- Services
- Case Studies
- Technologies
- NextJs development
- Flutter development
- NodeJs development
- ReactJs development
- About
- Contact
- Tools
- Blogs
- FAQ
Integrate Semantic UI React with Redux Guide
Discover best practices, code examples, and real-world implementations.
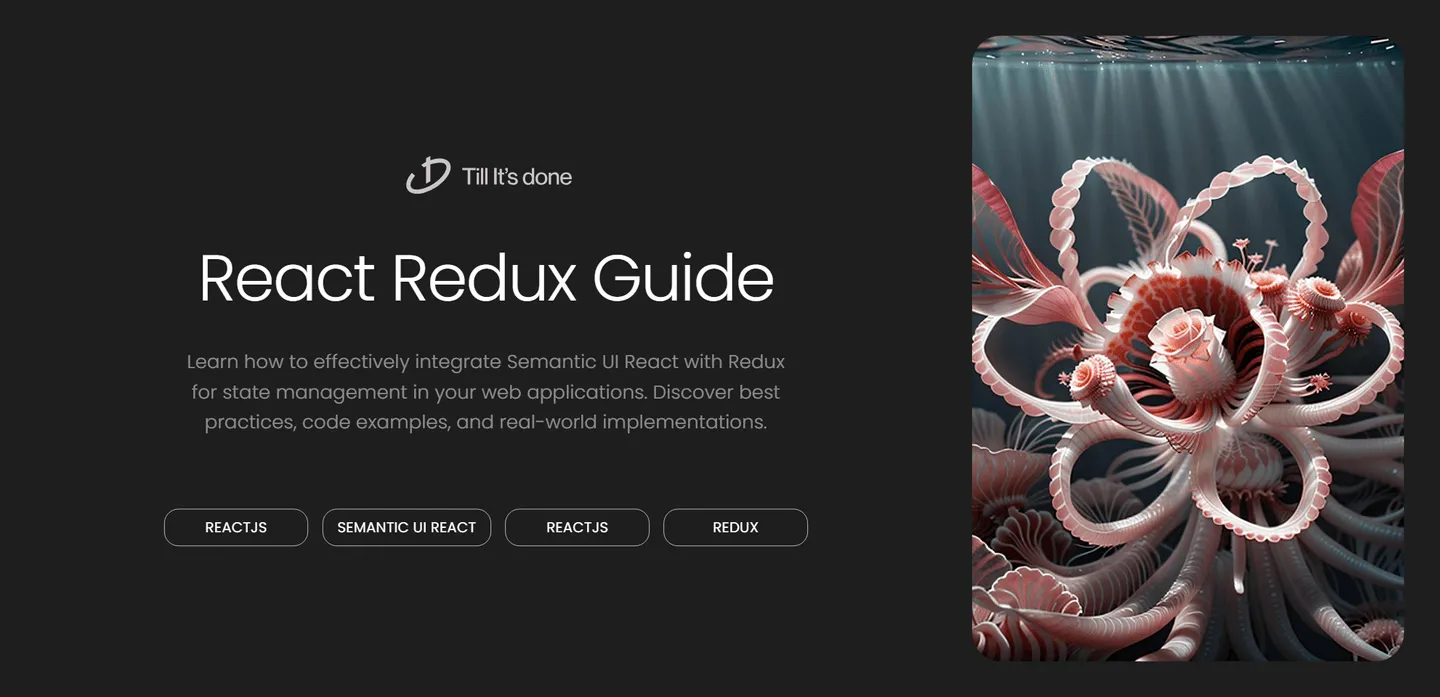
How to Integrate Semantic UI React with Redux for State Management
Redux and Semantic UI React make a powerful combination for building modern web applications. Today, I’ll walk you through integrating these technologies to create a robust and beautiful application with proper state management.
Getting Started
Before diving in, make sure you have your React project set up. The first step is installing the necessary dependencies. You’ll need semantic-ui-react, redux, and react-redux packages.
npm install semantic-ui-react redux react-redux @reduxjs/toolkit
Setting Up Redux Store
First, let’s create a basic Redux store structure. I’ve found this organization works well for most projects:
import { configureStore } from '@reduxjs/toolkit';import uiReducer from './uiSlice';
export const store = configureStore({ reducer: { ui: uiReducer, },});
Creating Redux Slices
One of the things I love about Redux Toolkit is how it simplifies state management. Here’s an example of a slice for managing UI state:
import { createSlice } from '@reduxjs/toolkit';
const uiSlice = createSlice({ name: 'ui', initialState: { activeModal: null, theme: 'light', sidebarVisible: false, }, reducers: { toggleSidebar: (state) => { state.sidebarVisible = !state.sidebarVisible; }, setTheme: (state, action) => { state.theme = action.payload; }, setActiveModal: (state, action) => { state.activeModal = action.payload; }, },});
export const { toggleSidebar, setTheme, setActiveModal } = uiSlice.actions;export default uiSlice.reducer;
Integrating with Semantic UI Components
Now for the fun part - connecting our Redux state to Semantic UI React components. Here’s a practical example:
import React from 'react';import { useSelector, useDispatch } from 'react-redux';import { Sidebar, Menu, Button } from 'semantic-ui-react';import { toggleSidebar } from './store/uiSlice';
const AppSidebar = () => { const dispatch = useDispatch(); const visible = useSelector((state) => state.ui.sidebarVisible);
return ( <> <Button onClick={() => dispatch(toggleSidebar())}> Toggle Sidebar </Button> <Sidebar as={Menu} animation='overlay' vertical visible={visible} width='wide' > {/* Sidebar content */} </Sidebar> </> );};
Best Practices and Tips
Through my experience, I’ve found these practices particularly helpful:
- Use Redux for global state only. Keep component-specific state local using useState.
- Leverage Semantic UI React’s built-in state props for simple interactions.
- Combine Redux actions to handle complex UI state changes.
- Utilize Redux Toolkit’s createAsyncThunk for API calls.
Real-World Example: Theme Switcher
Here’s how you can implement a theme switcher using both technologies:
import React from 'react';import { useSelector, useDispatch } from 'react-redux';import { Dropdown } from 'semantic-ui-react';import { setTheme } from './store/uiSlice';
const ThemeSwitcher = () => { const dispatch = useDispatch(); const currentTheme = useSelector((state) => state.ui.theme);
const themeOptions = [ { key: 'light', text: 'Light Theme', value: 'light' }, { key: 'dark', text: 'Dark Theme', value: 'dark' }, ];
return ( <Dropdown selection value={currentTheme} options={themeOptions} onChange={(_, data) => dispatch(setTheme(data.value))} /> );};
Remember, the key to successful integration is finding the right balance between Redux state management and Semantic UI React’s built-in component states.
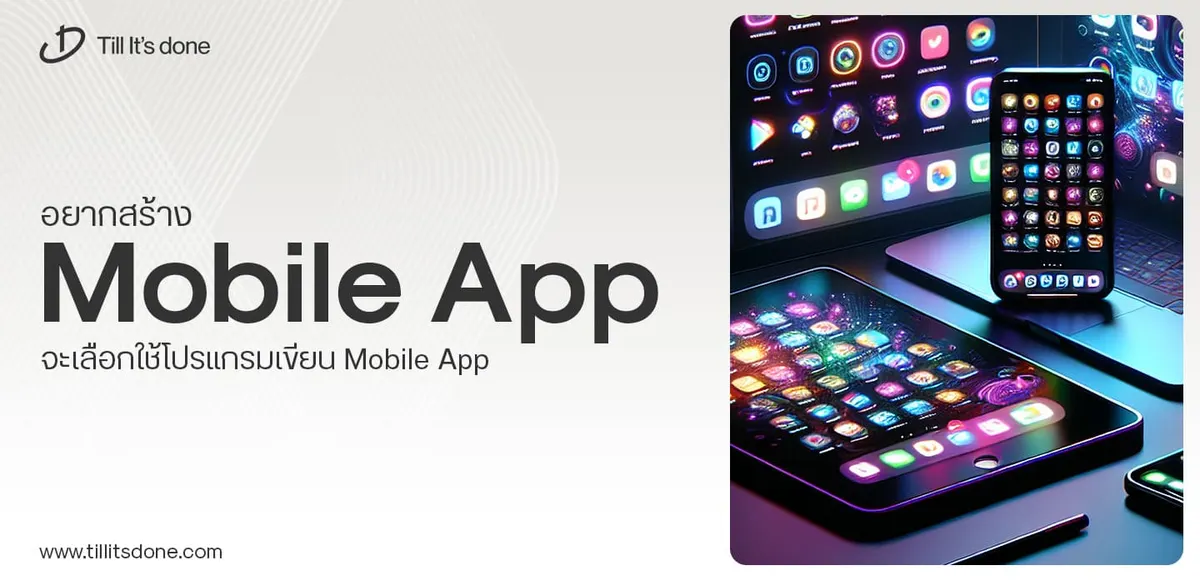
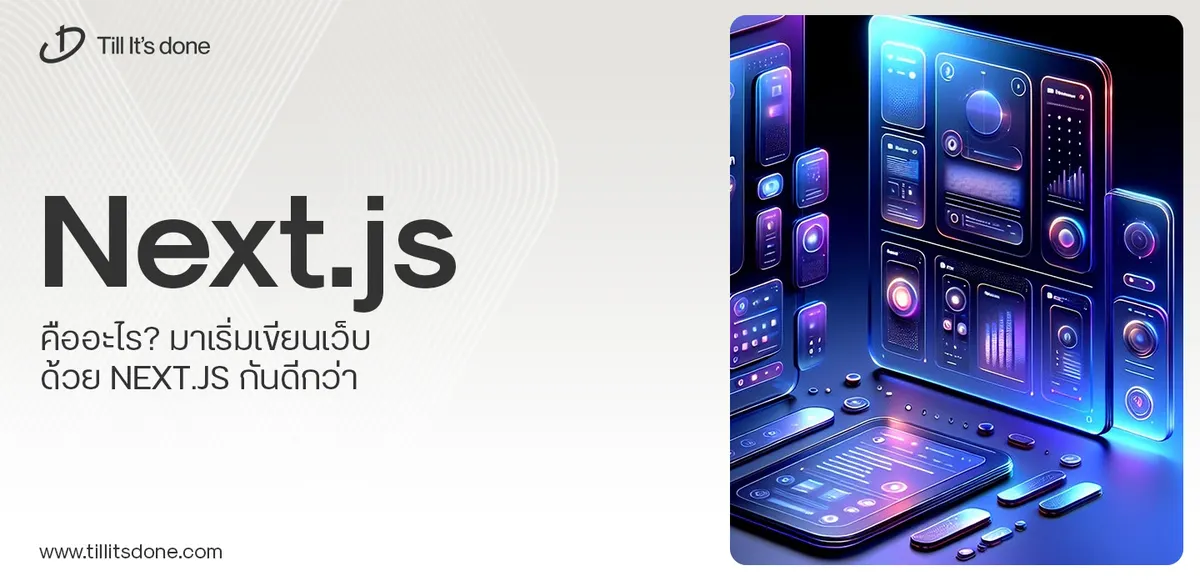
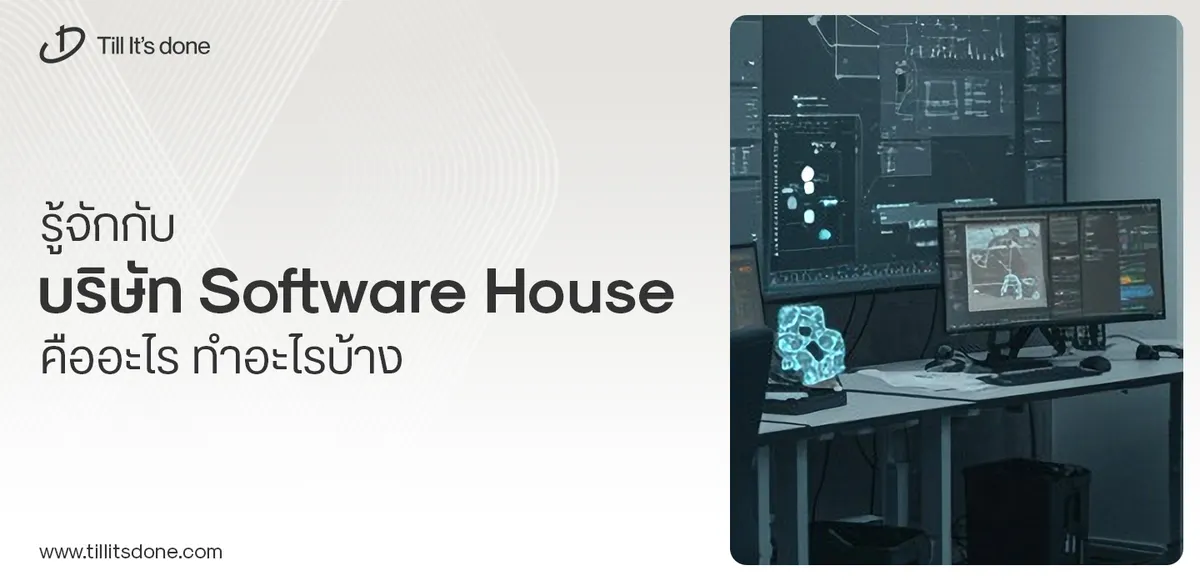
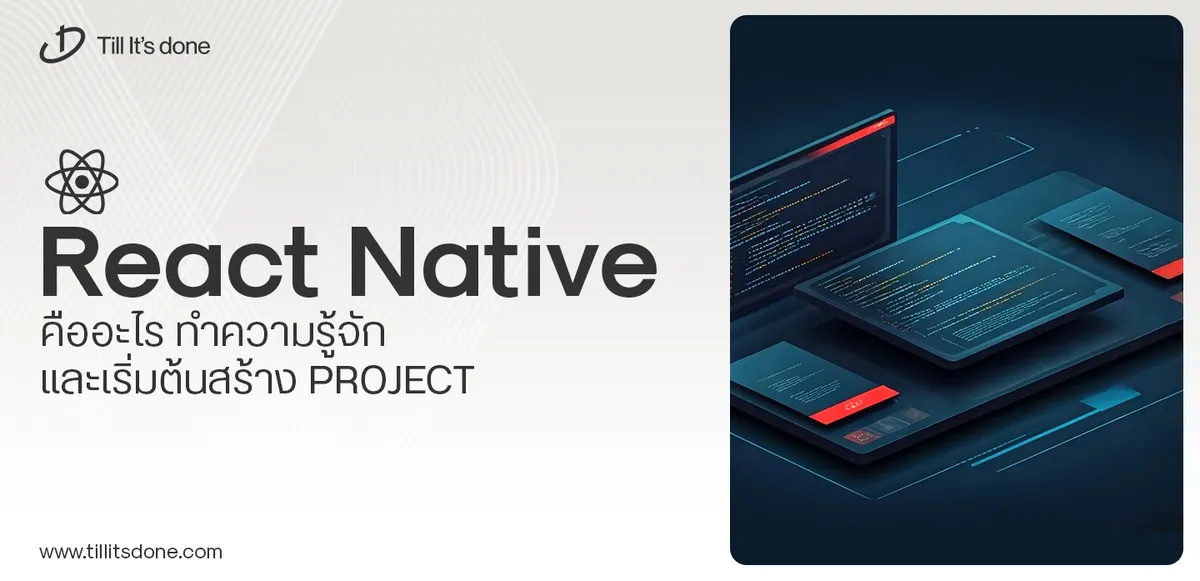
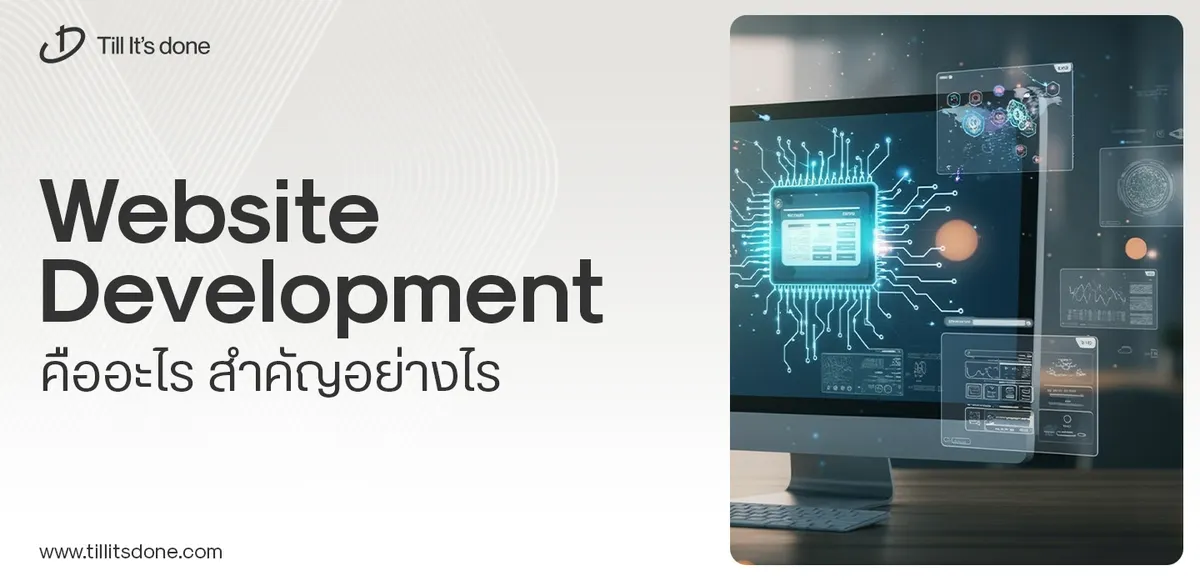
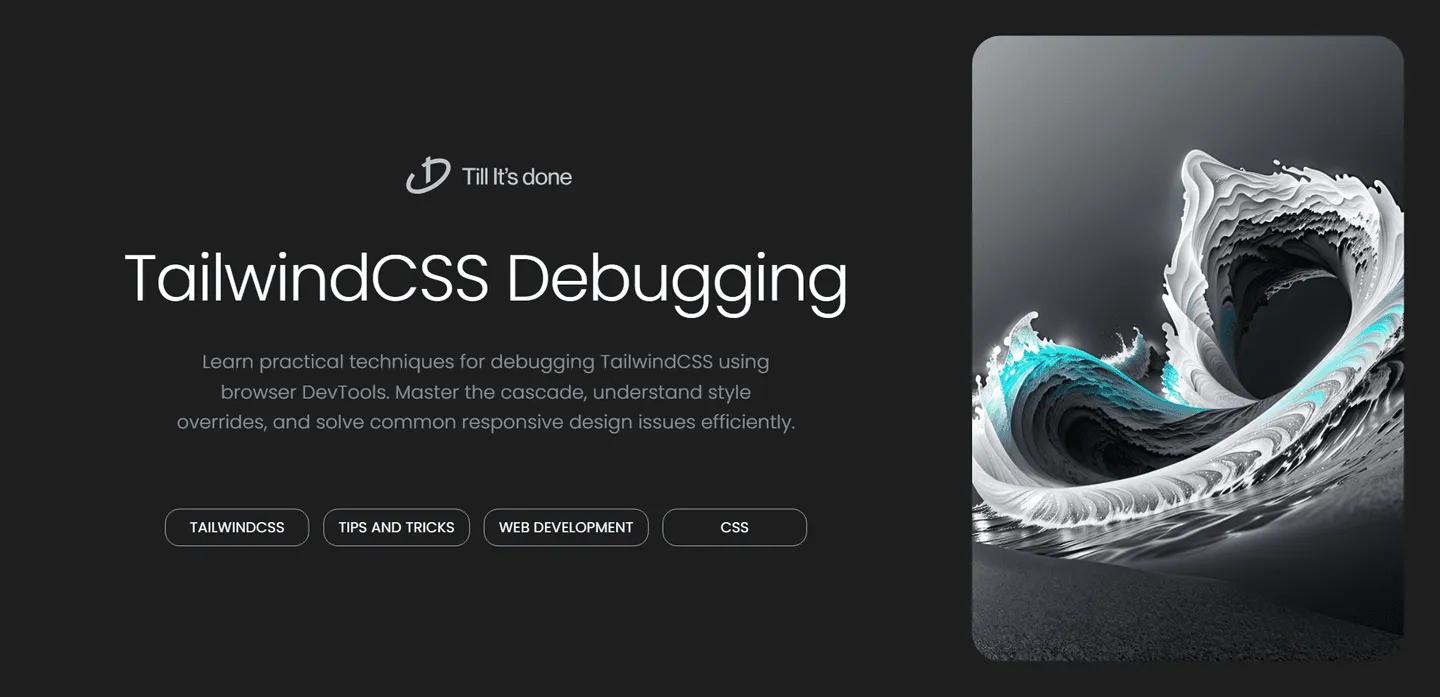
Talk with CEO
We'll be right here with you every step of the way.
We'll be here, prepared to commence this promising collaboration.
Whether you're curious about features, warranties, or shopping policies, we provide comprehensive answers to assist you.