- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Test-Driven Development (TDD) in Flutter Guide

Test-Driven Development (TDD) in Flutter: A Practical Guide
Test-Driven Development (TDD) is more than just a development practice – it’s a mindset that can transform how we build Flutter applications. In this guide, we’ll explore how TDD can make your Flutter development process more robust and reliable.
Understanding TDD in Flutter Context
At its core, TDD follows a simple yet powerful cycle: Red (write a failing test), Green (make the test pass), and Refactor (improve the code while keeping tests green). In Flutter, this approach becomes particularly valuable when dealing with complex UI components and business logic.
Setting Up Your Flutter Testing Environment
Before diving into TDD, ensure your Flutter project is properly configured for testing. Your pubspec.yaml
should include the necessary testing dependencies:
dev_dependencies: flutter_test: sdk: flutter mockito: ^5.4.0 build_runner: ^2.4.0
Practical TDD Example: Building a Counter Widget
Let’s walk through a practical example of implementing a counter widget using TDD. We’ll start with a simple test:
void main() { testWidgets('Counter increments when plus button is tapped', (WidgetTester tester) async { await tester.pumpWidget(const CounterWidget()); expect(find.text('0'), findsOneWidget); await tester.tap(find.byIcon(Icons.add)); await tester.pump(); expect(find.text('1'), findsOneWidget); });}
Best Practices for Flutter TDD
- Start with Widget Tests: They’re faster than integration tests and more comprehensive than unit tests.
- Mock Dependencies: Use Mockito to isolate components and test edge cases.
- Test User Interactions: Cover all possible user interactions with your widgets.
- Keep Tests Focused: Each test should verify one specific behavior.
Advanced TDD Patterns
The Repository Pattern
abstract class Repository { Future<List<Item>> getItems();}
class MockRepository extends Mock implements Repository {}
State Management Testing
testWidgets('Provider updates state correctly', (tester) async { await tester.pumpWidget( ChangeNotifierProvider( create: (context) => CounterProvider(), child: const MyApp(), ), );});
Conclusion
TDD in Flutter might seem like extra work initially, but it pays off in maintainability, reliability, and confidence in your code. Start small, be consistent, and gradually build up your testing expertise.
Tags: Flutter, Testing, TDD, Development, Mobile Development
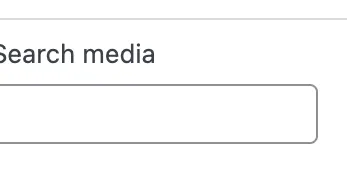





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.