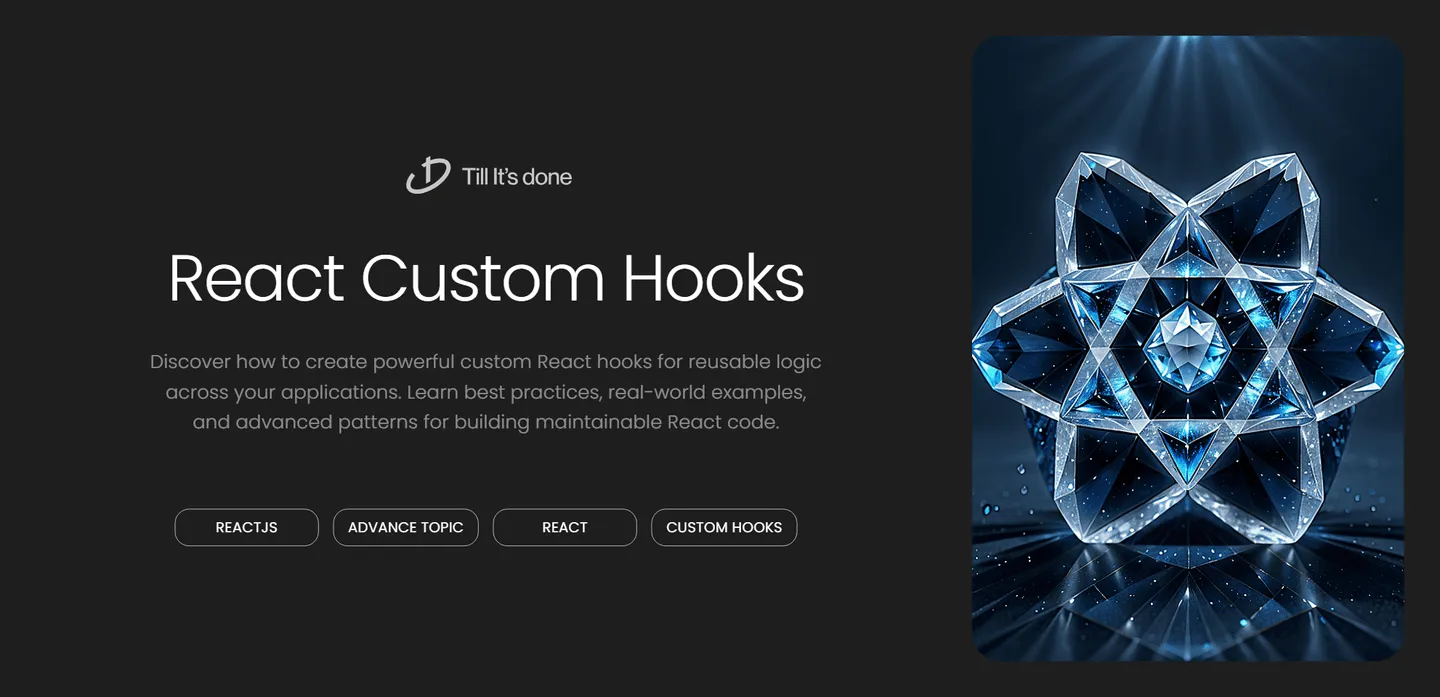
Building Custom Hooks for Reusable Logic: A Deep Dive into React’s Powerful Feature
Ever felt like you’re writing the same code over and over in your React components? I’ve been there! Today, let’s explore one of React’s most powerful features that changed how I write components - Custom Hooks. They’re like your secret weapon for creating reusable logic that you can share across your entire application.
Understanding Custom Hooks: The Basics
Think of custom hooks as your personal recipe book for React logic. Just like how you wouldn’t want to reinvent your grandmother’s secret sauce every time you cook, you shouldn’t have to rewrite common component logic repeatedly.
Custom hooks always start with the word “use” - it’s not just a convention, it’s how React recognizes our hooks. Here’s a simple example that really opened my eyes to their power:
function useWindowSize() { const [windowSize, setWindowSize] = useState({ width: window.innerWidth, height: window.innerHeight });
useEffect(() => { const handleResize = () => { setWindowSize({ width: window.innerWidth, height: window.innerHeight }); };
window.addEventListener('resize', handleResize); return () => window.removeEventListener('resize', handleResize); }, []);
return windowSize;}
Real-World Applications
Let’s dive into some practical examples where custom hooks shine. I’ve used these patterns in multiple projects, and they’ve saved me countless hours of development time.
Managing API Calls
One of my favorite custom hooks manages API calls. Here’s a pattern I use frequently:
function useFetch(url) { const [data, setData] = useState(null); const [loading, setLoading] = useState(true); const [error, setError] = useState(null);
useEffect(() => { const fetchData = async () => { try { const response = await fetch(url); const json = await response.json(); setData(json); setLoading(false); } catch (error) { setError(error); setLoading(false); } };
fetchData(); }, [url]);
return { data, loading, error };}
Form Management
Another game-changer is creating hooks for form handling:
function useForm(initialValues) { const [values, setValues] = useState(initialValues);
const handleChange = (e) => { setValues({ ...values, [e.target.name]: e.target.value }); };
const reset = () => setValues(initialValues);
return [values, handleChange, reset];}
Best Practices I’ve Learned
Through trial and error, I’ve discovered some crucial practices that make custom hooks more effective:
- Keep them focused on a single responsibility
- Name them clearly and descriptively
- Document their parameters and return values
- Handle cleanup properly in useEffect
- Consider edge cases and error states
Composing Custom Hooks
The real magic happens when you start combining custom hooks. Just like LEGO blocks, you can build complex functionality by connecting simpler hooks together:
function useAuthenticatedApi(url) { const { token } = useAuth(); const { data, loading, error } = useFetch(`${url}?token=${token}`);
return { data, loading, error };}
Wrapping Up
Custom hooks have revolutionized how we share logic in React applications. They’re not just a pattern - they’re a superpower that helps us write cleaner, more maintainable code. Start small, experiment often, and you’ll soon discover countless opportunities to extract and reuse logic in your React applications.
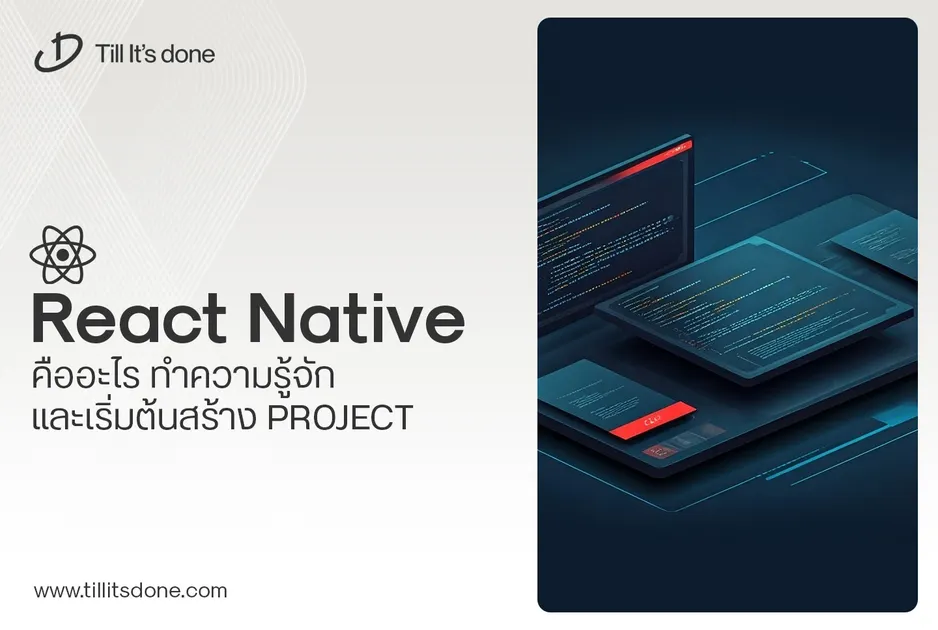
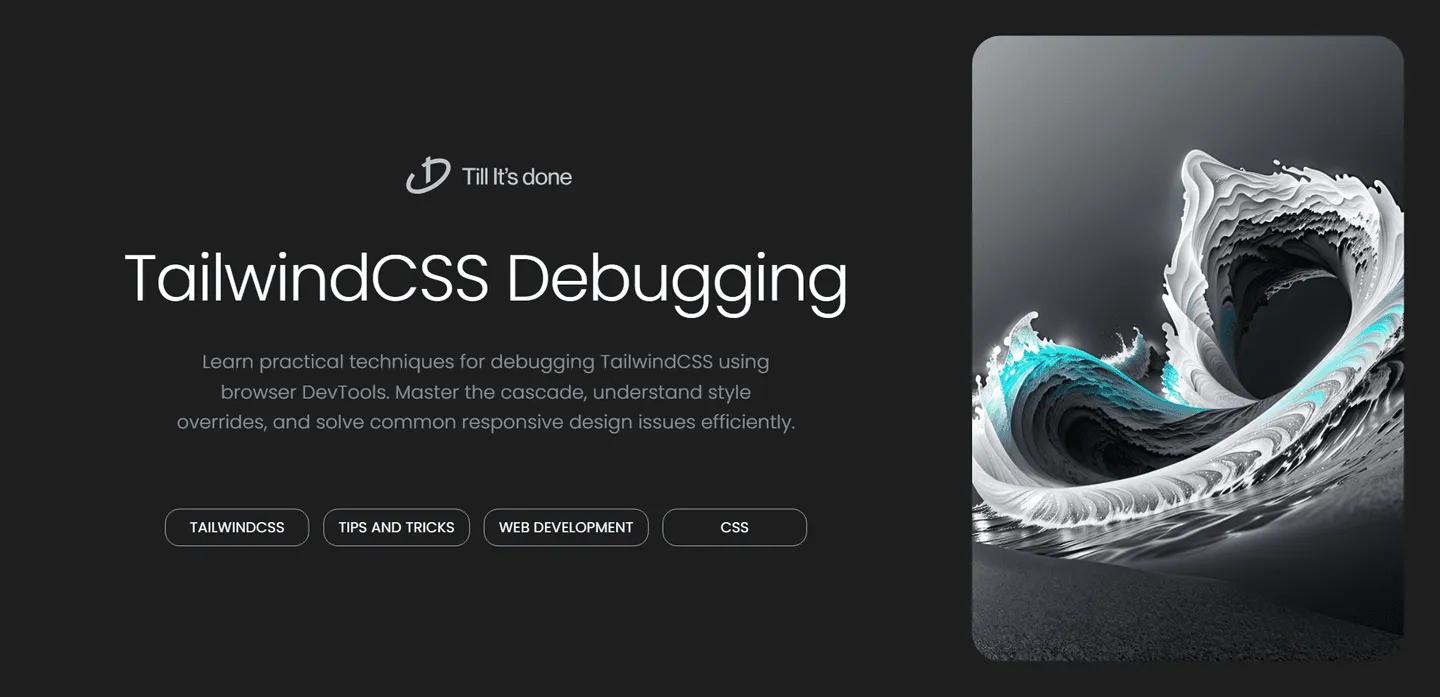
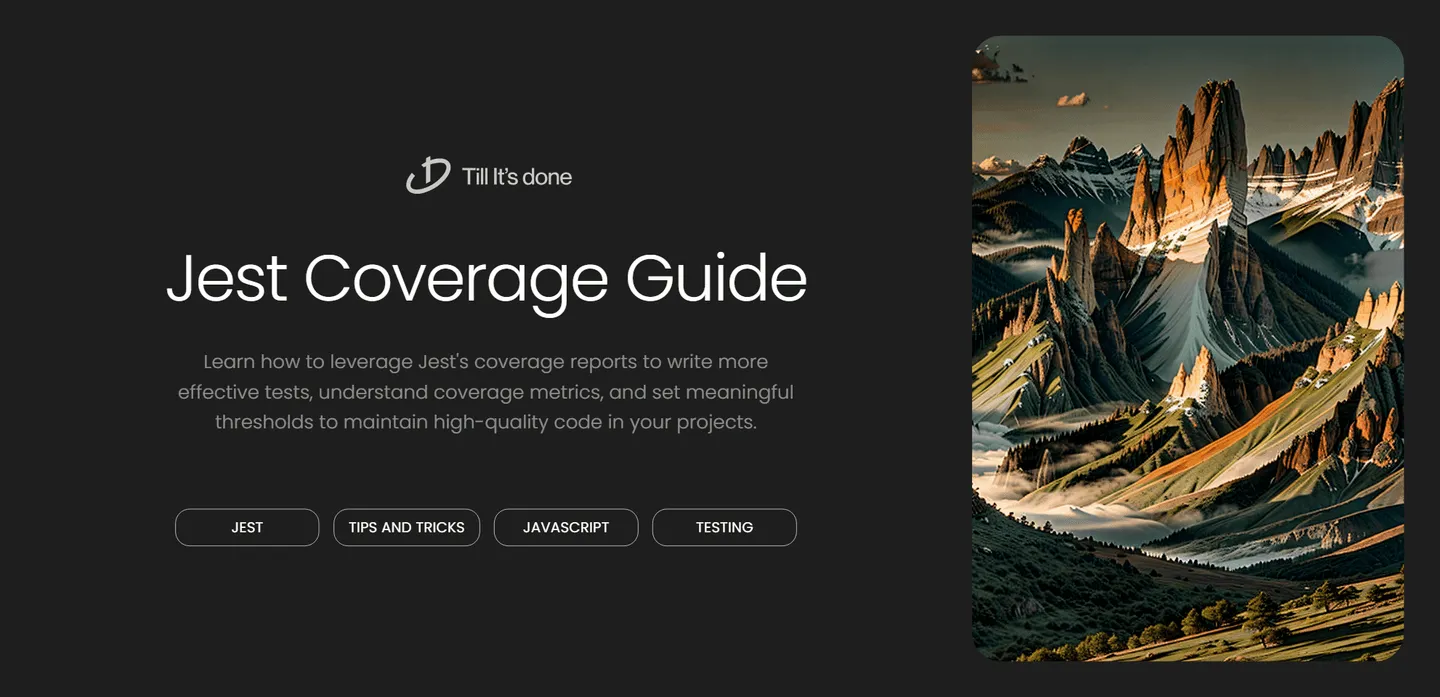
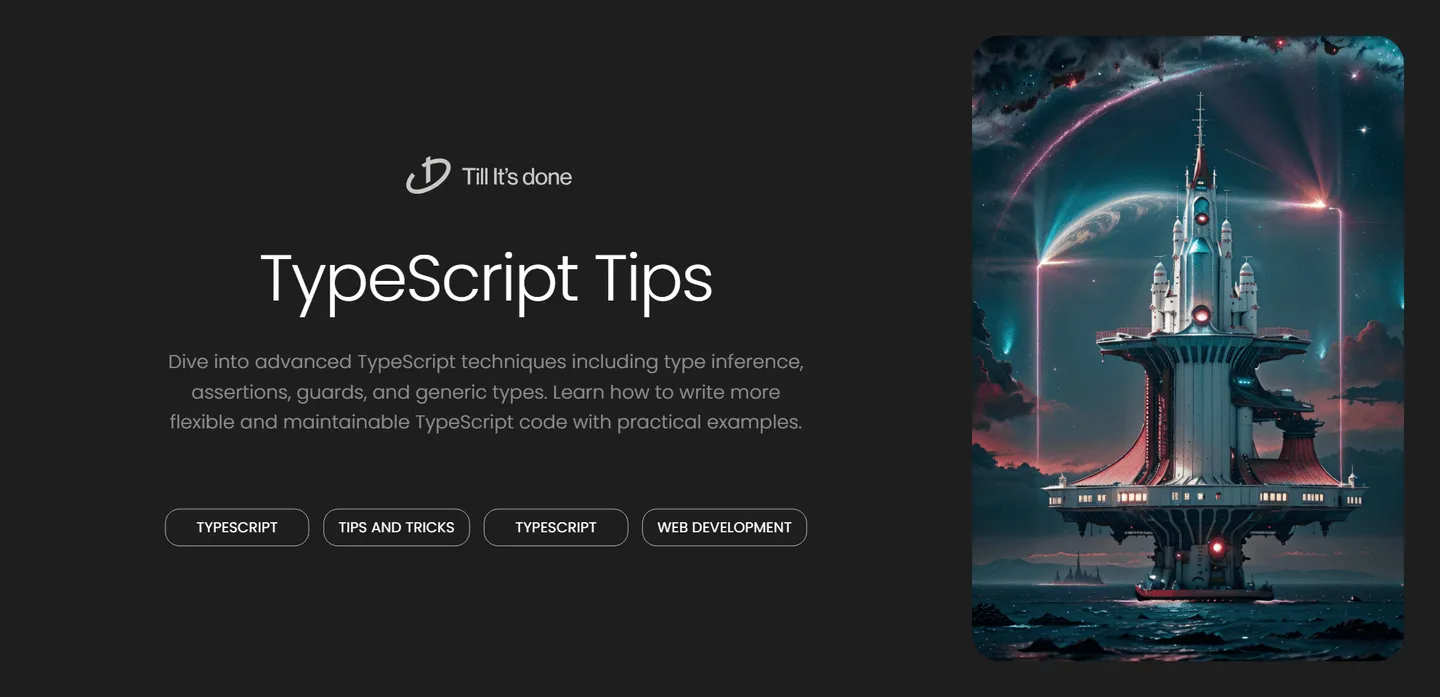
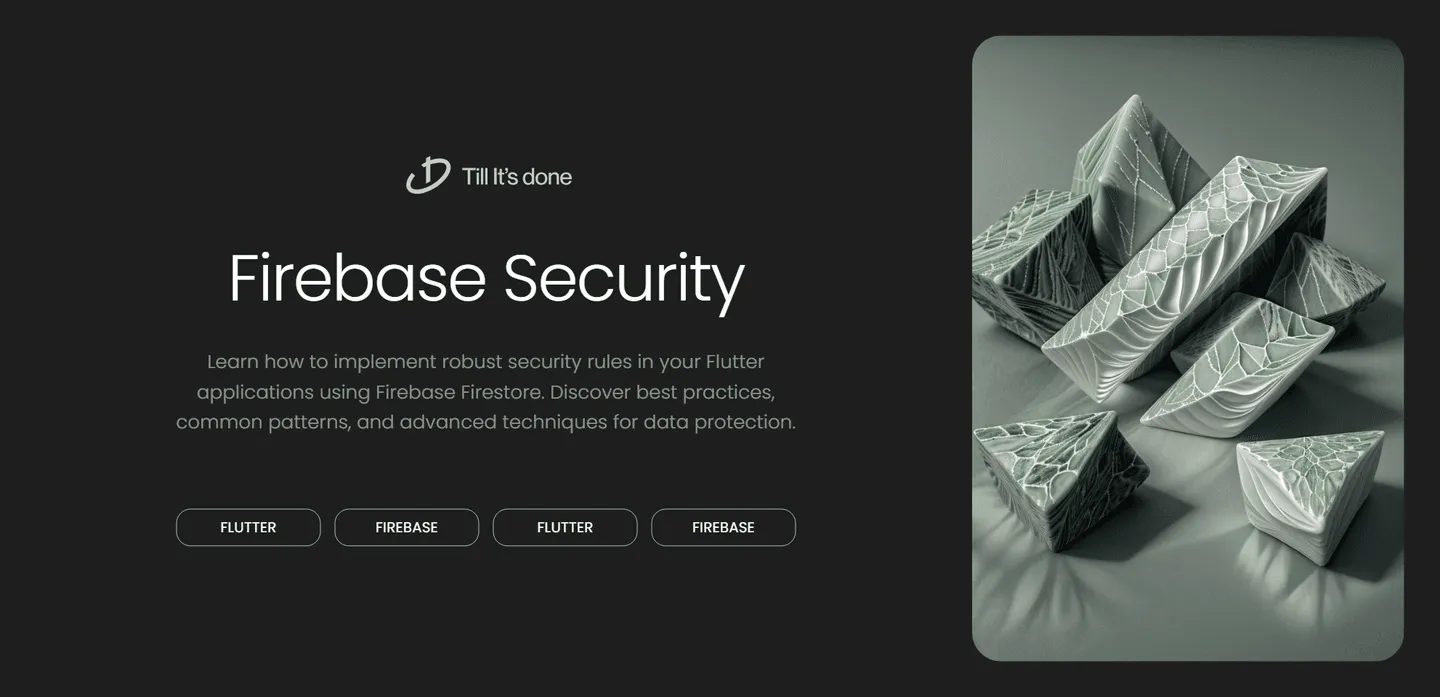
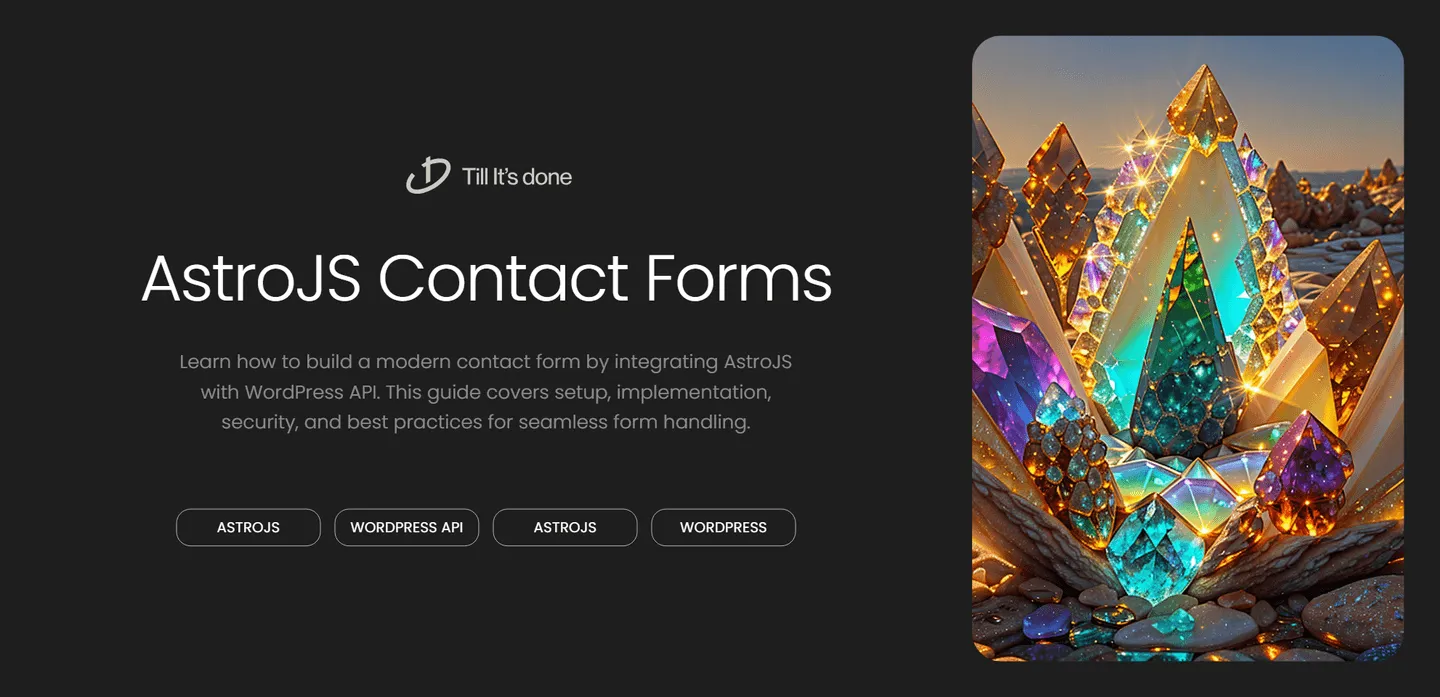
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.