- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Writing Table-Driven Tests Using Testify in Go
Discover best practices, patterns, and advanced techniques for better testing.
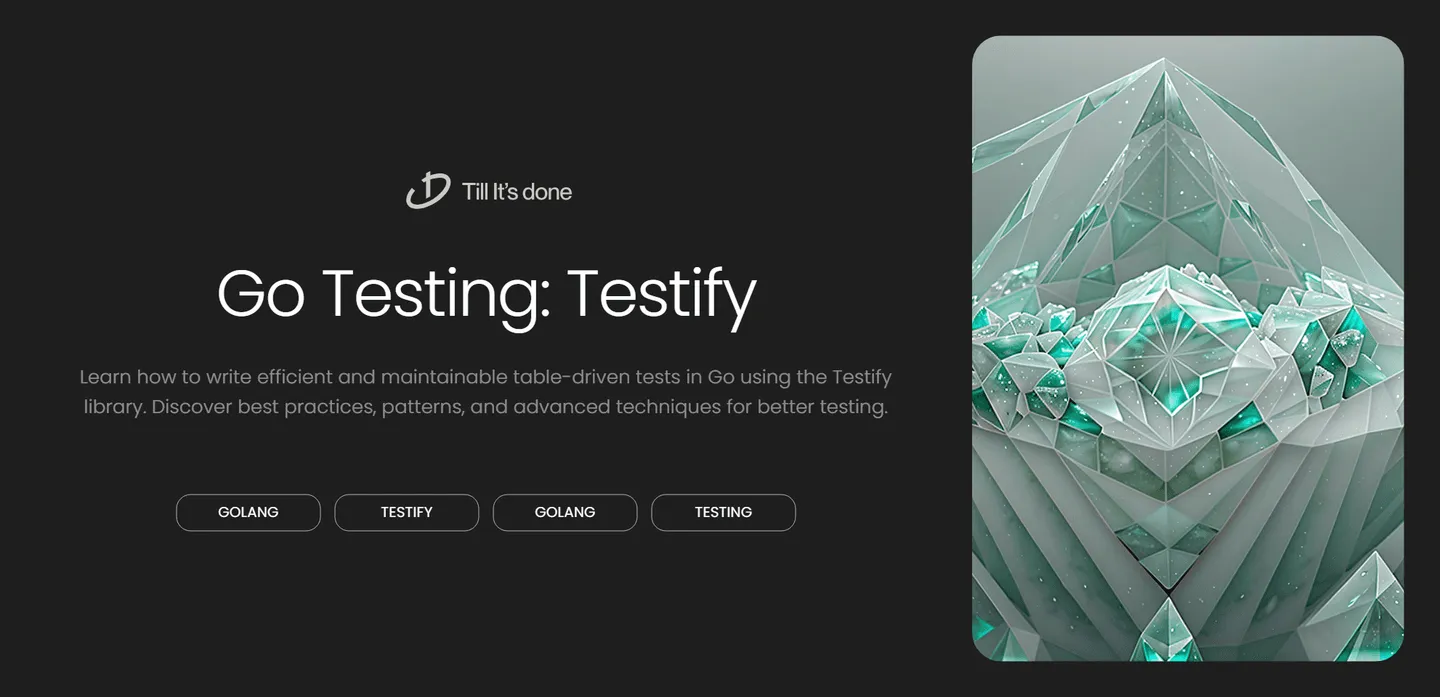
Writing Table-Driven Tests Using Testify in Golang
Table-driven tests are a powerful pattern in Go that allows you to write more maintainable and comprehensive test cases. When combined with the popular Testify library, you can create even more expressive and readable tests. Let’s dive into how to leverage this powerful combination.
Understanding Table-Driven Tests
Think of table-driven tests as a spreadsheet where each row represents a different test scenario. Instead of writing separate test functions for each case, you define a single test function that iterates through multiple test cases. This approach reduces code duplication and makes it easier to add new test cases.
Setting Up Testify
First, you’ll need to install Testify:
go get github.com/stretchr/testify
Let’s say we have a simple calculator package with an Add
function that we want to test:
package calculator
func Add(a, b int) int { return a + b}
Writing Table-Driven Tests with Testify
Here’s how we can write table-driven tests using Testify:
package calculator
import ( "testing" "github.com/stretchr/testify/assert")
func TestAdd(t *testing.T) { tests := []struct { name string a, b int expected int }{ {"positive_numbers", 2, 3, 5}, {"negative_numbers", -2, -3, -5}, {"zero_sum", 0, 0, 0}, {"positive_negative", -5, 5, 0}, }
for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { result := Add(tt.a, tt.b) assert.Equal(t, tt.expected, result, "Add(%d, %d) should be %d", tt.a, tt.b, tt.expected) }) }}
Best Practices
-
Descriptive Test Names: Give each test case a clear, descriptive name that indicates what’s being tested.
-
Edge Cases: Include edge cases in your test table to ensure your function handles all scenarios correctly.
-
Organized Structure: Group related test cases together and use comments to separate different categories of tests.
-
Clear Failure Messages: Use Testify’s assertion messages to provide helpful debugging information when tests fail.
Advanced Techniques
You can enhance your table-driven tests with more complex scenarios:
func TestComplexCalculation(t *testing.T) { tests := []struct { name string input []int expected int shouldError bool }{ { name: "valid_input", input: []int{1, 2, 3}, expected: 6, shouldError: false, }, { name: "empty_input", input: []int{}, expected: 0, shouldError: true, }, }
for _, tt := range tests { t.Run(tt.name, func(t *testing.T) { result, err := ComplexCalculation(tt.input) if tt.shouldError { assert.Error(t, err) } else { assert.NoError(t, err) assert.Equal(t, tt.expected, result) } }) }}
Conclusion
Table-driven tests with Testify provide a robust way to test your Go code. They help you write more maintainable tests, catch edge cases, and provide clear feedback when things go wrong. Start using this pattern in your projects, and you’ll see how it makes testing more enjoyable and efficient.
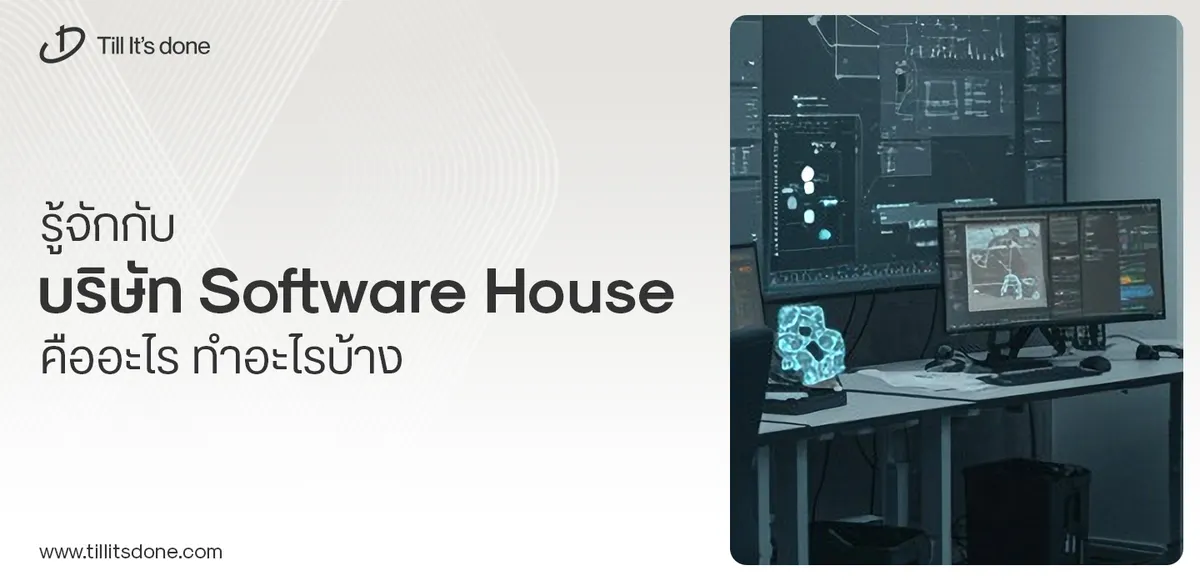
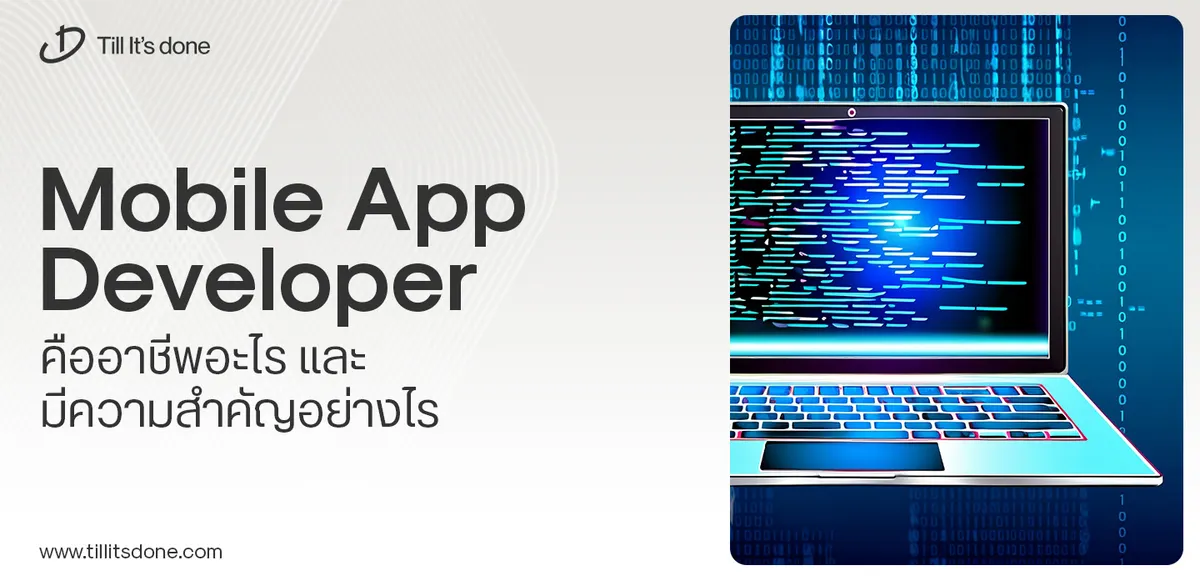
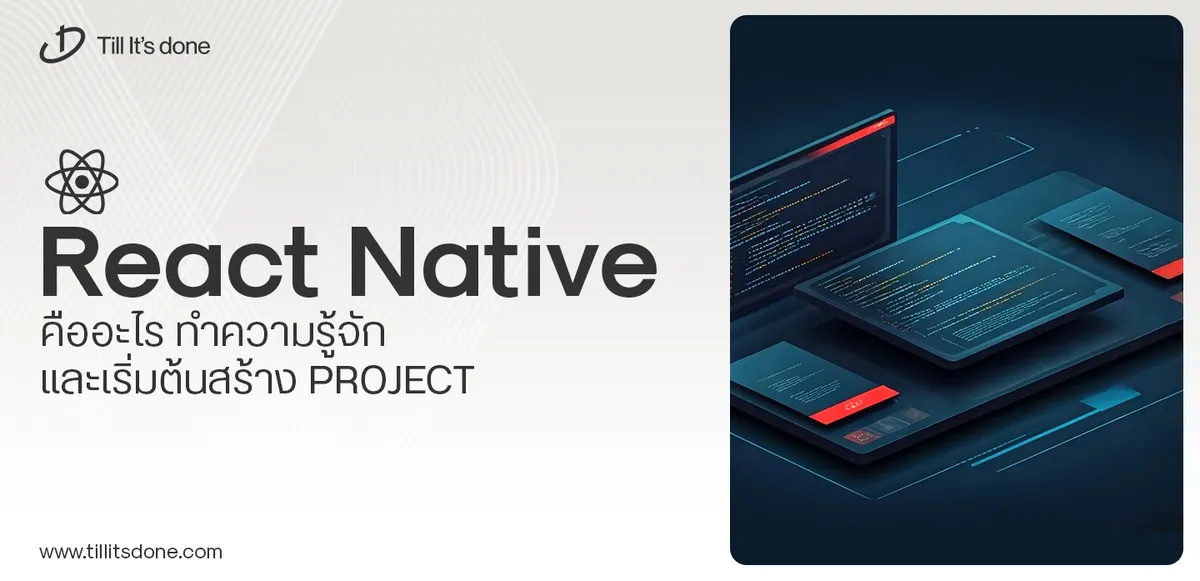
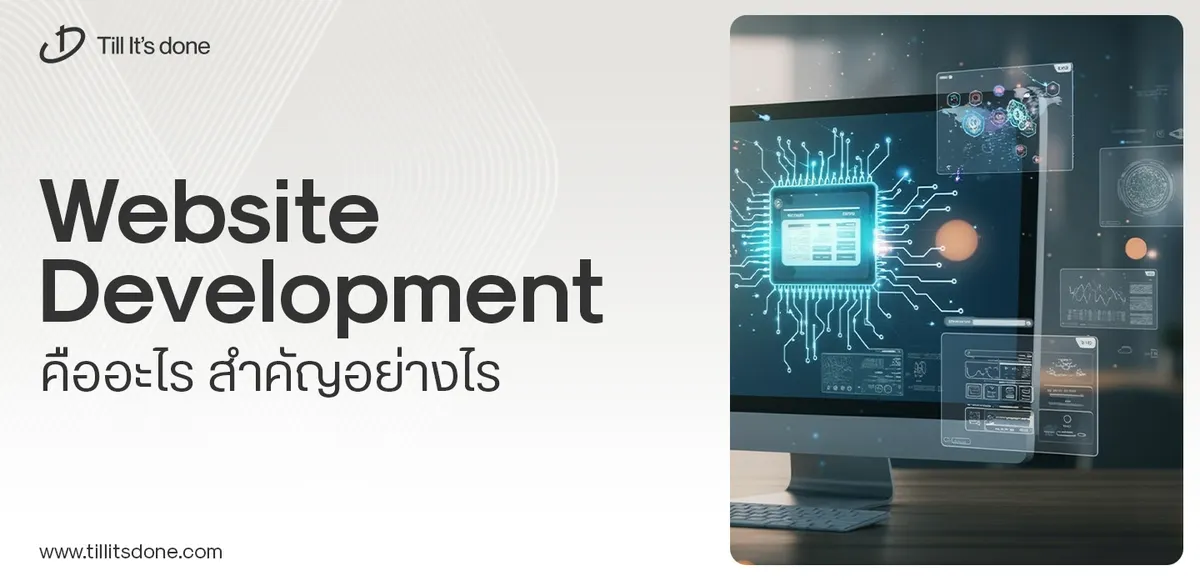
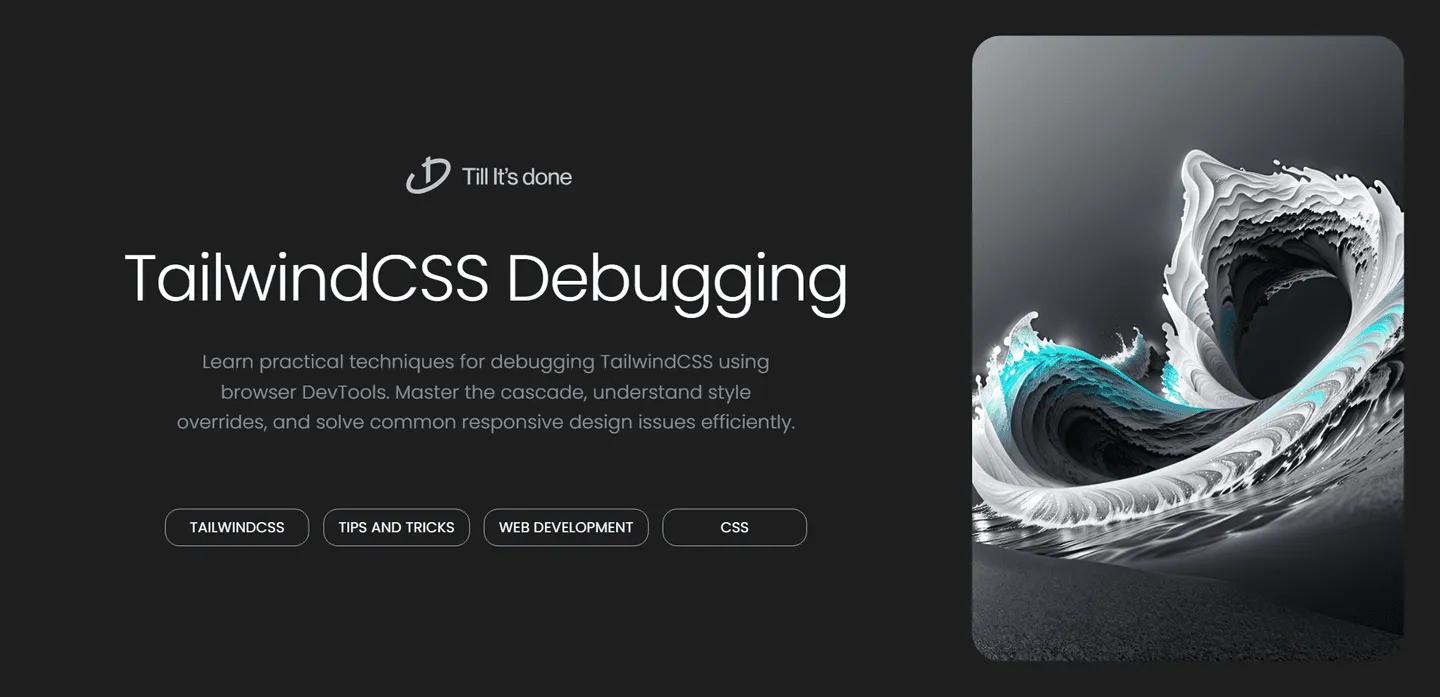
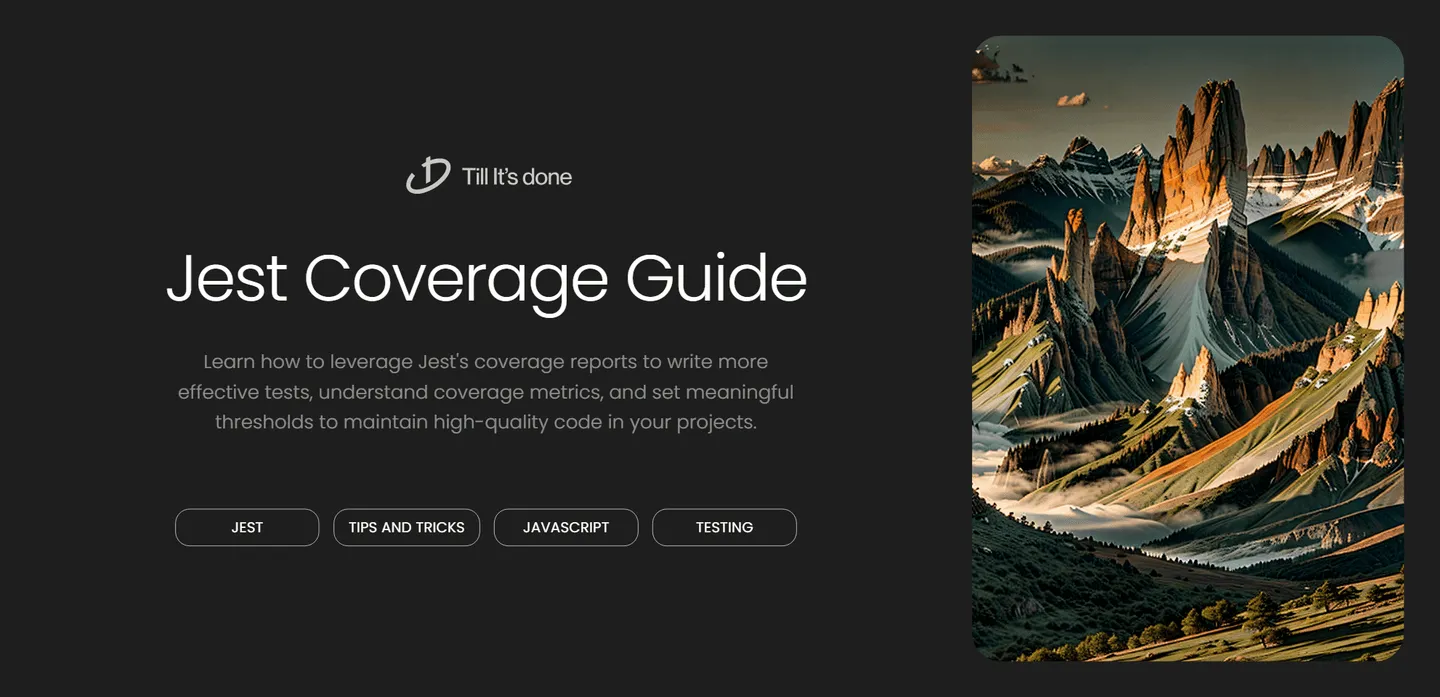
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.