- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Using Lodash to Simplify Complex JS Code
Learn practical examples for cleaner, more efficient code.
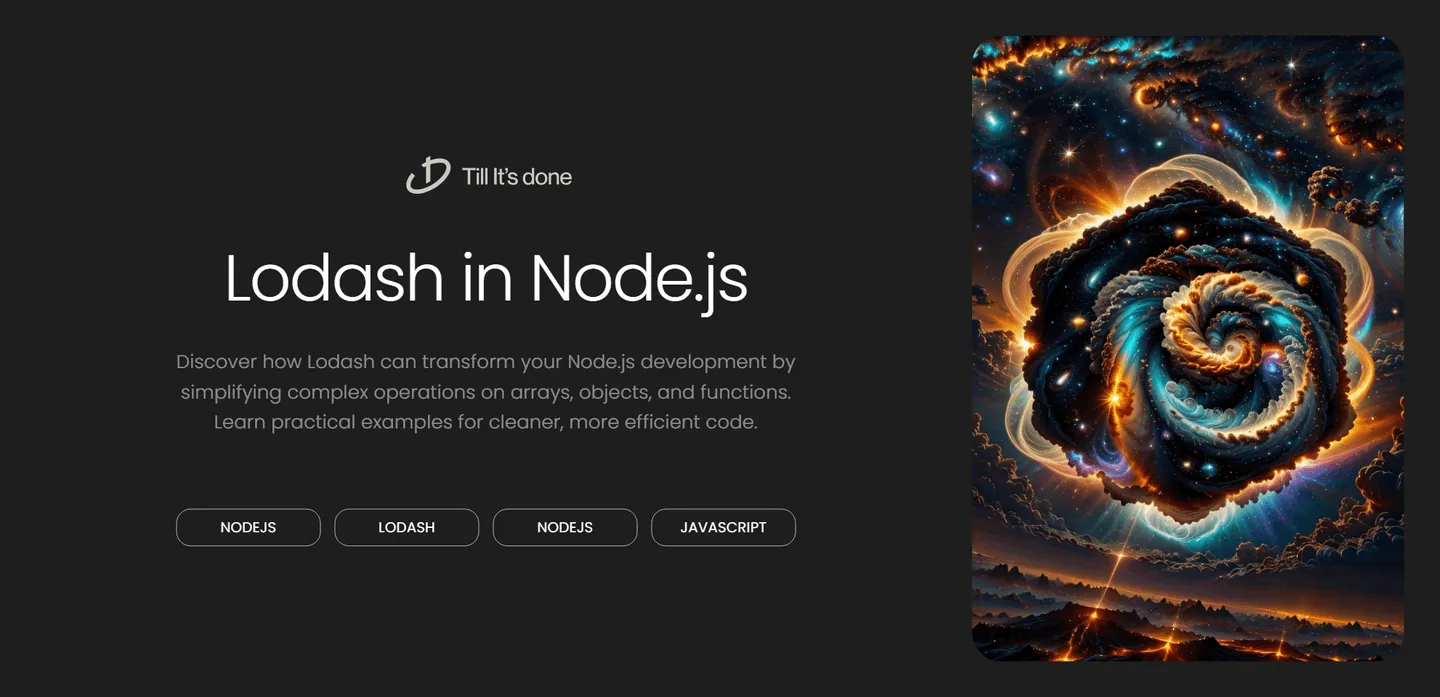
Using Lodash to Simplify Complex JavaScript Code in Node.js
JavaScript development can sometimes feel like navigating through a maze of complex data structures and operations. Enter Lodash – your trusty companion in the Node.js ecosystem that makes handling arrays, objects, and functions a breeze. Let’s dive into how this powerful utility library can transform your code from complex to elegantly simple.
The Power of Collection Manipulation
One of Lodash’s strongest suits is how it handles collections. Think about those times when you needed to filter, map, and reduce arrays in a single operation. Instead of chaining multiple array methods and keeping track of intermediate states, Lodash provides elegant solutions.
const _ = require('lodash');
// Without Lodashconst users = [ { name: 'John', age: 30, active: true }, { name: 'Jane', age: 28, active: false }, { name: 'Mike', age: 32, active: true }];
const activeAdults = users .filter(user => user.active) .map(user => ({ fullName: user.name, yearOfBirth: new Date().getFullYear() - user.age }));
// With Lodashconst activeAdultsLodash = _.chain(users) .filter('active') .map(user => ({ fullName: user.name, yearOfBirth: new Date().getFullYear() - user.age })) .value();
Deep Object Operations Made Simple
Working with deeply nested objects in JavaScript can be tricky. Lodash provides intuitive methods for accessing, setting, and manipulating nested properties without the worry of null references.
const complexObject = { user: { profile: { details: { address: { street: '123 Main St' } } } }};
// Without Lodash - prone to errorsconst street = complexObject?.user?.profile?.details?.address?.street;
// With Lodash - safe and cleanconst streetLodash = _.get(complexObject, 'user.profile.details.address.street', 'Not found');
Function Utilities That Save the Day
Performance optimization is crucial in Node.js applications. Lodash offers excellent function utilities like debounce and throttle that help manage function calls in high-frequency events.
const saveToDatabase = _.debounce(async (data) => { // Database operation here}, 1000);
// This will only execute once after 1 second of inactivitysaveToDatabase(userData);saveToDatabase(userData);saveToDatabase(userData);
Conclusion
Lodash is more than just a utility library – it’s a Swiss Army knife for JavaScript developers. By incorporating Lodash into your Node.js projects, you can write more maintainable, readable, and efficient code. The beauty of Lodash lies not just in what it can do, but in how it makes complex operations feel natural and straightforward.
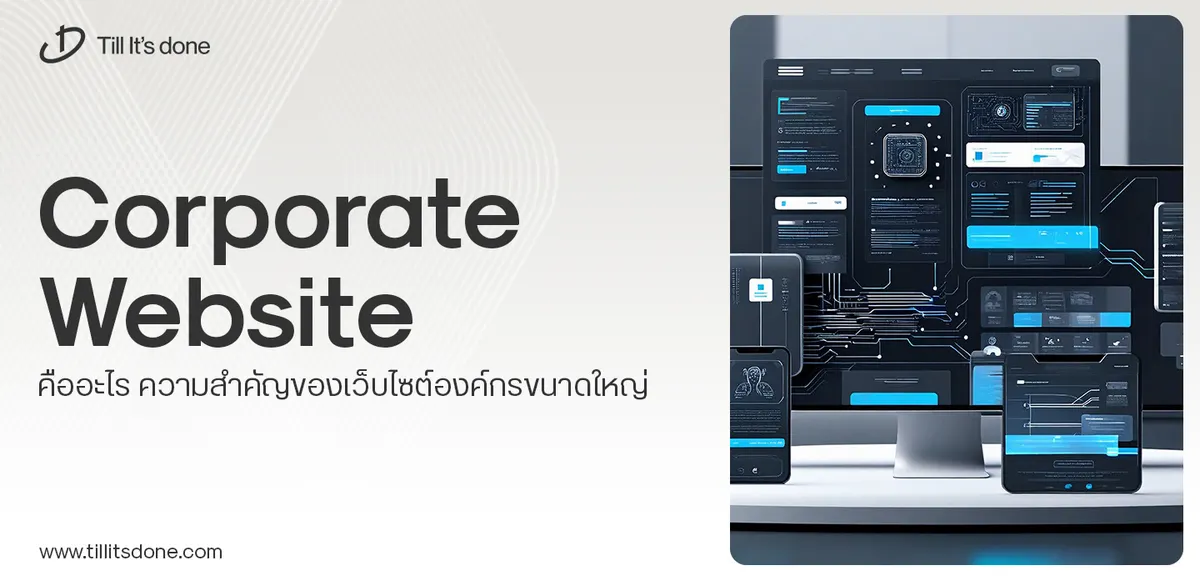
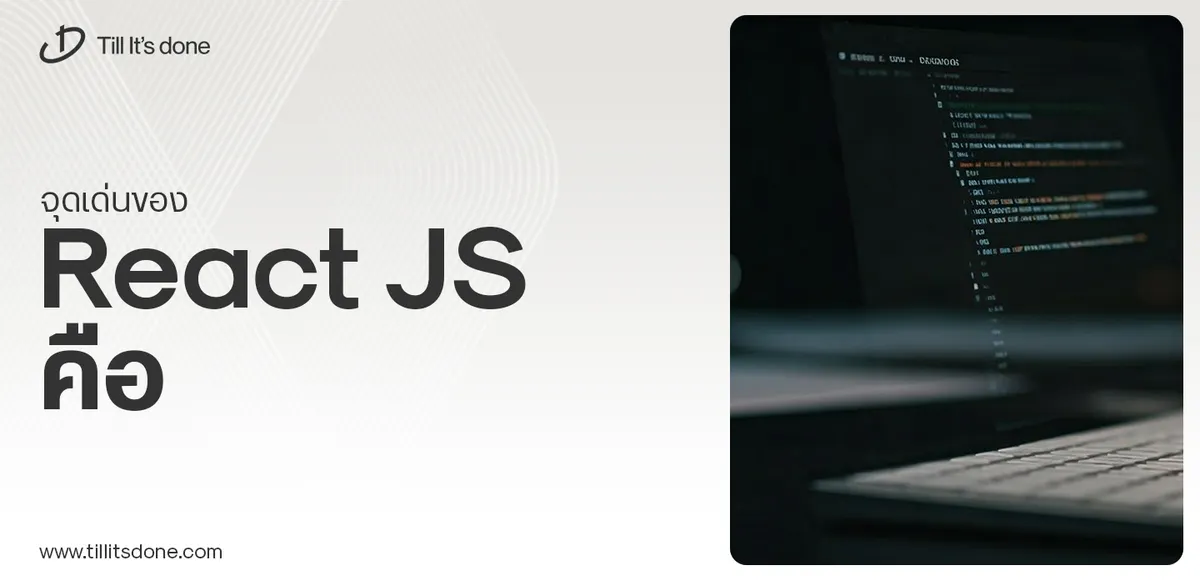
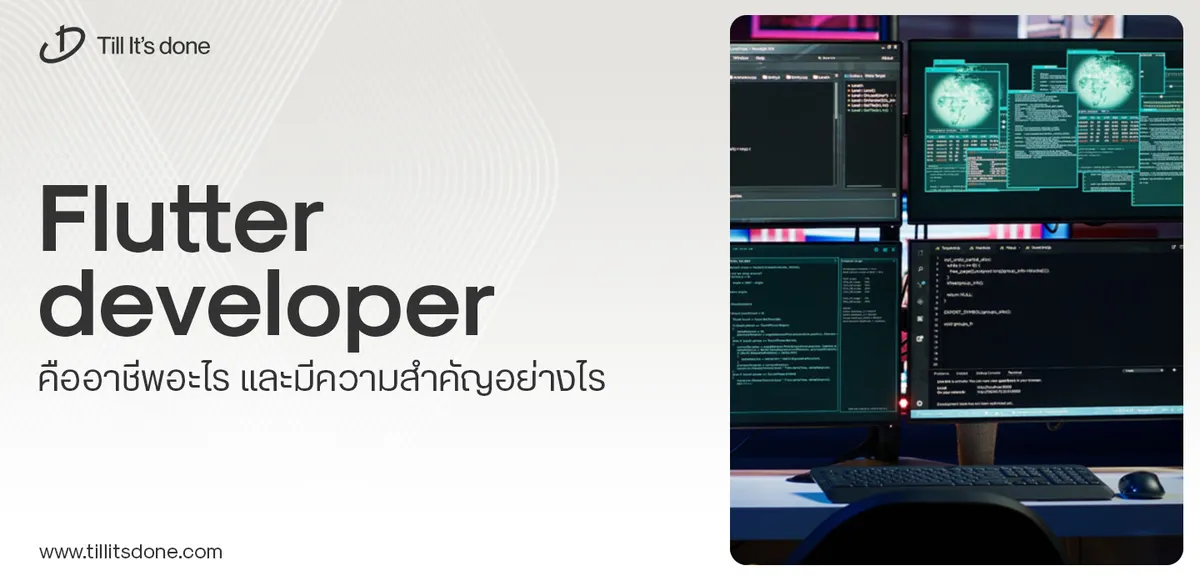
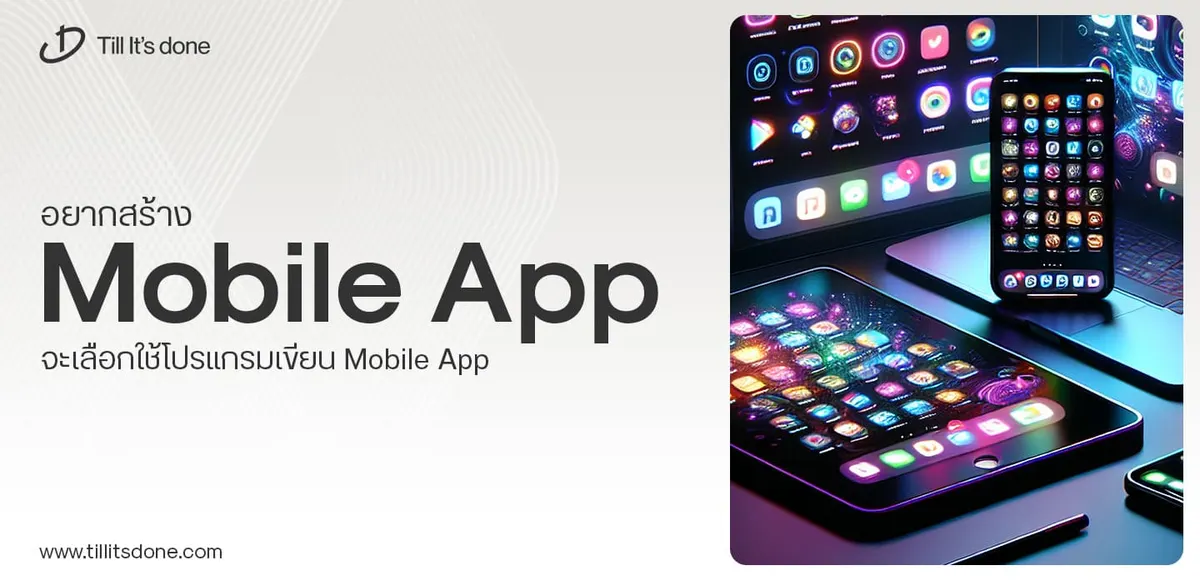
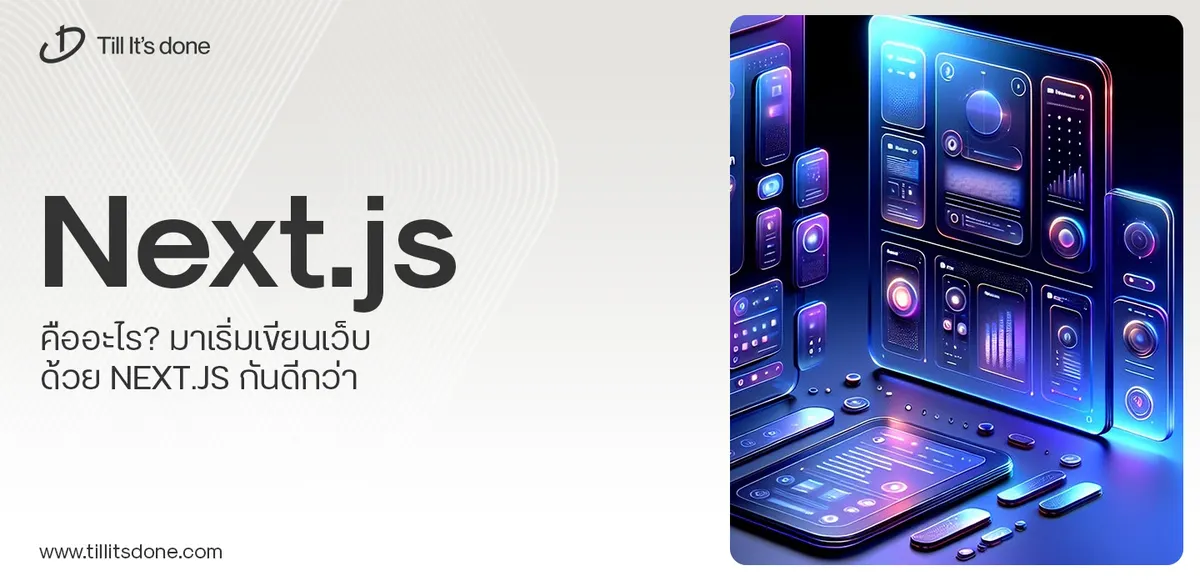
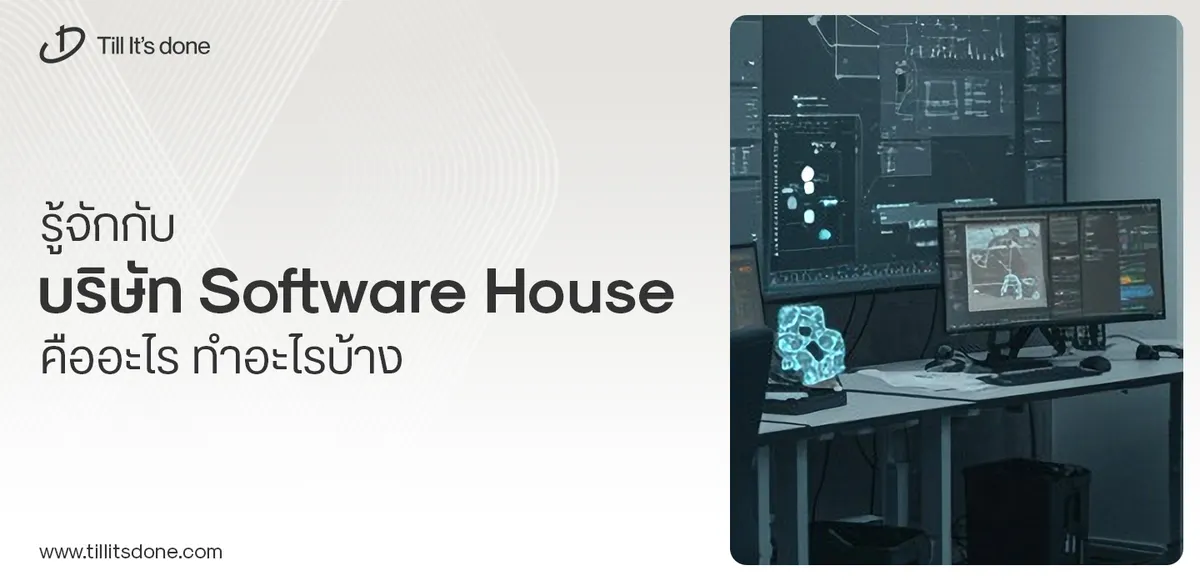
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.