- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Handling Socket.IO Events in Node.js Guide
This beginner-friendly guide covers basic setup, event patterns, rooms, and best practices for real-time features.

Handling Socket.IO Events in Node.js: A Beginner’s Guide
Real-time communication has become essential in modern web applications. Whether you’re building a chat application, a live dashboard, or a multiplayer game, Socket.IO with Node.js provides a robust solution for implementing real-time features. Let’s dive into how to handle Socket.IO events effectively.
Understanding Socket.IO Events
At its core, Socket.IO uses an event-driven architecture. Think of it as a two-way radio communication system where both the server and client can broadcast and listen for messages. This bidirectional communication happens through events, making it perfect for real-time applications.
Setting Up Your First Socket.IO Server
Before diving into event handling, let’s set up a basic Socket.IO server:
const express = require('express');const app = express();const http = require('http').createServer(app);const io = require('socket.io')(http);
io.on('connection', (socket) => { console.log('A user connected');});
http.listen(3000, () => { console.log('Server running on port 3000');});
Common Event Handling Patterns
Basic Events
The most straightforward way to handle events is using the on
and emit
methods:
// Server-sideio.on('connection', (socket) => { socket.on('chat message', (msg) => { io.emit('chat message', msg); });});
// Client-sidesocket.emit('chat message', 'Hello World!');
Custom Events
You can create custom events for specific features:
socket.on('user typing', (username) => { socket.broadcast.emit('user typing', username);});
socket.on('stop typing', (username) => { socket.broadcast.emit('stop typing', username);});
Room-Based Events
Rooms are useful for grouping sockets and broadcasting to specific groups:
socket.on('join room', (room) => { socket.join(room); io.to(room).emit('user joined', 'A new user joined the room');});
Best Practices
- Always handle disconnection events
- Implement error handling for failed connections
- Use namespaces for different features
- Validate data before processing
- Consider implementing reconnection logic
Debugging Tips
When debugging Socket.IO applications:
- Use the built-in debug mode
- Monitor server-side logs
- Check browser console for client-side issues
- Test with multiple clients
Conclusion
Socket.IO provides a powerful way to handle real-time events in Node.js applications. By understanding these basic concepts and patterns, you’re well-equipped to build robust real-time features in your applications.
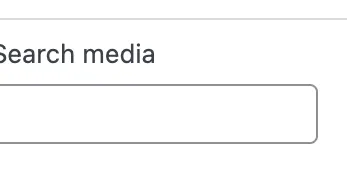





Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.