- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
EN
TH
rick@tillitsdone.com
+66824564755
Leveraging Test-Driven Development (TDD) with Jest
Master Test-Driven Development using Jest framework.
Learn essential TDD cycles, best practices, and advanced patterns with practical examples for writing maintainable JavaScript tests.
Learn essential TDD cycles, best practices, and advanced patterns with practical examples for writing maintainable JavaScript tests.
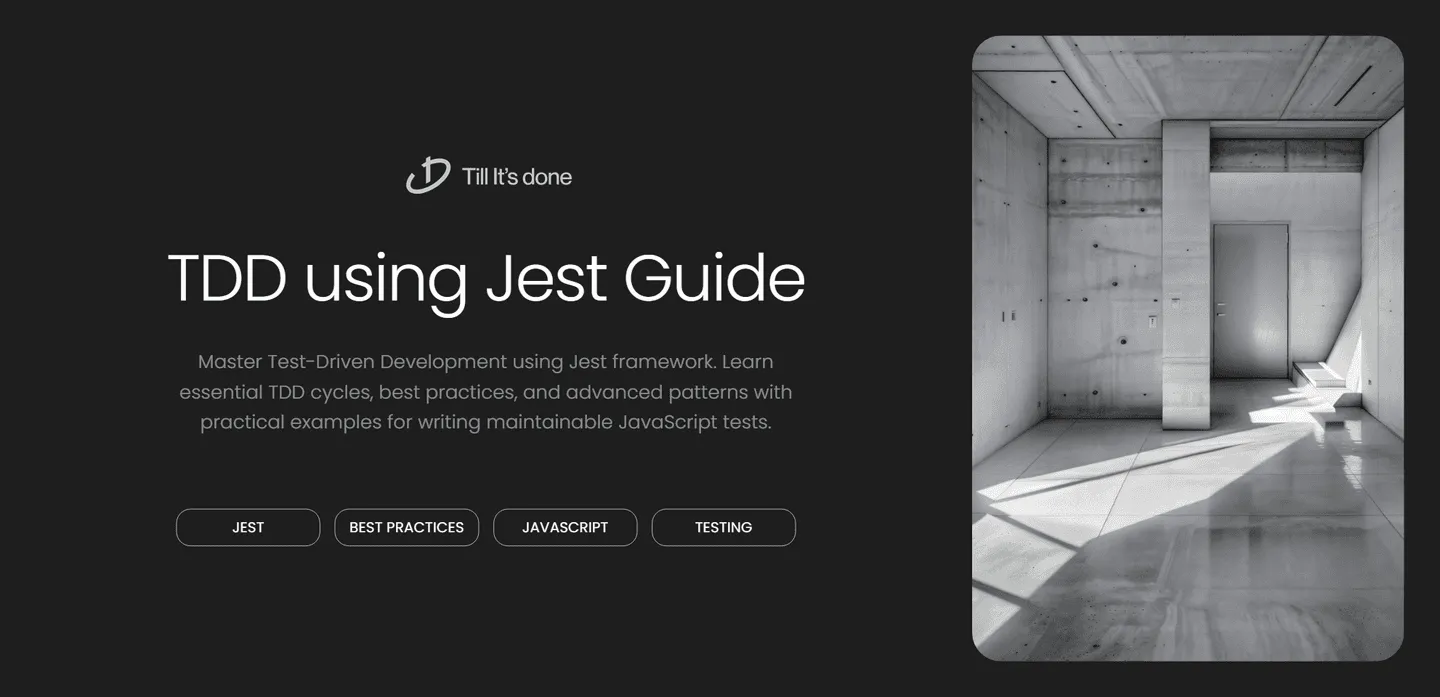
Leveraging Test-Driven Development (TDD) with Jest
Test-Driven Development has revolutionized how we approach software development. In this guide, we’ll explore how to effectively implement TDD using Jest, one of the most popular testing frameworks in the JavaScript ecosystem.
Understanding TDD Fundamentals
The core TDD cycle consists of three simple steps: Red (write a failing test), Green (make the test pass), and Refactor (improve the code while maintaining functionality).
Getting Started with Jest TDD
First, let’s set up a simple project:
npm init -ynpm install --save-dev jest
Update your package.json:
{ "scripts": { "test": "jest --watchAll" }}
Practical TDD Example
Let’s create a simple calculator module using TDD:
describe('Calculator', () => { test('should add two numbers correctly', () => { expect(calculator.add(2, 3)).toBe(5); });});
Now implement the calculator:
const calculator = { add: (a, b) => a + b,};
module.exports = calculator;
Best Practices for TDD with Jest
- Keep tests focused and atomic
- Use descriptive test names
- Follow the Arrange-Act-Assert pattern
- Maintain test isolation
- Write the minimum code needed to pass tests
Advanced TDD Patterns
describe('Advanced Calculator', () => { beforeEach(() => { // Setup });
test('should handle complex calculations', () => { const result = calculator.evaluate('2 + 3 * 4'); expect(result).toBe(14); });});
Discover our top articles, selected to support the growth of your business.
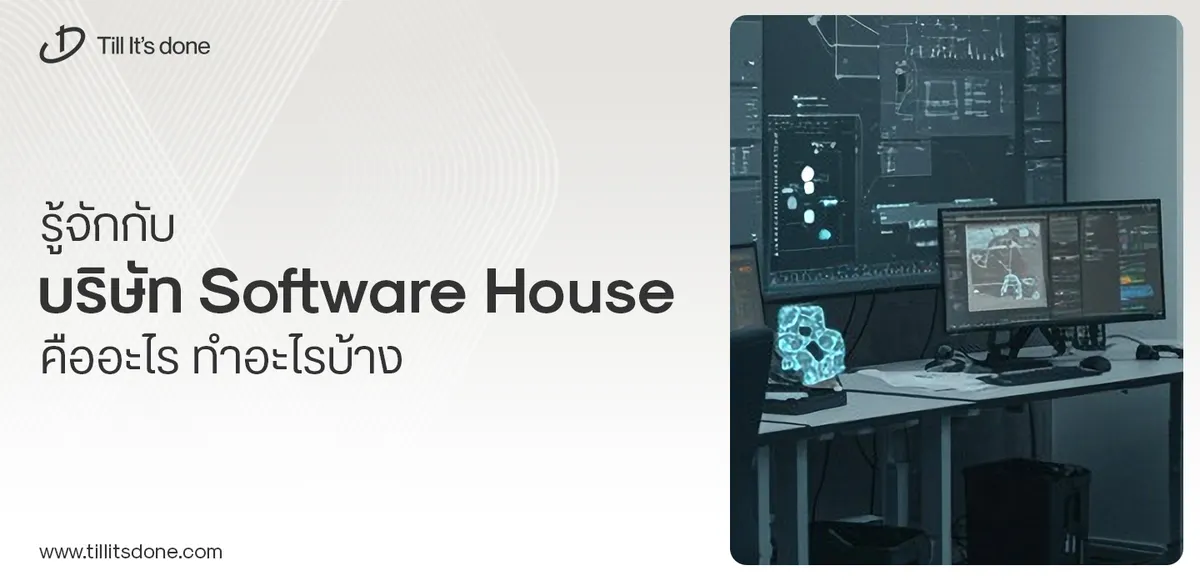
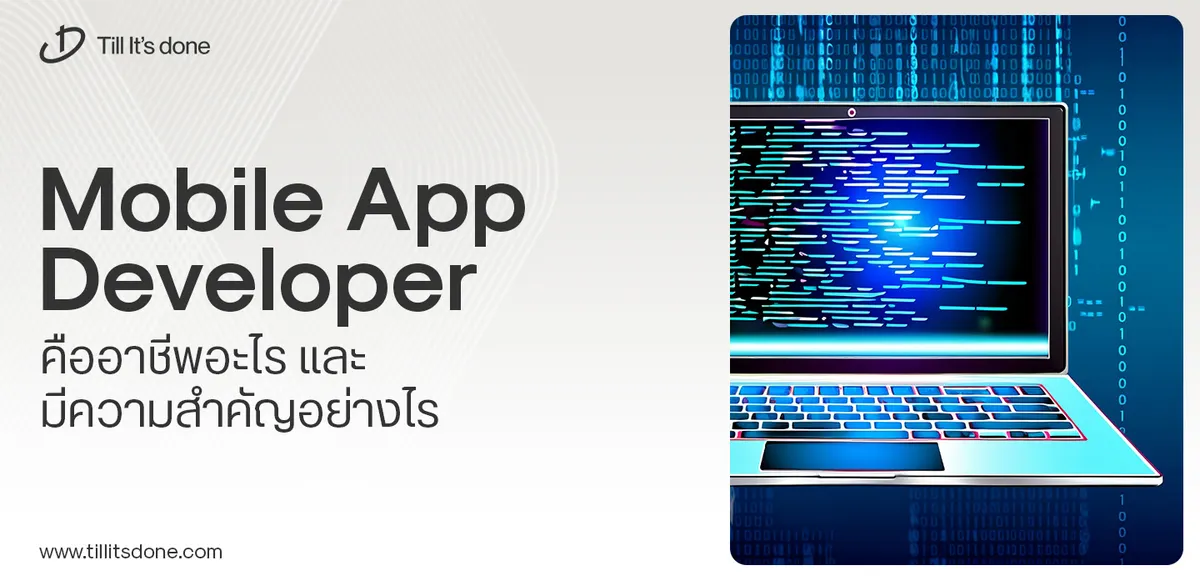
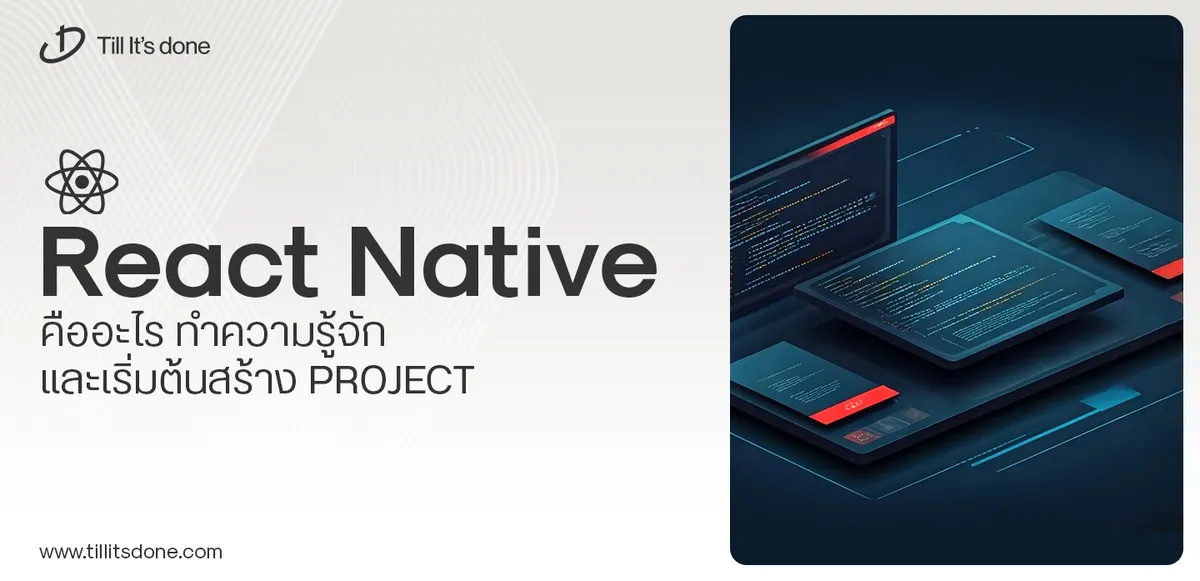
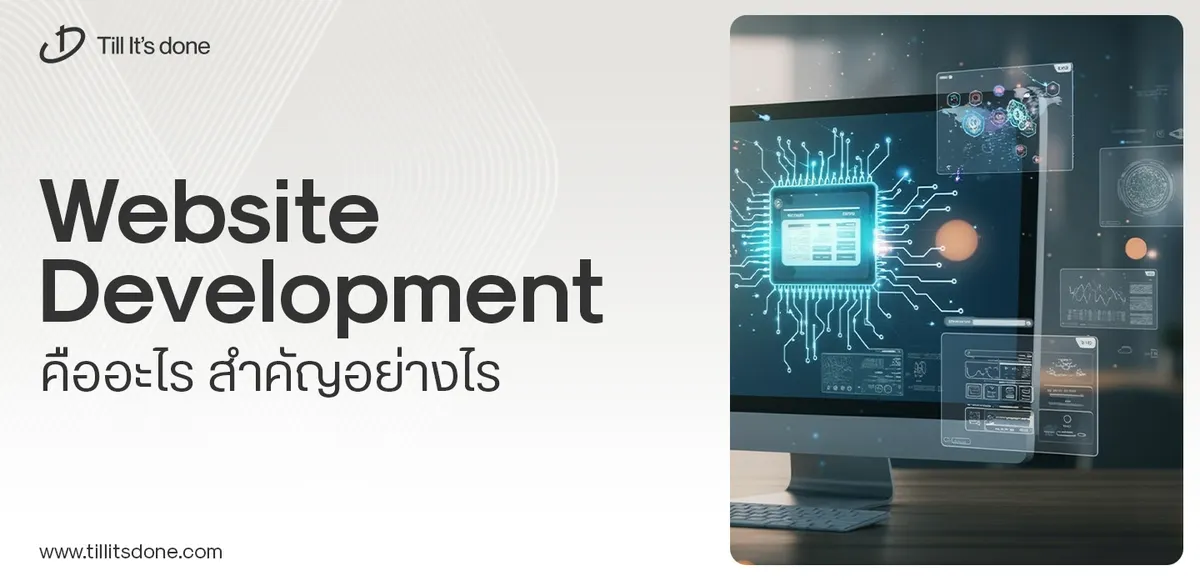
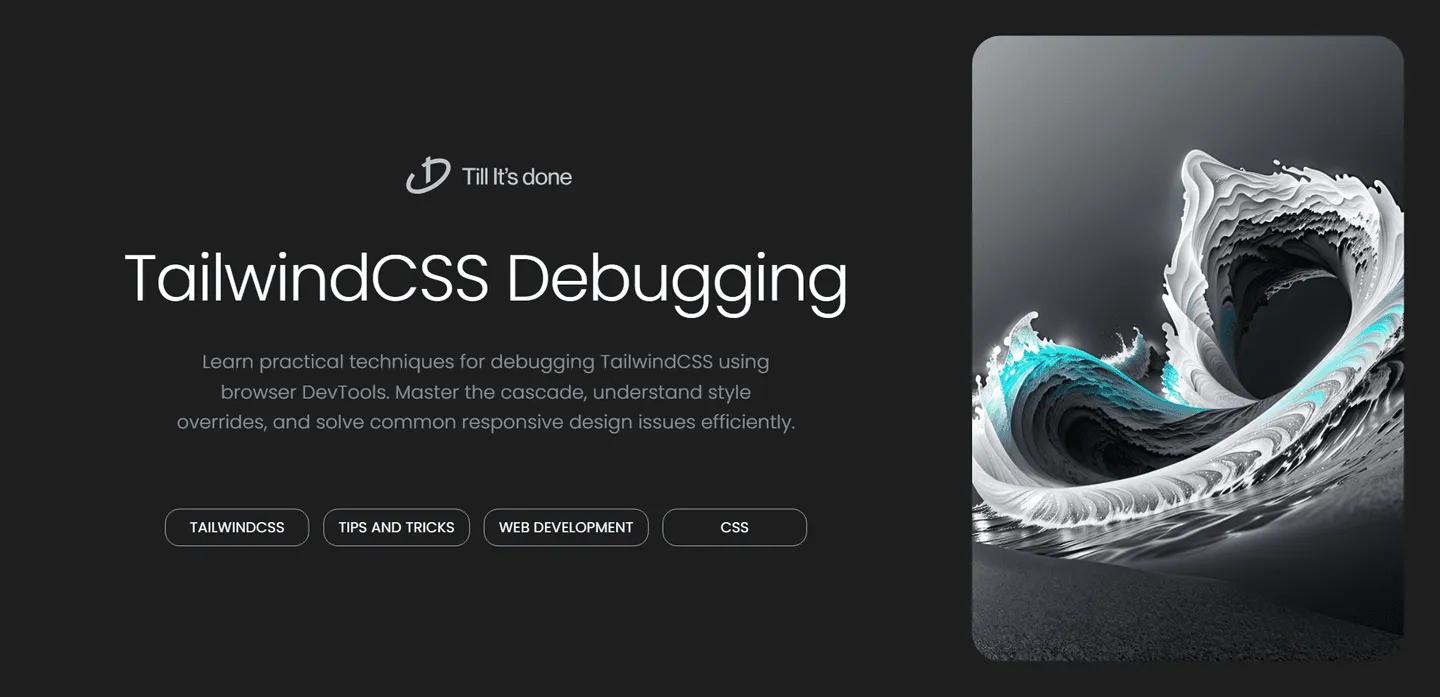
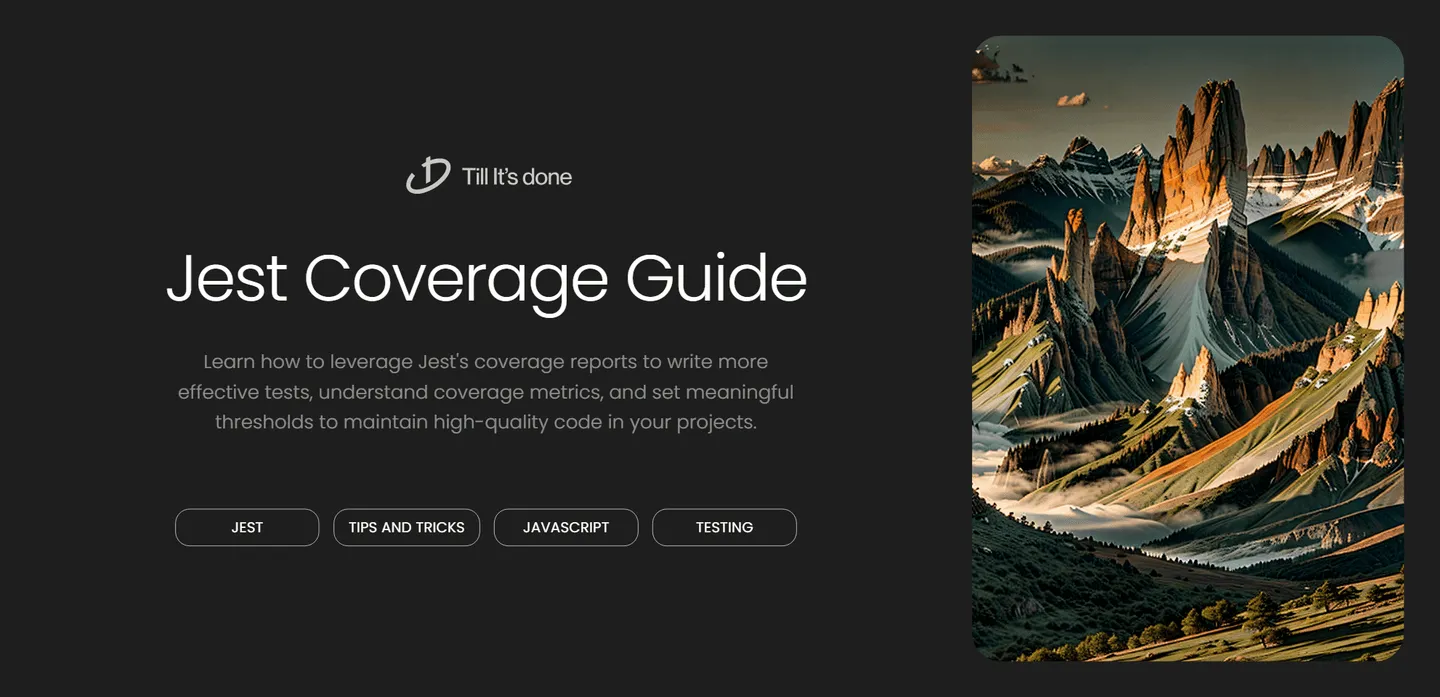
Talk with CEO
Ready to bring your web/app to life or boost your team with expert Thai developers?
Contact us today to discuss your needs, and let’s create tailored solutions to achieve your goals. We’re here to help at every step!
🖐️ Contact us 196 Articles
Explore Popular JavaScript library for building user interfaces with a component-based architecture.
160 Articles
Explore UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase.
144 Articles
Explore JavaScript runtime for building scalable, high-performance server-side applications.
58 Articles
Explore React framework enabling server-side rendering and static site generation for optimized performance.
38 Articles
Explore Utility-first CSS framework for rapid UI development.
36 Articles
Explore Superset of JavaScript adding static types for improved code quality and maintainability.
126 Articles
Explore Programming language known for its simplicity, concurrency model, and performance.
67 Articles
Explore Astro is an all-in-one web framework. It includes everything you need to create a website, built-in.
38 Articles
Explore Versatile testing framework for JavaScript applications supporting various test types.
3 Articles
Explore 2 Articles
Explore 1 Articles
Explore 1 Articles
Explore 337 Articles
Explore CSS3 is the latest version of Cascading Style Sheets, offering advanced styling features like animations, transitions, shadows, gradients, and responsive design.
Let's keep in Touch
Thank you for your interest in Tillitsdone! Whether you have a question about our services, want to discuss a potential project, or simply want to say hello, we're here and ready to assist you.
We'll be right here with you every step of the way.
We'll be right here with you every step of the way.
Contact Information
rick@tillitsdone.com+66824564755
Address
9 Phahonyothin Rd, Khlong Nueng, Khlong Luang District, Pathum Thani, Bangkok Thailand
Social media
FacebookInstagramLinkedIn
We anticipate your communication and look forward to discussing how we can contribute to your business's success.
We'll be here, prepared to commence this promising collaboration.
We'll be here, prepared to commence this promising collaboration.
Frequently Asked Questions
Explore frequently asked questions about our products and services.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.