- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Setting Up a ReactJS Project from Scratch
This guide covers essential setup steps, dependencies, configuration, and best practices for beginners.
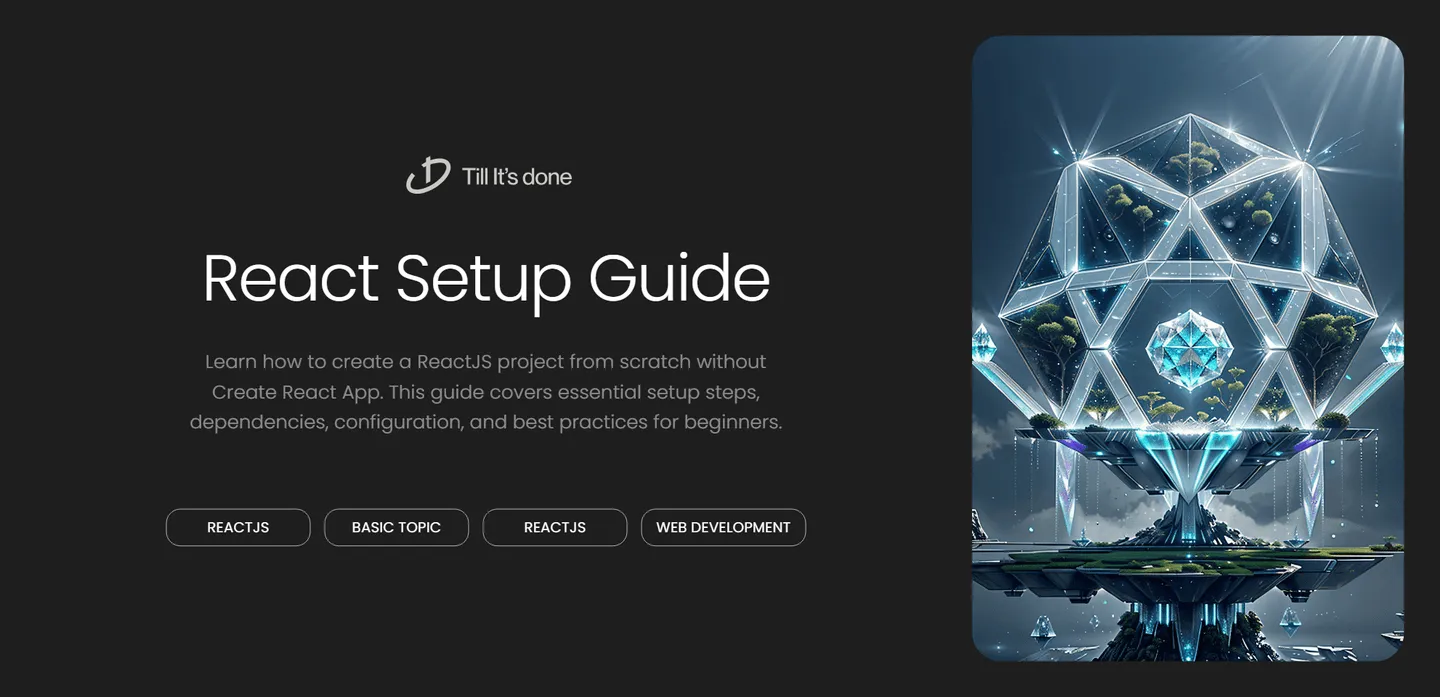
Setting Up a ReactJS Project from Scratch: A Beginner’s Guide
Getting started with ReactJS can feel overwhelming at first, but I’m here to break it down into simple, manageable steps. Today, I’ll walk you through creating a React project from the ground up, without using Create React App. This hands-on approach will give you a deeper understanding of how everything fits together.
Prerequisites
Before we dive in, make sure you have:
- Node.js installed on your computer
- A code editor (I personally love VS Code)
- Basic knowledge of JavaScript
- Terminal/Command Prompt familiarity
Step-by-Step Setup Process
1. Creating Your Project Directory
First, let’s create a new directory for our project and navigate into it. Open your terminal and run:
mkdir my-react-projectcd my-react-project
2. Initializing the Project
Initialize your project with npm:
npm init -y
This creates a package.json file with default values. Don’t worry - we can modify these later!
3. Installing Essential Dependencies
Now for the exciting part - installing React and its core dependencies:
npm install react react-dom
For development dependencies:
npm install --save-dev @babel/core @babel/preset-react @babel/preset-envnpm install --save-dev webpack webpack-cli webpack-dev-servernpm install --save-dev babel-loader html-webpack-plugin
4. Setting Up Configuration Files
Create a .babelrc
file:
{ "presets": ["@babel/preset-react", "@babel/preset-env"]}
Create webpack.config.js
:
const HtmlWebpackPlugin = require('html-webpack-plugin');const path = require('path');
module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: 'babel-loader', }, ], }, plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], devServer: { static: { directory: path.join(__dirname, 'public'), }, port: 3000, open: true, },};
5. Creating Source Files
Create a src
folder with these essential files:
src/index.html
:
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>React From Scratch</title></head><body> <div id="root"></div></body></html>
src/index.js
:
import React from 'react';import ReactDOM from 'react-dom/client';import App from './App';
const root = ReactDOM.createRoot(document.getElementById('root'));root.render( <React.StrictMode> <App /> </React.StrictMode>);
src/App.js
:
import React from 'react';
function App() { return ( <div> <h1>Hello from React!</h1> </div> );}
export default App;
6. Adding NPM Scripts
Update your package.json
with these scripts:
{ "scripts": { "start": "webpack serve --mode development", "build": "webpack --mode production" }}
7. Running Your Project
Finally, start your development server:
npm start
Visit http://localhost:3000
to see your React app in action!
And there you have it! You’ve successfully set up a React project from scratch. This foundation gives you complete control over your build process and helps you understand what’s happening under the hood. Remember, while this setup works great for learning, for larger projects you might want to consider using Create React App or Next.js for a more feature-rich development environment.
Happy coding! 🚀
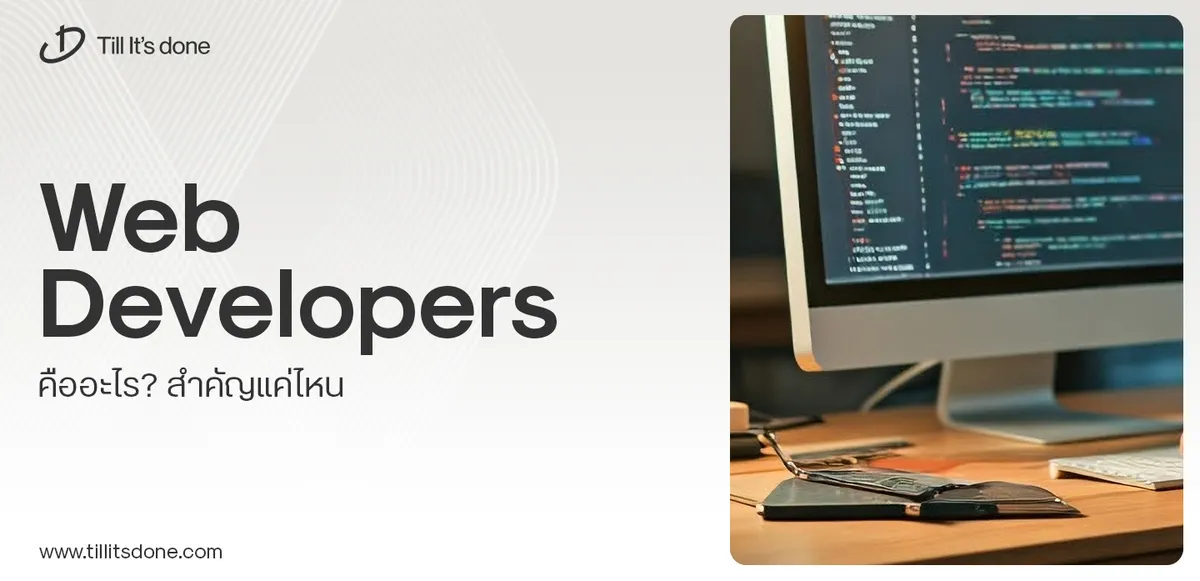
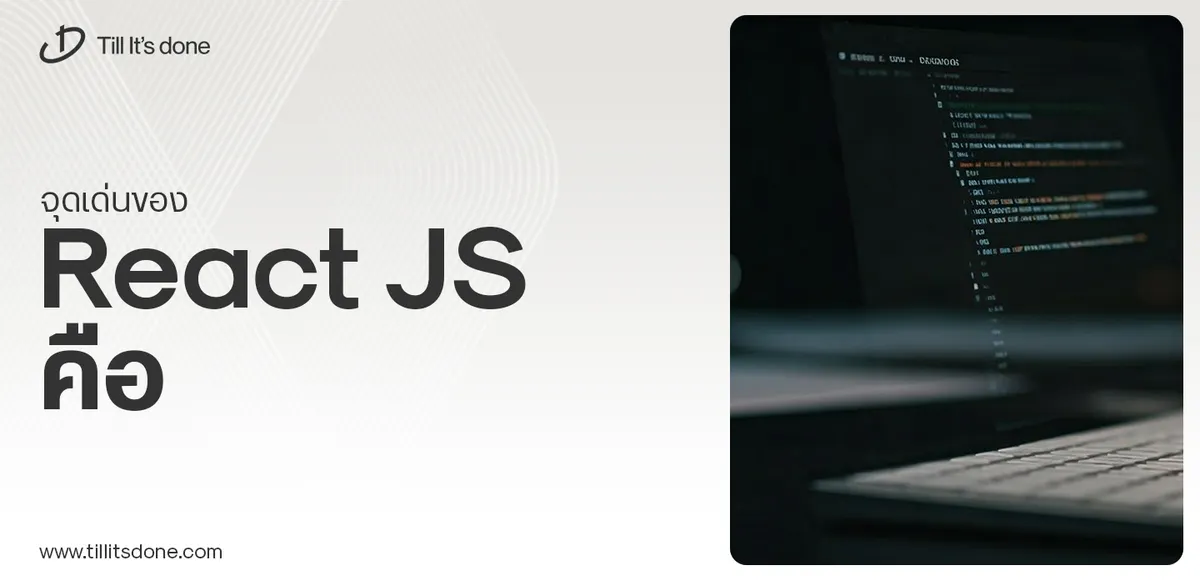
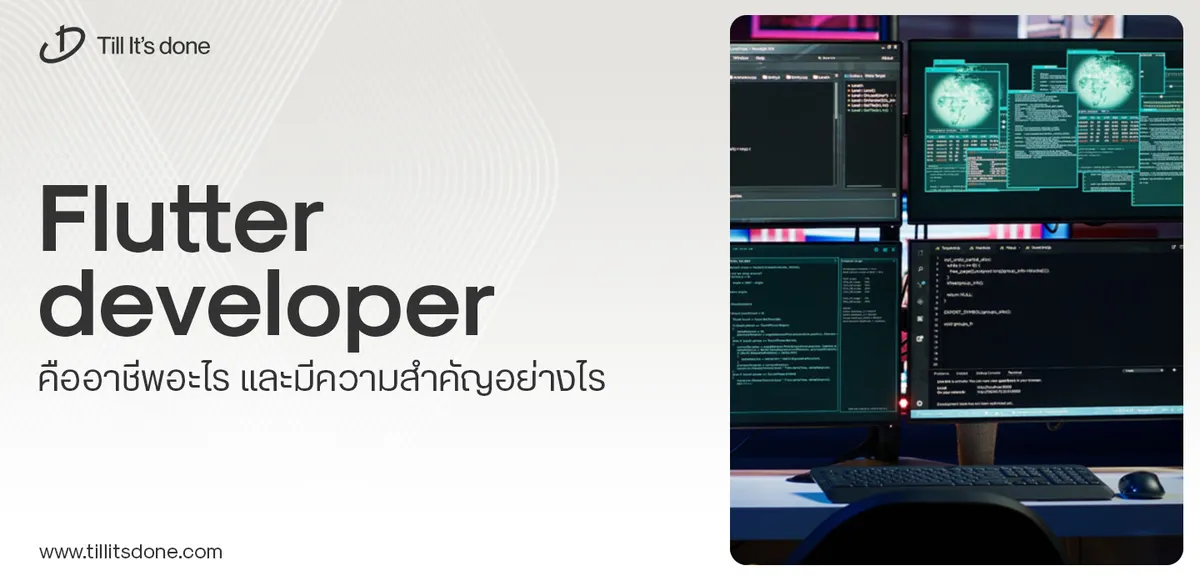
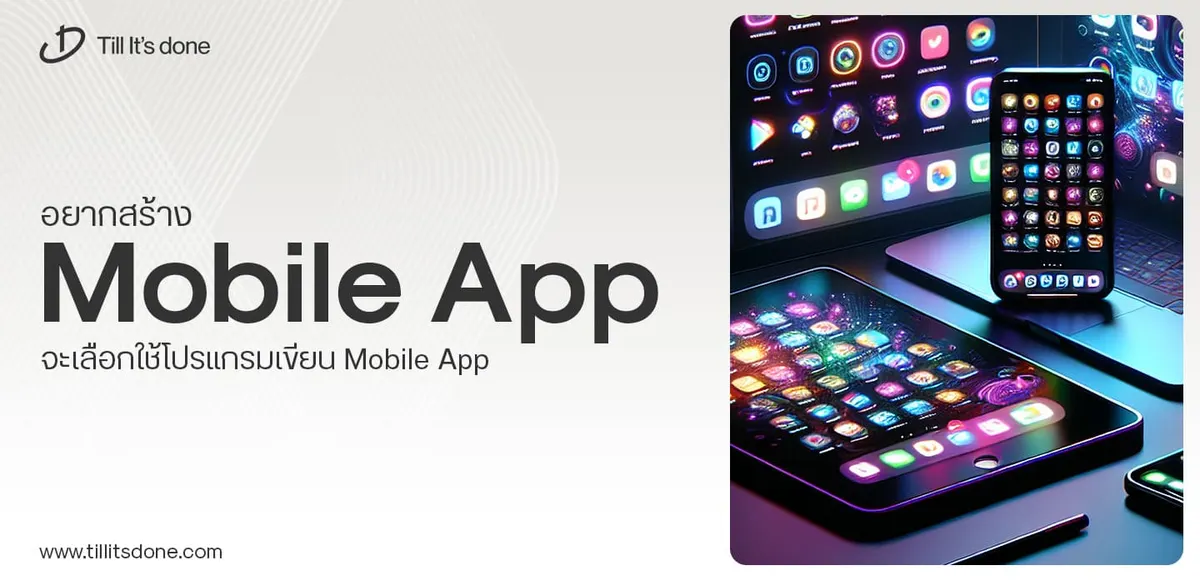
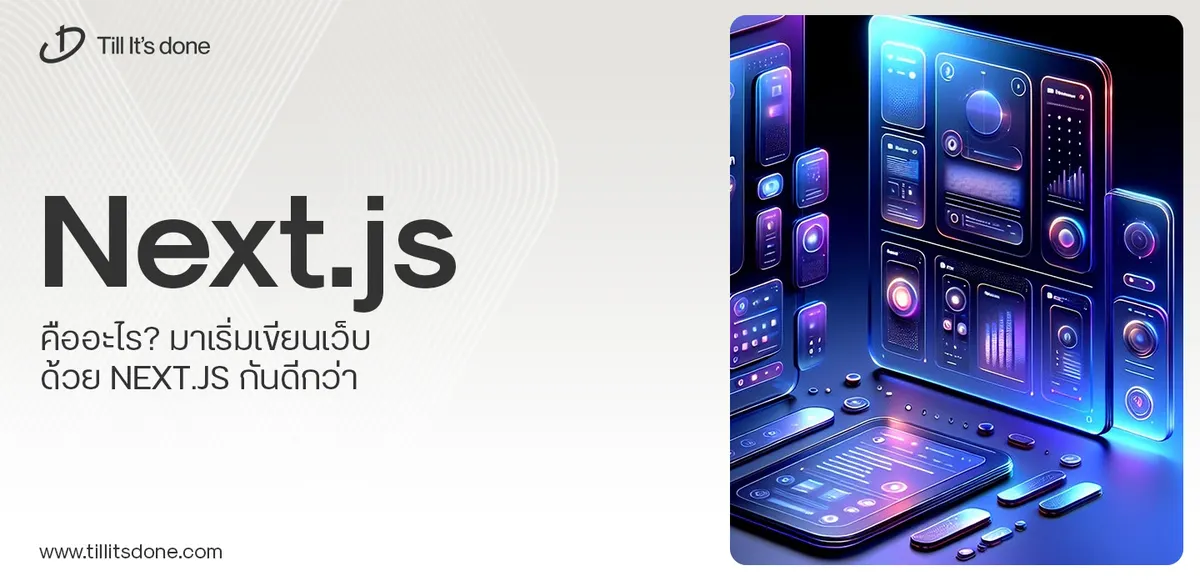
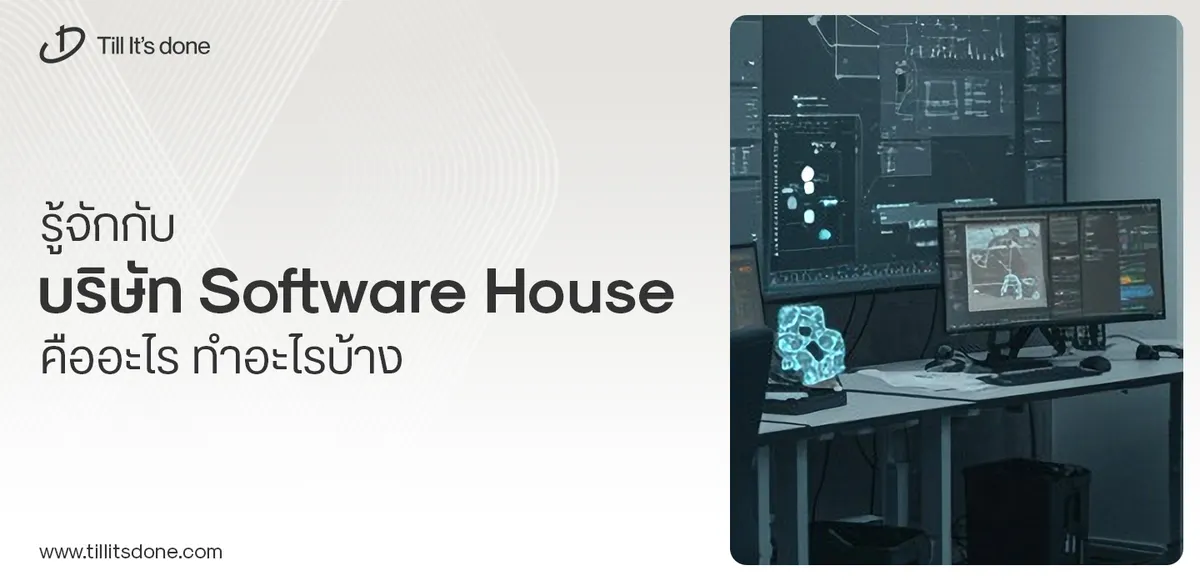
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.